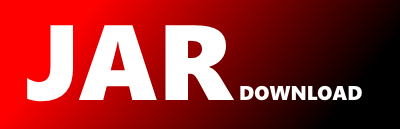
org.greencheek.related.indexing.util.StringBuilderWriter Maven / Gradle / Ivy
/*
*
* * Licensed to Relateit under one or more contributor
* * license agreements. See the NOTICE file distributed with
* * this work for additional information regarding copyright
* * ownership. Relateit licenses this file to you under
* * the Apache License, Version 2.0 (the "License"); you may
* * not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing,
* * software distributed under the License is distributed on an
* * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* * KIND, either express or implied. See the License for the
* * specific language governing permissions and limitations
* * under the License.
*
*/
package org.greencheek.related.indexing.util;
import java.io.IOException;
import java.io.Writer;
/**
* Version of StringWriter that uses a StringBuilder rather than a StringBuffer,
* as the synchronisation of the StringBuffer isn't required.
*
*
*/
class StringBuilderWriter extends Writer {
private StringBuilder buf;
/**
* Create a new string writer using the default initial string-buffer
* size.
*/
public StringBuilderWriter() {
buf = new StringBuilder();
}
/**
* Create a new string writer using the specified initial string-buffer
* size.
*
* @param initialSize The number of char values that will fit into this buffer
* before it is automatically expanded
* @throws IllegalArgumentException If initialSize is negative
*/
public StringBuilderWriter(int initialSize) {
if (initialSize < 0) {
throw new IllegalArgumentException("Negative buffer size");
}
buf = new StringBuilder(initialSize);
}
/**
* Write a single character.
*/
@Override
public void write(int c) {
buf.append((char) c);
}
/**
* Write a portion of an array of characters.
*
* @param cbuf Array of characters
* @param off Offset from which to start writing characters
* @param len Number of characters to write
*/
@Override
public void write(char cbuf[], int off, int len) {
buf.append(cbuf, off, len);
}
/**
* Write a string.
*/
@Override
public void write(String str) {
buf.append(str);
}
/**
* Write a portion of a string.
*
* @param str String to be written
* @param off Offset from which to start writing characters
* @param len Number of characters to write
*/
@Override
public void write(String str, int off, int len) {
buf.append(str, off, off + len);
}
/**
* Appends the specified character sequence to this writer.
*
* An invocation of this method of the form out.append(csq)
* behaves in exactly the same way as the invocation
*
*
* out.write(csq.toUrlQueryTypeString())
*
* Depending on the specification of toUrlQueryTypeString for the
* character sequence csq, the entire sequence may not be
* appended. For instance, invoking the toUrlQueryTypeString method of a
* character buffer will return a subsequence whose content depends upon
* the buffer's position and limit.
*
* @param csq The character sequence to append. If csq is
* null, then the four characters "null" are
* appended to this writer.
* @return This writer
* @since 1.5
*/
@Override
public StringBuilderWriter append(CharSequence csq) {
if (csq == null)
buf.append("null");
else
buf.append(csq);
return this;
}
/**
* Appends a subsequence of the specified character sequence to this writer.
*
* An invocation of this method of the form out.append(csq, start,
* end) when csq is not null, behaves in
* exactly the same way as the invocation
*
*
* out.write(csq.subSequence(start, end).toUrlQueryTypeString())
*
* @param csq The character sequence from which a subsequence will be
* appended. If csq is null, then characters
* will be appended as if csq contained the four
* characters "null".
* @param start The index of the first character in the subsequence
* @param end The index of the character following the last character in the
* subsequence
* @return This writer
* @throws IndexOutOfBoundsException If start or end are negative, start
* is greater than end, or end is greater than
* csq.length()
* @since 1.5
*/
@Override
public StringBuilderWriter append(CharSequence csq, int start, int end) {
CharSequence cs = (csq == null ? "null" : csq);
buf.append(cs, start, end);
return this;
}
/**
* Appends the specified character to this writer.
*
* An invocation of this method of the form out.append(c)
* behaves in exactly the same way as the invocation
*
*
* out.write(c)
*
* @param c The 16-bit character to append
* @return This writer
* @since 1.5
*/
@Override
public StringBuilderWriter append(char c) {
buf.append(c);
return this;
}
/**
* Return the buffer's current value as a string.
*/
@Override
public String toString() {
return buf.toString();
}
/**
* Return the string buffer itself.
*
* @return StringBuffer holding the current buffer value.
*/
public StringBuilder getBuffer() {
return buf;
}
/**
* Flush the stream.
*/
@Override
public void flush() {
}
/**
* Closing a StringWriter has no effect. The methods in this
* class can be called after the stream has been closed without generating
* an IOException.
*/
@Override
public void close() throws IOException {
}
/**
* Writes an array of characters.
*
* @param cbuf
* Array of characters to be written
*
* @throws IOException
* If an I/O error occurs
*/
@Override
public void write(char cbuf[]) throws IOException {
buf.append(cbuf);
}
}