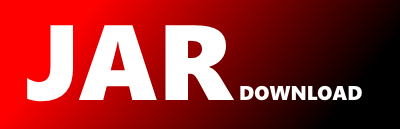
org.graylog2.indexer.results.ResultMessage Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package org.graylog2.indexer.results;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.google.common.collect.Maps;
import com.google.common.collect.Multimap;
import com.google.common.collect.Range;
import org.graylog2.plugin.Message;
import org.graylog2.plugin.MessageFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Map;
import static org.graylog2.plugin.Tools.ES_DATE_FORMAT_FORMATTER;
public class ResultMessage {
private static final Logger LOG = LoggerFactory.getLogger(ResultMessage.class);
private final MessageFactory messageFactory;
private Message message;
private String index;
@JsonInclude(JsonInclude.Include.NON_NULL)
public Multimap> highlightRanges;
ResultMessage(MessageFactory messageFactory) { /* use factory method */
this.messageFactory = messageFactory;
}
ResultMessage(MessageFactory messageFactory, String id, String index, Map message, Multimap> highlightRanges) {
this(messageFactory);
this.index = index;
this.highlightRanges = highlightRanges;
setMessage(id, message);
}
public void setMessage(Message message) {
this.message = message;
}
public void setMessage(String id, Map message) {
Map tmp = Maps.newHashMap();
tmp.putAll(message);
tmp.put(Message.FIELD_ID, id);
if (tmp.containsKey(Message.FIELD_TIMESTAMP)) {
final Object tsField = tmp.get(Message.FIELD_TIMESTAMP);
try {
tmp.put(Message.FIELD_TIMESTAMP, ES_DATE_FORMAT_FORMATTER.parseDateTime(String.valueOf(tsField)));
} catch (IllegalArgumentException e) {
// could not parse date string, this is likely a bug, but we will leave the original value alone
LOG.warn("Could not parse timestamp of message {}", message.get("id"), e);
}
}
this.message = messageFactory.createMessage(tmp);
}
public void setIndex(String index) {
this.index = index;
}
public Message getMessage() {
return message;
}
public String getIndex() {
return index;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy