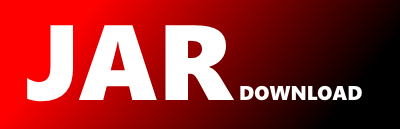
org.graylog.events.contentpack.entities.AutoValue_HttpEventNotificationConfigV2Entity Maven / Gradle / Ivy
package org.graylog.events.contentpack.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_HttpEventNotificationConfigV2Entity extends HttpEventNotificationConfigV2Entity {
private final String type;
private final ValueReference url;
private final ValueReference skipTLSVerification;
private final ValueReference httpMethod;
private final ValueReference timeZone;
private final ValueReference contentType;
private final ValueReference headers;
private final ValueReference bodyTemplate;
private AutoValue_HttpEventNotificationConfigV2Entity(
String type,
ValueReference url,
ValueReference skipTLSVerification,
ValueReference httpMethod,
ValueReference timeZone,
ValueReference contentType,
ValueReference headers,
ValueReference bodyTemplate) {
this.type = type;
this.url = url;
this.skipTLSVerification = skipTLSVerification;
this.httpMethod = httpMethod;
this.timeZone = timeZone;
this.contentType = contentType;
this.headers = headers;
this.bodyTemplate = bodyTemplate;
}
@JsonProperty("type")
@Override
public String type() {
return type;
}
@JsonProperty("url")
@Override
public ValueReference url() {
return url;
}
@JsonProperty("skip_tls_verification")
@Override
public ValueReference skipTLSVerification() {
return skipTLSVerification;
}
@JsonProperty("method")
@Override
public ValueReference httpMethod() {
return httpMethod;
}
@JsonProperty("time_zone")
@Override
public ValueReference timeZone() {
return timeZone;
}
@JsonProperty("content_type")
@Override
public ValueReference contentType() {
return contentType;
}
@JsonProperty("headers")
@Override
public ValueReference headers() {
return headers;
}
@JsonProperty("body_template")
@Override
public ValueReference bodyTemplate() {
return bodyTemplate;
}
@Override
public String toString() {
return "HttpEventNotificationConfigV2Entity{"
+ "type=" + type + ", "
+ "url=" + url + ", "
+ "skipTLSVerification=" + skipTLSVerification + ", "
+ "httpMethod=" + httpMethod + ", "
+ "timeZone=" + timeZone + ", "
+ "contentType=" + contentType + ", "
+ "headers=" + headers + ", "
+ "bodyTemplate=" + bodyTemplate
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof HttpEventNotificationConfigV2Entity) {
HttpEventNotificationConfigV2Entity that = (HttpEventNotificationConfigV2Entity) o;
return this.type.equals(that.type())
&& this.url.equals(that.url())
&& this.skipTLSVerification.equals(that.skipTLSVerification())
&& this.httpMethod.equals(that.httpMethod())
&& this.timeZone.equals(that.timeZone())
&& this.contentType.equals(that.contentType())
&& this.headers.equals(that.headers())
&& this.bodyTemplate.equals(that.bodyTemplate());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= url.hashCode();
h$ *= 1000003;
h$ ^= skipTLSVerification.hashCode();
h$ *= 1000003;
h$ ^= httpMethod.hashCode();
h$ *= 1000003;
h$ ^= timeZone.hashCode();
h$ *= 1000003;
h$ ^= contentType.hashCode();
h$ *= 1000003;
h$ ^= headers.hashCode();
h$ *= 1000003;
h$ ^= bodyTemplate.hashCode();
return h$;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends HttpEventNotificationConfigV2Entity.Builder {
private String type;
private ValueReference url;
private ValueReference skipTLSVerification;
private ValueReference httpMethod;
private ValueReference timeZone;
private ValueReference contentType;
private ValueReference headers;
private ValueReference bodyTemplate;
Builder() {
}
private Builder(HttpEventNotificationConfigV2Entity source) {
this.type = source.type();
this.url = source.url();
this.skipTLSVerification = source.skipTLSVerification();
this.httpMethod = source.httpMethod();
this.timeZone = source.timeZone();
this.contentType = source.contentType();
this.headers = source.headers();
this.bodyTemplate = source.bodyTemplate();
}
@Override
public HttpEventNotificationConfigV2Entity.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder url(ValueReference url) {
if (url == null) {
throw new NullPointerException("Null url");
}
this.url = url;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder skipTLSVerification(ValueReference skipTLSVerification) {
if (skipTLSVerification == null) {
throw new NullPointerException("Null skipTLSVerification");
}
this.skipTLSVerification = skipTLSVerification;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder httpMethod(ValueReference httpMethod) {
if (httpMethod == null) {
throw new NullPointerException("Null httpMethod");
}
this.httpMethod = httpMethod;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder timeZone(ValueReference timeZone) {
if (timeZone == null) {
throw new NullPointerException("Null timeZone");
}
this.timeZone = timeZone;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder contentType(ValueReference contentType) {
if (contentType == null) {
throw new NullPointerException("Null contentType");
}
this.contentType = contentType;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder headers(ValueReference headers) {
if (headers == null) {
throw new NullPointerException("Null headers");
}
this.headers = headers;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity.Builder bodyTemplate(ValueReference bodyTemplate) {
if (bodyTemplate == null) {
throw new NullPointerException("Null bodyTemplate");
}
this.bodyTemplate = bodyTemplate;
return this;
}
@Override
public HttpEventNotificationConfigV2Entity build() {
if (this.type == null
|| this.url == null
|| this.skipTLSVerification == null
|| this.httpMethod == null
|| this.timeZone == null
|| this.contentType == null
|| this.headers == null
|| this.bodyTemplate == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.url == null) {
missing.append(" url");
}
if (this.skipTLSVerification == null) {
missing.append(" skipTLSVerification");
}
if (this.httpMethod == null) {
missing.append(" httpMethod");
}
if (this.timeZone == null) {
missing.append(" timeZone");
}
if (this.contentType == null) {
missing.append(" contentType");
}
if (this.headers == null) {
missing.append(" headers");
}
if (this.bodyTemplate == null) {
missing.append(" bodyTemplate");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_HttpEventNotificationConfigV2Entity(
this.type,
this.url,
this.skipTLSVerification,
this.httpMethod,
this.timeZone,
this.contentType,
this.headers,
this.bodyTemplate);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy