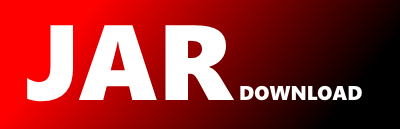
org.gridgain.client.util.GridConcurrentHashSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gridgain-hadoop2 Show documentation
Show all versions of gridgain-hadoop2 Show documentation
Java-based middleware for in-memory processing of big data in a distributed environment.
/*
Copyright (C) GridGain Systems. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
/* _________ _____ __________________ _____
* __ ____/___________(_)______ /__ ____/______ ____(_)_______
* _ / __ __ ___/__ / _ __ / _ / __ _ __ `/__ / __ __ \
* / /_/ / _ / _ / / /_/ / / /_/ / / /_/ / _ / _ / / /
* \____/ /_/ /_/ \_,__/ \____/ \__,_/ /_/ /_/ /_/
*/
package org.gridgain.client.util;
import java.util.*;
import java.util.concurrent.*;
/**
* Wrapper around concurrent map.
*/
public class GridConcurrentHashSet extends AbstractSet {
/** Dummy value. */
protected static final Object VAL = Boolean.TRUE;
/** Base map. */
protected ConcurrentMap map;
/**
* Creates new set based on {@link ConcurrentHashMap}.
*/
public GridConcurrentHashSet() {
this(new ConcurrentHashMap());
}
/**
* Creates new set based on the given map.
*
* @param map Map to be used for set implementation.
*/
@SuppressWarnings({"unchecked"})
public GridConcurrentHashSet(ConcurrentMap map) {
this.map = (ConcurrentMap)map;
}
/**
* Gets wrapped map.
*
* @return Wrapped map.
*/
@SuppressWarnings({"unchecked"})
protected final > T map() {
return (T)map;
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
@Override public boolean add(E e) {
return map.put(e, VAL) == null;
}
/** {@inheritDoc} */
@SuppressWarnings("unchecked")
@Override public Iterator iterator() {
return map.keySet().iterator();
}
/** {@inheritDoc} */
@Override public int size() {
return map.size();
}
/** {@inheritDoc} */
@Override public boolean isEmpty() {
return map.isEmpty();
}
/** {@inheritDoc} */
@SuppressWarnings({"SuspiciousMethodCalls"})
@Override public boolean contains(Object o) {
return map.containsKey(o);
}
/** {@inheritDoc} */
@Override public Object[] toArray() {
return map.keySet().toArray();
}
/** {@inheritDoc} */
@SuppressWarnings({"SuspiciousToArrayCall"})
@Override public T[] toArray(T[] a) {
return map.keySet().toArray(a);
}
/** {@inheritDoc} */
@Override public boolean remove(Object o) {
return map.remove(o) != null;
}
/** {@inheritDoc} */
@Override public void clear() {
map.clear();
}
/** {@inheritDoc} */
@Override public String toString() {
return map.keySet().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy