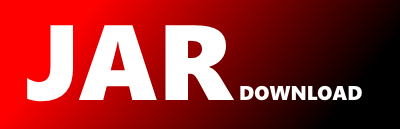
org.graylog2.contentpacks.model.parameters.AutoValue_DoubleParameter Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.parameters;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Optional;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueType;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_DoubleParameter extends DoubleParameter {
private final String name;
private final String title;
private final String description;
private final ValueType valueType;
private final Optional defaultValue;
private AutoValue_DoubleParameter(
String name,
String title,
String description,
ValueType valueType,
Optional defaultValue) {
this.name = name;
this.title = title;
this.description = description;
this.valueType = valueType;
this.defaultValue = defaultValue;
}
@JsonProperty("name")
@Override
public String name() {
return name;
}
@JsonProperty("title")
@Override
public String title() {
return title;
}
@JsonProperty("description")
@Override
public String description() {
return description;
}
@JsonProperty("type")
@Override
public ValueType valueType() {
return valueType;
}
@JsonProperty("default_value")
@Override
public Optional defaultValue() {
return defaultValue;
}
@Override
public String toString() {
return "DoubleParameter{"
+ "name=" + name + ", "
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "valueType=" + valueType + ", "
+ "defaultValue=" + defaultValue
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof DoubleParameter) {
DoubleParameter that = (DoubleParameter) o;
return this.name.equals(that.name())
&& this.title.equals(that.title())
&& this.description.equals(that.description())
&& this.valueType.equals(that.valueType())
&& this.defaultValue.equals(that.defaultValue());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= valueType.hashCode();
h$ *= 1000003;
h$ ^= defaultValue.hashCode();
return h$;
}
static final class Builder extends DoubleParameter.Builder {
private String name;
private String title;
private String description;
private ValueType valueType;
private Optional defaultValue = Optional.empty();
Builder() {
}
@Override
public DoubleParameter.Builder name(String name) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
return this;
}
@Override
public DoubleParameter.Builder title(String title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public DoubleParameter.Builder description(String description) {
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
return this;
}
@Override
public DoubleParameter.Builder valueType(ValueType valueType) {
if (valueType == null) {
throw new NullPointerException("Null valueType");
}
this.valueType = valueType;
return this;
}
@Override
public DoubleParameter.Builder defaultValue(Optional defaultValue) {
if (defaultValue == null) {
throw new NullPointerException("Null defaultValue");
}
this.defaultValue = defaultValue;
return this;
}
@Override
DoubleParameter autoBuild() {
if (this.name == null
|| this.title == null
|| this.description == null
|| this.valueType == null) {
StringBuilder missing = new StringBuilder();
if (this.name == null) {
missing.append(" name");
}
if (this.title == null) {
missing.append(" title");
}
if (this.description == null) {
missing.append(" description");
}
if (this.valueType == null) {
missing.append(" valueType");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_DoubleParameter(
this.name,
this.title,
this.description,
this.valueType,
this.defaultValue);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy