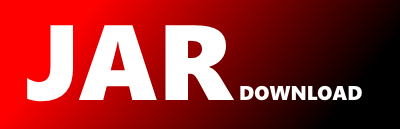
org.hamcrest.CoreMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hamcrest-core Show documentation
Show all versions of hamcrest-core Show documentation
This is the core API of hamcrest matcher framework
to be used by third-party framework providers.
This includes the a foundation set of matcher
implementations for common operations.
// Generated source.
package org.hamcrest;
public class CoreMatchers {
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T>... param1) {
return org.hamcrest.core.AllOf.allOf(param1);
}
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T> p0, org.hamcrest.Matcher super T> p1) {
return org.hamcrest.core.AllOf.allOf(p0, p1);
}
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T> p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2) {
return org.hamcrest.core.AllOf.allOf(p0, p1, p2);
}
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T> p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3) {
return org.hamcrest.core.AllOf.allOf(p0, p1, p2, p3);
}
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T> p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3, org.hamcrest.Matcher super T> p4) {
return org.hamcrest.core.AllOf.allOf(p0, p1, p2, p3, p4);
}
public static org.hamcrest.Matcher allOf(org.hamcrest.Matcher super T> p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3, org.hamcrest.Matcher super T> p4, org.hamcrest.Matcher super T> p5) {
return org.hamcrest.core.AllOf.allOf(p0, p1, p2, p3, p4, p5);
}
public static org.hamcrest.Matcher allOf(java.lang.Iterable> p0) {
return org.hamcrest.core.AllOf.allOf(p0);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3) {
return org.hamcrest.core.AnyOf.anyOf(p0, p1, p2, p3);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher p0, org.hamcrest.Matcher super T> p1) {
return org.hamcrest.core.AnyOf.anyOf(p0, p1);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2) {
return org.hamcrest.core.AnyOf.anyOf(p0, p1, p2);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher super T>... param1) {
return org.hamcrest.core.AnyOf.anyOf(param1);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3, org.hamcrest.Matcher super T> p4) {
return org.hamcrest.core.AnyOf.anyOf(p0, p1, p2, p3, p4);
}
public static org.hamcrest.core.AnyOf anyOf(org.hamcrest.Matcher p0, org.hamcrest.Matcher super T> p1, org.hamcrest.Matcher super T> p2, org.hamcrest.Matcher super T> p3, org.hamcrest.Matcher super T> p4, org.hamcrest.Matcher super T> p5) {
return org.hamcrest.core.AnyOf.anyOf(p0, p1, p2, p3, p4, p5);
}
public static org.hamcrest.core.AnyOf anyOf(java.lang.Iterable> p0) {
return org.hamcrest.core.AnyOf.anyOf(p0);
}
public static org.hamcrest.core.CombinableMatcher both(org.hamcrest.Matcher super LHS> p0) {
return org.hamcrest.core.CombinableMatcher.both(p0);
}
public static org.hamcrest.core.CombinableMatcher either(org.hamcrest.Matcher super LHS> p0) {
return org.hamcrest.core.CombinableMatcher.either(p0);
}
public static org.hamcrest.Matcher describedAs(java.lang.String param1, org.hamcrest.Matcher param2, java.lang.Object... param3) {
return org.hamcrest.core.DescribedAs.describedAs(param1, param2, param3);
}
public static org.hamcrest.Matcher> everyItem(org.hamcrest.Matcher p0) {
return org.hamcrest.core.Every.everyItem(p0);
}
public static org.hamcrest.Matcher is(org.hamcrest.Matcher p0) {
return org.hamcrest.core.Is.is(p0);
}
public static org.hamcrest.Matcher is(T param1) {
return org.hamcrest.core.Is.is(param1);
}
public static org.hamcrest.Matcher is(java.lang.Class p0) {
return org.hamcrest.core.Is.is(p0);
}
public static org.hamcrest.Matcher isA(java.lang.Class p0) {
return org.hamcrest.core.Is.isA(p0);
}
public static org.hamcrest.Matcher anything() {
return org.hamcrest.core.IsAnything.anything();
}
public static org.hamcrest.Matcher anything(java.lang.String p0) {
return org.hamcrest.core.IsAnything.anything(p0);
}
public static org.hamcrest.Matcher> hasItem(T param1) {
return org.hamcrest.core.IsCollectionContaining.hasItem(param1);
}
public static org.hamcrest.Matcher> hasItem(org.hamcrest.Matcher super T> p0) {
return org.hamcrest.core.IsCollectionContaining.hasItem(p0);
}
public static org.hamcrest.Matcher> hasItems(org.hamcrest.Matcher super T>... param1) {
return org.hamcrest.core.IsCollectionContaining.hasItems(param1);
}
public static org.hamcrest.Matcher> hasItems(T... param1) {
return org.hamcrest.core.IsCollectionContaining.hasItems(param1);
}
public static org.hamcrest.Matcher equalTo(T param1) {
return org.hamcrest.core.IsEqual.equalTo(param1);
}
public static org.hamcrest.Matcher any(java.lang.Class p0) {
return org.hamcrest.core.IsInstanceOf.any(p0);
}
public static org.hamcrest.Matcher instanceOf(java.lang.Class> p0) {
return org.hamcrest.core.IsInstanceOf.instanceOf(p0);
}
public static org.hamcrest.Matcher not(org.hamcrest.Matcher p0) {
return org.hamcrest.core.IsNot.not(p0);
}
public static org.hamcrest.Matcher not(T param1) {
return org.hamcrest.core.IsNot.not(param1);
}
public static org.hamcrest.Matcher nullValue() {
return org.hamcrest.core.IsNull.nullValue();
}
public static org.hamcrest.Matcher nullValue(java.lang.Class p0) {
return org.hamcrest.core.IsNull.nullValue(p0);
}
public static org.hamcrest.Matcher notNullValue() {
return org.hamcrest.core.IsNull.notNullValue();
}
public static org.hamcrest.Matcher notNullValue(java.lang.Class p0) {
return org.hamcrest.core.IsNull.notNullValue(p0);
}
public static org.hamcrest.Matcher sameInstance(T param1) {
return org.hamcrest.core.IsSame.sameInstance(param1);
}
public static org.hamcrest.Matcher containsString(java.lang.String p0) {
return org.hamcrest.core.StringContains.containsString(p0);
}
public static org.hamcrest.Matcher startsWith(java.lang.String p0) {
return org.hamcrest.core.StringStartsWith.startsWith(p0);
}
public static org.hamcrest.Matcher endsWith(java.lang.String p0) {
return org.hamcrest.core.StringEndsWith.endsWith(p0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy