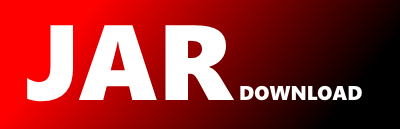
org.graylog2.indexer.indexset.$AutoValue_IndexSetConfig Maven / Gradle / Ivy
package org.graylog2.indexer.indexset;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.ZonedDateTime;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import org.graylog2.plugin.indexer.retention.RetentionStrategyConfig;
import org.graylog2.plugin.indexer.rotation.RotationStrategyConfig;
import org.graylog2.validation.SizeInBytes;
import org.joda.time.Duration;
import org.mongojack.Id;
import org.mongojack.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_IndexSetConfig extends IndexSetConfig {
@Nullable
private final String id;
private final @NotBlank String title;
@Nullable
private final String description;
private final boolean isWritable;
private final Optional isRegular;
private final @NotBlank @Pattern(regexp = "^[a-z0-9][a-z0-9_+-]*$") @SizeInBytes(message = "Index prefix must have a length in bytes between {min} and {max}", min = 1, max = 250) String indexPrefix;
@Nullable
private final String indexMatchPattern;
@Nullable
private final String indexWildcard;
private final @Min(1L) int shards;
private final @Min(0L) int replicas;
@Nullable
private final String rotationStrategyClass;
private final @NotNull RotationStrategyConfig rotationStrategy;
@Nullable
private final String retentionStrategyClass;
private final @NotNull RetentionStrategyConfig retentionStrategy;
private final @NotNull ZonedDateTime creationDate;
private final @NotBlank String indexAnalyzer;
private final @NotBlank String indexTemplateName;
private final @NotBlank Optional indexTemplateType;
private final @Min(1L) int indexOptimizationMaxNumSegments;
private final boolean indexOptimizationDisabled;
private final Duration fieldTypeRefreshInterval;
private final CustomFieldMappings customFieldMappings;
$AutoValue_IndexSetConfig(
@Nullable String id,
@NotBlank String title,
@Nullable String description,
boolean isWritable,
Optional isRegular,
@NotBlank @Pattern(regexp = "^[a-z0-9][a-z0-9_+-]*$") @SizeInBytes(message = "Index prefix must have a length in bytes between {min} and {max}", min = 1, max = 250) String indexPrefix,
@Nullable String indexMatchPattern,
@Nullable String indexWildcard,
@Min(1L) int shards,
@Min(0L) int replicas,
@Nullable String rotationStrategyClass,
@NotNull RotationStrategyConfig rotationStrategy,
@Nullable String retentionStrategyClass,
@NotNull RetentionStrategyConfig retentionStrategy,
@NotNull ZonedDateTime creationDate,
@NotBlank String indexAnalyzer,
@NotBlank String indexTemplateName,
@NotBlank Optional indexTemplateType,
@Min(1L) int indexOptimizationMaxNumSegments,
boolean indexOptimizationDisabled,
Duration fieldTypeRefreshInterval,
CustomFieldMappings customFieldMappings) {
this.id = id;
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
this.description = description;
this.isWritable = isWritable;
if (isRegular == null) {
throw new NullPointerException("Null isRegular");
}
this.isRegular = isRegular;
if (indexPrefix == null) {
throw new NullPointerException("Null indexPrefix");
}
this.indexPrefix = indexPrefix;
this.indexMatchPattern = indexMatchPattern;
this.indexWildcard = indexWildcard;
this.shards = shards;
this.replicas = replicas;
this.rotationStrategyClass = rotationStrategyClass;
if (rotationStrategy == null) {
throw new NullPointerException("Null rotationStrategy");
}
this.rotationStrategy = rotationStrategy;
this.retentionStrategyClass = retentionStrategyClass;
if (retentionStrategy == null) {
throw new NullPointerException("Null retentionStrategy");
}
this.retentionStrategy = retentionStrategy;
if (creationDate == null) {
throw new NullPointerException("Null creationDate");
}
this.creationDate = creationDate;
if (indexAnalyzer == null) {
throw new NullPointerException("Null indexAnalyzer");
}
this.indexAnalyzer = indexAnalyzer;
if (indexTemplateName == null) {
throw new NullPointerException("Null indexTemplateName");
}
this.indexTemplateName = indexTemplateName;
if (indexTemplateType == null) {
throw new NullPointerException("Null indexTemplateType");
}
this.indexTemplateType = indexTemplateType;
this.indexOptimizationMaxNumSegments = indexOptimizationMaxNumSegments;
this.indexOptimizationDisabled = indexOptimizationDisabled;
if (fieldTypeRefreshInterval == null) {
throw new NullPointerException("Null fieldTypeRefreshInterval");
}
this.fieldTypeRefreshInterval = fieldTypeRefreshInterval;
if (customFieldMappings == null) {
throw new NullPointerException("Null customFieldMappings");
}
this.customFieldMappings = customFieldMappings;
}
@JsonProperty("id")
@Nullable
@Id
@ObjectId
@Override
public String id() {
return id;
}
@JsonProperty("title")
@Override
public @NotBlank String title() {
return title;
}
@JsonProperty("description")
@Nullable
@Override
public String description() {
return description;
}
@JsonProperty("writable")
@Override
public boolean isWritable() {
return isWritable;
}
@JsonProperty("regular")
@Override
public Optional isRegular() {
return isRegular;
}
@JsonProperty("index_prefix")
@Override
public @NotBlank @Pattern(regexp = "^[a-z0-9][a-z0-9_+-]*$") @SizeInBytes(message = "Index prefix must have a length in bytes between {min} and {max}", min = 1, max = 250) String indexPrefix() {
return indexPrefix;
}
@JsonProperty("index_match_pattern")
@Nullable
@Override
public String indexMatchPattern() {
return indexMatchPattern;
}
@JsonProperty("index_wildcard")
@Nullable
@Override
public String indexWildcard() {
return indexWildcard;
}
@JsonProperty("shards")
@Override
public @Min(1L) int shards() {
return shards;
}
@JsonProperty("replicas")
@Override
public @Min(0L) int replicas() {
return replicas;
}
@JsonProperty("rotation_strategy_class")
@Nullable
@Override
public String rotationStrategyClass() {
return rotationStrategyClass;
}
@JsonProperty("rotation_strategy")
@Override
public @NotNull RotationStrategyConfig rotationStrategy() {
return rotationStrategy;
}
@JsonProperty("retention_strategy_class")
@Nullable
@Override
public String retentionStrategyClass() {
return retentionStrategyClass;
}
@JsonProperty("retention_strategy")
@Override
public @NotNull RetentionStrategyConfig retentionStrategy() {
return retentionStrategy;
}
@JsonProperty("creation_date")
@Override
public @NotNull ZonedDateTime creationDate() {
return creationDate;
}
@JsonProperty("index_analyzer")
@Override
public @NotBlank String indexAnalyzer() {
return indexAnalyzer;
}
@JsonProperty("index_template_name")
@Override
public @NotBlank String indexTemplateName() {
return indexTemplateName;
}
@JsonProperty("index_template_type")
@Override
public @NotBlank Optional indexTemplateType() {
return indexTemplateType;
}
@JsonProperty("index_optimization_max_num_segments")
@Override
public @Min(1L) int indexOptimizationMaxNumSegments() {
return indexOptimizationMaxNumSegments;
}
@JsonProperty("index_optimization_disabled")
@Override
public boolean indexOptimizationDisabled() {
return indexOptimizationDisabled;
}
@JsonProperty("field_type_refresh_interval")
@Override
public Duration fieldTypeRefreshInterval() {
return fieldTypeRefreshInterval;
}
@JsonProperty("custom_field_mappings")
@Override
public CustomFieldMappings customFieldMappings() {
return customFieldMappings;
}
@Override
public String toString() {
return "IndexSetConfig{"
+ "id=" + id + ", "
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "isWritable=" + isWritable + ", "
+ "isRegular=" + isRegular + ", "
+ "indexPrefix=" + indexPrefix + ", "
+ "indexMatchPattern=" + indexMatchPattern + ", "
+ "indexWildcard=" + indexWildcard + ", "
+ "shards=" + shards + ", "
+ "replicas=" + replicas + ", "
+ "rotationStrategyClass=" + rotationStrategyClass + ", "
+ "rotationStrategy=" + rotationStrategy + ", "
+ "retentionStrategyClass=" + retentionStrategyClass + ", "
+ "retentionStrategy=" + retentionStrategy + ", "
+ "creationDate=" + creationDate + ", "
+ "indexAnalyzer=" + indexAnalyzer + ", "
+ "indexTemplateName=" + indexTemplateName + ", "
+ "indexTemplateType=" + indexTemplateType + ", "
+ "indexOptimizationMaxNumSegments=" + indexOptimizationMaxNumSegments + ", "
+ "indexOptimizationDisabled=" + indexOptimizationDisabled + ", "
+ "fieldTypeRefreshInterval=" + fieldTypeRefreshInterval + ", "
+ "customFieldMappings=" + customFieldMappings
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IndexSetConfig) {
IndexSetConfig that = (IndexSetConfig) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.title.equals(that.title())
&& (this.description == null ? that.description() == null : this.description.equals(that.description()))
&& this.isWritable == that.isWritable()
&& this.isRegular.equals(that.isRegular())
&& this.indexPrefix.equals(that.indexPrefix())
&& (this.indexMatchPattern == null ? that.indexMatchPattern() == null : this.indexMatchPattern.equals(that.indexMatchPattern()))
&& (this.indexWildcard == null ? that.indexWildcard() == null : this.indexWildcard.equals(that.indexWildcard()))
&& this.shards == that.shards()
&& this.replicas == that.replicas()
&& (this.rotationStrategyClass == null ? that.rotationStrategyClass() == null : this.rotationStrategyClass.equals(that.rotationStrategyClass()))
&& this.rotationStrategy.equals(that.rotationStrategy())
&& (this.retentionStrategyClass == null ? that.retentionStrategyClass() == null : this.retentionStrategyClass.equals(that.retentionStrategyClass()))
&& this.retentionStrategy.equals(that.retentionStrategy())
&& this.creationDate.equals(that.creationDate())
&& this.indexAnalyzer.equals(that.indexAnalyzer())
&& this.indexTemplateName.equals(that.indexTemplateName())
&& this.indexTemplateType.equals(that.indexTemplateType())
&& this.indexOptimizationMaxNumSegments == that.indexOptimizationMaxNumSegments()
&& this.indexOptimizationDisabled == that.indexOptimizationDisabled()
&& this.fieldTypeRefreshInterval.equals(that.fieldTypeRefreshInterval())
&& this.customFieldMappings.equals(that.customFieldMappings());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= (description == null) ? 0 : description.hashCode();
h$ *= 1000003;
h$ ^= isWritable ? 1231 : 1237;
h$ *= 1000003;
h$ ^= isRegular.hashCode();
h$ *= 1000003;
h$ ^= indexPrefix.hashCode();
h$ *= 1000003;
h$ ^= (indexMatchPattern == null) ? 0 : indexMatchPattern.hashCode();
h$ *= 1000003;
h$ ^= (indexWildcard == null) ? 0 : indexWildcard.hashCode();
h$ *= 1000003;
h$ ^= shards;
h$ *= 1000003;
h$ ^= replicas;
h$ *= 1000003;
h$ ^= (rotationStrategyClass == null) ? 0 : rotationStrategyClass.hashCode();
h$ *= 1000003;
h$ ^= rotationStrategy.hashCode();
h$ *= 1000003;
h$ ^= (retentionStrategyClass == null) ? 0 : retentionStrategyClass.hashCode();
h$ *= 1000003;
h$ ^= retentionStrategy.hashCode();
h$ *= 1000003;
h$ ^= creationDate.hashCode();
h$ *= 1000003;
h$ ^= indexAnalyzer.hashCode();
h$ *= 1000003;
h$ ^= indexTemplateName.hashCode();
h$ *= 1000003;
h$ ^= indexTemplateType.hashCode();
h$ *= 1000003;
h$ ^= indexOptimizationMaxNumSegments;
h$ *= 1000003;
h$ ^= indexOptimizationDisabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= fieldTypeRefreshInterval.hashCode();
h$ *= 1000003;
h$ ^= customFieldMappings.hashCode();
return h$;
}
@Override
public IndexSetConfig.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends IndexSetConfig.Builder {
private String id;
private @NotBlank String title;
private String description;
private boolean isWritable;
private Optional isRegular = Optional.empty();
private @NotBlank @Pattern(regexp = "^[a-z0-9][a-z0-9_+-]*$") @SizeInBytes(message = "Index prefix must have a length in bytes between {min} and {max}", min = 1, max = 250) String indexPrefix;
private String indexMatchPattern;
private String indexWildcard;
private @Min(1L) int shards;
private @Min(0L) int replicas;
private String rotationStrategyClass;
private @NotNull RotationStrategyConfig rotationStrategy;
private String retentionStrategyClass;
private @NotNull RetentionStrategyConfig retentionStrategy;
private @NotNull ZonedDateTime creationDate;
private @NotBlank String indexAnalyzer;
private @NotBlank String indexTemplateName;
private @NotBlank Optional indexTemplateType = Optional.empty();
private @Min(1L) int indexOptimizationMaxNumSegments;
private boolean indexOptimizationDisabled;
private Duration fieldTypeRefreshInterval;
private CustomFieldMappings customFieldMappings;
private byte set$0;
Builder() {
}
private Builder(IndexSetConfig source) {
this.id = source.id();
this.title = source.title();
this.description = source.description();
this.isWritable = source.isWritable();
this.isRegular = source.isRegular();
this.indexPrefix = source.indexPrefix();
this.indexMatchPattern = source.indexMatchPattern();
this.indexWildcard = source.indexWildcard();
this.shards = source.shards();
this.replicas = source.replicas();
this.rotationStrategyClass = source.rotationStrategyClass();
this.rotationStrategy = source.rotationStrategy();
this.retentionStrategyClass = source.retentionStrategyClass();
this.retentionStrategy = source.retentionStrategy();
this.creationDate = source.creationDate();
this.indexAnalyzer = source.indexAnalyzer();
this.indexTemplateName = source.indexTemplateName();
this.indexTemplateType = source.indexTemplateType();
this.indexOptimizationMaxNumSegments = source.indexOptimizationMaxNumSegments();
this.indexOptimizationDisabled = source.indexOptimizationDisabled();
this.fieldTypeRefreshInterval = source.fieldTypeRefreshInterval();
this.customFieldMappings = source.customFieldMappings();
set$0 = (byte) 0x1f;
}
@Override
public IndexSetConfig.Builder id(String id) {
this.id = id;
return this;
}
@Override
public IndexSetConfig.Builder title(String title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public IndexSetConfig.Builder description(String description) {
this.description = description;
return this;
}
@Override
public IndexSetConfig.Builder isWritable(boolean isWritable) {
this.isWritable = isWritable;
set$0 |= (byte) 1;
return this;
}
@Override
public IndexSetConfig.Builder isRegular(@Nullable Boolean isRegular) {
this.isRegular = Optional.ofNullable(isRegular);
return this;
}
@Override
public IndexSetConfig.Builder indexPrefix(String indexPrefix) {
if (indexPrefix == null) {
throw new NullPointerException("Null indexPrefix");
}
this.indexPrefix = indexPrefix;
return this;
}
@Override
public IndexSetConfig.Builder indexMatchPattern(String indexMatchPattern) {
this.indexMatchPattern = indexMatchPattern;
return this;
}
@Override
public IndexSetConfig.Builder indexWildcard(String indexWildcard) {
this.indexWildcard = indexWildcard;
return this;
}
@Override
public IndexSetConfig.Builder shards(int shards) {
this.shards = shards;
set$0 |= (byte) 2;
return this;
}
@Override
public IndexSetConfig.Builder replicas(int replicas) {
this.replicas = replicas;
set$0 |= (byte) 4;
return this;
}
@Override
public IndexSetConfig.Builder rotationStrategyClass(String rotationStrategyClass) {
this.rotationStrategyClass = rotationStrategyClass;
return this;
}
@Override
public IndexSetConfig.Builder rotationStrategy(RotationStrategyConfig rotationStrategy) {
if (rotationStrategy == null) {
throw new NullPointerException("Null rotationStrategy");
}
this.rotationStrategy = rotationStrategy;
return this;
}
@Override
public IndexSetConfig.Builder retentionStrategyClass(String retentionStrategyClass) {
this.retentionStrategyClass = retentionStrategyClass;
return this;
}
@Override
public IndexSetConfig.Builder retentionStrategy(RetentionStrategyConfig retentionStrategy) {
if (retentionStrategy == null) {
throw new NullPointerException("Null retentionStrategy");
}
this.retentionStrategy = retentionStrategy;
return this;
}
@Override
public IndexSetConfig.Builder creationDate(ZonedDateTime creationDate) {
if (creationDate == null) {
throw new NullPointerException("Null creationDate");
}
this.creationDate = creationDate;
return this;
}
@Override
public IndexSetConfig.Builder indexAnalyzer(String indexAnalyzer) {
if (indexAnalyzer == null) {
throw new NullPointerException("Null indexAnalyzer");
}
this.indexAnalyzer = indexAnalyzer;
return this;
}
@Override
public IndexSetConfig.Builder indexTemplateName(String indexTemplateName) {
if (indexTemplateName == null) {
throw new NullPointerException("Null indexTemplateName");
}
this.indexTemplateName = indexTemplateName;
return this;
}
@Override
public IndexSetConfig.Builder indexTemplateType(@Nullable String indexTemplateType) {
this.indexTemplateType = Optional.ofNullable(indexTemplateType);
return this;
}
@Override
public IndexSetConfig.Builder indexOptimizationMaxNumSegments(int indexOptimizationMaxNumSegments) {
this.indexOptimizationMaxNumSegments = indexOptimizationMaxNumSegments;
set$0 |= (byte) 8;
return this;
}
@Override
public IndexSetConfig.Builder indexOptimizationDisabled(boolean indexOptimizationDisabled) {
this.indexOptimizationDisabled = indexOptimizationDisabled;
set$0 |= (byte) 0x10;
return this;
}
@Override
public IndexSetConfig.Builder fieldTypeRefreshInterval(Duration fieldTypeRefreshInterval) {
if (fieldTypeRefreshInterval == null) {
throw new NullPointerException("Null fieldTypeRefreshInterval");
}
this.fieldTypeRefreshInterval = fieldTypeRefreshInterval;
return this;
}
@Override
public IndexSetConfig.Builder customFieldMappings(CustomFieldMappings customFieldMappings) {
if (customFieldMappings == null) {
throw new NullPointerException("Null customFieldMappings");
}
this.customFieldMappings = customFieldMappings;
return this;
}
@Override
public IndexSetConfig build() {
if (set$0 != 0x1f
|| this.title == null
|| this.indexPrefix == null
|| this.rotationStrategy == null
|| this.retentionStrategy == null
|| this.creationDate == null
|| this.indexAnalyzer == null
|| this.indexTemplateName == null
|| this.fieldTypeRefreshInterval == null
|| this.customFieldMappings == null) {
StringBuilder missing = new StringBuilder();
if (this.title == null) {
missing.append(" title");
}
if ((set$0 & 1) == 0) {
missing.append(" isWritable");
}
if (this.indexPrefix == null) {
missing.append(" indexPrefix");
}
if ((set$0 & 2) == 0) {
missing.append(" shards");
}
if ((set$0 & 4) == 0) {
missing.append(" replicas");
}
if (this.rotationStrategy == null) {
missing.append(" rotationStrategy");
}
if (this.retentionStrategy == null) {
missing.append(" retentionStrategy");
}
if (this.creationDate == null) {
missing.append(" creationDate");
}
if (this.indexAnalyzer == null) {
missing.append(" indexAnalyzer");
}
if (this.indexTemplateName == null) {
missing.append(" indexTemplateName");
}
if ((set$0 & 8) == 0) {
missing.append(" indexOptimizationMaxNumSegments");
}
if ((set$0 & 0x10) == 0) {
missing.append(" indexOptimizationDisabled");
}
if (this.fieldTypeRefreshInterval == null) {
missing.append(" fieldTypeRefreshInterval");
}
if (this.customFieldMappings == null) {
missing.append(" customFieldMappings");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_IndexSetConfig(
this.id,
this.title,
this.description,
this.isWritable,
this.isRegular,
this.indexPrefix,
this.indexMatchPattern,
this.indexWildcard,
this.shards,
this.replicas,
this.rotationStrategyClass,
this.rotationStrategy,
this.retentionStrategyClass,
this.retentionStrategy,
this.creationDate,
this.indexAnalyzer,
this.indexTemplateName,
this.indexTemplateType,
this.indexOptimizationMaxNumSegments,
this.indexOptimizationDisabled,
this.fieldTypeRefreshInterval,
this.customFieldMappings);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy