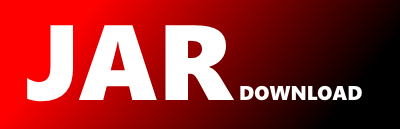
org.gridgain.grid.util.lang.GridClosure3 Maven / Gradle / Ivy
/*
Copyright (C) GridGain Systems. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
/* _________ _____ __________________ _____
* __ ____/___________(_)______ /__ ____/______ ____(_)_______
* _ / __ __ ___/__ / _ __ / _ / __ _ __ `/__ / __ __ \
* / /_/ / _ / _ / / /_/ / / /_/ / / /_/ / _ / _ / / /
* \____/ /_/ /_/ \_,__/ \____/ \__,_/ /_/ /_/ /_/
*/
package org.gridgain.grid.util.lang;
import org.gridgain.grid.util.typedef.*;
/**
* Defines generic {@code for-each} type of closure. Closure is a first-class function that is defined with
* (or closed over) its free variables that are bound to the closure scope at execution. Since
* Java 6 doesn't provide a language construct for first-class function the closures are implemented
* as abstract classes.
* Type Alias
* To provide for more terse code you can use a typedef {@link C3} class or various factory methods in
* {@link GridFunc} class. Note, however, that since typedefs in Java rely on inheritance you should
* not use these type aliases in signatures.
* Thread Safety
* Note that this interface does not impose or assume any specific thread-safety by its
* implementations. Each implementation can elect what type of thread-safety it provides,
* if any.
* @param Type of the first free variable, i.e. the element the closure is called or closed on.
* @param Type of the second free variable, i.e. the element the closure is called or closed on.
* @param Type of the third free variable, i.e. the element the closure is called or closed on.
* @param Type of the closure's return value.
* @see C3
* @see GridFunc
*/
public interface GridClosure3 {
/**
* Closure body.
*
* @param e1 First bound free variable, i.e. the element the closure is called or closed on.
* @param e2 Second bound free variable, i.e. the element the closure is called or closed on.
* @param e3 Third bound free variable, i.e. the element the closure is called or closed on.
* @return Optional return value.
*/
public R apply(E1 e1, E2 e2, E3 e3);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy