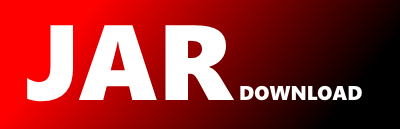
org.graylog2.streams.$AutoValue_StreamDTO Maven / Gradle / Ivy
package org.graylog2.streams;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Collection;
import java.util.Date;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.bson.types.ObjectId;
import org.graylog2.plugin.streams.StreamRule;
import org.graylog2.rest.models.alarmcallbacks.requests.AlertReceivers;
import org.graylog2.rest.models.streams.alerts.AlertConditionSummary;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_StreamDTO extends StreamDTO {
private final String id;
private final String creatorUserId;
@Nullable
private final Collection outputs;
private final String matchingType;
@Nullable
private final String description;
private final Date createdAt;
@Nullable
private final Collection rules;
private final boolean disabled;
@Nullable
private final Collection alertConditions;
@Nullable
private final AlertReceivers alertReceivers;
private final String title;
@Nullable
private final String contentPack;
@Nullable
private final Boolean isDefault;
@Nullable
private final Boolean removeMatchesFromDefaultStream;
private final String indexSetId;
private final boolean isEditable;
$AutoValue_StreamDTO(
String id,
String creatorUserId,
@Nullable Collection outputs,
String matchingType,
@Nullable String description,
Date createdAt,
@Nullable Collection rules,
boolean disabled,
@Nullable Collection alertConditions,
@Nullable AlertReceivers alertReceivers,
String title,
@Nullable String contentPack,
@Nullable Boolean isDefault,
@Nullable Boolean removeMatchesFromDefaultStream,
String indexSetId,
boolean isEditable) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (creatorUserId == null) {
throw new NullPointerException("Null creatorUserId");
}
this.creatorUserId = creatorUserId;
this.outputs = outputs;
if (matchingType == null) {
throw new NullPointerException("Null matchingType");
}
this.matchingType = matchingType;
this.description = description;
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
this.rules = rules;
this.disabled = disabled;
this.alertConditions = alertConditions;
this.alertReceivers = alertReceivers;
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
this.contentPack = contentPack;
this.isDefault = isDefault;
this.removeMatchesFromDefaultStream = removeMatchesFromDefaultStream;
if (indexSetId == null) {
throw new NullPointerException("Null indexSetId");
}
this.indexSetId = indexSetId;
this.isEditable = isEditable;
}
@JsonProperty("id")
@Override
public String id() {
return id;
}
@JsonProperty("creator_user_id")
@Override
public String creatorUserId() {
return creatorUserId;
}
@JsonProperty("outputs")
@Nullable
@Override
public Collection outputs() {
return outputs;
}
@JsonProperty("matching_type")
@Override
public String matchingType() {
return matchingType;
}
@JsonProperty("description")
@Nullable
@Override
public String description() {
return description;
}
@JsonProperty("created_at")
@Override
public Date createdAt() {
return createdAt;
}
@JsonProperty("rules")
@Nullable
@Override
public Collection rules() {
return rules;
}
@JsonProperty("disabled")
@Override
public boolean disabled() {
return disabled;
}
@JsonProperty("alert_conditions")
@Deprecated
@Nullable
@Override
public Collection alertConditions() {
return alertConditions;
}
@JsonProperty("alert_receivers")
@Deprecated
@Nullable
@Override
public AlertReceivers alertReceivers() {
return alertReceivers;
}
@JsonProperty("title")
@Override
public String title() {
return title;
}
@JsonProperty("content_pack")
@Nullable
@Override
public String contentPack() {
return contentPack;
}
@JsonProperty("is_default")
@Nullable
@Override
public Boolean isDefault() {
return isDefault;
}
@JsonProperty("remove_matches_from_default_stream")
@Nullable
@Override
public Boolean removeMatchesFromDefaultStream() {
return removeMatchesFromDefaultStream;
}
@JsonProperty("index_set_id")
@Override
public String indexSetId() {
return indexSetId;
}
@JsonProperty("is_editable")
@Override
public boolean isEditable() {
return isEditable;
}
@Override
public String toString() {
return "StreamDTO{"
+ "id=" + id + ", "
+ "creatorUserId=" + creatorUserId + ", "
+ "outputs=" + outputs + ", "
+ "matchingType=" + matchingType + ", "
+ "description=" + description + ", "
+ "createdAt=" + createdAt + ", "
+ "rules=" + rules + ", "
+ "disabled=" + disabled + ", "
+ "alertConditions=" + alertConditions + ", "
+ "alertReceivers=" + alertReceivers + ", "
+ "title=" + title + ", "
+ "contentPack=" + contentPack + ", "
+ "isDefault=" + isDefault + ", "
+ "removeMatchesFromDefaultStream=" + removeMatchesFromDefaultStream + ", "
+ "indexSetId=" + indexSetId + ", "
+ "isEditable=" + isEditable
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof StreamDTO) {
StreamDTO that = (StreamDTO) o;
return this.id.equals(that.id())
&& this.creatorUserId.equals(that.creatorUserId())
&& (this.outputs == null ? that.outputs() == null : this.outputs.equals(that.outputs()))
&& this.matchingType.equals(that.matchingType())
&& (this.description == null ? that.description() == null : this.description.equals(that.description()))
&& this.createdAt.equals(that.createdAt())
&& (this.rules == null ? that.rules() == null : this.rules.equals(that.rules()))
&& this.disabled == that.disabled()
&& (this.alertConditions == null ? that.alertConditions() == null : this.alertConditions.equals(that.alertConditions()))
&& (this.alertReceivers == null ? that.alertReceivers() == null : this.alertReceivers.equals(that.alertReceivers()))
&& this.title.equals(that.title())
&& (this.contentPack == null ? that.contentPack() == null : this.contentPack.equals(that.contentPack()))
&& (this.isDefault == null ? that.isDefault() == null : this.isDefault.equals(that.isDefault()))
&& (this.removeMatchesFromDefaultStream == null ? that.removeMatchesFromDefaultStream() == null : this.removeMatchesFromDefaultStream.equals(that.removeMatchesFromDefaultStream()))
&& this.indexSetId.equals(that.indexSetId())
&& this.isEditable == that.isEditable();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= creatorUserId.hashCode();
h$ *= 1000003;
h$ ^= (outputs == null) ? 0 : outputs.hashCode();
h$ *= 1000003;
h$ ^= matchingType.hashCode();
h$ *= 1000003;
h$ ^= (description == null) ? 0 : description.hashCode();
h$ *= 1000003;
h$ ^= createdAt.hashCode();
h$ *= 1000003;
h$ ^= (rules == null) ? 0 : rules.hashCode();
h$ *= 1000003;
h$ ^= disabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (alertConditions == null) ? 0 : alertConditions.hashCode();
h$ *= 1000003;
h$ ^= (alertReceivers == null) ? 0 : alertReceivers.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= (contentPack == null) ? 0 : contentPack.hashCode();
h$ *= 1000003;
h$ ^= (isDefault == null) ? 0 : isDefault.hashCode();
h$ *= 1000003;
h$ ^= (removeMatchesFromDefaultStream == null) ? 0 : removeMatchesFromDefaultStream.hashCode();
h$ *= 1000003;
h$ ^= indexSetId.hashCode();
h$ *= 1000003;
h$ ^= isEditable ? 1231 : 1237;
return h$;
}
@Override
public StreamDTO.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends StreamDTO.Builder {
private String id;
private String creatorUserId;
private Collection outputs;
private String matchingType;
private String description;
private Date createdAt;
private Collection rules;
private boolean disabled;
private Collection alertConditions;
private AlertReceivers alertReceivers;
private String title;
private String contentPack;
private Boolean isDefault;
private Boolean removeMatchesFromDefaultStream;
private String indexSetId;
private boolean isEditable;
private byte set$0;
Builder() {
}
private Builder(StreamDTO source) {
this.id = source.id();
this.creatorUserId = source.creatorUserId();
this.outputs = source.outputs();
this.matchingType = source.matchingType();
this.description = source.description();
this.createdAt = source.createdAt();
this.rules = source.rules();
this.disabled = source.disabled();
this.alertConditions = source.alertConditions();
this.alertReceivers = source.alertReceivers();
this.title = source.title();
this.contentPack = source.contentPack();
this.isDefault = source.isDefault();
this.removeMatchesFromDefaultStream = source.removeMatchesFromDefaultStream();
this.indexSetId = source.indexSetId();
this.isEditable = source.isEditable();
set$0 = (byte) 3;
}
@Override
public StreamDTO.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public String id() {
if (this.id == null) {
throw new IllegalStateException("Property \"id\" has not been set");
}
return id;
}
@Override
public StreamDTO.Builder creatorUserId(String creatorUserId) {
if (creatorUserId == null) {
throw new NullPointerException("Null creatorUserId");
}
this.creatorUserId = creatorUserId;
return this;
}
@Override
public StreamDTO.Builder outputs(Collection outputs) {
this.outputs = outputs;
return this;
}
@Override
public StreamDTO.Builder matchingType(String matchingType) {
if (matchingType == null) {
throw new NullPointerException("Null matchingType");
}
this.matchingType = matchingType;
return this;
}
@Override
public StreamDTO.Builder description(String description) {
this.description = description;
return this;
}
@Override
public StreamDTO.Builder createdAt(Date createdAt) {
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
return this;
}
@Override
public StreamDTO.Builder rules(Collection rules) {
this.rules = rules;
return this;
}
@Override
public StreamDTO.Builder disabled(boolean disabled) {
this.disabled = disabled;
set$0 |= (byte) 1;
return this;
}
@Override
public StreamDTO.Builder alertConditions(Collection alertConditions) {
this.alertConditions = alertConditions;
return this;
}
@Override
public StreamDTO.Builder alertReceivers(AlertReceivers alertReceivers) {
this.alertReceivers = alertReceivers;
return this;
}
@Override
public StreamDTO.Builder title(String title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public StreamDTO.Builder contentPack(String contentPack) {
this.contentPack = contentPack;
return this;
}
@Override
public StreamDTO.Builder isDefault(Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
@Override
public StreamDTO.Builder removeMatchesFromDefaultStream(Boolean removeMatchesFromDefaultStream) {
this.removeMatchesFromDefaultStream = removeMatchesFromDefaultStream;
return this;
}
@Override
public StreamDTO.Builder indexSetId(String indexSetId) {
if (indexSetId == null) {
throw new NullPointerException("Null indexSetId");
}
this.indexSetId = indexSetId;
return this;
}
@Override
public StreamDTO.Builder isEditable(boolean isEditable) {
this.isEditable = isEditable;
set$0 |= (byte) 2;
return this;
}
@Override
public StreamDTO autoBuild() {
if (set$0 != 3
|| this.id == null
|| this.creatorUserId == null
|| this.matchingType == null
|| this.createdAt == null
|| this.title == null
|| this.indexSetId == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.creatorUserId == null) {
missing.append(" creatorUserId");
}
if (this.matchingType == null) {
missing.append(" matchingType");
}
if (this.createdAt == null) {
missing.append(" createdAt");
}
if ((set$0 & 1) == 0) {
missing.append(" disabled");
}
if (this.title == null) {
missing.append(" title");
}
if (this.indexSetId == null) {
missing.append(" indexSetId");
}
if ((set$0 & 2) == 0) {
missing.append(" isEditable");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_StreamDTO(
this.id,
this.creatorUserId,
this.outputs,
this.matchingType,
this.description,
this.createdAt,
this.rules,
this.disabled,
this.alertConditions,
this.alertReceivers,
this.title,
this.contentPack,
this.isDefault,
this.removeMatchesFromDefaultStream,
this.indexSetId,
this.isEditable);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy