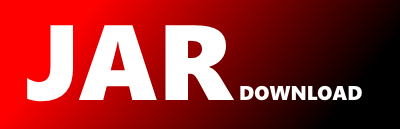
org.gwizard.logging.LogCallInterceptor Maven / Gradle / Ivy
package org.gwizard.logging;
import lombok.extern.slf4j.Slf4j;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import org.slf4j.Logger;
/**
* Guice AOP interceptor which looks for {@code @LogCall} annotation on methods to log method calls.
*
* Put this in your Guice module configure():
* {@code bindInterceptor(Matchers.any(), Matchers.annotatedWith(LogCall.class), new LoggingInterceptor());}
*
* @author Jeff Schnitzer
*/
@Slf4j
public class LogCallInterceptor implements MethodInterceptor
{
/* (non-Javadoc)
* @see org.aopalliance.intercept.MethodInterceptor#invoke(org.aopalliance.intercept.MethodInvocation)
*/
@Override
public Object invoke(MethodInvocation inv) throws Throwable
{
final LogCall logCallAnnotation = inv.getMethod().getAnnotation(LogCall.class);
final Object obj = inv.getThis();
// The actual class will be the guice enhanced one, so get super
final Class> clazz = obj.getClass().getSuperclass();
// Turns out GAE always overrides logger value, so no point in doing the lookup
//final Logger logger = LoggerFactory.getLogger(clazz);
final Logger logger = log;
final StringBuilder msg = new StringBuilder();
msg.append(clazz.getSimpleName());
msg.append('.');
msg.append(inv.getMethod().getName());
msg.append('(');
for (int i=0; i