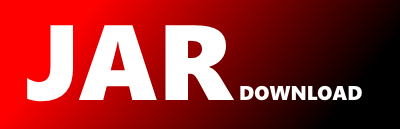
org.graylog2.lookup.adapters.$AutoValue_DnsLookupDataAdapter_Config Maven / Gradle / Ivy
package org.graylog2.lookup.adapters;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.concurrent.TimeUnit;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog2.lookup.adapters.dnslookup.DnsLookupType;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_DnsLookupDataAdapter_Config extends DnsLookupDataAdapter.Config {
private final String type;
private final DnsLookupType lookupType;
private final String serverIps;
private final int requestTimeout;
private final boolean cacheTTLOverrideEnabled;
@Nullable
private final Long cacheTTLOverride;
@Nullable
private final TimeUnit cacheTTLOverrideUnit;
$AutoValue_DnsLookupDataAdapter_Config(
String type,
DnsLookupType lookupType,
String serverIps,
int requestTimeout,
boolean cacheTTLOverrideEnabled,
@Nullable Long cacheTTLOverride,
@Nullable TimeUnit cacheTTLOverrideUnit) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (lookupType == null) {
throw new NullPointerException("Null lookupType");
}
this.lookupType = lookupType;
if (serverIps == null) {
throw new NullPointerException("Null serverIps");
}
this.serverIps = serverIps;
this.requestTimeout = requestTimeout;
this.cacheTTLOverrideEnabled = cacheTTLOverrideEnabled;
this.cacheTTLOverride = cacheTTLOverride;
this.cacheTTLOverrideUnit = cacheTTLOverrideUnit;
}
@JsonProperty("type")
@Override
public String type() {
return type;
}
@JsonProperty("lookup_type")
@Override
public DnsLookupType lookupType() {
return lookupType;
}
@JsonProperty("server_ips")
@Override
public String serverIps() {
return serverIps;
}
@JsonProperty("request_timeout")
@Override
public int requestTimeout() {
return requestTimeout;
}
@JsonProperty("cache_ttl_override_enabled")
@Override
public boolean cacheTTLOverrideEnabled() {
return cacheTTLOverrideEnabled;
}
@JsonProperty("cache_ttl_override")
@Nullable
@Override
public Long cacheTTLOverride() {
return cacheTTLOverride;
}
@JsonProperty("cache_ttl_override_unit")
@Nullable
@Override
public TimeUnit cacheTTLOverrideUnit() {
return cacheTTLOverrideUnit;
}
@Override
public String toString() {
return "Config{"
+ "type=" + type + ", "
+ "lookupType=" + lookupType + ", "
+ "serverIps=" + serverIps + ", "
+ "requestTimeout=" + requestTimeout + ", "
+ "cacheTTLOverrideEnabled=" + cacheTTLOverrideEnabled + ", "
+ "cacheTTLOverride=" + cacheTTLOverride + ", "
+ "cacheTTLOverrideUnit=" + cacheTTLOverrideUnit
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof DnsLookupDataAdapter.Config) {
DnsLookupDataAdapter.Config that = (DnsLookupDataAdapter.Config) o;
return this.type.equals(that.type())
&& this.lookupType.equals(that.lookupType())
&& this.serverIps.equals(that.serverIps())
&& this.requestTimeout == that.requestTimeout()
&& this.cacheTTLOverrideEnabled == that.cacheTTLOverrideEnabled()
&& (this.cacheTTLOverride == null ? that.cacheTTLOverride() == null : this.cacheTTLOverride.equals(that.cacheTTLOverride()))
&& (this.cacheTTLOverrideUnit == null ? that.cacheTTLOverrideUnit() == null : this.cacheTTLOverrideUnit.equals(that.cacheTTLOverrideUnit()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= lookupType.hashCode();
h$ *= 1000003;
h$ ^= serverIps.hashCode();
h$ *= 1000003;
h$ ^= requestTimeout;
h$ *= 1000003;
h$ ^= cacheTTLOverrideEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (cacheTTLOverride == null) ? 0 : cacheTTLOverride.hashCode();
h$ *= 1000003;
h$ ^= (cacheTTLOverrideUnit == null) ? 0 : cacheTTLOverrideUnit.hashCode();
return h$;
}
static class Builder extends DnsLookupDataAdapter.Config.Builder {
private String type;
private DnsLookupType lookupType;
private String serverIps;
private int requestTimeout;
private boolean cacheTTLOverrideEnabled;
private Long cacheTTLOverride;
private TimeUnit cacheTTLOverrideUnit;
private byte set$0;
Builder() {
}
@Override
public DnsLookupDataAdapter.Config.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder lookupType(DnsLookupType lookupType) {
if (lookupType == null) {
throw new NullPointerException("Null lookupType");
}
this.lookupType = lookupType;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder serverIps(String serverIps) {
if (serverIps == null) {
throw new NullPointerException("Null serverIps");
}
this.serverIps = serverIps;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder requestTimeout(int requestTimeout) {
this.requestTimeout = requestTimeout;
set$0 |= (byte) 1;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder cacheTTLOverrideEnabled(boolean cacheTTLOverrideEnabled) {
this.cacheTTLOverrideEnabled = cacheTTLOverrideEnabled;
set$0 |= (byte) 2;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder cacheTTLOverride(Long cacheTTLOverride) {
this.cacheTTLOverride = cacheTTLOverride;
return this;
}
@Override
public DnsLookupDataAdapter.Config.Builder cacheTTLOverrideUnit(@Nullable TimeUnit cacheTTLOverrideUnit) {
this.cacheTTLOverrideUnit = cacheTTLOverrideUnit;
return this;
}
@Override
DnsLookupDataAdapter.Config autoBuild() {
if (set$0 != 3
|| this.type == null
|| this.lookupType == null
|| this.serverIps == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.lookupType == null) {
missing.append(" lookupType");
}
if (this.serverIps == null) {
missing.append(" serverIps");
}
if ((set$0 & 1) == 0) {
missing.append(" requestTimeout");
}
if ((set$0 & 2) == 0) {
missing.append(" cacheTTLOverrideEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_DnsLookupDataAdapter_Config(
this.type,
this.lookupType,
this.serverIps,
this.requestTimeout,
this.cacheTTLOverrideEnabled,
this.cacheTTLOverride,
this.cacheTTLOverrideUnit);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy