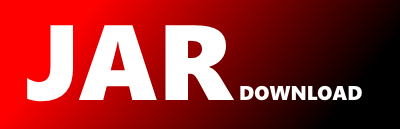
org.graylog2.indexer.ranges.$AutoValue_MongoIndexRange Maven / Gradle / Ivy
package org.graylog2.indexer.ranges;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.bson.types.ObjectId;
import org.joda.time.DateTime;
import org.mongojack.Id;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_MongoIndexRange extends MongoIndexRange {
@Nullable
private final ObjectId id;
private final String indexName;
private final DateTime begin;
private final DateTime end;
private final DateTime calculatedAt;
private final int calculationDuration;
@Nullable
private final List streamIds;
$AutoValue_MongoIndexRange(
@Nullable ObjectId id,
String indexName,
DateTime begin,
DateTime end,
DateTime calculatedAt,
int calculationDuration,
@Nullable List streamIds) {
this.id = id;
if (indexName == null) {
throw new NullPointerException("Null indexName");
}
this.indexName = indexName;
if (begin == null) {
throw new NullPointerException("Null begin");
}
this.begin = begin;
if (end == null) {
throw new NullPointerException("Null end");
}
this.end = end;
if (calculatedAt == null) {
throw new NullPointerException("Null calculatedAt");
}
this.calculatedAt = calculatedAt;
this.calculationDuration = calculationDuration;
this.streamIds = streamIds;
}
@JsonProperty("_id")
@Nullable
@Id
@Override
public ObjectId id() {
return id;
}
@JsonProperty("index_name")
@Override
public String indexName() {
return indexName;
}
@Override
public DateTime begin() {
return begin;
}
@Override
public DateTime end() {
return end;
}
@Override
public DateTime calculatedAt() {
return calculatedAt;
}
@JsonProperty("took_ms")
@Override
public int calculationDuration() {
return calculationDuration;
}
@JsonProperty("stream_ids")
@Nullable
@Override
public List streamIds() {
return streamIds;
}
@Override
public String toString() {
return "MongoIndexRange{"
+ "id=" + id + ", "
+ "indexName=" + indexName + ", "
+ "begin=" + begin + ", "
+ "end=" + end + ", "
+ "calculatedAt=" + calculatedAt + ", "
+ "calculationDuration=" + calculationDuration + ", "
+ "streamIds=" + streamIds
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof MongoIndexRange) {
MongoIndexRange that = (MongoIndexRange) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.indexName.equals(that.indexName())
&& this.begin.equals(that.begin())
&& this.end.equals(that.end())
&& this.calculatedAt.equals(that.calculatedAt())
&& this.calculationDuration == that.calculationDuration()
&& (this.streamIds == null ? that.streamIds() == null : this.streamIds.equals(that.streamIds()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= indexName.hashCode();
h$ *= 1000003;
h$ ^= begin.hashCode();
h$ *= 1000003;
h$ ^= end.hashCode();
h$ *= 1000003;
h$ ^= calculatedAt.hashCode();
h$ *= 1000003;
h$ ^= calculationDuration;
h$ *= 1000003;
h$ ^= (streamIds == null) ? 0 : streamIds.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy