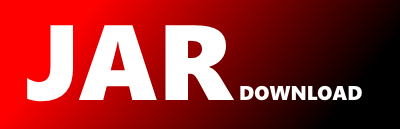
org.graylog2.system.processing.AutoValue_ProcessingStatusDto_ReceiveTimes Maven / Gradle / Ivy
package org.graylog2.system.processing;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ProcessingStatusDto_ReceiveTimes extends ProcessingStatusDto.ReceiveTimes {
private final DateTime ingest;
private final DateTime postProcessing;
private final DateTime postIndexing;
private AutoValue_ProcessingStatusDto_ReceiveTimes(
DateTime ingest,
DateTime postProcessing,
DateTime postIndexing) {
this.ingest = ingest;
this.postProcessing = postProcessing;
this.postIndexing = postIndexing;
}
@JsonProperty("ingest")
@Override
public DateTime ingest() {
return ingest;
}
@JsonProperty("post_processing")
@Override
public DateTime postProcessing() {
return postProcessing;
}
@JsonProperty("post_indexing")
@Override
public DateTime postIndexing() {
return postIndexing;
}
@Override
public String toString() {
return "ReceiveTimes{"
+ "ingest=" + ingest + ", "
+ "postProcessing=" + postProcessing + ", "
+ "postIndexing=" + postIndexing
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ProcessingStatusDto.ReceiveTimes) {
ProcessingStatusDto.ReceiveTimes that = (ProcessingStatusDto.ReceiveTimes) o;
return this.ingest.equals(that.ingest())
&& this.postProcessing.equals(that.postProcessing())
&& this.postIndexing.equals(that.postIndexing());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= ingest.hashCode();
h$ *= 1000003;
h$ ^= postProcessing.hashCode();
h$ *= 1000003;
h$ ^= postIndexing.hashCode();
return h$;
}
static final class Builder extends ProcessingStatusDto.ReceiveTimes.Builder {
private DateTime ingest;
private DateTime postProcessing;
private DateTime postIndexing;
Builder() {
}
@Override
public ProcessingStatusDto.ReceiveTimes.Builder ingest(DateTime ingest) {
if (ingest == null) {
throw new NullPointerException("Null ingest");
}
this.ingest = ingest;
return this;
}
@Override
public ProcessingStatusDto.ReceiveTimes.Builder postProcessing(DateTime postProcessing) {
if (postProcessing == null) {
throw new NullPointerException("Null postProcessing");
}
this.postProcessing = postProcessing;
return this;
}
@Override
public ProcessingStatusDto.ReceiveTimes.Builder postIndexing(DateTime postIndexing) {
if (postIndexing == null) {
throw new NullPointerException("Null postIndexing");
}
this.postIndexing = postIndexing;
return this;
}
@Override
public ProcessingStatusDto.ReceiveTimes build() {
if (this.ingest == null
|| this.postProcessing == null
|| this.postIndexing == null) {
StringBuilder missing = new StringBuilder();
if (this.ingest == null) {
missing.append(" ingest");
}
if (this.postProcessing == null) {
missing.append(" postProcessing");
}
if (this.postIndexing == null) {
missing.append(" postIndexing");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ProcessingStatusDto_ReceiveTimes(
this.ingest,
this.postProcessing,
this.postIndexing);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy