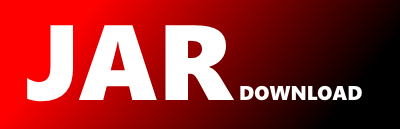
org.graylog2.contentpacks.model.entities.$AutoValue_StreamEntity Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import jakarta.validation.constraints.NotBlank;
import jakarta.validation.constraints.NotNull;
import java.util.List;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_StreamEntity extends StreamEntity {
private final @NotBlank ValueReference title;
private final ValueReference description;
private final ValueReference disabled;
private final ValueReference matchingType;
private final @NotNull List streamRules;
private final @NotNull List alertConditions;
private final @NotNull List alarmCallbacks;
private final @NotNull Set outputs;
private final ValueReference defaultStream;
private final ValueReference removeMatches;
$AutoValue_StreamEntity(
@NotBlank ValueReference title,
ValueReference description,
ValueReference disabled,
ValueReference matchingType,
@NotNull List streamRules,
@NotNull List alertConditions,
@NotNull List alarmCallbacks,
@NotNull Set outputs,
ValueReference defaultStream,
ValueReference removeMatches) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
if (disabled == null) {
throw new NullPointerException("Null disabled");
}
this.disabled = disabled;
if (matchingType == null) {
throw new NullPointerException("Null matchingType");
}
this.matchingType = matchingType;
if (streamRules == null) {
throw new NullPointerException("Null streamRules");
}
this.streamRules = streamRules;
if (alertConditions == null) {
throw new NullPointerException("Null alertConditions");
}
this.alertConditions = alertConditions;
if (alarmCallbacks == null) {
throw new NullPointerException("Null alarmCallbacks");
}
this.alarmCallbacks = alarmCallbacks;
if (outputs == null) {
throw new NullPointerException("Null outputs");
}
this.outputs = outputs;
if (defaultStream == null) {
throw new NullPointerException("Null defaultStream");
}
this.defaultStream = defaultStream;
if (removeMatches == null) {
throw new NullPointerException("Null removeMatches");
}
this.removeMatches = removeMatches;
}
@JsonProperty("title")
@Override
public @NotBlank ValueReference title() {
return title;
}
@JsonProperty("description")
@Override
public ValueReference description() {
return description;
}
@JsonProperty("disabled")
@Override
public ValueReference disabled() {
return disabled;
}
@JsonProperty("matching_type")
@Override
public ValueReference matchingType() {
return matchingType;
}
@JsonProperty("stream_rules")
@Override
public @NotNull List streamRules() {
return streamRules;
}
@JsonProperty("alert_conditions")
@Deprecated
@Override
public @NotNull List alertConditions() {
return alertConditions;
}
@JsonProperty("alarm_callbacks")
@Override
public @NotNull List alarmCallbacks() {
return alarmCallbacks;
}
@JsonProperty("outputs")
@Override
public @NotNull Set outputs() {
return outputs;
}
@JsonProperty("default_stream")
@Override
public ValueReference defaultStream() {
return defaultStream;
}
@JsonProperty("remove_matches")
@Override
public ValueReference removeMatches() {
return removeMatches;
}
@Override
public String toString() {
return "StreamEntity{"
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "disabled=" + disabled + ", "
+ "matchingType=" + matchingType + ", "
+ "streamRules=" + streamRules + ", "
+ "alertConditions=" + alertConditions + ", "
+ "alarmCallbacks=" + alarmCallbacks + ", "
+ "outputs=" + outputs + ", "
+ "defaultStream=" + defaultStream + ", "
+ "removeMatches=" + removeMatches
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof StreamEntity) {
StreamEntity that = (StreamEntity) o;
return this.title.equals(that.title())
&& this.description.equals(that.description())
&& this.disabled.equals(that.disabled())
&& this.matchingType.equals(that.matchingType())
&& this.streamRules.equals(that.streamRules())
&& this.alertConditions.equals(that.alertConditions())
&& this.alarmCallbacks.equals(that.alarmCallbacks())
&& this.outputs.equals(that.outputs())
&& this.defaultStream.equals(that.defaultStream())
&& this.removeMatches.equals(that.removeMatches());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= disabled.hashCode();
h$ *= 1000003;
h$ ^= matchingType.hashCode();
h$ *= 1000003;
h$ ^= streamRules.hashCode();
h$ *= 1000003;
h$ ^= alertConditions.hashCode();
h$ *= 1000003;
h$ ^= alarmCallbacks.hashCode();
h$ *= 1000003;
h$ ^= outputs.hashCode();
h$ *= 1000003;
h$ ^= defaultStream.hashCode();
h$ *= 1000003;
h$ ^= removeMatches.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy