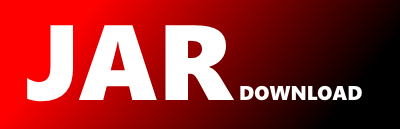
org.graylog2.plugin.cluster.ClusterConfigService Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package org.graylog2.plugin.cluster;
import org.graylog2.cluster.ClusterConfig;
import java.util.Set;
/**
* Service to save and retrieve cluster configuration beans.
*/
public interface ClusterConfigService {
/**
* Retrieve Java class of a certain type from the cluster configuration.
*
* @param type The {@link Class} of the Java configuration bean to retrieve.
* @param The type of the Java configuration bean.
* @return An instance of the requested type or {@code null} if it couldn't be retrieved.
*/
T get(Class type);
T extractPayload(Object payload, Class type);
/**
* Retrieve Java class of a certain type for the given key from the cluster configuration.
*
* @param key The key that is used to find the cluster config object in the database.
* @param type The {@link Class} of the Java configuration bean to retrieve.
* @param The type of the Java configuration bean.
* @return An instance of the requested type or {@code null} if it couldn't be retrieved.
*/
T get(String key, Class type);
/**
* Retrieve raw persisted Java class.
*
* @param key The key/type that is used to find the cluster config object in the database.
* @return An instance of the raw persisted Java class or {@code null} if it couldn't be retrieved.
*/
ClusterConfig getRaw(Class> key);
/**
* Retrieve Java class of a certain type from the cluster configuration or return a default value
* in case that failed.
*
* @param type The {@link Class} of the Java configuration bean to retrieve.
* @param defaultValue An instance of {@code T} which is returned as default value.
* @param The type of the Java configuration bean.
* @return An instance of the requested type.
*/
T getOrDefault(Class type, T defaultValue);
/**
* Write a configuration bean to the cluster configuration with the specified key.
* @param key The key that is used to write the cluster config object to the database.
* @param payload The object to write to the cluster configuration. Must be serializable by Jackson!
* @param The type of the Java configuration bean.
*/
void write(String key, T payload);
/**
* Write a configuration bean to the cluster configuration.
*
* @param payload The object to write to the cluster configuration. Must be serializable by Jackson!
* @param The type of the Java configuration bean.
*/
void write(T payload);
/**
* Remove a configuration bean from the cluster configuration.
*
* @param type The {@link Class} of the Java configuration bean to remove.
* @return The number of removed entries from the cluster configuration.
*/
int remove(Class type);
/**
* List all classes of configuration beans in the database.
*
* @return The list of Java classes being used in the database.
*/
Set> list();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy