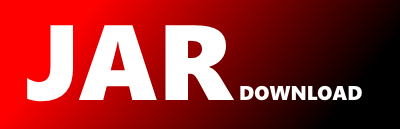
org.groovymc.modsdotgroovy.gradle.tasks.AbstractMDGConvertTask.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-plugin Show documentation
Show all versions of gradle-plugin Show documentation
A Gradle plugin for creation of mods metadata file from a groovy file
The newest version!
package org.groovymc.modsdotgroovy.gradle.tasks
import groovy.json.JsonSlurper
import groovy.transform.CompileStatic
import org.codehaus.groovy.runtime.StringGroovyMethods
import org.gradle.api.DefaultTask
import org.gradle.api.file.ConfigurableFileCollection
import org.gradle.api.file.Directory
import org.gradle.api.file.ProjectLayout
import org.gradle.api.file.RegularFileProperty
import org.gradle.api.provider.MapProperty
import org.gradle.api.provider.Property
import org.gradle.api.services.ServiceReference
import org.gradle.api.tasks.*
import org.gradle.work.NormalizeLineEndings
import org.groovymc.modsdotgroovy.types.core.Platform
import org.groovymc.modsdotgroovy.gradle.internal.MapUtils
import org.groovymc.modsdotgroovy.gradle.internal.ConvertService
import org.groovymc.modsdotgroovy.types.runner.FilteredStream
import org.jetbrains.annotations.ApiStatus
import javax.inject.Inject
import java.nio.file.Files
@CacheableTask
@CompileStatic
abstract class AbstractMDGConvertTask extends DefaultTask {
@InputFile
@NormalizeLineEndings
@PathSensitive(PathSensitivity.NONE)
abstract RegularFileProperty getInput()
@OutputFile
abstract RegularFileProperty getOutput()
// included in the output file name
@Internal
abstract Property getOutputName()
@CompileClasspath
abstract ConfigurableFileCollection getMdgRuntimeFiles()
@Input
@Optional
abstract MapProperty getBuildProperties()
@Input
@Optional
abstract Property getPlatform()
@Input
abstract Property getIsMultiplatform()
@Input
abstract Property getProjectVersion()
@Input
abstract Property getProjectGroup()
/**
* Determines whether the MDG conversion should be run in offline mode. If this is set to true, any attempts during
* the conversion process to download files will be skipped. If this is false but gradle runs in offline mode, such
* attempts in the conversion will cause it to fail.
*/
@Input
abstract Property getOffline()
/**
* A file containing platform-specific details, such as the Minecraft version and loader version.
* Generated by a task that extends {@link AbstractGatherPlatformDetailsTask}
*/
@InputFile
@NormalizeLineEndings
@PathSensitive(PathSensitivity.NONE)
abstract RegularFileProperty getPlatformDetailsFile()
@Inject
protected abstract ProjectLayout getProjectLayout()
@ServiceReference('org.groovymc.modsdotgroovy.gradle.internal.ConvertService')
@ApiStatus.Internal
protected abstract Property getConvertService()
@InputFiles
@Classpath
@ApiStatus.Internal
protected abstract ConfigurableFileCollection getRunnerClasspath()
// Should not be used for caching -- if ran offline, the task will either fail or produce the same output
@ApiStatus.Internal
@Internal
protected abstract Property getGradleOffline()
AbstractMDGConvertTask() {
// default to e.g. build/modsDotGroovyToToml/mods.toml
output.convention(projectLayout.buildDirectory.dir('generated/modsDotGroovy/' + name.replaceFirst('ConvertTo', 'modsDotGroovyTo')).map((Directory dir) -> dir.file(outputName.get())))
output.finalizeValueOnRead()
projectVersion.convention(project.provider(() -> project.version.toString()))
projectGroup.convention(project.provider(() -> project.group.toString()))
isMultiplatform.convention(project.provider(() -> false))
runnerClasspath.from(project.configurations.maybeCreate('modsDotGroovyRunnerClasspath'))
offline.convention(project.providers.gradleProperty('org.groovymc.modsdotgroovy.offline').map(StringGroovyMethods::toBoolean).orElse(false))
gradleOffline.convention(project.gradle.startParameter.offline)
}
protected abstract String writeData(Map data)
@TaskAction
void run() {
final input = input.get().asFile
if (!input.exists()) {
logger.warn("Input file {} for task '{}' could not be found!", input, name)
return
}
final Map data = FilteredStream.convertToSerializable(from(input))
final outPath = output.get().asFile.toPath()
if (outPath.parent !== null && !Files.exists(outPath.parent))
Files.createDirectories(outPath.parent)
Files.deleteIfExists(outPath)
Files.writeString(outPath, writeData(data))
}
protected Map from(File script) {
Map bindingValues = [
buildProperties: buildProperties.get(),
version: projectVersion.get(),
group: projectGroup.get(),
]
bindingValues.conversionSettings = [
onlineBehavior: offline.get() ? 'SKIP' : (gradleOffline.get() ? 'ERROR' : 'ALLOW')
]
final json = new JsonSlurper()
bindingValues = MapUtils.recursivelyMergeOnlyMaps(bindingValues, json.parse(platformDetailsFile.get().asFile) as Map)
return convertService.get().run(getRunnerClasspath().getAsPath(), mdgRuntimeFiles.files.collect { it.toURI().toURL() }.toArray(URL[]::new), script, platform.get(), isMultiplatform.get(), FilteredStream.convertToSerializable(bindingValues) as Map)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy