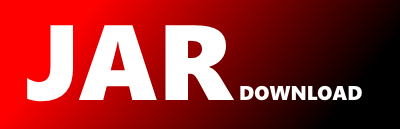
org.grouplens.lenskit.eval.metrics.ResultConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lenskit-eval Show documentation
Show all versions of lenskit-eval Show documentation
Facilities for evaluating recommender algorithms.
/*
* LensKit, an open source recommender systems toolkit.
* Copyright 2010-2014 LensKit Contributors. See CONTRIBUTORS.md.
* Work on LensKit has been funded by the National Science Foundation under
* grants IIS 05-34939, 08-08692, 08-12148, and 10-17697.
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program; if not, write to the Free Software Foundation, Inc., 51
* Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.grouplens.lenskit.eval.metrics;
import com.google.common.base.Function;
import com.google.common.base.Throwables;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.common.primitives.Ints;
import javax.annotation.Nullable;
import java.lang.reflect.AnnotatedElement;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.List;
/**
* Utility class for converting typed results into row data for table writers.
*
* @author GroupLens Research
* @since 2.1
*/
public class ResultConverter {
private final Class resultType;
private final List columns;
private final List columnLabels;
ResultConverter(Class type, List cols) {
resultType = type;
columns = ImmutableList.copyOf(cols);
columnLabels = Lists.transform(columns, new Function() {
@Nullable
@Override
public String apply(@Nullable Column input) {
assert input != null;
return input.getName();
}
});
}
public Class getResultType() {
return resultType;
}
/**
* Get the labels of the columns produced by this converter.
* @return The column labels.
*/
public List getColumnLabels() {
return columnLabels;
}
/**
* Get the column values for a result instance.
* @param result The result instance.
* @return The list of column values.
*/
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy