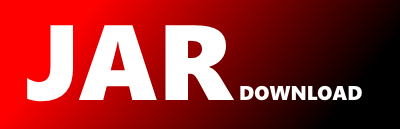
org.graylog2.plugin.utilities.AutoValue_FileInfo Maven / Gradle / Ivy
package org.graylog2.plugin.utilities;
import java.nio.file.Path;
import java.nio.file.attribute.FileTime;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_FileInfo extends FileInfo {
@Nullable
private final Object key;
private final long size;
@Nullable
private final FileTime modificationTime;
private final Path path;
private AutoValue_FileInfo(
@Nullable Object key,
long size,
@Nullable FileTime modificationTime,
Path path) {
this.key = key;
this.size = size;
this.modificationTime = modificationTime;
this.path = path;
}
@Nullable
@Override
public Object key() {
return key;
}
@Override
public long size() {
return size;
}
@Nullable
@Override
public FileTime modificationTime() {
return modificationTime;
}
@Override
public Path path() {
return path;
}
@Override
public String toString() {
return "FileInfo{"
+ "key=" + key + ", "
+ "size=" + size + ", "
+ "modificationTime=" + modificationTime + ", "
+ "path=" + path
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof FileInfo) {
FileInfo that = (FileInfo) o;
return (this.key == null ? that.key() == null : this.key.equals(that.key()))
&& this.size == that.size()
&& (this.modificationTime == null ? that.modificationTime() == null : this.modificationTime.equals(that.modificationTime()))
&& this.path.equals(that.path());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (key == null) ? 0 : key.hashCode();
h$ *= 1000003;
h$ ^= (int) ((size >>> 32) ^ size);
h$ *= 1000003;
h$ ^= (modificationTime == null) ? 0 : modificationTime.hashCode();
h$ *= 1000003;
h$ ^= path.hashCode();
return h$;
}
@Override
protected FileInfo.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends FileInfo.Builder {
private Object key;
private long size;
private FileTime modificationTime;
private Path path;
private byte set$0;
Builder() {
}
private Builder(FileInfo source) {
this.key = source.key();
this.size = source.size();
this.modificationTime = source.modificationTime();
this.path = source.path();
set$0 = (byte) 1;
}
@Override
public FileInfo.Builder key(@Nullable Object key) {
this.key = key;
return this;
}
@Override
public FileInfo.Builder size(long size) {
this.size = size;
set$0 |= (byte) 1;
return this;
}
@Override
public FileInfo.Builder modificationTime(@Nullable FileTime modificationTime) {
this.modificationTime = modificationTime;
return this;
}
@Override
public FileInfo.Builder path(Path path) {
if (path == null) {
throw new NullPointerException("Null path");
}
this.path = path;
return this;
}
@Override
public FileInfo build() {
if (set$0 != 1
|| this.path == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" size");
}
if (this.path == null) {
missing.append(" path");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_FileInfo(
this.key,
this.size,
this.modificationTime,
this.path);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy