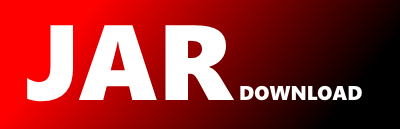
org.greenrobot.greendao.query.CountQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of greendao Show documentation
Show all versions of greendao Show documentation
greenDAO is a light and fast ORM for Android
/*
* Copyright (C) 2011-2016 Markus Junginger, greenrobot (http://greenrobot.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.greenrobot.greendao.query;
import android.database.Cursor;
import org.greenrobot.greendao.AbstractDao;
import org.greenrobot.greendao.DaoException;
public class CountQuery extends AbstractQuery {
private final static class QueryData extends AbstractQueryData> {
private QueryData(AbstractDao dao, String sql, String[] initialValues) {
super(dao, sql, initialValues);
}
@Override
protected CountQuery createQuery() {
return new CountQuery(this, dao, sql, initialValues.clone());
}
}
static CountQuery create(AbstractDao dao, String sql, Object[] initialValues) {
QueryData queryData = new QueryData(dao, sql, toStringArray(initialValues));
return queryData.forCurrentThread();
}
private final QueryData queryData;
private CountQuery(QueryData queryData, AbstractDao dao, String sql, String[] initialValues) {
super(dao, sql, initialValues);
this.queryData = queryData;
}
public CountQuery forCurrentThread() {
return queryData.forCurrentThread(this);
}
/** Returns the count (number of results matching the query). Uses SELECT COUNT (*) sematics. */
public long count() {
checkThread();
Cursor cursor = dao.getDatabase().rawQuery(sql, parameters);
try {
if (!cursor.moveToNext()) {
throw new DaoException("No result for count");
} else if (!cursor.isLast()) {
throw new DaoException("Unexpected row count: " + cursor.getCount());
} else if (cursor.getColumnCount() != 1) {
throw new DaoException("Unexpected column count: " + cursor.getColumnCount());
}
return cursor.getLong(0);
} finally {
cursor.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy