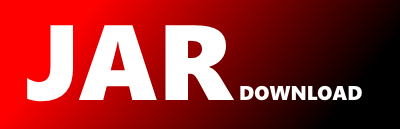
org.graylog2.shared.rest.PrintModelProcessor Maven / Gradle / Ivy
/**
* This file is part of Graylog.
*
* Graylog is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Graylog is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Graylog. If not, see .
*/
package org.graylog2.shared.rest;
import com.google.common.base.Joiner;
import org.glassfish.jersey.server.model.ModelProcessor;
import org.glassfish.jersey.server.model.Resource;
import org.glassfish.jersey.server.model.ResourceMethod;
import org.glassfish.jersey.server.model.ResourceModel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.ws.rs.core.Configuration;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
public class PrintModelProcessor implements ModelProcessor {
private static final Logger LOG = LoggerFactory.getLogger("REST API");
@Override
public ResourceModel processResourceModel(ResourceModel resourceModel, Configuration configuration) {
LOG.debug("Map for resource model <" + resourceModel + ">:");
final List resources = new ArrayList<>();
for (Resource resource : resourceModel.getResources()) {
resources.add(resource);
resources.addAll(findChildResources(resource));
}
logResources(resources);
return resourceModel;
}
@Override
public ResourceModel processSubResource(ResourceModel subResourceModel, Configuration configuration) {
LOG.debug("Map for sub-resource model <" + subResourceModel + ">:");
logResources(subResourceModel.getResources());
return subResourceModel;
}
private void logResources(List resources) {
final List resourceDescriptions = new ArrayList<>();
for (Resource resource : resources) {
for (ResourceMethod resourceMethod : resource.getAllMethods()) {
String path = resource.getPath();
Resource parent = resource.getParent();
while (parent != null) {
if (!path.startsWith("/")) {
path = "/" + path;
}
path = parent.getPath() + path;
parent = parent.getParent();
}
resourceDescriptions.add(new ResourceDescription(resourceMethod.getHttpMethod(), path, resource.getHandlerClasses()));
}
}
Collections.sort(resourceDescriptions);
for (ResourceDescription resource : resourceDescriptions) {
LOG.debug(resource.toString());
}
}
private List findChildResources(Resource parentResource) {
final List childResources = new ArrayList<>();
for (Resource resource : parentResource.getChildResources()) {
childResources.add(resource);
childResources.addAll(findChildResources(resource));
}
return childResources;
}
private static class ResourceDescription implements Comparable {
private String method;
private String path;
private Set> handlerClasses;
private ResourceDescription(String method, String path, Set> handlerClasses) {
this.method = method;
this.path = path;
this.handlerClasses = handlerClasses;
}
@Override
public int compareTo(final ResourceDescription o) {
if (this.path.compareTo(o.path) == 0) {
return this.method.compareTo(o.method);
} else {
return this.path.compareTo(o.path);
}
}
@Override
public String toString() {
return String.format(" %-7s %s (%s)", method, path, Joiner.on(", ").join(handlerClasses));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy