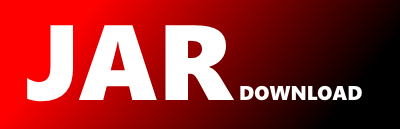
org.graylog.events.search.AutoValue_EventsSearchParameters Maven / Gradle / Ivy
package org.graylog.events.search;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Optional;
import javax.annotation.processing.Generated;
import org.graylog2.plugin.indexer.searches.timeranges.TimeRange;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventsSearchParameters extends EventsSearchParameters {
private final int page;
private final int perPage;
private final TimeRange timerange;
private final String query;
private final EventsSearchFilter filter;
private final String sortBy;
private final EventsSearchParameters.SortDirection sortDirection;
private final Optional sortUnmappedType;
private AutoValue_EventsSearchParameters(
int page,
int perPage,
TimeRange timerange,
String query,
EventsSearchFilter filter,
String sortBy,
EventsSearchParameters.SortDirection sortDirection,
Optional sortUnmappedType) {
this.page = page;
this.perPage = perPage;
this.timerange = timerange;
this.query = query;
this.filter = filter;
this.sortBy = sortBy;
this.sortDirection = sortDirection;
this.sortUnmappedType = sortUnmappedType;
}
@JsonProperty("page")
@Override
public int page() {
return page;
}
@JsonProperty("per_page")
@Override
public int perPage() {
return perPage;
}
@JsonProperty("timerange")
@Override
public TimeRange timerange() {
return timerange;
}
@JsonProperty("query")
@Override
public String query() {
return query;
}
@JsonProperty("filter")
@Override
public EventsSearchFilter filter() {
return filter;
}
@JsonProperty("sort_by")
@Override
public String sortBy() {
return sortBy;
}
@JsonProperty("sort_direction")
@Override
public EventsSearchParameters.SortDirection sortDirection() {
return sortDirection;
}
@JsonProperty("sort_unmapped_type")
@Override
public Optional sortUnmappedType() {
return sortUnmappedType;
}
@Override
public String toString() {
return "EventsSearchParameters{"
+ "page=" + page + ", "
+ "perPage=" + perPage + ", "
+ "timerange=" + timerange + ", "
+ "query=" + query + ", "
+ "filter=" + filter + ", "
+ "sortBy=" + sortBy + ", "
+ "sortDirection=" + sortDirection + ", "
+ "sortUnmappedType=" + sortUnmappedType
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventsSearchParameters) {
EventsSearchParameters that = (EventsSearchParameters) o;
return this.page == that.page()
&& this.perPage == that.perPage()
&& this.timerange.equals(that.timerange())
&& this.query.equals(that.query())
&& this.filter.equals(that.filter())
&& this.sortBy.equals(that.sortBy())
&& this.sortDirection.equals(that.sortDirection())
&& this.sortUnmappedType.equals(that.sortUnmappedType());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= page;
h$ *= 1000003;
h$ ^= perPage;
h$ *= 1000003;
h$ ^= timerange.hashCode();
h$ *= 1000003;
h$ ^= query.hashCode();
h$ *= 1000003;
h$ ^= filter.hashCode();
h$ *= 1000003;
h$ ^= sortBy.hashCode();
h$ *= 1000003;
h$ ^= sortDirection.hashCode();
h$ *= 1000003;
h$ ^= sortUnmappedType.hashCode();
return h$;
}
@Override
public EventsSearchParameters.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EventsSearchParameters.Builder {
private int page;
private int perPage;
private TimeRange timerange;
private String query;
private EventsSearchFilter filter;
private String sortBy;
private EventsSearchParameters.SortDirection sortDirection;
private Optional sortUnmappedType = Optional.empty();
private byte set$0;
Builder() {
}
private Builder(EventsSearchParameters source) {
this.page = source.page();
this.perPage = source.perPage();
this.timerange = source.timerange();
this.query = source.query();
this.filter = source.filter();
this.sortBy = source.sortBy();
this.sortDirection = source.sortDirection();
this.sortUnmappedType = source.sortUnmappedType();
set$0 = (byte) 3;
}
@Override
public EventsSearchParameters.Builder page(int page) {
this.page = page;
set$0 |= (byte) 1;
return this;
}
@Override
public EventsSearchParameters.Builder perPage(int perPage) {
this.perPage = perPage;
set$0 |= (byte) 2;
return this;
}
@Override
public EventsSearchParameters.Builder timerange(TimeRange timerange) {
if (timerange == null) {
throw new NullPointerException("Null timerange");
}
this.timerange = timerange;
return this;
}
@Override
public EventsSearchParameters.Builder query(String query) {
if (query == null) {
throw new NullPointerException("Null query");
}
this.query = query;
return this;
}
@Override
public EventsSearchParameters.Builder filter(EventsSearchFilter filter) {
if (filter == null) {
throw new NullPointerException("Null filter");
}
this.filter = filter;
return this;
}
@Override
public EventsSearchParameters.Builder sortBy(String sortBy) {
if (sortBy == null) {
throw new NullPointerException("Null sortBy");
}
this.sortBy = sortBy;
return this;
}
@Override
public EventsSearchParameters.Builder sortDirection(EventsSearchParameters.SortDirection sortDirection) {
if (sortDirection == null) {
throw new NullPointerException("Null sortDirection");
}
this.sortDirection = sortDirection;
return this;
}
@Override
public EventsSearchParameters.Builder sortUnmappedType(String sortUnmappedType) {
this.sortUnmappedType = Optional.of(sortUnmappedType);
return this;
}
@Override
public EventsSearchParameters build() {
if (set$0 != 3
|| this.timerange == null
|| this.query == null
|| this.filter == null
|| this.sortBy == null
|| this.sortDirection == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" page");
}
if ((set$0 & 2) == 0) {
missing.append(" perPage");
}
if (this.timerange == null) {
missing.append(" timerange");
}
if (this.query == null) {
missing.append(" query");
}
if (this.filter == null) {
missing.append(" filter");
}
if (this.sortBy == null) {
missing.append(" sortBy");
}
if (this.sortDirection == null) {
missing.append(" sortDirection");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventsSearchParameters(
this.page,
this.perPage,
this.timerange,
this.query,
this.filter,
this.sortBy,
this.sortDirection,
this.sortUnmappedType);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy