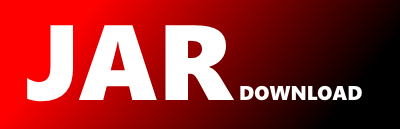
org.greports.utils.ReflectionUtils Maven / Gradle / Ivy
package org.greports.utils;
import org.greports.exceptions.ReportEngineReflectionException;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ReflectionUtils {
private static final List gettersPrefixes = new ArrayList<>(Arrays.asList("get", "is"));
private static final List settersPrefixes = new ArrayList<>(Collections.singletonList("set"));
private ReflectionUtils() {}
public static T newInstance(Class clazz) throws ReportEngineReflectionException {
try {
final Constructor declaredConstructor = clazz.getDeclaredConstructor();
declaredConstructor.setAccessible(true);
return declaredConstructor.newInstance();
} catch (ReflectiveOperationException e) {
throw new ReportEngineReflectionException(String.format(ErrorMessages.SHOULD_HAVE_EMPTY_CONSTRUCTOR, clazz), e, clazz);
}
}
public static Method getMethodWithName(Class clazz, String methodName, Class>... parameters) {
try {
return clazz.getDeclaredMethod(methodName, parameters);
} catch (NoSuchMethodException e) {
if(clazz.getSuperclass() != null){
return getMethodWithName(clazz.getSuperclass(), methodName, parameters);
}
return null;
}
}
public static Method fetchFieldGetter(String fieldName, Class clazz) throws ReportEngineReflectionException {
List getterPossibleNames = generateMethodNames(fieldName, gettersPrefixes);
return catchFieldMethod(getterPossibleNames, clazz, fieldName);
}
public static Method fetchFieldGetter(Field field, Class clazz) throws ReportEngineReflectionException {
List getterPossibleNames = generateMethodNames(field, gettersPrefixes);
return catchFieldMethod(getterPossibleNames, clazz, field.getName());
}
private static Method catchFieldMethod(List getterPossibleNames, Class clazz, String fieldName) throws ReportEngineReflectionException {
for (String getterPossibleName : getterPossibleNames) {
final Method method = getMethodWithName(clazz, getterPossibleName);
if (method != null) {
return method;
}
}
throw new ReportEngineReflectionException(
String.format("No getter was found with any of these names \"%s\" for field %s in class %s", String.join(", ", getterPossibleNames), fieldName, clazz.getName()),
clazz
);
}
public static Method fetchFieldSetter(Field field, Class clazz) throws ReportEngineReflectionException {
List setterPossibleNames = generateMethodNames(field, settersPrefixes);
for (String setterPossibleName : setterPossibleNames) {
final Method method = getMethodWithName(clazz, setterPossibleName, field.getType());
if (method != null) {
return method;
}
}
throw new ReportEngineReflectionException(
String.format("No setter was found with any of these names \"%s\" for field %s in class %s", String.join(", ", setterPossibleNames), field.getName(), clazz.getName()),
clazz
);
}
private static List generateMethodNames(Field field, List prefixes) {
return generateMethodNames(field.getName(), prefixes);
}
private static List generateMethodNames(String fieldName, List prefixes) {
List possibleNames = new ArrayList<>();
prefixes.forEach(prefix -> {
possibleNames.add(prefix + Utils.capitalizeString(fieldName));
possibleNames.add(prefix + fieldName);
});
return possibleNames;
}
public static Object invokeMethod(Method method, Object object) throws ReportEngineReflectionException {
try {
return method.invoke(object);
} catch (IllegalAccessException e) {
throw new ReportEngineReflectionException(ErrorMessages.INV_METHOD_WITH_NO_ACCESS, e, ReflectionUtils.class);
} catch (InvocationTargetException e) {
throw new ReportEngineReflectionException(ErrorMessages.INV_METHOD, e, ReflectionUtils.class);
}
}
public static boolean isListOrArray(Class> clazz){
return clazz != null && (clazz.isArray() || clazz.equals(List.class));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy