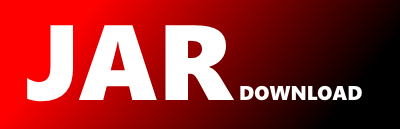
org.gvnix.web.datatables.util.DatatablesUtilsBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.gvnix.web.datatables Show documentation
Show all versions of org.gvnix.web.datatables Show documentation
Dandelion-DataTables utilities for Spring MVC based projects.
/*
* Copyright 2015 DiSiD Technologies S.L.L. All rights reserved.
*
* Project : DiSiD org.gvnix.web.datatables
* SVN Id : $Id$
*/
package org.gvnix.web.datatables.util;
import java.text.DateFormat;
import java.util.List;
import java.util.Map;
import javax.persistence.EntityManager;
import javax.servlet.http.HttpServletRequest;
import org.gvnix.web.datatables.query.SearchResults;
import org.springframework.core.convert.ConversionService;
import com.github.dandelion.datatables.core.ajax.ColumnDef;
import com.github.dandelion.datatables.core.ajax.DataSet;
import com.github.dandelion.datatables.core.ajax.DatatablesCriterias;
import com.github.dandelion.datatables.core.export.ExportConf;
import com.github.dandelion.datatables.core.html.HtmlTable;
import com.mysema.query.BooleanBuilder;
import com.mysema.query.jpa.impl.JPAQuery;
import com.mysema.query.types.path.PathBuilder;
public interface DatatablesUtilsBean {
public static final String ISNULL_OPE = "ISNULL";
public static final String NOTNULL_OPE = "NOTNULL";
public static final String G_ISNULL_OPE = "global.filters.operations.all.isnull";
public static final String G_NOTNULL_OPE = "global.filters.operations.all.notnull";
public static final String ROWS_ON_TOP_IDS_PARAM = "dtt_row_on_top_ids";
public static final String SEPARATOR_FIELDS = ".";
public static final String SEPARATOR_FIELDS_ESCAPED = "_~~_";
/**
* Creates and returns a new JPAQuery instance for the provided
* EntityManager
*
* @param em ActiveRecord JPA EntityManager
* @return JPAQuery instance for provided EntityManager
*/
public JPAQuery newJPAQuery(EntityManager em);
/**
*
*
* @param name
* @return
*/
public boolean isSpecialFilterParameters(String name);
/**
* Execute a select query on entityClass using {@code DatatablesCriterias}
* information for filter, sort and paginate result.
*
* @param entityClass entity to use in search
* @param datatablesCriterias datatables parameters for query
* @return
*/
public SearchResults findByCriteria(Class entityClass,
DatatablesCriterias datatablesCriterias);
/**
* Execute a select query on entityClass using {@code DatatablesCriterias}
* information for filter, sort and paginate result.
*
* @param entityClass entity to use in search
* @param datatablesCriterias datatables parameters for query
* @param baseSearchValuesMap (optional) base filter values
* @return
*/
public SearchResults findByCriteria(Class entityClass,
DatatablesCriterias datatablesCriterias,
Map baseSearchValuesMap);
/**
* Execute a select query on entityClass using {@code DatatablesCriterias}
* information for filter, sort and paginate result.
*
* @param entityClass entity to use in search
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @return
*/
public SearchResults findByCriteria(Class entityClass,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias);
/**
* Execute a select query on entityClass using {@code DatatablesCriterias}
* information for filter, sort and paginate result.
*
* @param entityClass entity to use in search
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param baseSearchValuesMap (optional) base filter values
* @return
*/
public SearchResults findByCriteria(Class entityClass,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
Map baseSearchValuesMap);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* This method can receive rows-on-top as parameter on
* baseSearchValueMap
using {@link #ROWS_ON_TOP_IDS_PARAM}
* name.
*
* @param entityClass entity to use in search
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param baseSearchValuesMap (optional) base filter values
* @param distinct use distinct query
* @return
*/
public > SearchResults findByCriteria(
Class entityClass,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
Map baseSearchValuesMap, boolean distinct);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entityClass entity to use in search
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @param distinct use distinct query
* @return
*/
public > SearchResults findByCriteria(
Class entityClass,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate, boolean distinct);
/**
* Execute a select query on entityClass using {@code DatatablesCriterias}
* information for filter, sort and paginate result.
*
* This method can receive rows-on-top as parameter on
* baseSearchValueMap
using {@link #ROWS_ON_TOP_IDS_PARAM}
* name.
*
* @param entityClass entity to use in search
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter
* @param distinct use distinct query
* @param rowsOnTopIds (optional) array with id of rows to show on top of
* result list
* @return
* @throws IllegalArgumentException
*/
public > SearchResults findByCriteria(
Class entityClass,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate, boolean distinct,
Object[] rowsOnTopIds);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entity builder for entity to use in search. Represents the entity
* and gives access to its properties for query purposes
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @return
*/
public > SearchResults findByCriteria(
PathBuilder entity,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entity builder for entity to use in search. Represents the entity
* and gives access to its properties for query purposes
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @return
*/
public > SearchResults findByCriteria(
PathBuilder entity, DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entity builder for entity to use in search. Represents the entity
* and gives access to its properties for query purposes
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @param rowsOnTopIds (optional) array with id of rows to show on top of
* result list
* @return
* @throws IllegalArgumentException
*/
public > SearchResults findByCriteria(
PathBuilder entity, DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate, Object[] rowsOnTopIds);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entity builder for entity to use in search. Represents the entity
* and gives access to its properties for query purposes
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @param distinct use distinct query
* @return
*/
public > SearchResults findByCriteria(
PathBuilder entity,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate, boolean distinct);
/**
* Execute a select query on entityClass using Querydsl which enables the
* construction of type-safe SQL-like queries.
*
* @param entity builder for entity to use in search. Represents the entity
* and gives access to its properties for query purposes
* @param filterByAssociations (optional) for each related entity to join
* contain as key the name of the association and as value the List
* of related entity fields to filter by
* @param orderByAssociations (optional) for each related entity to order
* contain as key the name of the association and as value the List
* of related entity fields to order by
* @param datatablesCriterias datatables parameters for query
* @param basePredicate (optional) base filter conditions
* @param distinct use distinct query
* @param rowsOnTopIds (optional) array with id of rows to show on top of
* result list
* @return
* @throws IllegalArgumentException
*/
public > SearchResults findByCriteria(
PathBuilder entity,
Map> filterByAssociations,
Map> orderByAssociations,
DatatablesCriterias datatablesCriterias,
BooleanBuilder basePredicate, boolean distinct,
Object[] rowsOnTopIds);
/**
* Prepares associationMap for findByCriteria
*
* @param entity
* @param filterByAssociations
* @param datatablesCriterias
* @param findInAllColumns
* @param query
* @param associationMap
* @return
*/
public JPAQuery prepareQueryAssociationMap(PathBuilder entity,
Map> filterByAssociations,
DatatablesCriterias datatablesCriterias, boolean findInAllColumns,
JPAQuery query, Map> associationMap);
/**
* Populate a {@link DataSet} from given entity list.
*
* Field values will be converted to String using given
* {@link ConversionService} and Date fields will be converted to Date using
* {@link DateFormat} with given date patterns.
*
* @param entities List of T entities to convert to Datatables data
* @param pkFieldName The T entity field that contains the PK
* @param totalRecords Total amount of records
* @param totalDisplayRecords Amount of records found
* @param columns {@link ColumnDef} list
* @param datePatterns Patterns to convert Date fields to String. The Map
* contains one pattern for each entity Date field keyed by field
* name. For Roo compatibility the key could follow the pattern
* {@code uncapitalize( ENTITY ) + "_" + lower_case( FIELD ) + "_date_format"}
* too
* @return
*/
public DataSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy