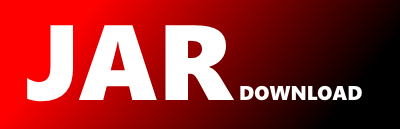
org.graylog2.dashboards.widgets.AutoValue_WidgetPosition Maven / Gradle / Ivy
package org.graylog2.dashboards.widgets;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_WidgetPosition extends WidgetPosition {
private final String id;
private final int width;
private final int height;
private final int col;
private final int row;
private AutoValue_WidgetPosition(
String id,
int width,
int height,
int col,
int row) {
this.id = id;
this.width = width;
this.height = height;
this.col = col;
this.row = row;
}
@JsonProperty("id")
@Override
public String id() {
return id;
}
@JsonProperty("width")
@Override
public int width() {
return width;
}
@JsonProperty("height")
@Override
public int height() {
return height;
}
@JsonProperty("col")
@Override
public int col() {
return col;
}
@JsonProperty("row")
@Override
public int row() {
return row;
}
@Override
public String toString() {
return "WidgetPosition{"
+ "id=" + id + ", "
+ "width=" + width + ", "
+ "height=" + height + ", "
+ "col=" + col + ", "
+ "row=" + row
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof WidgetPosition) {
WidgetPosition that = (WidgetPosition) o;
return this.id.equals(that.id())
&& this.width == that.width()
&& this.height == that.height()
&& this.col == that.col()
&& this.row == that.row();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= width;
h$ *= 1000003;
h$ ^= height;
h$ *= 1000003;
h$ ^= col;
h$ *= 1000003;
h$ ^= row;
return h$;
}
@Override
public WidgetPosition.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends WidgetPosition.Builder {
private String id;
private int width;
private int height;
private int col;
private int row;
private byte set$0;
Builder() {
}
private Builder(WidgetPosition source) {
this.id = source.id();
this.width = source.width();
this.height = source.height();
this.col = source.col();
this.row = source.row();
set$0 = (byte) 0xf;
}
@Override
public WidgetPosition.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public WidgetPosition.Builder width(int width) {
this.width = width;
set$0 |= (byte) 1;
return this;
}
@Override
public WidgetPosition.Builder height(int height) {
this.height = height;
set$0 |= (byte) 2;
return this;
}
@Override
public WidgetPosition.Builder col(int col) {
this.col = col;
set$0 |= (byte) 4;
return this;
}
@Override
public WidgetPosition.Builder row(int row) {
this.row = row;
set$0 |= (byte) 8;
return this;
}
@Override
public WidgetPosition build() {
if (set$0 != 0xf
|| this.id == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if ((set$0 & 1) == 0) {
missing.append(" width");
}
if ((set$0 & 2) == 0) {
missing.append(" height");
}
if ((set$0 & 4) == 0) {
missing.append(" col");
}
if ((set$0 & 8) == 0) {
missing.append(" row");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_WidgetPosition(
this.id,
this.width,
this.height,
this.col,
this.row);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy