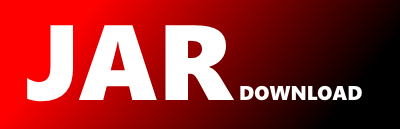
org.gridkit.coherence.search.PlugableSearchIndex Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2011 Alexey Ragozin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.gridkit.coherence.search;
import java.util.Map;
import java.util.Set;
import com.tangosol.util.filter.IndexAwareFilter;
/**
* SPI interface to be implemented by provided of custom index engine.
* @author Alexey Ragozin ([email protected])
*
* @param type of index instance
* @param type of index configuration options, passed to index up on creation
* @param type of query object for this index plugin
*/
public interface PlugableSearchIndex {
/**
* Due to usage of generic extractors and filters. A some token is required to distinguish
* different custom indexes.
* Token object should be compatible with cache serializer.
*/
public Object createIndexCompatibilityToken(IC indexConfig);
/**
* Creates instance of index structure using config.
* @param indexConfig
* @return index instance
*/
public I createIndexInstance(IC indexConfig);
/**
* Plugin has a last chance to enforce engine compabilities required
* to index functioning. This method is being called just befor index creation and
* may override settings done on {@link SearchFactory} level.
* @param config
*/
public void configure(IndexEngineConfig config);
/**
* Updates instance of index using change set map.
*/
public void updateIndexEntries(I index, Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy