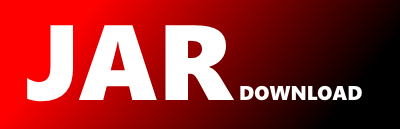
org.graylog2.indexer.searches.$AutoValue_SearchesClusterConfig Maven / Gradle / Ivy
package org.graylog2.indexer.searches;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.graylog2.indexer.searches.timerangepresets.TimerangePreset;
import org.joda.time.Period;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_SearchesClusterConfig extends SearchesClusterConfig {
private final List quickAccessTimerangePresets;
private final Period queryTimeRangeLimit;
private final Map relativeTimerangeOptions;
private final Map surroundingTimerangeOptions;
private final Set surroundingFilterFields;
private final Set analysisDisabledFields;
private final Map autoRefreshTimerangeOptions;
private final Period defaultAutoRefreshOption;
$AutoValue_SearchesClusterConfig(
List quickAccessTimerangePresets,
Period queryTimeRangeLimit,
Map relativeTimerangeOptions,
Map surroundingTimerangeOptions,
Set surroundingFilterFields,
Set analysisDisabledFields,
Map autoRefreshTimerangeOptions,
Period defaultAutoRefreshOption) {
if (quickAccessTimerangePresets == null) {
throw new NullPointerException("Null quickAccessTimerangePresets");
}
this.quickAccessTimerangePresets = quickAccessTimerangePresets;
if (queryTimeRangeLimit == null) {
throw new NullPointerException("Null queryTimeRangeLimit");
}
this.queryTimeRangeLimit = queryTimeRangeLimit;
if (relativeTimerangeOptions == null) {
throw new NullPointerException("Null relativeTimerangeOptions");
}
this.relativeTimerangeOptions = relativeTimerangeOptions;
if (surroundingTimerangeOptions == null) {
throw new NullPointerException("Null surroundingTimerangeOptions");
}
this.surroundingTimerangeOptions = surroundingTimerangeOptions;
if (surroundingFilterFields == null) {
throw new NullPointerException("Null surroundingFilterFields");
}
this.surroundingFilterFields = surroundingFilterFields;
if (analysisDisabledFields == null) {
throw new NullPointerException("Null analysisDisabledFields");
}
this.analysisDisabledFields = analysisDisabledFields;
if (autoRefreshTimerangeOptions == null) {
throw new NullPointerException("Null autoRefreshTimerangeOptions");
}
this.autoRefreshTimerangeOptions = autoRefreshTimerangeOptions;
if (defaultAutoRefreshOption == null) {
throw new NullPointerException("Null defaultAutoRefreshOption");
}
this.defaultAutoRefreshOption = defaultAutoRefreshOption;
}
@JsonProperty("quick_access_timerange_presets")
@Override
public List quickAccessTimerangePresets() {
return quickAccessTimerangePresets;
}
@JsonProperty("query_time_range_limit")
@Override
public Period queryTimeRangeLimit() {
return queryTimeRangeLimit;
}
@JsonProperty("relative_timerange_options")
@Override
public Map relativeTimerangeOptions() {
return relativeTimerangeOptions;
}
@JsonProperty("surrounding_timerange_options")
@Override
public Map surroundingTimerangeOptions() {
return surroundingTimerangeOptions;
}
@JsonProperty("surrounding_filter_fields")
@Override
public Set surroundingFilterFields() {
return surroundingFilterFields;
}
@JsonProperty("analysis_disabled_fields")
@Override
public Set analysisDisabledFields() {
return analysisDisabledFields;
}
@JsonProperty("auto_refresh_timerange_options")
@Override
public Map autoRefreshTimerangeOptions() {
return autoRefreshTimerangeOptions;
}
@JsonProperty("default_auto_refresh_option")
@Override
public Period defaultAutoRefreshOption() {
return defaultAutoRefreshOption;
}
@Override
public String toString() {
return "SearchesClusterConfig{"
+ "quickAccessTimerangePresets=" + quickAccessTimerangePresets + ", "
+ "queryTimeRangeLimit=" + queryTimeRangeLimit + ", "
+ "relativeTimerangeOptions=" + relativeTimerangeOptions + ", "
+ "surroundingTimerangeOptions=" + surroundingTimerangeOptions + ", "
+ "surroundingFilterFields=" + surroundingFilterFields + ", "
+ "analysisDisabledFields=" + analysisDisabledFields + ", "
+ "autoRefreshTimerangeOptions=" + autoRefreshTimerangeOptions + ", "
+ "defaultAutoRefreshOption=" + defaultAutoRefreshOption
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof SearchesClusterConfig) {
SearchesClusterConfig that = (SearchesClusterConfig) o;
return this.quickAccessTimerangePresets.equals(that.quickAccessTimerangePresets())
&& this.queryTimeRangeLimit.equals(that.queryTimeRangeLimit())
&& this.relativeTimerangeOptions.equals(that.relativeTimerangeOptions())
&& this.surroundingTimerangeOptions.equals(that.surroundingTimerangeOptions())
&& this.surroundingFilterFields.equals(that.surroundingFilterFields())
&& this.analysisDisabledFields.equals(that.analysisDisabledFields())
&& this.autoRefreshTimerangeOptions.equals(that.autoRefreshTimerangeOptions())
&& this.defaultAutoRefreshOption.equals(that.defaultAutoRefreshOption());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= quickAccessTimerangePresets.hashCode();
h$ *= 1000003;
h$ ^= queryTimeRangeLimit.hashCode();
h$ *= 1000003;
h$ ^= relativeTimerangeOptions.hashCode();
h$ *= 1000003;
h$ ^= surroundingTimerangeOptions.hashCode();
h$ *= 1000003;
h$ ^= surroundingFilterFields.hashCode();
h$ *= 1000003;
h$ ^= analysisDisabledFields.hashCode();
h$ *= 1000003;
h$ ^= autoRefreshTimerangeOptions.hashCode();
h$ *= 1000003;
h$ ^= defaultAutoRefreshOption.hashCode();
return h$;
}
@Override
public SearchesClusterConfig.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends SearchesClusterConfig.Builder {
private List quickAccessTimerangePresets;
private Period queryTimeRangeLimit;
private Map relativeTimerangeOptions;
private Map surroundingTimerangeOptions;
private Set surroundingFilterFields;
private Set analysisDisabledFields;
private Map autoRefreshTimerangeOptions;
private Period defaultAutoRefreshOption;
Builder() {
}
private Builder(SearchesClusterConfig source) {
this.quickAccessTimerangePresets = source.quickAccessTimerangePresets();
this.queryTimeRangeLimit = source.queryTimeRangeLimit();
this.relativeTimerangeOptions = source.relativeTimerangeOptions();
this.surroundingTimerangeOptions = source.surroundingTimerangeOptions();
this.surroundingFilterFields = source.surroundingFilterFields();
this.analysisDisabledFields = source.analysisDisabledFields();
this.autoRefreshTimerangeOptions = source.autoRefreshTimerangeOptions();
this.defaultAutoRefreshOption = source.defaultAutoRefreshOption();
}
@Override
public SearchesClusterConfig.Builder quickAccessTimerangePresets(List quickAccessTimerangePresets) {
if (quickAccessTimerangePresets == null) {
throw new NullPointerException("Null quickAccessTimerangePresets");
}
this.quickAccessTimerangePresets = quickAccessTimerangePresets;
return this;
}
@Override
public SearchesClusterConfig.Builder queryTimeRangeLimit(Period queryTimeRangeLimit) {
if (queryTimeRangeLimit == null) {
throw new NullPointerException("Null queryTimeRangeLimit");
}
this.queryTimeRangeLimit = queryTimeRangeLimit;
return this;
}
@Override
public SearchesClusterConfig.Builder relativeTimerangeOptions(Map relativeTimerangeOptions) {
if (relativeTimerangeOptions == null) {
throw new NullPointerException("Null relativeTimerangeOptions");
}
this.relativeTimerangeOptions = relativeTimerangeOptions;
return this;
}
@Override
public SearchesClusterConfig.Builder surroundingTimerangeOptions(Map surroundingTimerangeOptions) {
if (surroundingTimerangeOptions == null) {
throw new NullPointerException("Null surroundingTimerangeOptions");
}
this.surroundingTimerangeOptions = surroundingTimerangeOptions;
return this;
}
@Override
public SearchesClusterConfig.Builder surroundingFilterFields(Set surroundingFilterFields) {
if (surroundingFilterFields == null) {
throw new NullPointerException("Null surroundingFilterFields");
}
this.surroundingFilterFields = surroundingFilterFields;
return this;
}
@Override
public SearchesClusterConfig.Builder analysisDisabledFields(Set analysisDisabledFields) {
if (analysisDisabledFields == null) {
throw new NullPointerException("Null analysisDisabledFields");
}
this.analysisDisabledFields = analysisDisabledFields;
return this;
}
@Override
public SearchesClusterConfig.Builder autoRefreshTimerangeOptions(Map autoRefreshTimerangeOptions) {
if (autoRefreshTimerangeOptions == null) {
throw new NullPointerException("Null autoRefreshTimerangeOptions");
}
this.autoRefreshTimerangeOptions = autoRefreshTimerangeOptions;
return this;
}
@Override
public SearchesClusterConfig.Builder defaultAutoRefreshOption(Period defaultAutoRefreshOption) {
if (defaultAutoRefreshOption == null) {
throw new NullPointerException("Null defaultAutoRefreshOption");
}
this.defaultAutoRefreshOption = defaultAutoRefreshOption;
return this;
}
@Override
public SearchesClusterConfig build() {
if (this.quickAccessTimerangePresets == null
|| this.queryTimeRangeLimit == null
|| this.relativeTimerangeOptions == null
|| this.surroundingTimerangeOptions == null
|| this.surroundingFilterFields == null
|| this.analysisDisabledFields == null
|| this.autoRefreshTimerangeOptions == null
|| this.defaultAutoRefreshOption == null) {
StringBuilder missing = new StringBuilder();
if (this.quickAccessTimerangePresets == null) {
missing.append(" quickAccessTimerangePresets");
}
if (this.queryTimeRangeLimit == null) {
missing.append(" queryTimeRangeLimit");
}
if (this.relativeTimerangeOptions == null) {
missing.append(" relativeTimerangeOptions");
}
if (this.surroundingTimerangeOptions == null) {
missing.append(" surroundingTimerangeOptions");
}
if (this.surroundingFilterFields == null) {
missing.append(" surroundingFilterFields");
}
if (this.analysisDisabledFields == null) {
missing.append(" analysisDisabledFields");
}
if (this.autoRefreshTimerangeOptions == null) {
missing.append(" autoRefreshTimerangeOptions");
}
if (this.defaultAutoRefreshOption == null) {
missing.append(" defaultAutoRefreshOption");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_SearchesClusterConfig(
this.quickAccessTimerangePresets,
this.queryTimeRangeLimit,
this.relativeTimerangeOptions,
this.surroundingTimerangeOptions,
this.surroundingFilterFields,
this.analysisDisabledFields,
this.autoRefreshTimerangeOptions,
this.defaultAutoRefreshOption);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy