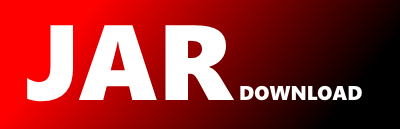
org.graylog2.rest.resources.entities.AutoValue_EntityAttribute Maven / Gradle / Ivy
package org.graylog2.rest.resources.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog2.search.SearchQueryField;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EntityAttribute extends EntityAttribute {
private final String id;
private final String title;
private final SearchQueryField.Type type;
@Nullable
private final Boolean sortable;
@Nullable
private final Boolean filterable;
@Nullable
private final Boolean searchable;
@Nullable
private final Boolean hidden;
@Nullable
private final String relatedCollection;
@Nullable
private final Set filterOptions;
private AutoValue_EntityAttribute(
String id,
String title,
SearchQueryField.Type type,
@Nullable Boolean sortable,
@Nullable Boolean filterable,
@Nullable Boolean searchable,
@Nullable Boolean hidden,
@Nullable String relatedCollection,
@Nullable Set filterOptions) {
this.id = id;
this.title = title;
this.type = type;
this.sortable = sortable;
this.filterable = filterable;
this.searchable = searchable;
this.hidden = hidden;
this.relatedCollection = relatedCollection;
this.filterOptions = filterOptions;
}
@JsonProperty("id")
@Override
public String id() {
return id;
}
@JsonProperty("title")
@Override
public String title() {
return title;
}
@JsonProperty("type")
@Override
public SearchQueryField.Type type() {
return type;
}
@JsonProperty("sortable")
@Nullable
@Override
public Boolean sortable() {
return sortable;
}
@JsonProperty("filterable")
@Nullable
@Override
public Boolean filterable() {
return filterable;
}
@JsonProperty("searchable")
@Nullable
@Override
public Boolean searchable() {
return searchable;
}
@JsonProperty("hidden")
@Nullable
@Override
public Boolean hidden() {
return hidden;
}
@JsonProperty("related_collection")
@Nullable
@Override
public String relatedCollection() {
return relatedCollection;
}
@JsonProperty("filter_options")
@Nullable
@Override
public Set filterOptions() {
return filterOptions;
}
@Override
public String toString() {
return "EntityAttribute{"
+ "id=" + id + ", "
+ "title=" + title + ", "
+ "type=" + type + ", "
+ "sortable=" + sortable + ", "
+ "filterable=" + filterable + ", "
+ "searchable=" + searchable + ", "
+ "hidden=" + hidden + ", "
+ "relatedCollection=" + relatedCollection + ", "
+ "filterOptions=" + filterOptions
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EntityAttribute) {
EntityAttribute that = (EntityAttribute) o;
return this.id.equals(that.id())
&& this.title.equals(that.title())
&& this.type.equals(that.type())
&& (this.sortable == null ? that.sortable() == null : this.sortable.equals(that.sortable()))
&& (this.filterable == null ? that.filterable() == null : this.filterable.equals(that.filterable()))
&& (this.searchable == null ? that.searchable() == null : this.searchable.equals(that.searchable()))
&& (this.hidden == null ? that.hidden() == null : this.hidden.equals(that.hidden()))
&& (this.relatedCollection == null ? that.relatedCollection() == null : this.relatedCollection.equals(that.relatedCollection()))
&& (this.filterOptions == null ? that.filterOptions() == null : this.filterOptions.equals(that.filterOptions()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= (sortable == null) ? 0 : sortable.hashCode();
h$ *= 1000003;
h$ ^= (filterable == null) ? 0 : filterable.hashCode();
h$ *= 1000003;
h$ ^= (searchable == null) ? 0 : searchable.hashCode();
h$ *= 1000003;
h$ ^= (hidden == null) ? 0 : hidden.hashCode();
h$ *= 1000003;
h$ ^= (relatedCollection == null) ? 0 : relatedCollection.hashCode();
h$ *= 1000003;
h$ ^= (filterOptions == null) ? 0 : filterOptions.hashCode();
return h$;
}
static final class Builder extends EntityAttribute.Builder {
private String id;
private String title;
private SearchQueryField.Type type;
private Boolean sortable;
private Boolean filterable;
private Boolean searchable;
private Boolean hidden;
private String relatedCollection;
private Set filterOptions;
Builder() {
}
@Override
public EntityAttribute.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public EntityAttribute.Builder title(String title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public EntityAttribute.Builder type(SearchQueryField.Type type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public EntityAttribute.Builder sortable(Boolean sortable) {
this.sortable = sortable;
return this;
}
@Override
public EntityAttribute.Builder filterable(Boolean filterable) {
this.filterable = filterable;
return this;
}
@Override
public EntityAttribute.Builder searchable(Boolean searchable) {
this.searchable = searchable;
return this;
}
@Override
public EntityAttribute.Builder hidden(Boolean hidden) {
this.hidden = hidden;
return this;
}
@Override
public EntityAttribute.Builder relatedCollection(String relatedCollection) {
this.relatedCollection = relatedCollection;
return this;
}
@Override
public EntityAttribute.Builder filterOptions(Set filterOptions) {
this.filterOptions = filterOptions;
return this;
}
@Override
public EntityAttribute build() {
if (this.id == null
|| this.title == null
|| this.type == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.title == null) {
missing.append(" title");
}
if (this.type == null) {
missing.append(" type");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EntityAttribute(
this.id,
this.title,
this.type,
this.sortable,
this.filterable,
this.searchable,
this.hidden,
this.relatedCollection,
this.filterOptions);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy