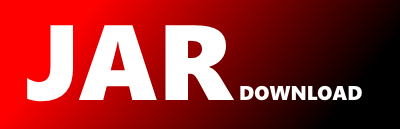
org.graylog2.users.AutoValue_UserOverviewDTO Maven / Gradle / Ivy
package org.graylog2.users;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Date;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog2.plugin.database.users.User;
import org.mongojack.Id;
import org.mongojack.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_UserOverviewDTO extends UserOverviewDTO {
@Nullable
private final String id;
private final Optional authServiceId;
private final Optional authServiceUid;
private final String username;
private final String email;
private final Optional firstName;
private final Optional lastName;
private final Optional fullName;
@Nullable
private final Boolean externalUser;
private final Set roles;
@Nullable
private final Boolean readOnly;
@Nullable
private final Boolean sessionActive;
@Nullable
private final Date lastActivity;
@Nullable
private final String clientAddress;
private final User.AccountStatus accountStatus;
private final boolean serviceAccount;
private final boolean authServiceEnabled;
private AutoValue_UserOverviewDTO(
@Nullable String id,
Optional authServiceId,
Optional authServiceUid,
String username,
String email,
Optional firstName,
Optional lastName,
Optional fullName,
@Nullable Boolean externalUser,
Set roles,
@Nullable Boolean readOnly,
@Nullable Boolean sessionActive,
@Nullable Date lastActivity,
@Nullable String clientAddress,
User.AccountStatus accountStatus,
boolean serviceAccount,
boolean authServiceEnabled) {
this.id = id;
this.authServiceId = authServiceId;
this.authServiceUid = authServiceUid;
this.username = username;
this.email = email;
this.firstName = firstName;
this.lastName = lastName;
this.fullName = fullName;
this.externalUser = externalUser;
this.roles = roles;
this.readOnly = readOnly;
this.sessionActive = sessionActive;
this.lastActivity = lastActivity;
this.clientAddress = clientAddress;
this.accountStatus = accountStatus;
this.serviceAccount = serviceAccount;
this.authServiceEnabled = authServiceEnabled;
}
@JsonProperty("id")
@Nullable
@Id
@ObjectId
@Override
public String id() {
return id;
}
@JsonProperty("auth_service_id")
@Override
public Optional authServiceId() {
return authServiceId;
}
@JsonProperty("auth_service_uid")
@Override
public Optional authServiceUid() {
return authServiceUid;
}
@JsonProperty("username")
@Override
public String username() {
return username;
}
@JsonProperty("email")
@Override
public String email() {
return email;
}
@JsonProperty("first_name")
@Override
public Optional firstName() {
return firstName;
}
@JsonProperty("last_name")
@Override
public Optional lastName() {
return lastName;
}
@JsonProperty("full_name")
@Override
public Optional fullName() {
return fullName;
}
@JsonProperty("external_user")
@Nullable
@Override
public Boolean externalUser() {
return externalUser;
}
@JsonProperty("roles")
@ObjectId
@Override
public Set roles() {
return roles;
}
@JsonProperty("read_only")
@Nullable
@Override
public Boolean readOnly() {
return readOnly;
}
@JsonProperty("session_active")
@Nullable
@Override
public Boolean sessionActive() {
return sessionActive;
}
@JsonProperty("last_activity")
@Nullable
@Override
public Date lastActivity() {
return lastActivity;
}
@JsonProperty("client_address")
@Nullable
@Override
public String clientAddress() {
return clientAddress;
}
@JsonProperty("account_status")
@Override
public User.AccountStatus accountStatus() {
return accountStatus;
}
@JsonProperty("service_account")
@Override
public boolean serviceAccount() {
return serviceAccount;
}
@JsonProperty("auth_service_enabled")
@Override
public boolean authServiceEnabled() {
return authServiceEnabled;
}
@Override
public String toString() {
return "UserOverviewDTO{"
+ "id=" + id + ", "
+ "authServiceId=" + authServiceId + ", "
+ "authServiceUid=" + authServiceUid + ", "
+ "username=" + username + ", "
+ "email=" + email + ", "
+ "firstName=" + firstName + ", "
+ "lastName=" + lastName + ", "
+ "fullName=" + fullName + ", "
+ "externalUser=" + externalUser + ", "
+ "roles=" + roles + ", "
+ "readOnly=" + readOnly + ", "
+ "sessionActive=" + sessionActive + ", "
+ "lastActivity=" + lastActivity + ", "
+ "clientAddress=" + clientAddress + ", "
+ "accountStatus=" + accountStatus + ", "
+ "serviceAccount=" + serviceAccount + ", "
+ "authServiceEnabled=" + authServiceEnabled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof UserOverviewDTO) {
UserOverviewDTO that = (UserOverviewDTO) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.authServiceId.equals(that.authServiceId())
&& this.authServiceUid.equals(that.authServiceUid())
&& this.username.equals(that.username())
&& this.email.equals(that.email())
&& this.firstName.equals(that.firstName())
&& this.lastName.equals(that.lastName())
&& this.fullName.equals(that.fullName())
&& (this.externalUser == null ? that.externalUser() == null : this.externalUser.equals(that.externalUser()))
&& this.roles.equals(that.roles())
&& (this.readOnly == null ? that.readOnly() == null : this.readOnly.equals(that.readOnly()))
&& (this.sessionActive == null ? that.sessionActive() == null : this.sessionActive.equals(that.sessionActive()))
&& (this.lastActivity == null ? that.lastActivity() == null : this.lastActivity.equals(that.lastActivity()))
&& (this.clientAddress == null ? that.clientAddress() == null : this.clientAddress.equals(that.clientAddress()))
&& this.accountStatus.equals(that.accountStatus())
&& this.serviceAccount == that.serviceAccount()
&& this.authServiceEnabled == that.authServiceEnabled();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= authServiceId.hashCode();
h$ *= 1000003;
h$ ^= authServiceUid.hashCode();
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= email.hashCode();
h$ *= 1000003;
h$ ^= firstName.hashCode();
h$ *= 1000003;
h$ ^= lastName.hashCode();
h$ *= 1000003;
h$ ^= fullName.hashCode();
h$ *= 1000003;
h$ ^= (externalUser == null) ? 0 : externalUser.hashCode();
h$ *= 1000003;
h$ ^= roles.hashCode();
h$ *= 1000003;
h$ ^= (readOnly == null) ? 0 : readOnly.hashCode();
h$ *= 1000003;
h$ ^= (sessionActive == null) ? 0 : sessionActive.hashCode();
h$ *= 1000003;
h$ ^= (lastActivity == null) ? 0 : lastActivity.hashCode();
h$ *= 1000003;
h$ ^= (clientAddress == null) ? 0 : clientAddress.hashCode();
h$ *= 1000003;
h$ ^= accountStatus.hashCode();
h$ *= 1000003;
h$ ^= serviceAccount ? 1231 : 1237;
h$ *= 1000003;
h$ ^= authServiceEnabled ? 1231 : 1237;
return h$;
}
@Override
public UserOverviewDTO.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends UserOverviewDTO.Builder {
private String id;
private Optional authServiceId = Optional.empty();
private Optional authServiceUid = Optional.empty();
private String username;
private String email;
private Optional firstName = Optional.empty();
private Optional lastName = Optional.empty();
private Optional fullName = Optional.empty();
private Boolean externalUser;
private Set roles;
private Boolean readOnly;
private Boolean sessionActive;
private Date lastActivity;
private String clientAddress;
private User.AccountStatus accountStatus;
private boolean serviceAccount;
private boolean authServiceEnabled;
private byte set$0;
Builder() {
}
private Builder(UserOverviewDTO source) {
this.id = source.id();
this.authServiceId = source.authServiceId();
this.authServiceUid = source.authServiceUid();
this.username = source.username();
this.email = source.email();
this.firstName = source.firstName();
this.lastName = source.lastName();
this.fullName = source.fullName();
this.externalUser = source.externalUser();
this.roles = source.roles();
this.readOnly = source.readOnly();
this.sessionActive = source.sessionActive();
this.lastActivity = source.lastActivity();
this.clientAddress = source.clientAddress();
this.accountStatus = source.accountStatus();
this.serviceAccount = source.serviceAccount();
this.authServiceEnabled = source.authServiceEnabled();
set$0 = (byte) 3;
}
@Override
public UserOverviewDTO.Builder id(String id) {
this.id = id;
return this;
}
@Override
public UserOverviewDTO.Builder authServiceId(@Nullable String authServiceId) {
this.authServiceId = Optional.ofNullable(authServiceId);
return this;
}
@Override
public UserOverviewDTO.Builder authServiceUid(@Nullable String authServiceUid) {
this.authServiceUid = Optional.ofNullable(authServiceUid);
return this;
}
@Override
public UserOverviewDTO.Builder username(String username) {
if (username == null) {
throw new NullPointerException("Null username");
}
this.username = username;
return this;
}
@Override
public UserOverviewDTO.Builder email(String email) {
if (email == null) {
throw new NullPointerException("Null email");
}
this.email = email;
return this;
}
@Override
public UserOverviewDTO.Builder firstName(@Nullable String firstName) {
this.firstName = Optional.ofNullable(firstName);
return this;
}
@Override
public UserOverviewDTO.Builder lastName(@Nullable String lastName) {
this.lastName = Optional.ofNullable(lastName);
return this;
}
@Override
public UserOverviewDTO.Builder fullName(@Nullable String fullName) {
this.fullName = Optional.ofNullable(fullName);
return this;
}
@Override
public UserOverviewDTO.Builder externalUser(@Nullable Boolean externalUser) {
this.externalUser = externalUser;
return this;
}
@Override
public UserOverviewDTO.Builder roles(Set roles) {
if (roles == null) {
throw new NullPointerException("Null roles");
}
this.roles = roles;
return this;
}
@Override
public UserOverviewDTO.Builder readOnly(@Nullable Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
@Override
public UserOverviewDTO.Builder sessionActive(@Nullable Boolean sessionActive) {
this.sessionActive = sessionActive;
return this;
}
@Override
public UserOverviewDTO.Builder lastActivity(@Nullable Date lastActivity) {
this.lastActivity = lastActivity;
return this;
}
@Override
public UserOverviewDTO.Builder clientAddress(@Nullable String clientAddress) {
this.clientAddress = clientAddress;
return this;
}
@Override
public UserOverviewDTO.Builder accountStatus(User.AccountStatus accountStatus) {
if (accountStatus == null) {
throw new NullPointerException("Null accountStatus");
}
this.accountStatus = accountStatus;
return this;
}
@Override
public UserOverviewDTO.Builder serviceAccount(boolean serviceAccount) {
this.serviceAccount = serviceAccount;
set$0 |= (byte) 1;
return this;
}
@Override
public UserOverviewDTO.Builder authServiceEnabled(boolean authServiceEnabled) {
this.authServiceEnabled = authServiceEnabled;
set$0 |= (byte) 2;
return this;
}
@Override
public UserOverviewDTO build() {
if (set$0 != 3
|| this.username == null
|| this.email == null
|| this.roles == null
|| this.accountStatus == null) {
StringBuilder missing = new StringBuilder();
if (this.username == null) {
missing.append(" username");
}
if (this.email == null) {
missing.append(" email");
}
if (this.roles == null) {
missing.append(" roles");
}
if (this.accountStatus == null) {
missing.append(" accountStatus");
}
if ((set$0 & 1) == 0) {
missing.append(" serviceAccount");
}
if ((set$0 & 2) == 0) {
missing.append(" authServiceEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_UserOverviewDTO(
this.id,
this.authServiceId,
this.authServiceUid,
this.username,
this.email,
this.firstName,
this.lastName,
this.fullName,
this.externalUser,
this.roles,
this.readOnly,
this.sessionActive,
this.lastActivity,
this.clientAddress,
this.accountStatus,
this.serviceAccount,
this.authServiceEnabled);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy