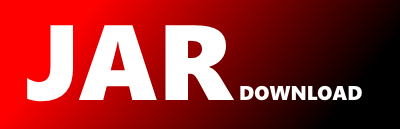
org.graylog2.cluster.nodes.AutoValue_ServerNodeDto Maven / Gradle / Ivy
package org.graylog2.cluster.nodes;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ServerNodeDto extends ServerNodeDto {
@Nullable
private final String objectId;
private final String id;
private final String transportAddress;
@Nullable
private final DateTime lastSeen;
@Nullable
private final String hostname;
private final boolean leader;
private AutoValue_ServerNodeDto(
@Nullable String objectId,
String id,
String transportAddress,
@Nullable DateTime lastSeen,
@Nullable String hostname,
boolean leader) {
this.objectId = objectId;
this.id = id;
this.transportAddress = transportAddress;
this.lastSeen = lastSeen;
this.hostname = hostname;
this.leader = leader;
}
@JsonProperty("object_id")
@Nullable
@Override
public String getObjectId() {
return objectId;
}
@JsonProperty("id")
@Override
public String getId() {
return id;
}
@JsonProperty("transport_address")
@Override
public String getTransportAddress() {
return transportAddress;
}
@JsonProperty("last_seen")
@Nullable
@Override
public DateTime getLastSeen() {
return lastSeen;
}
@JsonProperty("hostname")
@Nullable
@Override
public String getHostname() {
return hostname;
}
@JsonProperty("is_leader")
@Override
public boolean isLeader() {
return leader;
}
@Override
public String toString() {
return "ServerNodeDto{"
+ "objectId=" + objectId + ", "
+ "id=" + id + ", "
+ "transportAddress=" + transportAddress + ", "
+ "lastSeen=" + lastSeen + ", "
+ "hostname=" + hostname + ", "
+ "leader=" + leader
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ServerNodeDto) {
ServerNodeDto that = (ServerNodeDto) o;
return (this.objectId == null ? that.getObjectId() == null : this.objectId.equals(that.getObjectId()))
&& this.id.equals(that.getId())
&& this.transportAddress.equals(that.getTransportAddress())
&& (this.lastSeen == null ? that.getLastSeen() == null : this.lastSeen.equals(that.getLastSeen()))
&& (this.hostname == null ? that.getHostname() == null : this.hostname.equals(that.getHostname()))
&& this.leader == that.isLeader();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (objectId == null) ? 0 : objectId.hashCode();
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= transportAddress.hashCode();
h$ *= 1000003;
h$ ^= (lastSeen == null) ? 0 : lastSeen.hashCode();
h$ *= 1000003;
h$ ^= (hostname == null) ? 0 : hostname.hashCode();
h$ *= 1000003;
h$ ^= leader ? 1231 : 1237;
return h$;
}
@Override
public ServerNodeDto.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends ServerNodeDto.Builder {
private String objectId;
private String id;
private String transportAddress;
private DateTime lastSeen;
private String hostname;
private boolean leader;
private byte set$0;
Builder() {
}
private Builder(ServerNodeDto source) {
this.objectId = source.getObjectId();
this.id = source.getId();
this.transportAddress = source.getTransportAddress();
this.lastSeen = source.getLastSeen();
this.hostname = source.getHostname();
this.leader = source.isLeader();
set$0 = (byte) 1;
}
@Override
public ServerNodeDto.Builder setObjectId(String objectId) {
this.objectId = objectId;
return this;
}
@Override
public ServerNodeDto.Builder setId(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public ServerNodeDto.Builder setTransportAddress(String transportAddress) {
if (transportAddress == null) {
throw new NullPointerException("Null transportAddress");
}
this.transportAddress = transportAddress;
return this;
}
@Override
public ServerNodeDto.Builder setLastSeen(DateTime lastSeen) {
this.lastSeen = lastSeen;
return this;
}
@Override
public ServerNodeDto.Builder setHostname(String hostname) {
this.hostname = hostname;
return this;
}
@Override
public ServerNodeDto.Builder setLeader(boolean leader) {
this.leader = leader;
set$0 |= (byte) 1;
return this;
}
@Override
public ServerNodeDto build() {
if (set$0 != 1
|| this.id == null
|| this.transportAddress == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.transportAddress == null) {
missing.append(" transportAddress");
}
if ((set$0 & 1) == 0) {
missing.append(" leader");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ServerNodeDto(
this.objectId,
this.id,
this.transportAddress,
this.lastSeen,
this.hostname,
this.leader);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy