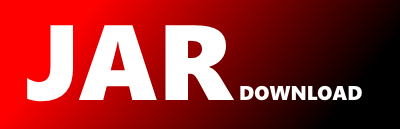
org.graylog.events.notifications.AutoValue_EventNotificationStatus Maven / Gradle / Ivy
package org.graylog.events.notifications;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.joda.time.DateTime;
import org.mongojack.Id;
import org.mongojack.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventNotificationStatus extends EventNotificationStatus {
@Nullable
private final String id;
private final String notificationId;
private final String eventDefinitionId;
private final String eventKey;
private final long gracePeriodMs;
private final Optional triggeredAt;
private final Optional notifiedAt;
private AutoValue_EventNotificationStatus(
@Nullable String id,
String notificationId,
String eventDefinitionId,
String eventKey,
long gracePeriodMs,
Optional triggeredAt,
Optional notifiedAt) {
this.id = id;
this.notificationId = notificationId;
this.eventDefinitionId = eventDefinitionId;
this.eventKey = eventKey;
this.gracePeriodMs = gracePeriodMs;
this.triggeredAt = triggeredAt;
this.notifiedAt = notifiedAt;
}
@JsonProperty("id")
@Nullable
@Id
@ObjectId
@Override
public String id() {
return id;
}
@JsonProperty("notification_id")
@Override
public String notificationId() {
return notificationId;
}
@JsonProperty("event_definition_id")
@Override
public String eventDefinitionId() {
return eventDefinitionId;
}
@JsonProperty("event_key")
@Override
public String eventKey() {
return eventKey;
}
@JsonProperty("grace_period_ms")
@Override
public long gracePeriodMs() {
return gracePeriodMs;
}
@JsonProperty("triggered_at")
@Override
public Optional triggeredAt() {
return triggeredAt;
}
@JsonProperty("notified_at")
@Override
public Optional notifiedAt() {
return notifiedAt;
}
@Override
public String toString() {
return "EventNotificationStatus{"
+ "id=" + id + ", "
+ "notificationId=" + notificationId + ", "
+ "eventDefinitionId=" + eventDefinitionId + ", "
+ "eventKey=" + eventKey + ", "
+ "gracePeriodMs=" + gracePeriodMs + ", "
+ "triggeredAt=" + triggeredAt + ", "
+ "notifiedAt=" + notifiedAt
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventNotificationStatus) {
EventNotificationStatus that = (EventNotificationStatus) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.notificationId.equals(that.notificationId())
&& this.eventDefinitionId.equals(that.eventDefinitionId())
&& this.eventKey.equals(that.eventKey())
&& this.gracePeriodMs == that.gracePeriodMs()
&& this.triggeredAt.equals(that.triggeredAt())
&& this.notifiedAt.equals(that.notifiedAt());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= notificationId.hashCode();
h$ *= 1000003;
h$ ^= eventDefinitionId.hashCode();
h$ *= 1000003;
h$ ^= eventKey.hashCode();
h$ *= 1000003;
h$ ^= (int) ((gracePeriodMs >>> 32) ^ gracePeriodMs);
h$ *= 1000003;
h$ ^= triggeredAt.hashCode();
h$ *= 1000003;
h$ ^= notifiedAt.hashCode();
return h$;
}
@Override
public EventNotificationStatus.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EventNotificationStatus.Builder {
private String id;
private String notificationId;
private String eventDefinitionId;
private String eventKey;
private long gracePeriodMs;
private Optional triggeredAt = Optional.empty();
private Optional notifiedAt = Optional.empty();
private byte set$0;
Builder() {
}
private Builder(EventNotificationStatus source) {
this.id = source.id();
this.notificationId = source.notificationId();
this.eventDefinitionId = source.eventDefinitionId();
this.eventKey = source.eventKey();
this.gracePeriodMs = source.gracePeriodMs();
this.triggeredAt = source.triggeredAt();
this.notifiedAt = source.notifiedAt();
set$0 = (byte) 1;
}
@Override
public EventNotificationStatus.Builder id(String id) {
this.id = id;
return this;
}
@Override
public EventNotificationStatus.Builder notificationId(String notificationId) {
if (notificationId == null) {
throw new NullPointerException("Null notificationId");
}
this.notificationId = notificationId;
return this;
}
@Override
public EventNotificationStatus.Builder eventDefinitionId(String eventDefinitionId) {
if (eventDefinitionId == null) {
throw new NullPointerException("Null eventDefinitionId");
}
this.eventDefinitionId = eventDefinitionId;
return this;
}
@Override
public EventNotificationStatus.Builder eventKey(String eventKey) {
if (eventKey == null) {
throw new NullPointerException("Null eventKey");
}
this.eventKey = eventKey;
return this;
}
@Override
public EventNotificationStatus.Builder gracePeriodMs(long gracePeriodMs) {
this.gracePeriodMs = gracePeriodMs;
set$0 |= (byte) 1;
return this;
}
@Override
public EventNotificationStatus.Builder triggeredAt(Optional triggeredAt) {
if (triggeredAt == null) {
throw new NullPointerException("Null triggeredAt");
}
this.triggeredAt = triggeredAt;
return this;
}
@Override
public EventNotificationStatus.Builder notifiedAt(Optional notifiedAt) {
if (notifiedAt == null) {
throw new NullPointerException("Null notifiedAt");
}
this.notifiedAt = notifiedAt;
return this;
}
@Override
public EventNotificationStatus build() {
if (set$0 != 1
|| this.notificationId == null
|| this.eventDefinitionId == null
|| this.eventKey == null) {
StringBuilder missing = new StringBuilder();
if (this.notificationId == null) {
missing.append(" notificationId");
}
if (this.eventDefinitionId == null) {
missing.append(" eventDefinitionId");
}
if (this.eventKey == null) {
missing.append(" eventKey");
}
if ((set$0 & 1) == 0) {
missing.append(" gracePeriodMs");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventNotificationStatus(
this.id,
this.notificationId,
this.eventDefinitionId,
this.eventKey,
this.gracePeriodMs,
this.triggeredAt,
this.notifiedAt);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy