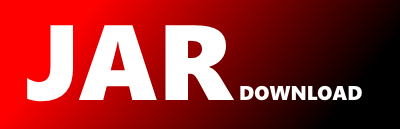
org.gwtbootstrap3.extras.select.client.ui.OptGroup Maven / Gradle / Ivy
package org.gwtbootstrap3.extras.select.client.ui;
/*
* #%L
* GwtBootstrap3
* %%
* Copyright (C) 2013 - 2016 GwtBootstrap3
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import static org.gwtbootstrap3.extras.select.client.ui.SelectOptions.ICON;
import static org.gwtbootstrap3.extras.select.client.ui.SelectOptions.MAX_OPTIONS;
import static org.gwtbootstrap3.extras.select.client.ui.SelectOptions.SUBTEXT;
import java.util.HashMap;
import java.util.Map;
import org.gwtbootstrap3.client.ui.base.ComplexWidget;
import org.gwtbootstrap3.client.ui.base.mixin.AttributeMixin;
import org.gwtbootstrap3.client.ui.base.mixin.EnabledMixin;
import org.gwtbootstrap3.client.ui.constants.IconType;
import com.google.gwt.dom.client.Document;
import com.google.gwt.dom.client.OptionElement;
import com.google.gwt.user.client.ui.HasEnabled;
import com.google.gwt.user.client.ui.Widget;
/**
* Select option group widget.
*
* @author Xiaodong Sun
*/
public class OptGroup extends ComplexWidget implements HasEnabled {
private final Map itemMap = new HashMap<>(0);
private final AttributeMixin
© 2015 - 2025 Weber Informatics LLC | Privacy Policy