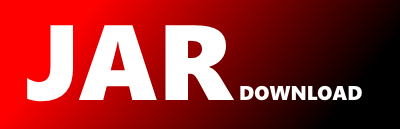
org.greenrobot.greendao.query.AbstractQueryWithLimit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of greendao Show documentation
Show all versions of greendao Show documentation
greenDAO is a light and fast ORM for Android
/*
* Copyright (C) 2011-2016 Markus Junginger, greenrobot (http://greenrobot.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.greenrobot.greendao.query;
import org.greenrobot.greendao.AbstractDao;
import java.util.Date;
/**
* Base class for queries returning data (entities or cursor).
*
* @param The entity class the query will return results for.
* @author Markus
*/
// TODO Query for PKs/ROW IDs
abstract class AbstractQueryWithLimit extends AbstractQuery {
protected final int limitPosition;
protected final int offsetPosition;
protected AbstractQueryWithLimit(AbstractDao dao, String sql, String[] initialValues, int limitPosition,
int offsetPosition) {
super(dao, sql, initialValues);
this.limitPosition = limitPosition;
this.offsetPosition = offsetPosition;
}
/**
* Sets the parameter (0 based) using the position in which it was added during building the query. Note: all
* standard WHERE parameters come first. After that come the WHERE parameters of joins (if any).
*/
public AbstractQueryWithLimit setParameter(int index, Object parameter) {
if (index >= 0 && (index == limitPosition || index == offsetPosition)) {
throw new IllegalArgumentException("Illegal parameter index: " + index);
}
return (AbstractQueryWithLimit) super.setParameter(index, parameter);
}
public AbstractQueryWithLimit setParameter(int index, Date parameter) {
Long converted = parameter != null ? parameter.getTime() : null;
return setParameter(index, converted);
}
public AbstractQueryWithLimit setParameter(int index, Boolean parameter) {
Integer converted = parameter != null ? (parameter ? 1 : 0) : null;
return setParameter(index, converted);
}
/**
* Sets the limit of the maximum number of results returned by this Query. {@link
* org.greenrobot.greendao.query.QueryBuilder#limit(int)} must
* have been called on the QueryBuilder that created this Query object.
*/
public void setLimit(int limit) {
checkThread();
if (limitPosition == -1) {
throw new IllegalStateException("Limit must be set with QueryBuilder before it can be used here");
}
parameters[limitPosition] = Integer.toString(limit);
}
/**
* Sets the offset for results returned by this Query. {@link org.greenrobot.greendao.query.QueryBuilder#offset(int)} must
* have been called on
* the QueryBuilder that created this Query object.
*/
public void setOffset(int offset) {
checkThread();
if (offsetPosition == -1) {
throw new IllegalStateException("Offset must be set with QueryBuilder before it can be used here");
}
parameters[offsetPosition] = Integer.toString(offset);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy