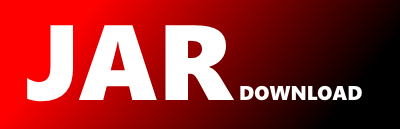
org.graylog2.datatiering.hotonly.$AutoValue_HotOnlyDataTieringConfig Maven / Gradle / Ivy
package org.graylog2.datatiering.hotonly;
import com.fasterxml.jackson.annotation.JsonProperty;
import jakarta.validation.constraints.NotNull;
import javax.annotation.processing.Generated;
import org.joda.time.Period;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_HotOnlyDataTieringConfig extends HotOnlyDataTieringConfig {
private final @NotNull String type;
private final @NotNull Period indexLifetimeMin;
private final @NotNull Period indexLifetimeMax;
$AutoValue_HotOnlyDataTieringConfig(
@NotNull String type,
@NotNull Period indexLifetimeMin,
@NotNull Period indexLifetimeMax) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (indexLifetimeMin == null) {
throw new NullPointerException("Null indexLifetimeMin");
}
this.indexLifetimeMin = indexLifetimeMin;
if (indexLifetimeMax == null) {
throw new NullPointerException("Null indexLifetimeMax");
}
this.indexLifetimeMax = indexLifetimeMax;
}
@JsonProperty("type")
@Override
public @NotNull String type() {
return type;
}
@JsonProperty("index_lifetime_min")
@Override
public @NotNull Period indexLifetimeMin() {
return indexLifetimeMin;
}
@JsonProperty("index_lifetime_max")
@Override
public @NotNull Period indexLifetimeMax() {
return indexLifetimeMax;
}
@Override
public String toString() {
return "HotOnlyDataTieringConfig{"
+ "type=" + type + ", "
+ "indexLifetimeMin=" + indexLifetimeMin + ", "
+ "indexLifetimeMax=" + indexLifetimeMax
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof HotOnlyDataTieringConfig) {
HotOnlyDataTieringConfig that = (HotOnlyDataTieringConfig) o;
return this.type.equals(that.type())
&& this.indexLifetimeMin.equals(that.indexLifetimeMin())
&& this.indexLifetimeMax.equals(that.indexLifetimeMax());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= indexLifetimeMin.hashCode();
h$ *= 1000003;
h$ ^= indexLifetimeMax.hashCode();
return h$;
}
static class Builder extends HotOnlyDataTieringConfig.Builder {
private @NotNull String type;
private @NotNull Period indexLifetimeMin;
private @NotNull Period indexLifetimeMax;
Builder() {
}
@Override
public HotOnlyDataTieringConfig.Builder type(@NotNull String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public HotOnlyDataTieringConfig.Builder indexLifetimeMin(Period indexLifetimeMin) {
if (indexLifetimeMin == null) {
throw new NullPointerException("Null indexLifetimeMin");
}
this.indexLifetimeMin = indexLifetimeMin;
return this;
}
@Override
public HotOnlyDataTieringConfig.Builder indexLifetimeMax(Period indexLifetimeMax) {
if (indexLifetimeMax == null) {
throw new NullPointerException("Null indexLifetimeMax");
}
this.indexLifetimeMax = indexLifetimeMax;
return this;
}
@Override
public HotOnlyDataTieringConfig build() {
if (this.type == null
|| this.indexLifetimeMin == null
|| this.indexLifetimeMax == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.indexLifetimeMin == null) {
missing.append(" indexLifetimeMin");
}
if (this.indexLifetimeMax == null) {
missing.append(" indexLifetimeMax");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_HotOnlyDataTieringConfig(
this.type,
this.indexLifetimeMin,
this.indexLifetimeMax);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy