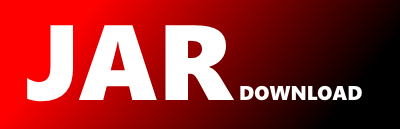
org.gridgain.grid.logger.log4j.GridLog4jLogger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gridgain-hadoop2 Show documentation
Show all versions of gridgain-hadoop2 Show documentation
Java-based middleware for in-memory processing of big data in a distributed environment.
/*
Copyright (C) GridGain Systems. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
/* _________ _____ __________________ _____
* __ ____/___________(_)______ /__ ____/______ ____(_)_______
* _ / __ __ ___/__ / _ __ / _ / __ _ __ `/__ / __ __ \
* / /_/ / _ / _ / / /_/ / / /_/ / / /_/ / _ / _ / / /
* \____/ /_/ /_/ \_,__/ \____/ \__,_/ /_/ /_/ /_/
*/
package org.gridgain.grid.logger.log4j;
import org.apache.log4j.*;
import org.apache.log4j.varia.*;
import org.apache.log4j.xml.*;
import org.gridgain.grid.*;
import org.gridgain.grid.lang.*;
import org.gridgain.grid.logger.*;
import org.gridgain.grid.resources.*;
import org.gridgain.grid.util.*;
import org.gridgain.grid.util.typedef.*;
import org.gridgain.grid.util.typedef.internal.*;
import org.gridgain.grid.util.lang.*;
import org.gridgain.grid.util.tostring.*;
import org.jetbrains.annotations.*;
import java.io.*;
import java.net.*;
import java.util.*;
import static org.gridgain.grid.GridSystemProperties.*;
/**
* Log4j-based implementation for logging. This logger should be used
* by loaders that have prefer log4j-based logging.
*
* Here is a typical example of configuring log4j logger in GridGain configuration file:
*
* <property name="gridLogger">
* <bean class="org.gridgain.grid.logger.log4j.GridLog4jLogger">
* <constructor-arg type="java.lang.String" value="config/gridgain-log4j.xml"/>
* </bean>
* </property>
*
* and from your code:
*
* GridConfiguration cfg = new GridConfiguration();
* ...
* URL xml = U.resolveGridGainUrl("config/custom-log4j.xml");
* GridLogger log = new GridLog4jLogger(xml);
* ...
* cfg.setGridLogger(log);
*
*
* Please take a look at () {
@Override public Logger apply(Boolean init) {
return impl;
}
});
quiet = quiet0;
}
/**
* Creates new logger with given configuration {@code path}.
*
* @param path Path to log4j configuration XML file.
* @throws GridException Thrown in case logger can't be created.
*/
public GridLog4jLogger(String path) throws GridException {
if (path == null)
throw new GridException("Configuration XML file for Log4j must be specified.");
this.path = path;
final URL cfgUrl = U.resolveGridGainUrl(path);
if (cfgUrl == null)
throw new GridException("Log4j configuration path was not found: " + path);
addConsoleAppenderIfNeeded(null, new C1() {
@Override public Logger apply(Boolean init) {
if (init)
DOMConfigurator.configure(cfgUrl);
return Logger.getRootLogger();
}
});
quiet = quiet0;
}
/**
* Creates new logger with given configuration {@code cfgFile}.
*
* @param cfgFile Log4j configuration XML file.
* @throws GridException Thrown in case logger can't be created.
*/
public GridLog4jLogger(File cfgFile) throws GridException {
if (cfgFile == null)
throw new GridException("Configuration XML file for Log4j must be specified.");
if (!cfgFile.exists() || cfgFile.isDirectory())
throw new GridException("Log4j configuration path was not found or is a directory: " + cfgFile);
path = cfgFile.getAbsolutePath();
addConsoleAppenderIfNeeded(null, new C1() {
@Override public Logger apply(Boolean init) {
if (init)
DOMConfigurator.configure(path);
return Logger.getRootLogger();
}
});
quiet = quiet0;
}
/**
* Creates new logger with given configuration {@code cfgUrl}.
*
* @param cfgUrl URL for Log4j configuration XML file.
* @throws GridException Thrown in case logger can't be created.
*/
public GridLog4jLogger(final URL cfgUrl) throws GridException {
if (cfgUrl == null)
throw new GridException("Configuration XML file for Log4j must be specified.");
path = null;
addConsoleAppenderIfNeeded(null, new C1() {
@Override public Logger apply(Boolean init) {
if (init)
DOMConfigurator.configure(cfgUrl);
return Logger.getRootLogger();
}
});
quiet = quiet0;
}
/**
* Checks if Log4j is already configured within this VM or not.
*
* @return {@code True} if log4j was already configured, {@code false} otherwise.
*/
public static boolean isConfigured() {
return Logger.getRootLogger().getAllAppenders().hasMoreElements();
}
/**
* Sets level for internal log4j implementation.
*
* @param level Log level to set.
*/
public void setLevel(Level level) {
impl.setLevel(level);
}
/**
* Gets name of the file to log to if file appender is enabled, {@code null} otherwise.
*
* @return Name of the file to log to or {@code null} if one does not exist.
*/
@Nullable public String fileName() {
FileAppender fapp = F.first(fileAppenders);
return fapp != null ? fapp.getFile() : null;
}
/**
* Adds console appender when needed with some default logging settings.
*
* @param logLevel Optional log level.
* @param implInitC Optional log implementation init closure.
*/
private void addConsoleAppenderIfNeeded(@Nullable Level logLevel,
@Nullable GridClosure implInitC) {
if (inited) {
if (implInitC != null)
// Do not init.
impl = implInitC.apply(false);
return;
}
synchronized (mux) {
if (inited) {
if (implInitC != null)
// Do not init.
impl = implInitC.apply(false);
return;
}
if (implInitC != null)
// Init logger impl.
impl = implInitC.apply(true);
boolean quiet = Boolean.valueOf(System.getProperty(GG_QUIET, "true"));
boolean consoleAppenderFound = false;
Category rootCategory = null;
ConsoleAppender errAppender = null;
for (Category l = impl; l != null; ) {
if (!consoleAppenderFound) {
for (Enumeration appenders = l.getAllAppenders(); appenders.hasMoreElements(); ) {
Appender appender = (Appender)appenders.nextElement();
if (appender instanceof ConsoleAppender) {
if ("CONSOLE_ERR".equals(appender.getName())) {
// Treat CONSOLE_ERR appender as a system one and don't count it.
errAppender = (ConsoleAppender)appender;
continue;
}
consoleAppenderFound = true;
break;
}
}
}
if (l.getParent() == null) {
rootCategory = l;
break;
}
else
l = l.getParent();
}
if (consoleAppenderFound && quiet)
// User configured console appender, but log is quiet.
quiet = false;
if (!consoleAppenderFound && !quiet && Boolean.valueOf(System.getProperty(GG_CONSOLE_APPENDER, "true"))) {
// Console appender not found => we've looked through all categories up to root.
assert rootCategory != null;
// User launched gridgain in verbose mode and did not add console appender with INFO level
// to configuration and did not set GG_CONSOLE_APPENDER to false.
if (errAppender != null) {
rootCategory.addAppender(createConsoleAppender(Level.INFO));
if (errAppender.getThreshold() == Level.ERROR)
errAppender.setThreshold(Level.WARN);
}
else
// No error console appender => create console appender with no level limit.
rootCategory.addAppender(createConsoleAppender(Level.OFF));
if (logLevel != null)
impl.setLevel(logLevel);
}
quiet0 = quiet;
inited = true;
}
}
/**
* Creates console appender with some reasonable default logging settings.
*
* @param maxLevel Max logging level.
* @return New console appender.
*/
private Appender createConsoleAppender(Level maxLevel) {
String fmt = "[%d{ABSOLUTE}][%-5p][%t][%c{1}] %m%n";
// Configure output that should go to System.out
Appender app = new ConsoleAppender(new PatternLayout(fmt), ConsoleAppender.SYSTEM_OUT);
LevelRangeFilter lvlFilter = new LevelRangeFilter();
lvlFilter.setLevelMin(Level.TRACE);
lvlFilter.setLevelMax(maxLevel);
app.addFilter(lvlFilter);
return app;
}
/**
* Adds file appender.
*
* @param a Appender.
*/
public static void addAppender(FileAppender a) {
A.notNull(a, "a");
fileAppenders.add(a);
}
/**
* Removes file appender.
*
* @param a Appender.
*/
public static void removeAppender(FileAppender a) {
A.notNull(a, "a");
fileAppenders.remove(a);
}
/**
* Sets node ID on all file appenders.
*
* @param nodeId Node ID.
*/
public static void setNodeId(UUID nodeId) {
A.notNull(nodeId, "nodeId");
for (FileAppender a : fileAppenders) {
if (a instanceof GridLog4jNodeIdSupported) {
((GridLog4jNodeIdSupported)a).setNodeId(nodeId);
a.activateOptions();
}
}
}
/**
* Gets files for all registered file appenders.
*
* @return List of files.
*/
public static Collection logFiles() {
Collection res = new ArrayList<>(fileAppenders.size());
for (FileAppender a : fileAppenders)
res.add(a.getFile());
return res;
}
/**
* Gets {@link GridLogger} wrapper around log4j logger for the given
* category. If category is {@code null}, then root logger is returned. If
* category is an instance of {@link Class} then {@code (Class)ctgr).getName()}
* is used as category name.
*
* @param ctgr {@inheritDoc}
* @return {@link GridLogger} wrapper around log4j logger.
*/
@Override public GridLog4jLogger getLogger(Object ctgr) {
return new GridLog4jLogger(ctgr == null ? Logger.getRootLogger() :
ctgr instanceof Class ? Logger.getLogger(((Class>)ctgr).getName()) :
Logger.getLogger(ctgr.toString()));
}
/** {@inheritDoc} */
@Override public void trace(String msg) {
if (!impl.isTraceEnabled())
warning("Logging at TRACE level without checking if TRACE level is enabled: " + msg);
impl.trace(msg);
}
/** {@inheritDoc} */
@Override public void debug(String msg) {
if (!impl.isDebugEnabled())
warning("Logging at DEBUG level without checking if DEBUG level is enabled: " + msg);
impl.debug(msg);
}
/** {@inheritDoc} */
@Override public void info(String msg) {
if (!impl.isInfoEnabled())
warning("Logging at INFO level without checking if INFO level is enabled: " + msg);
impl.info(msg);
}
/** {@inheritDoc} */
@Override public void warning(String msg) {
impl.warn(msg);
}
/** {@inheritDoc} */
@Override public void warning(String msg, @Nullable Throwable e) {
impl.warn(msg, e);
}
/** {@inheritDoc} */
@Override public void error(String msg) {
impl.error(msg);
}
/** {@inheritDoc} */
@Override public void error(String msg, @Nullable Throwable e) {
impl.error(msg, e);
}
/** {@inheritDoc} */
@Override public boolean isTraceEnabled() {
return impl.isTraceEnabled();
}
/** {@inheritDoc} */
@Override public boolean isDebugEnabled() {
return impl.isDebugEnabled();
}
/** {@inheritDoc} */
@Override public boolean isInfoEnabled() {
return impl.isInfoEnabled();
}
/** {@inheritDoc} */
@Override public boolean isQuiet() {
return quiet;
}
/** {@inheritDoc} */
@Override public String toString() {
return S.toString(GridLog4jLogger.class, this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy