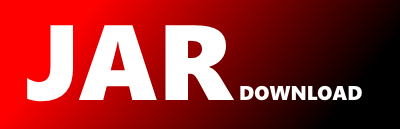
org.graylog2.indexer.searches.AutoValue_ChunkCommand Maven / Gradle / Ivy
package org.graylog2.indexer.searches;
import java.util.List;
import java.util.Optional;
import java.util.OptionalInt;
import java.util.OptionalLong;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog.plugins.views.search.searchfilters.model.UsedSearchFilter;
import org.graylog2.plugin.indexer.searches.timeranges.TimeRange;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ChunkCommand extends ChunkCommand {
private final String query;
private final Set indices;
private final Optional> streams;
private final Optional sorting;
private final Optional filter;
private final List filters;
private final Optional range;
private final OptionalInt limit;
private final OptionalInt offset;
private final List fields;
private final OptionalLong batchSize;
private final Optional sliceParams;
private final boolean highlight;
private AutoValue_ChunkCommand(
String query,
Set indices,
Optional> streams,
Optional sorting,
Optional filter,
List filters,
Optional range,
OptionalInt limit,
OptionalInt offset,
List fields,
OptionalLong batchSize,
Optional sliceParams,
boolean highlight) {
this.query = query;
this.indices = indices;
this.streams = streams;
this.sorting = sorting;
this.filter = filter;
this.filters = filters;
this.range = range;
this.limit = limit;
this.offset = offset;
this.fields = fields;
this.batchSize = batchSize;
this.sliceParams = sliceParams;
this.highlight = highlight;
}
@Override
public String query() {
return query;
}
@Override
public Set indices() {
return indices;
}
@Override
public Optional> streams() {
return streams;
}
@Override
public Optional sorting() {
return sorting;
}
@Override
public Optional filter() {
return filter;
}
@Override
public List filters() {
return filters;
}
@Override
public Optional range() {
return range;
}
@Override
public OptionalInt limit() {
return limit;
}
@Override
public OptionalInt offset() {
return offset;
}
@Override
public List fields() {
return fields;
}
@Override
public OptionalLong batchSize() {
return batchSize;
}
@Override
public Optional sliceParams() {
return sliceParams;
}
@Override
public boolean highlight() {
return highlight;
}
@Override
public String toString() {
return "ChunkCommand{"
+ "query=" + query + ", "
+ "indices=" + indices + ", "
+ "streams=" + streams + ", "
+ "sorting=" + sorting + ", "
+ "filter=" + filter + ", "
+ "filters=" + filters + ", "
+ "range=" + range + ", "
+ "limit=" + limit + ", "
+ "offset=" + offset + ", "
+ "fields=" + fields + ", "
+ "batchSize=" + batchSize + ", "
+ "sliceParams=" + sliceParams + ", "
+ "highlight=" + highlight
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ChunkCommand) {
ChunkCommand that = (ChunkCommand) o;
return this.query.equals(that.query())
&& this.indices.equals(that.indices())
&& this.streams.equals(that.streams())
&& this.sorting.equals(that.sorting())
&& this.filter.equals(that.filter())
&& this.filters.equals(that.filters())
&& this.range.equals(that.range())
&& this.limit.equals(that.limit())
&& this.offset.equals(that.offset())
&& this.fields.equals(that.fields())
&& this.batchSize.equals(that.batchSize())
&& this.sliceParams.equals(that.sliceParams())
&& this.highlight == that.highlight();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= query.hashCode();
h$ *= 1000003;
h$ ^= indices.hashCode();
h$ *= 1000003;
h$ ^= streams.hashCode();
h$ *= 1000003;
h$ ^= sorting.hashCode();
h$ *= 1000003;
h$ ^= filter.hashCode();
h$ *= 1000003;
h$ ^= filters.hashCode();
h$ *= 1000003;
h$ ^= range.hashCode();
h$ *= 1000003;
h$ ^= limit.hashCode();
h$ *= 1000003;
h$ ^= offset.hashCode();
h$ *= 1000003;
h$ ^= fields.hashCode();
h$ *= 1000003;
h$ ^= batchSize.hashCode();
h$ *= 1000003;
h$ ^= sliceParams.hashCode();
h$ *= 1000003;
h$ ^= highlight ? 1231 : 1237;
return h$;
}
@Override
public ChunkCommand.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends ChunkCommand.Builder {
private String query;
private Set indices;
private Optional> streams = Optional.empty();
private Optional sorting = Optional.empty();
private Optional filter = Optional.empty();
private List filters;
private Optional range = Optional.empty();
private OptionalInt limit = OptionalInt.empty();
private OptionalInt offset = OptionalInt.empty();
private List fields;
private OptionalLong batchSize = OptionalLong.empty();
private Optional sliceParams = Optional.empty();
private boolean highlight;
private byte set$0;
Builder() {
}
private Builder(ChunkCommand source) {
this.query = source.query();
this.indices = source.indices();
this.streams = source.streams();
this.sorting = source.sorting();
this.filter = source.filter();
this.filters = source.filters();
this.range = source.range();
this.limit = source.limit();
this.offset = source.offset();
this.fields = source.fields();
this.batchSize = source.batchSize();
this.sliceParams = source.sliceParams();
this.highlight = source.highlight();
set$0 = (byte) 1;
}
@Override
public ChunkCommand.Builder query(String query) {
if (query == null) {
throw new NullPointerException("Null query");
}
this.query = query;
return this;
}
@Override
public ChunkCommand.Builder indices(Set indices) {
if (indices == null) {
throw new NullPointerException("Null indices");
}
this.indices = indices;
return this;
}
@Override
public ChunkCommand.Builder streams(Set streams) {
this.streams = Optional.of(streams);
return this;
}
@Override
public ChunkCommand.Builder sorting(Sorting sorting) {
this.sorting = Optional.of(sorting);
return this;
}
@Override
public ChunkCommand.Builder filter(@Nullable String filter) {
this.filter = Optional.ofNullable(filter);
return this;
}
@Override
public ChunkCommand.Builder filters(List filters) {
if (filters == null) {
throw new NullPointerException("Null filters");
}
this.filters = filters;
return this;
}
@Override
public ChunkCommand.Builder range(TimeRange range) {
this.range = Optional.of(range);
return this;
}
@Override
public ChunkCommand.Builder limit(int limit) {
this.limit = OptionalInt.of(limit);
return this;
}
@Override
public ChunkCommand.Builder offset(int offset) {
this.offset = OptionalInt.of(offset);
return this;
}
@Override
public ChunkCommand.Builder fields(List fields) {
if (fields == null) {
throw new NullPointerException("Null fields");
}
this.fields = fields;
return this;
}
@Override
public ChunkCommand.Builder batchSize(int batchSize) {
this.batchSize = OptionalLong.of(batchSize);
return this;
}
@Override
public ChunkCommand.Builder sliceParams(@Nullable ChunkCommand.SliceParams sliceParams) {
this.sliceParams = Optional.ofNullable(sliceParams);
return this;
}
@Override
public ChunkCommand.Builder highlight(boolean highlight) {
this.highlight = highlight;
set$0 |= (byte) 1;
return this;
}
@Override
public ChunkCommand build() {
if (set$0 != 1
|| this.query == null
|| this.indices == null
|| this.filters == null
|| this.fields == null) {
StringBuilder missing = new StringBuilder();
if (this.query == null) {
missing.append(" query");
}
if (this.indices == null) {
missing.append(" indices");
}
if (this.filters == null) {
missing.append(" filters");
}
if (this.fields == null) {
missing.append(" fields");
}
if ((set$0 & 1) == 0) {
missing.append(" highlight");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ChunkCommand(
this.query,
this.indices,
this.streams,
this.sorting,
this.filter,
this.filters,
this.range,
this.limit,
this.offset,
this.fields,
this.batchSize,
this.sliceParams,
this.highlight);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy