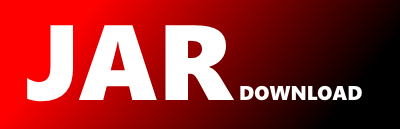
org.graylog2.contentpacks.model.entities.AutoValue_QueryEntity Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog.plugins.views.search.Filter;
import org.graylog.plugins.views.search.GlobalOverride;
import org.graylog.plugins.views.search.engine.BackendQuery;
import org.graylog.plugins.views.search.searchfilters.model.UsedSearchFilter;
import org.graylog2.plugin.indexer.searches.timeranges.TimeRange;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_QueryEntity extends QueryEntity {
private final String id;
private final TimeRange timerange;
@Nullable
private final Filter filter;
private final List filters;
private final BackendQuery query;
private final Optional globalOverride;
private final ImmutableSet searchTypes;
private AutoValue_QueryEntity(
String id,
TimeRange timerange,
@Nullable Filter filter,
List filters,
BackendQuery query,
Optional globalOverride,
ImmutableSet searchTypes) {
this.id = id;
this.timerange = timerange;
this.filter = filter;
this.filters = filters;
this.query = query;
this.globalOverride = globalOverride;
this.searchTypes = searchTypes;
}
@JsonProperty
@Override
public String id() {
return id;
}
@JsonProperty
@Override
public TimeRange timerange() {
return timerange;
}
@JsonProperty
@Nullable
@Override
public Filter filter() {
return filter;
}
@JsonProperty
@Override
public List filters() {
return filters;
}
@JsonProperty
@Nonnull
@Override
public BackendQuery query() {
return query;
}
@JsonIgnore
@Override
public Optional globalOverride() {
return globalOverride;
}
@JsonProperty("search_types")
@Nonnull
@Override
public ImmutableSet searchTypes() {
return searchTypes;
}
@Override
public String toString() {
return "QueryEntity{"
+ "id=" + id + ", "
+ "timerange=" + timerange + ", "
+ "filter=" + filter + ", "
+ "filters=" + filters + ", "
+ "query=" + query + ", "
+ "globalOverride=" + globalOverride + ", "
+ "searchTypes=" + searchTypes
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof QueryEntity) {
QueryEntity that = (QueryEntity) o;
return this.id.equals(that.id())
&& this.timerange.equals(that.timerange())
&& (this.filter == null ? that.filter() == null : this.filter.equals(that.filter()))
&& this.filters.equals(that.filters())
&& this.query.equals(that.query())
&& this.globalOverride.equals(that.globalOverride())
&& this.searchTypes.equals(that.searchTypes());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= timerange.hashCode();
h$ *= 1000003;
h$ ^= (filter == null) ? 0 : filter.hashCode();
h$ *= 1000003;
h$ ^= filters.hashCode();
h$ *= 1000003;
h$ ^= query.hashCode();
h$ *= 1000003;
h$ ^= globalOverride.hashCode();
h$ *= 1000003;
h$ ^= searchTypes.hashCode();
return h$;
}
@Override
public QueryEntity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends QueryEntity.Builder {
private String id;
private TimeRange timerange;
private Filter filter;
private List filters;
private BackendQuery query;
private Optional globalOverride = Optional.empty();
private ImmutableSet searchTypes;
Builder() {
}
private Builder(QueryEntity source) {
this.id = source.id();
this.timerange = source.timerange();
this.filter = source.filter();
this.filters = source.filters();
this.query = source.query();
this.globalOverride = source.globalOverride();
this.searchTypes = source.searchTypes();
}
@Override
public QueryEntity.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public QueryEntity.Builder timerange(TimeRange timerange) {
if (timerange == null) {
throw new NullPointerException("Null timerange");
}
this.timerange = timerange;
return this;
}
@Override
public QueryEntity.Builder filter(Filter filter) {
this.filter = filter;
return this;
}
@Override
public QueryEntity.Builder filters(List filters) {
if (filters == null) {
throw new NullPointerException("Null filters");
}
this.filters = filters;
return this;
}
@Override
public QueryEntity.Builder query(BackendQuery query) {
if (query == null) {
throw new NullPointerException("Null query");
}
this.query = query;
return this;
}
@Override
public QueryEntity.Builder globalOverride(@Nullable GlobalOverride globalOverride) {
this.globalOverride = Optional.ofNullable(globalOverride);
return this;
}
@Override
public QueryEntity.Builder searchTypes(@Nullable Set searchTypes) {
this.searchTypes = ImmutableSet.copyOf(searchTypes);
return this;
}
@Override
QueryEntity autoBuild() {
if (this.id == null
|| this.timerange == null
|| this.filters == null
|| this.query == null
|| this.searchTypes == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.timerange == null) {
missing.append(" timerange");
}
if (this.filters == null) {
missing.append(" filters");
}
if (this.query == null) {
missing.append(" query");
}
if (this.searchTypes == null) {
missing.append(" searchTypes");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_QueryEntity(
this.id,
this.timerange,
this.filter,
this.filters,
this.query,
this.globalOverride,
this.searchTypes);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy