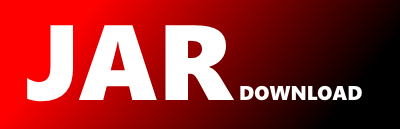
org.graylog.events.notifications.AutoValue_EventNotificationContext Maven / Gradle / Ivy
package org.graylog.events.notifications;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog.events.event.EventDto;
import org.graylog.events.processor.EventDefinitionDto;
import org.graylog.scheduler.JobTriggerDto;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventNotificationContext extends EventNotificationContext {
private final String notificationId;
private final EventNotificationConfig notificationConfig;
private final EventDto event;
private final Optional eventDefinition;
private final Optional jobTrigger;
private AutoValue_EventNotificationContext(
String notificationId,
EventNotificationConfig notificationConfig,
EventDto event,
Optional eventDefinition,
Optional jobTrigger) {
this.notificationId = notificationId;
this.notificationConfig = notificationConfig;
this.event = event;
this.eventDefinition = eventDefinition;
this.jobTrigger = jobTrigger;
}
@Override
public String notificationId() {
return notificationId;
}
@Override
public EventNotificationConfig notificationConfig() {
return notificationConfig;
}
@Override
public EventDto event() {
return event;
}
@Override
public Optional eventDefinition() {
return eventDefinition;
}
@Override
public Optional jobTrigger() {
return jobTrigger;
}
@Override
public String toString() {
return "EventNotificationContext{"
+ "notificationId=" + notificationId + ", "
+ "notificationConfig=" + notificationConfig + ", "
+ "event=" + event + ", "
+ "eventDefinition=" + eventDefinition + ", "
+ "jobTrigger=" + jobTrigger
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventNotificationContext) {
EventNotificationContext that = (EventNotificationContext) o;
return this.notificationId.equals(that.notificationId())
&& this.notificationConfig.equals(that.notificationConfig())
&& this.event.equals(that.event())
&& this.eventDefinition.equals(that.eventDefinition())
&& this.jobTrigger.equals(that.jobTrigger());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= notificationId.hashCode();
h$ *= 1000003;
h$ ^= notificationConfig.hashCode();
h$ *= 1000003;
h$ ^= event.hashCode();
h$ *= 1000003;
h$ ^= eventDefinition.hashCode();
h$ *= 1000003;
h$ ^= jobTrigger.hashCode();
return h$;
}
@Override
public EventNotificationContext.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EventNotificationContext.Builder {
private String notificationId;
private EventNotificationConfig notificationConfig;
private EventDto event;
private Optional eventDefinition = Optional.empty();
private Optional jobTrigger = Optional.empty();
Builder() {
}
private Builder(EventNotificationContext source) {
this.notificationId = source.notificationId();
this.notificationConfig = source.notificationConfig();
this.event = source.event();
this.eventDefinition = source.eventDefinition();
this.jobTrigger = source.jobTrigger();
}
@Override
public EventNotificationContext.Builder notificationId(String notificationId) {
if (notificationId == null) {
throw new NullPointerException("Null notificationId");
}
this.notificationId = notificationId;
return this;
}
@Override
public EventNotificationContext.Builder notificationConfig(EventNotificationConfig notificationConfig) {
if (notificationConfig == null) {
throw new NullPointerException("Null notificationConfig");
}
this.notificationConfig = notificationConfig;
return this;
}
@Override
public EventNotificationContext.Builder event(EventDto event) {
if (event == null) {
throw new NullPointerException("Null event");
}
this.event = event;
return this;
}
@Override
public EventNotificationContext.Builder eventDefinition(@Nullable EventDefinitionDto eventDefinition) {
this.eventDefinition = Optional.ofNullable(eventDefinition);
return this;
}
@Override
public EventNotificationContext.Builder jobTrigger(JobTriggerDto jobTrigger) {
this.jobTrigger = Optional.of(jobTrigger);
return this;
}
@Override
public EventNotificationContext build() {
if (this.notificationId == null
|| this.notificationConfig == null
|| this.event == null) {
StringBuilder missing = new StringBuilder();
if (this.notificationId == null) {
missing.append(" notificationId");
}
if (this.notificationConfig == null) {
missing.append(" notificationConfig");
}
if (this.event == null) {
missing.append(" event");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventNotificationContext(
this.notificationId,
this.notificationConfig,
this.event,
this.eventDefinition,
this.jobTrigger);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy