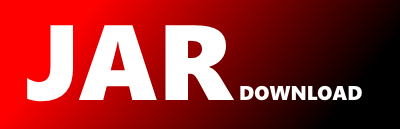
org.graylog2.cluster.preflight.$AutoValue_DataNodeProvisioningConfig Maven / Gradle / Ivy
package org.graylog2.cluster.preflight;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.mongojack.Id;
import org.mongojack.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_DataNodeProvisioningConfig extends DataNodeProvisioningConfig {
@Nullable
private final String id;
@Nullable
private final String nodeId;
@Nullable
private final List altNames;
@Nullable
private final Integer validFor;
@Nullable
private final DataNodeProvisioningConfig.State state;
@Nullable
private final String errorMsg;
@Nullable
private final String csr;
@Nullable
private final String certificate;
$AutoValue_DataNodeProvisioningConfig(
@Nullable String id,
@Nullable String nodeId,
@Nullable List altNames,
@Nullable Integer validFor,
@Nullable DataNodeProvisioningConfig.State state,
@Nullable String errorMsg,
@Nullable String csr,
@Nullable String certificate) {
this.id = id;
this.nodeId = nodeId;
this.altNames = altNames;
this.validFor = validFor;
this.state = state;
this.errorMsg = errorMsg;
this.csr = csr;
this.certificate = certificate;
}
@Nullable
@Id
@ObjectId
@Override
public String id() {
return id;
}
@JsonProperty
@Nullable
@Override
public String nodeId() {
return nodeId;
}
@JsonProperty
@Nullable
@Override
public List altNames() {
return altNames;
}
@JsonProperty
@Nullable
@Override
public Integer validFor() {
return validFor;
}
@JsonProperty
@Nullable
@Override
public DataNodeProvisioningConfig.State state() {
return state;
}
@JsonProperty
@Nullable
@Override
public String errorMsg() {
return errorMsg;
}
@JsonProperty
@Nullable
@Override
public String csr() {
return csr;
}
@JsonProperty
@Nullable
@Override
public String certificate() {
return certificate;
}
@Override
public String toString() {
return "DataNodeProvisioningConfig{"
+ "id=" + id + ", "
+ "nodeId=" + nodeId + ", "
+ "altNames=" + altNames + ", "
+ "validFor=" + validFor + ", "
+ "state=" + state + ", "
+ "errorMsg=" + errorMsg + ", "
+ "csr=" + csr + ", "
+ "certificate=" + certificate
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof DataNodeProvisioningConfig) {
DataNodeProvisioningConfig that = (DataNodeProvisioningConfig) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& (this.nodeId == null ? that.nodeId() == null : this.nodeId.equals(that.nodeId()))
&& (this.altNames == null ? that.altNames() == null : this.altNames.equals(that.altNames()))
&& (this.validFor == null ? that.validFor() == null : this.validFor.equals(that.validFor()))
&& (this.state == null ? that.state() == null : this.state.equals(that.state()))
&& (this.errorMsg == null ? that.errorMsg() == null : this.errorMsg.equals(that.errorMsg()))
&& (this.csr == null ? that.csr() == null : this.csr.equals(that.csr()))
&& (this.certificate == null ? that.certificate() == null : this.certificate.equals(that.certificate()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= (nodeId == null) ? 0 : nodeId.hashCode();
h$ *= 1000003;
h$ ^= (altNames == null) ? 0 : altNames.hashCode();
h$ *= 1000003;
h$ ^= (validFor == null) ? 0 : validFor.hashCode();
h$ *= 1000003;
h$ ^= (state == null) ? 0 : state.hashCode();
h$ *= 1000003;
h$ ^= (errorMsg == null) ? 0 : errorMsg.hashCode();
h$ *= 1000003;
h$ ^= (csr == null) ? 0 : csr.hashCode();
h$ *= 1000003;
h$ ^= (certificate == null) ? 0 : certificate.hashCode();
return h$;
}
@Override
public DataNodeProvisioningConfig.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends DataNodeProvisioningConfig.Builder {
private String id;
private String nodeId;
private List altNames;
private Integer validFor;
private DataNodeProvisioningConfig.State state;
private String errorMsg;
private String csr;
private String certificate;
Builder() {
}
private Builder(DataNodeProvisioningConfig source) {
this.id = source.id();
this.nodeId = source.nodeId();
this.altNames = source.altNames();
this.validFor = source.validFor();
this.state = source.state();
this.errorMsg = source.errorMsg();
this.csr = source.csr();
this.certificate = source.certificate();
}
@Override
public DataNodeProvisioningConfig.Builder id(String id) {
this.id = id;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder nodeId(String nodeId) {
this.nodeId = nodeId;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder altNames(List altNames) {
this.altNames = altNames;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder validFor(Integer validFor) {
this.validFor = validFor;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder state(DataNodeProvisioningConfig.State state) {
this.state = state;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder errorMsg(String errorMsg) {
this.errorMsg = errorMsg;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder csr(String csr) {
this.csr = csr;
return this;
}
@Override
public DataNodeProvisioningConfig.Builder certificate(String certificate) {
this.certificate = certificate;
return this;
}
@Override
public DataNodeProvisioningConfig build() {
return new AutoValue_DataNodeProvisioningConfig(
this.id,
this.nodeId,
this.altNames,
this.validFor,
this.state,
this.errorMsg,
this.csr,
this.certificate);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy