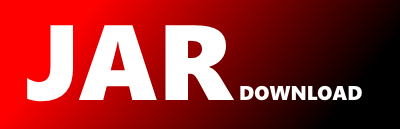
org.grouplens.lenskit.eval.traintest.JobGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lenskit-eval Show documentation
Show all versions of lenskit-eval Show documentation
Facilities for evaluating recommender algorithms.
/*
* LensKit, an open source recommender systems toolkit.
* Copyright 2010-2014 LensKit Contributors. See CONTRIBUTORS.md.
* Work on LensKit has been funded by the National Science Foundation under
* grants IIS 05-34939, 08-08692, 08-12148, and 10-17697.
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program; if not, write to the Free Software Foundation, Inc., 51
* Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
package org.grouplens.lenskit.eval.traintest;
import com.google.common.collect.ImmutableSet;
import com.google.common.io.Files;
import org.grouplens.grapht.Component;
import org.grouplens.grapht.Dependency;
import org.grouplens.grapht.graph.DAGNode;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Collection;
import java.util.Iterator;
import java.util.Set;
import java.util.concurrent.Callable;
/**
* @author GroupLens Research
*/
class JobGraph {
public static Node noopNode(String label) {
return new NoopNode(label);
}
public static Node jobNode(TrainTestJob job) {
return new JobNode(job);
}
public static Edge edge() {
return edge(ImmutableSet.>of());
}
public static Edge edge(Set> deps) {
return new Edge(deps);
}
public static void writeGraphDescription(DAGNode jobGraph, File taskGraphFile) throws IOException {
Files.createParentDirs(taskGraphFile);
PrintWriter print = new PrintWriter(taskGraphFile);
try {
for (DAGNode node: jobGraph.getSortedNodes()) {
print.print("- ");
print.println(node.getLabel());
Set> deps = node.getAdjacentNodes();
if (!deps.isEmpty()) {
print.println(" dependencies:");
for (DAGNode dep: deps) {
print.print(" - ");
print.println(dep.getLabel());
}
}
}
} finally {
print.close();
}
}
public abstract static interface Node extends Callable {
TrainTestJob getJob();
}
static class NoopNode implements Node {
private final String label;
public NoopNode(String lbl) {
label = lbl;
}
@Override
public Void call() throws Exception {
return null;
}
@Override
public String toString() {
return label;
}
@Override
public TrainTestJob getJob() {
return null;
}
}
static class JobNode implements Node {
TrainTestJob job;
JobNode(TrainTestJob job) {
this.job = job;
}
@Override
public TrainTestJob getJob() {
return job;
}
@Override
public Void call() throws Exception {
return job.call();
}
@Override
public String toString() {
return job.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
JobNode jobNode = (JobNode) o;
if (job != null ? !job.equals(jobNode.job) : jobNode.job != null) return false;
return true;
}
@Override
public int hashCode() {
return job != null ? job.hashCode() : 0;
}
}
public static class Edge implements Set> {
private final Set> dependencies;
public Edge(Set> deps) {
this.dependencies = ImmutableSet.copyOf(deps);
}
@Override
public int size() {
return dependencies.size();
}
@Override
public boolean isEmpty() {
return dependencies.isEmpty();
}
@Override
public boolean contains(Object o) {
return dependencies.contains(o);
}
@Override
public Iterator> iterator() {
return dependencies.iterator();
}
@Override
public Object[] toArray() {
return dependencies.toArray();
}
@Override
public T[] toArray(T[] a) {
return dependencies.toArray(a);
}
@Override
public boolean add(DAGNode ComponentDependencyDAGNode) {
return dependencies.add(ComponentDependencyDAGNode);
}
@Override
public boolean remove(Object o) {
return dependencies.remove(o);
}
@Override
public boolean containsAll(Collection> c) {
return dependencies.containsAll(c);
}
@Override
public boolean addAll(Collection extends DAGNode> c) {
return dependencies.addAll(c);
}
@Override
public boolean retainAll(Collection> c) {
return dependencies.retainAll(c);
}
@Override
public boolean removeAll(Collection> c) {
return dependencies.removeAll(c);
}
@Override
public void clear() {
dependencies.clear();
}
@Override
public boolean equals(Object o) {
return dependencies.equals(o);
}
@Override
public int hashCode() {
return dependencies.hashCode();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy