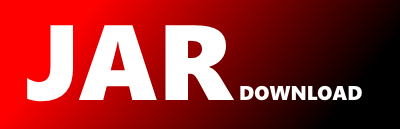
org.graylog.events.contentpack.entities.AutoValue_EmailEventNotificationConfigEntity Maven / Gradle / Ivy
package org.graylog.events.contentpack.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EmailEventNotificationConfigEntity extends EmailEventNotificationConfigEntity {
private final String type;
private final ValueReference sender;
private final ValueReference replyTo;
private final ValueReference subject;
private final ValueReference bodyTemplate;
private final ValueReference htmlBodyTemplate;
private final Set emailRecipients;
private final Set userRecipients;
private final ValueReference timeZone;
private final ValueReference lookupRecipientEmails;
private final ValueReference recipientsLUTName;
private final ValueReference recipientsLUTKey;
private final ValueReference lookupSenderEmail;
private final ValueReference senderLUTName;
private final ValueReference senderLUTKey;
private final ValueReference lookupReplyToEmail;
private final ValueReference replyToLUTName;
private final ValueReference replyToLUTKey;
private AutoValue_EmailEventNotificationConfigEntity(
String type,
ValueReference sender,
ValueReference replyTo,
ValueReference subject,
ValueReference bodyTemplate,
ValueReference htmlBodyTemplate,
Set emailRecipients,
Set userRecipients,
ValueReference timeZone,
ValueReference lookupRecipientEmails,
ValueReference recipientsLUTName,
ValueReference recipientsLUTKey,
ValueReference lookupSenderEmail,
ValueReference senderLUTName,
ValueReference senderLUTKey,
ValueReference lookupReplyToEmail,
ValueReference replyToLUTName,
ValueReference replyToLUTKey) {
this.type = type;
this.sender = sender;
this.replyTo = replyTo;
this.subject = subject;
this.bodyTemplate = bodyTemplate;
this.htmlBodyTemplate = htmlBodyTemplate;
this.emailRecipients = emailRecipients;
this.userRecipients = userRecipients;
this.timeZone = timeZone;
this.lookupRecipientEmails = lookupRecipientEmails;
this.recipientsLUTName = recipientsLUTName;
this.recipientsLUTKey = recipientsLUTKey;
this.lookupSenderEmail = lookupSenderEmail;
this.senderLUTName = senderLUTName;
this.senderLUTKey = senderLUTKey;
this.lookupReplyToEmail = lookupReplyToEmail;
this.replyToLUTName = replyToLUTName;
this.replyToLUTKey = replyToLUTKey;
}
@JsonProperty("type")
@Override
public String type() {
return type;
}
@JsonProperty("sender")
@Override
public ValueReference sender() {
return sender;
}
@JsonProperty("replyTo")
@Override
public ValueReference replyTo() {
return replyTo;
}
@JsonProperty("subject")
@Override
public ValueReference subject() {
return subject;
}
@JsonProperty("body_template")
@Override
public ValueReference bodyTemplate() {
return bodyTemplate;
}
@JsonProperty("html_body_template")
@Override
public ValueReference htmlBodyTemplate() {
return htmlBodyTemplate;
}
@JsonProperty("email_recipients")
@Override
public Set emailRecipients() {
return emailRecipients;
}
@JsonProperty("user_recipients")
@Override
public Set userRecipients() {
return userRecipients;
}
@JsonProperty("time_zone")
@Override
public ValueReference timeZone() {
return timeZone;
}
@JsonProperty("lookup_recipient_emails")
@Override
public ValueReference lookupRecipientEmails() {
return lookupRecipientEmails;
}
@JsonProperty("recipients_lut_name")
@Override
public ValueReference recipientsLUTName() {
return recipientsLUTName;
}
@JsonProperty("recipients_lut_key")
@Override
public ValueReference recipientsLUTKey() {
return recipientsLUTKey;
}
@JsonProperty("lookup_sender_email")
@Override
public ValueReference lookupSenderEmail() {
return lookupSenderEmail;
}
@JsonProperty("sender_lut_name")
@Override
public ValueReference senderLUTName() {
return senderLUTName;
}
@JsonProperty("sender_lut_key")
@Override
public ValueReference senderLUTKey() {
return senderLUTKey;
}
@JsonProperty("lookup_reply_to_email")
@Override
public ValueReference lookupReplyToEmail() {
return lookupReplyToEmail;
}
@JsonProperty("reply_to_lut_name")
@Override
public ValueReference replyToLUTName() {
return replyToLUTName;
}
@JsonProperty("reply_to_lut_key")
@Override
public ValueReference replyToLUTKey() {
return replyToLUTKey;
}
@Override
public String toString() {
return "EmailEventNotificationConfigEntity{"
+ "type=" + type + ", "
+ "sender=" + sender + ", "
+ "replyTo=" + replyTo + ", "
+ "subject=" + subject + ", "
+ "bodyTemplate=" + bodyTemplate + ", "
+ "htmlBodyTemplate=" + htmlBodyTemplate + ", "
+ "emailRecipients=" + emailRecipients + ", "
+ "userRecipients=" + userRecipients + ", "
+ "timeZone=" + timeZone + ", "
+ "lookupRecipientEmails=" + lookupRecipientEmails + ", "
+ "recipientsLUTName=" + recipientsLUTName + ", "
+ "recipientsLUTKey=" + recipientsLUTKey + ", "
+ "lookupSenderEmail=" + lookupSenderEmail + ", "
+ "senderLUTName=" + senderLUTName + ", "
+ "senderLUTKey=" + senderLUTKey + ", "
+ "lookupReplyToEmail=" + lookupReplyToEmail + ", "
+ "replyToLUTName=" + replyToLUTName + ", "
+ "replyToLUTKey=" + replyToLUTKey
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EmailEventNotificationConfigEntity) {
EmailEventNotificationConfigEntity that = (EmailEventNotificationConfigEntity) o;
return this.type.equals(that.type())
&& this.sender.equals(that.sender())
&& this.replyTo.equals(that.replyTo())
&& this.subject.equals(that.subject())
&& this.bodyTemplate.equals(that.bodyTemplate())
&& this.htmlBodyTemplate.equals(that.htmlBodyTemplate())
&& this.emailRecipients.equals(that.emailRecipients())
&& this.userRecipients.equals(that.userRecipients())
&& this.timeZone.equals(that.timeZone())
&& this.lookupRecipientEmails.equals(that.lookupRecipientEmails())
&& this.recipientsLUTName.equals(that.recipientsLUTName())
&& this.recipientsLUTKey.equals(that.recipientsLUTKey())
&& this.lookupSenderEmail.equals(that.lookupSenderEmail())
&& this.senderLUTName.equals(that.senderLUTName())
&& this.senderLUTKey.equals(that.senderLUTKey())
&& this.lookupReplyToEmail.equals(that.lookupReplyToEmail())
&& this.replyToLUTName.equals(that.replyToLUTName())
&& this.replyToLUTKey.equals(that.replyToLUTKey());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= sender.hashCode();
h$ *= 1000003;
h$ ^= replyTo.hashCode();
h$ *= 1000003;
h$ ^= subject.hashCode();
h$ *= 1000003;
h$ ^= bodyTemplate.hashCode();
h$ *= 1000003;
h$ ^= htmlBodyTemplate.hashCode();
h$ *= 1000003;
h$ ^= emailRecipients.hashCode();
h$ *= 1000003;
h$ ^= userRecipients.hashCode();
h$ *= 1000003;
h$ ^= timeZone.hashCode();
h$ *= 1000003;
h$ ^= lookupRecipientEmails.hashCode();
h$ *= 1000003;
h$ ^= recipientsLUTName.hashCode();
h$ *= 1000003;
h$ ^= recipientsLUTKey.hashCode();
h$ *= 1000003;
h$ ^= lookupSenderEmail.hashCode();
h$ *= 1000003;
h$ ^= senderLUTName.hashCode();
h$ *= 1000003;
h$ ^= senderLUTKey.hashCode();
h$ *= 1000003;
h$ ^= lookupReplyToEmail.hashCode();
h$ *= 1000003;
h$ ^= replyToLUTName.hashCode();
h$ *= 1000003;
h$ ^= replyToLUTKey.hashCode();
return h$;
}
@Override
public EmailEventNotificationConfigEntity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EmailEventNotificationConfigEntity.Builder {
private String type;
private ValueReference sender;
private ValueReference replyTo;
private ValueReference subject;
private ValueReference bodyTemplate;
private ValueReference htmlBodyTemplate;
private Set emailRecipients;
private Set userRecipients;
private ValueReference timeZone;
private ValueReference lookupRecipientEmails;
private ValueReference recipientsLUTName;
private ValueReference recipientsLUTKey;
private ValueReference lookupSenderEmail;
private ValueReference senderLUTName;
private ValueReference senderLUTKey;
private ValueReference lookupReplyToEmail;
private ValueReference replyToLUTName;
private ValueReference replyToLUTKey;
Builder() {
}
private Builder(EmailEventNotificationConfigEntity source) {
this.type = source.type();
this.sender = source.sender();
this.replyTo = source.replyTo();
this.subject = source.subject();
this.bodyTemplate = source.bodyTemplate();
this.htmlBodyTemplate = source.htmlBodyTemplate();
this.emailRecipients = source.emailRecipients();
this.userRecipients = source.userRecipients();
this.timeZone = source.timeZone();
this.lookupRecipientEmails = source.lookupRecipientEmails();
this.recipientsLUTName = source.recipientsLUTName();
this.recipientsLUTKey = source.recipientsLUTKey();
this.lookupSenderEmail = source.lookupSenderEmail();
this.senderLUTName = source.senderLUTName();
this.senderLUTKey = source.senderLUTKey();
this.lookupReplyToEmail = source.lookupReplyToEmail();
this.replyToLUTName = source.replyToLUTName();
this.replyToLUTKey = source.replyToLUTKey();
}
@Override
public EmailEventNotificationConfigEntity.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder sender(ValueReference sender) {
if (sender == null) {
throw new NullPointerException("Null sender");
}
this.sender = sender;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder replyTo(ValueReference replyTo) {
if (replyTo == null) {
throw new NullPointerException("Null replyTo");
}
this.replyTo = replyTo;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder subject(ValueReference subject) {
if (subject == null) {
throw new NullPointerException("Null subject");
}
this.subject = subject;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder bodyTemplate(ValueReference bodyTemplate) {
if (bodyTemplate == null) {
throw new NullPointerException("Null bodyTemplate");
}
this.bodyTemplate = bodyTemplate;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder htmlBodyTemplate(ValueReference htmlBodyTemplate) {
if (htmlBodyTemplate == null) {
throw new NullPointerException("Null htmlBodyTemplate");
}
this.htmlBodyTemplate = htmlBodyTemplate;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder emailRecipients(Set emailRecipients) {
if (emailRecipients == null) {
throw new NullPointerException("Null emailRecipients");
}
this.emailRecipients = emailRecipients;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder userRecipients(Set userRecipients) {
if (userRecipients == null) {
throw new NullPointerException("Null userRecipients");
}
this.userRecipients = userRecipients;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder timeZone(ValueReference timeZone) {
if (timeZone == null) {
throw new NullPointerException("Null timeZone");
}
this.timeZone = timeZone;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder lookupRecipientEmails(ValueReference lookupRecipientEmails) {
if (lookupRecipientEmails == null) {
throw new NullPointerException("Null lookupRecipientEmails");
}
this.lookupRecipientEmails = lookupRecipientEmails;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder recipientsLUTName(ValueReference recipientsLUTName) {
if (recipientsLUTName == null) {
throw new NullPointerException("Null recipientsLUTName");
}
this.recipientsLUTName = recipientsLUTName;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder recipientsLUTKey(ValueReference recipientsLUTKey) {
if (recipientsLUTKey == null) {
throw new NullPointerException("Null recipientsLUTKey");
}
this.recipientsLUTKey = recipientsLUTKey;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder lookupSenderEmail(ValueReference lookupSenderEmail) {
if (lookupSenderEmail == null) {
throw new NullPointerException("Null lookupSenderEmail");
}
this.lookupSenderEmail = lookupSenderEmail;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder senderLUTName(ValueReference senderLUTName) {
if (senderLUTName == null) {
throw new NullPointerException("Null senderLUTName");
}
this.senderLUTName = senderLUTName;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder senderLUTKey(ValueReference senderLUTKey) {
if (senderLUTKey == null) {
throw new NullPointerException("Null senderLUTKey");
}
this.senderLUTKey = senderLUTKey;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder lookupReplyToEmail(ValueReference lookupReplyToEmail) {
if (lookupReplyToEmail == null) {
throw new NullPointerException("Null lookupReplyToEmail");
}
this.lookupReplyToEmail = lookupReplyToEmail;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder replyToLUTName(ValueReference replyToLUTName) {
if (replyToLUTName == null) {
throw new NullPointerException("Null replyToLUTName");
}
this.replyToLUTName = replyToLUTName;
return this;
}
@Override
public EmailEventNotificationConfigEntity.Builder replyToLUTKey(ValueReference replyToLUTKey) {
if (replyToLUTKey == null) {
throw new NullPointerException("Null replyToLUTKey");
}
this.replyToLUTKey = replyToLUTKey;
return this;
}
@Override
public EmailEventNotificationConfigEntity build() {
if (this.type == null
|| this.sender == null
|| this.replyTo == null
|| this.subject == null
|| this.bodyTemplate == null
|| this.htmlBodyTemplate == null
|| this.emailRecipients == null
|| this.userRecipients == null
|| this.timeZone == null
|| this.lookupRecipientEmails == null
|| this.recipientsLUTName == null
|| this.recipientsLUTKey == null
|| this.lookupSenderEmail == null
|| this.senderLUTName == null
|| this.senderLUTKey == null
|| this.lookupReplyToEmail == null
|| this.replyToLUTName == null
|| this.replyToLUTKey == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.sender == null) {
missing.append(" sender");
}
if (this.replyTo == null) {
missing.append(" replyTo");
}
if (this.subject == null) {
missing.append(" subject");
}
if (this.bodyTemplate == null) {
missing.append(" bodyTemplate");
}
if (this.htmlBodyTemplate == null) {
missing.append(" htmlBodyTemplate");
}
if (this.emailRecipients == null) {
missing.append(" emailRecipients");
}
if (this.userRecipients == null) {
missing.append(" userRecipients");
}
if (this.timeZone == null) {
missing.append(" timeZone");
}
if (this.lookupRecipientEmails == null) {
missing.append(" lookupRecipientEmails");
}
if (this.recipientsLUTName == null) {
missing.append(" recipientsLUTName");
}
if (this.recipientsLUTKey == null) {
missing.append(" recipientsLUTKey");
}
if (this.lookupSenderEmail == null) {
missing.append(" lookupSenderEmail");
}
if (this.senderLUTName == null) {
missing.append(" senderLUTName");
}
if (this.senderLUTKey == null) {
missing.append(" senderLUTKey");
}
if (this.lookupReplyToEmail == null) {
missing.append(" lookupReplyToEmail");
}
if (this.replyToLUTName == null) {
missing.append(" replyToLUTName");
}
if (this.replyToLUTKey == null) {
missing.append(" replyToLUTKey");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EmailEventNotificationConfigEntity(
this.type,
this.sender,
this.replyTo,
this.subject,
this.bodyTemplate,
this.htmlBodyTemplate,
this.emailRecipients,
this.userRecipients,
this.timeZone,
this.lookupRecipientEmails,
this.recipientsLUTName,
this.recipientsLUTKey,
this.lookupSenderEmail,
this.senderLUTName,
this.senderLUTKey,
this.lookupReplyToEmail,
this.replyToLUTName,
this.replyToLUTKey);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy