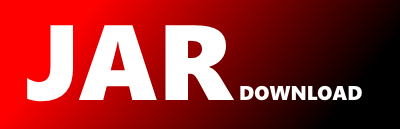
org.gwtopenmaps.openlayers.client.StyleMap Maven / Gradle / Ivy
package org.gwtopenmaps.openlayers.client;
import org.gwtopenmaps.openlayers.client.feature.VectorFeature;
import org.gwtopenmaps.openlayers.client.util.JSObject;
/**
*
* StyleMap contais a set of tree styles
*
* - default : the default style to render the feature
* - select : the style to render the feature when it is selected
* - temporary: style to render the feature when it is temporarily selected
*
*
* @author Rafael Ceravolo - LOGANN
* @author Lukas Johansson
*/
public class StyleMap extends OpenLayersObjectWrapper {
protected StyleMap(JSObject openLayersObject) {
super(openLayersObject);
}
/**
* Creates a StyleMap with default properties
*/
public StyleMap() {
this(StyleMapImpl.create());
}
/**
* Creates a StyleMap setting the same style for all renderer intents.
*
* From OpenLayers documentation: If just one style hash or style object is
* passed, this will be used for all known render intents (default, select,
* temporary)
*/
public StyleMap(Style style) {
this(
StyleMapImpl.create(
style.getJSObject(),
style.getJSObject(),
style.getJSObject()
)
);
}
/**
* Creates a StyleMap setting different styles for renderer intents.
*
* Avoid passing null for one of the styles. If just want to use 2 style,
* pass the same style for selectStyle and tempraryStyle parameters.
*
* @param defaultStyle
* the default style to render the feature
* @param selectStyle
* the style to render the feature when it is selected
* @param temporaryStyle
* style to render the feature when it is temporarily selected
*/
public StyleMap(Style defaultStyle, Style selectStyle, Style temporaryStyle) {
this(
StyleMapImpl.create(
defaultStyle == null ? null : defaultStyle.getJSObject(),
selectStyle == null ? null : selectStyle.getJSObject(),
temporaryStyle == null ? null : temporaryStyle.getJSObject()
)
);
}
public static StyleMap narrowToOpenLayersStyleMap(JSObject element) {
return (element == null) ? null: new StyleMap(element);
}
public Style createSymbolizer(VectorFeature feature, String renderIntent){
return Style.narrowToOpenLayersStyle(
StyleImpl.createSymbolizer(
getJSObject(),
feature.getJSObject(),
renderIntent
)
);
}
}