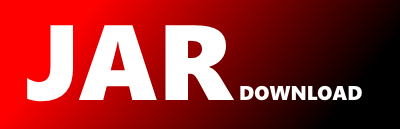
org.graylog.events.contentpack.entities.AutoValue_EventDefinitionEntity Maven / Gradle / Ivy
package org.graylog.events.contentpack.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import javax.annotation.processing.Generated;
import org.graylog.events.fields.EventFieldSpec;
import org.graylog.events.notifications.EventNotificationSettings;
import org.graylog.events.processor.storage.EventStorageHandler;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventDefinitionEntity extends EventDefinitionEntity {
@javax.annotation.Nullable
private final ValueReference scope;
private final ValueReference title;
private final ValueReference description;
@jakarta.annotation.Nullable
private final ValueReference remediationSteps;
@jakarta.annotation.Nullable
private final DateTime updatedAt;
@jakarta.annotation.Nullable
private final DateTime matchedAt;
private final ValueReference priority;
private final ValueReference alert;
private final EventProcessorConfigEntity config;
private final ImmutableMap fieldSpec;
private final ImmutableList keySpec;
private final EventNotificationSettings notificationSettings;
private final ImmutableList notifications;
private final ImmutableList storage;
private final ValueReference isScheduled;
private AutoValue_EventDefinitionEntity(
@javax.annotation.Nullable ValueReference scope,
ValueReference title,
ValueReference description,
@jakarta.annotation.Nullable ValueReference remediationSteps,
@jakarta.annotation.Nullable DateTime updatedAt,
@jakarta.annotation.Nullable DateTime matchedAt,
ValueReference priority,
ValueReference alert,
EventProcessorConfigEntity config,
ImmutableMap fieldSpec,
ImmutableList keySpec,
EventNotificationSettings notificationSettings,
ImmutableList notifications,
ImmutableList storage,
ValueReference isScheduled) {
this.scope = scope;
this.title = title;
this.description = description;
this.remediationSteps = remediationSteps;
this.updatedAt = updatedAt;
this.matchedAt = matchedAt;
this.priority = priority;
this.alert = alert;
this.config = config;
this.fieldSpec = fieldSpec;
this.keySpec = keySpec;
this.notificationSettings = notificationSettings;
this.notifications = notifications;
this.storage = storage;
this.isScheduled = isScheduled;
}
@JsonProperty("_scope")
@javax.annotation.Nullable
@Override
public ValueReference scope() {
return scope;
}
@JsonProperty("title")
@Override
public ValueReference title() {
return title;
}
@JsonProperty("description")
@Override
public ValueReference description() {
return description;
}
@JsonProperty("remediation_steps")
@jakarta.annotation.Nullable
@Override
public ValueReference remediationSteps() {
return remediationSteps;
}
@JsonProperty("updated_at")
@jakarta.annotation.Nullable
@Override
public DateTime updatedAt() {
return updatedAt;
}
@JsonProperty("matched_at")
@jakarta.annotation.Nullable
@Override
public DateTime matchedAt() {
return matchedAt;
}
@JsonProperty("priority")
@Override
public ValueReference priority() {
return priority;
}
@JsonProperty("alert")
@Override
public ValueReference alert() {
return alert;
}
@JsonProperty("config")
@Override
public EventProcessorConfigEntity config() {
return config;
}
@JsonProperty("field_spec")
@Override
public ImmutableMap fieldSpec() {
return fieldSpec;
}
@JsonProperty("key_spec")
@Override
public ImmutableList keySpec() {
return keySpec;
}
@JsonProperty("notification_settings")
@Override
public EventNotificationSettings notificationSettings() {
return notificationSettings;
}
@JsonProperty("notifications")
@Override
public ImmutableList notifications() {
return notifications;
}
@JsonProperty("storage")
@Override
public ImmutableList storage() {
return storage;
}
@JsonProperty("is_scheduled")
@Override
public ValueReference isScheduled() {
return isScheduled;
}
@Override
public String toString() {
return "EventDefinitionEntity{"
+ "scope=" + scope + ", "
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "remediationSteps=" + remediationSteps + ", "
+ "updatedAt=" + updatedAt + ", "
+ "matchedAt=" + matchedAt + ", "
+ "priority=" + priority + ", "
+ "alert=" + alert + ", "
+ "config=" + config + ", "
+ "fieldSpec=" + fieldSpec + ", "
+ "keySpec=" + keySpec + ", "
+ "notificationSettings=" + notificationSettings + ", "
+ "notifications=" + notifications + ", "
+ "storage=" + storage + ", "
+ "isScheduled=" + isScheduled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventDefinitionEntity) {
EventDefinitionEntity that = (EventDefinitionEntity) o;
return (this.scope == null ? that.scope() == null : this.scope.equals(that.scope()))
&& this.title.equals(that.title())
&& this.description.equals(that.description())
&& (this.remediationSteps == null ? that.remediationSteps() == null : this.remediationSteps.equals(that.remediationSteps()))
&& (this.updatedAt == null ? that.updatedAt() == null : this.updatedAt.equals(that.updatedAt()))
&& (this.matchedAt == null ? that.matchedAt() == null : this.matchedAt.equals(that.matchedAt()))
&& this.priority.equals(that.priority())
&& this.alert.equals(that.alert())
&& this.config.equals(that.config())
&& this.fieldSpec.equals(that.fieldSpec())
&& this.keySpec.equals(that.keySpec())
&& this.notificationSettings.equals(that.notificationSettings())
&& this.notifications.equals(that.notifications())
&& this.storage.equals(that.storage())
&& this.isScheduled.equals(that.isScheduled());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (scope == null) ? 0 : scope.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= (remediationSteps == null) ? 0 : remediationSteps.hashCode();
h$ *= 1000003;
h$ ^= (updatedAt == null) ? 0 : updatedAt.hashCode();
h$ *= 1000003;
h$ ^= (matchedAt == null) ? 0 : matchedAt.hashCode();
h$ *= 1000003;
h$ ^= priority.hashCode();
h$ *= 1000003;
h$ ^= alert.hashCode();
h$ *= 1000003;
h$ ^= config.hashCode();
h$ *= 1000003;
h$ ^= fieldSpec.hashCode();
h$ *= 1000003;
h$ ^= keySpec.hashCode();
h$ *= 1000003;
h$ ^= notificationSettings.hashCode();
h$ *= 1000003;
h$ ^= notifications.hashCode();
h$ *= 1000003;
h$ ^= storage.hashCode();
h$ *= 1000003;
h$ ^= isScheduled.hashCode();
return h$;
}
@Override
public EventDefinitionEntity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EventDefinitionEntity.Builder {
private ValueReference scope;
private ValueReference title;
private ValueReference description;
private ValueReference remediationSteps;
private DateTime updatedAt;
private DateTime matchedAt;
private ValueReference priority;
private ValueReference alert;
private EventProcessorConfigEntity config;
private ImmutableMap fieldSpec;
private ImmutableList keySpec;
private EventNotificationSettings notificationSettings;
private ImmutableList notifications;
private ImmutableList storage;
private ValueReference isScheduled;
Builder() {
}
private Builder(EventDefinitionEntity source) {
this.scope = source.scope();
this.title = source.title();
this.description = source.description();
this.remediationSteps = source.remediationSteps();
this.updatedAt = source.updatedAt();
this.matchedAt = source.matchedAt();
this.priority = source.priority();
this.alert = source.alert();
this.config = source.config();
this.fieldSpec = source.fieldSpec();
this.keySpec = source.keySpec();
this.notificationSettings = source.notificationSettings();
this.notifications = source.notifications();
this.storage = source.storage();
this.isScheduled = source.isScheduled();
}
@Override
public EventDefinitionEntity.Builder scope(ValueReference scope) {
this.scope = scope;
return this;
}
@Override
public EventDefinitionEntity.Builder title(ValueReference title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public EventDefinitionEntity.Builder description(ValueReference description) {
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
return this;
}
@Override
public EventDefinitionEntity.Builder remediationSteps(ValueReference remediationSteps) {
this.remediationSteps = remediationSteps;
return this;
}
@Override
public EventDefinitionEntity.Builder updatedAt(DateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
@Override
public EventDefinitionEntity.Builder matchedAt(DateTime matchedAt) {
this.matchedAt = matchedAt;
return this;
}
@Override
public EventDefinitionEntity.Builder priority(ValueReference priority) {
if (priority == null) {
throw new NullPointerException("Null priority");
}
this.priority = priority;
return this;
}
@Override
public EventDefinitionEntity.Builder alert(ValueReference alert) {
if (alert == null) {
throw new NullPointerException("Null alert");
}
this.alert = alert;
return this;
}
@Override
public EventDefinitionEntity.Builder config(EventProcessorConfigEntity config) {
if (config == null) {
throw new NullPointerException("Null config");
}
this.config = config;
return this;
}
@Override
public EventDefinitionEntity.Builder fieldSpec(ImmutableMap fieldSpec) {
if (fieldSpec == null) {
throw new NullPointerException("Null fieldSpec");
}
this.fieldSpec = fieldSpec;
return this;
}
@Override
public EventDefinitionEntity.Builder keySpec(ImmutableList keySpec) {
if (keySpec == null) {
throw new NullPointerException("Null keySpec");
}
this.keySpec = keySpec;
return this;
}
@Override
public EventDefinitionEntity.Builder notificationSettings(EventNotificationSettings notificationSettings) {
if (notificationSettings == null) {
throw new NullPointerException("Null notificationSettings");
}
this.notificationSettings = notificationSettings;
return this;
}
@Override
public EventDefinitionEntity.Builder notifications(ImmutableList notifications) {
if (notifications == null) {
throw new NullPointerException("Null notifications");
}
this.notifications = notifications;
return this;
}
@Override
public EventDefinitionEntity.Builder storage(ImmutableList storage) {
if (storage == null) {
throw new NullPointerException("Null storage");
}
this.storage = storage;
return this;
}
@Override
public EventDefinitionEntity.Builder isScheduled(ValueReference isScheduled) {
if (isScheduled == null) {
throw new NullPointerException("Null isScheduled");
}
this.isScheduled = isScheduled;
return this;
}
@Override
public EventDefinitionEntity build() {
if (this.title == null
|| this.description == null
|| this.priority == null
|| this.alert == null
|| this.config == null
|| this.fieldSpec == null
|| this.keySpec == null
|| this.notificationSettings == null
|| this.notifications == null
|| this.storage == null
|| this.isScheduled == null) {
StringBuilder missing = new StringBuilder();
if (this.title == null) {
missing.append(" title");
}
if (this.description == null) {
missing.append(" description");
}
if (this.priority == null) {
missing.append(" priority");
}
if (this.alert == null) {
missing.append(" alert");
}
if (this.config == null) {
missing.append(" config");
}
if (this.fieldSpec == null) {
missing.append(" fieldSpec");
}
if (this.keySpec == null) {
missing.append(" keySpec");
}
if (this.notificationSettings == null) {
missing.append(" notificationSettings");
}
if (this.notifications == null) {
missing.append(" notifications");
}
if (this.storage == null) {
missing.append(" storage");
}
if (this.isScheduled == null) {
missing.append(" isScheduled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventDefinitionEntity(
this.scope,
this.title,
this.description,
this.remediationSteps,
this.updatedAt,
this.matchedAt,
this.priority,
this.alert,
this.config,
this.fieldSpec,
this.keySpec,
this.notificationSettings,
this.notifications,
this.storage,
this.isScheduled);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy