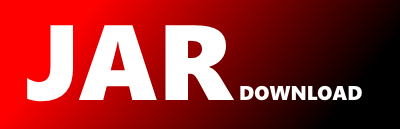
net.java.btrace.ext.Numbers Maven / Gradle / Ivy
/*
* Copyright (c) 2007, 2011, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package net.java.btrace.ext;
import net.java.btrace.api.extensions.BTraceExtension;
/*
* Wraps the numbers related BTrace utility methods
* @since 1.3
*/
@BTraceExtension
public class Numbers {
/**
* Returns a double
value with a positive sign, greater
* than or equal to 0.0
and less than 1.0
.
* Returned values are chosen pseudorandomly with (approximately)
* uniform distribution from that range.
*/
public static double random() {
return Math.random();
}
/**
* Returns the natural logarithm (base e) of a double
* value. Special cases:
* - If the argument is NaN or less than zero, then the result
* is NaN.
*
- If the argument is positive infinity, then the result is
* positive infinity.
*
- If the argument is positive zero or negative zero, then the
* result is negative infinity.
*
* The computed result must be within 1 ulp of the exact result.
* Results must be semi-monotonic.
*
* @param a a value
* @return the value ln a
, the natural logarithm of
* a
.
*/
public strictfp static double log(double a) {
return Math.log(a);
}
/**
* Returns the base 10 logarithm of a double
value.
* Special cases:
*
*
- If the argument is NaN or less than zero, then the result
* is NaN.
*
- If the argument is positive infinity, then the result is
* positive infinity.
*
- If the argument is positive zero or negative zero, then the
* result is negative infinity.
*
- If the argument is equal to 10n for
* integer n, then the result is n.
*
*
* The computed result must be within 1 ulp of the exact result.
* Results must be semi-monotonic.
*
* @param a a value
* @return the base 10 logarithm of a
.
*/
public strictfp static double log10(double a) {
return Math.log10(a);
}
/**
* Returns Euler's number e raised to the power of a
* double
value. Special cases:
*
- If the argument is NaN, the result is NaN.
*
- If the argument is positive infinity, then the result is
* positive infinity.
*
- If the argument is negative infinity, then the result is
* positive zero.
*
* The computed result must be within 1 ulp of the exact result.
* Results must be semi-monotonic.
*
* @param a the exponent to raise e to.
* @return the value ea
,
* where e is the base of the natural logarithms.
*/
public strictfp static double exp(double a) {
return Math.exp(a);
}
/**
* Returns true
if the specified number is a
* Not-a-Number (NaN) value, false
otherwise.
*
* @param d the value to be tested.
* @return true
if the value of the argument is NaN;
* false
otherwise.
*/
public static boolean isNaN(double d) {
return Double.isNaN(d);
}
/**
* Returns true
if the specified number is a
* Not-a-Number (NaN) value, false
otherwise.
*
* @param f the value to be tested.
* @return true
if the value of the argument is NaN;
* false
otherwise.
*/
public static boolean isNaN(float f) {
return Float.isNaN(f);
}
/**
* Returns true
if the specified number is infinitely
* large in magnitude, false
otherwise.
*
* @param d the value to be tested.
* @return true
if the value of the argument is positive
* infinity or negative infinity; false
otherwise.
*/
public static boolean isInfinite(double d) {
return Double.isInfinite(d);
}
/**
* Returns true
if the specified number is infinitely
* large in magnitude, false
otherwise.
*
* @param f the value to be tested.
* @return true
if the value of the argument is positive
* infinity or negative infinity; false
otherwise.
*/
public static boolean isInfinite(float f) {
return Float.isInfinite(f);
}
// string parsing methods
/**
* Parses the string argument as a boolean. The boolean
* returned represents the value true
if the string argument
* is not null
and is equal, ignoring case, to the string
* {@code "true"}.
* Example: {@code Boolean.parseBoolean("True")} returns true.
* Example: {@code Boolean.parseBoolean("yes")} returns false.
*
* @param s the String
containing the boolean
* representation to be parsed
* @return the boolean represented by the string argument
*/
public static boolean parseBoolean(String s) {
return Boolean.parseBoolean(s);
}
/**
* Parses the string argument as a signed decimal
* byte
. The characters in the string must all be
* decimal digits, except that the first character may be an ASCII
* minus sign '-'
('\u002D'
) to
* indicate a negative value. The resulting byte
value is
* returned.
*
* @param s a String
containing the
* byte
representation to be parsed
* @return the byte
value represented by the
* argument in decimal
*/
public static byte parseByte(String s) {
return Byte.parseByte(s);
}
/**
* Parses the string argument as a signed decimal
* short
. The characters in the string must all be
* decimal digits, except that the first character may be an ASCII
* minus sign '-'
('\u002D'
) to
* indicate a negative value. The resulting short
value is
* returned.
*
* @param s a String
containing the short
* representation to be parsed
* @return the short
value represented by the
* argument in decimal.
*/
public static short parseShort(String s) {
return Short.parseShort(s);
}
/**
* Parses the string argument as a signed decimal integer. The
* characters in the string must all be decimal digits, except that
* the first character may be an ASCII minus sign '-'
* ('\u002D'
) to indicate a negative value. The resulting
* integer value is returned.
*
* @param s a String
containing the int
* representation to be parsed
* @return the integer value represented by the argument in decimal.
*/
public static int parseInt(String s) {
return Integer.parseInt(s);
}
/**
* Parses the string argument as a signed decimal
* long
. The characters in the string must all be
* decimal digits, except that the first character may be an ASCII
* minus sign '-'
(\u002D'
) to
* indicate a negative value. The resulting long
* value is returned.
*
* Note that neither the character L
* ('\u004C'
) nor l
* ('\u006C'
) is permitted to appear at the end
* of the string as a type indicator, as would be permitted in
* Java programming language source code.
*
* @param s a String
containing the long
* representation to be parsed
* @return the long
represented by the argument in
* decimal.
*/
public static long parseLong(String s) {
return Long.parseLong(s);
}
/**
* Returns a new float
initialized to the value
* represented by the specified String
, as performed
* by the valueOf
method of class Float
.
*
* @param s the string to be parsed.
* @return the float
value represented by the string
* argument.
*/
public static float parseFloat(String s) {
return Float.parseFloat(s);
}
/**
* Returns a new double
initialized to the value
* represented by the specified String
, as performed
* by the valueOf
methcod of class
* Double
.
*
* @param s the string to be parsed.
* @return the double
value represented by the string
* argument.
*/
public static double parseDouble(String s) {
return Double.parseDouble(s);
}
// boxing methods
/**
* Returns a Boolean instance representing the specified
* boolean value. If the specified boolean value
* is true, this method returns Boolean.TRUE;
* if it is false, this method returns Boolean.FALSE.
*
* @param b a boolean value.
* @return a Boolean instance representing b.
*/
public static Boolean box(boolean b) {
return Boolean.valueOf(b);
}
/**
* Returns a Character instance representing the specified
* char value.
*
* @param c a char value.
* @return a Character instance representing c.
*/
public static Character box(char c) {
return Character.valueOf(c);
}
/**
* Returns a Byte instance representing the specified
* byte value.
*
* @param b a byte value.
* @return a Byte instance representing b.
*/
public static Byte box(byte b) {
return Byte.valueOf(b);
}
/**
* Returns a Short instance representing the specified
* short value.
*
* @param s a short value.
* @return a Short instance representing s.
*/
public static Short box(short s) {
return Short.valueOf(s);
}
/**
* Returns a Integer instance representing the specified
* int value.
*
* @param i an int
value.
* @return a Integer instance representing i.
*/
public static Integer box(int i) {
return Integer.valueOf(i);
}
/**
* Returns a Long instance representing the specified
* long value.
*
* @param l a long value.
* @return a Long instance representing l.
*/
public static Long box(long l) {
return Long.valueOf(l);
}
/**
* Returns a Float instance representing the specified
* float value.
*
* @param f a float value.
* @return a Float instance representing f.
*/
public static Float box(float f) {
return Float.valueOf(f);
}
/**
* Returns a Double instance representing the specified
* double value.
*
* @param d a double value.
* @return a Double instance representing d.
*/
public static Double box(double d) {
return Double.valueOf(d);
}
// unboxing methods
/**
* Returns the value of the given Boolean object as a boolean
* primitive.
*
* @param b the Boolean object whose value is returned.
* @return the primitive boolean
value of the object.
*/
public static boolean unbox(Boolean b) {
return b.booleanValue();
}
/**
* Returns the value of the given Character object as a char
* primitive.
*
* @param ch the Character object whose value is returned.
* @return the primitive char
value of the object.
*/
public static char unbox(Character ch) {
return ch.charValue();
}
/**
* Returns the value of the specified Byte as a byte
.
*
* @param b Byte that is unboxed
* @return the byte value represented by the Byte
.
*/
public static byte unbox(Byte b) {
return b.byteValue();
}
/**
* Returns the short value represented by Short
.
*
* @param s Short that is unboxed.
* @return the short value represented by the Short
.
*/
public static short unbox(Short s) {
return s.shortValue();
}
/**
* Returns the value of represented by Integer
.
*
* @param i Integer that is unboxed.
* @return the int value represented by the Integer
.
*/
public static int unbox(Integer i) {
return i.intValue();
}
/**
* Returns the long value represented by the specified Long
.
*
* @param l Long to be unboxed.
* @return the long value represented by the Long
.
*/
public static long unbox(Long l) {
return l.longValue();
}
/**
* Returns the float value represented by the specified Float
.
*
* @param f Float to be unboxed.
* @return the float value represented by the Float
.
*/
public static float unbox(Float f) {
return f.floatValue();
}
/**
* Returns the double value represented by the specified Double
.
*
* @param d Double to be unboxed.
*/
public static double unbox(Double d) {
return d.doubleValue();
}
}