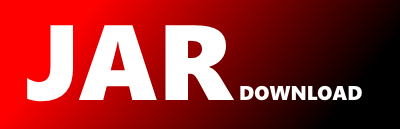
org.hamcrest.collection.IsArrayContainingInOrder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hamcrest Show documentation
Show all versions of hamcrest Show documentation
Core API and libraries of hamcrest matcher framework.
package org.hamcrest.collection;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import static java.util.Arrays.asList;
import static org.hamcrest.core.IsEqual.equalTo;
/**
* @deprecated As of release 2.1, replaced by {@link ArrayMatching}.
*/
public class IsArrayContainingInOrder extends TypeSafeMatcher {
private final Collection> matchers;
private final IsIterableContainingInOrder iterableMatcher;
public IsArrayContainingInOrder(List> matchers) {
this.iterableMatcher = new IsIterableContainingInOrder(matchers);
this.matchers = matchers;
}
@Override
public boolean matchesSafely(E[] item) {
return iterableMatcher.matches(asList(item));
}
@Override
public void describeMismatchSafely(E[] item, Description mismatchDescription) {
iterableMatcher.describeMismatch(asList(item), mismatchDescription);
}
@Override
public void describeTo(Description description) {
description.appendList("[", ", ", "]", matchers);
}
/**
* Creates a matcher for arrays that matcheswhen each item in the examined array is
* logically equal to the corresponding item in the specified items. For a positive match,
* the examined array must be of the same length as the number of specified items.
*
* For example:
*
assertThat(new String[]{"foo", "bar"}, contains("foo", "bar"))
*
* @deprecated As of version 2.1, use {@link ArrayMatching#arrayContaining(Object[])}.
*
* @param items
* the items that must equal the items within an examined array
*/
public static Matcher arrayContaining(E... items) {
List> matchers = new ArrayList>();
for (E item : items) {
matchers.add(equalTo(item));
}
return arrayContaining(matchers);
}
/**
* Creates a matcher for arrays that matches when each item in the examined array satisfies the
* corresponding matcher in the specified matchers. For a positive match, the examined array
* must be of the same length as the number of specified matchers.
*
* For example:
*
assertThat(new String[]{"foo", "bar"}, contains(equalTo("foo"), equalTo("bar")))
*
* @deprecated As of version 2.1, use {@link ArrayMatching#arrayContaining(Matcher[])}.
*
* @param itemMatchers
* the matchers that must be satisfied by the items in the examined array
*/
public static Matcher arrayContaining(Matcher super E>... itemMatchers) {
return arrayContaining(asList(itemMatchers));
}
/**
* Creates a matcher for arrays that matches when each item in the examined array satisfies the
* corresponding matcher in the specified list of matchers. For a positive match, the examined array
* must be of the same length as the specified list of matchers.
*
* For example:
*
assertThat(new String[]{"foo", "bar"}, contains(Arrays.asList(equalTo("foo"), equalTo("bar"))))
*
* @deprecated As of version 2.1, use {@link ArrayMatching#arrayContaining(List)}.
*
* @param itemMatchers
* a list of matchers, each of which must be satisfied by the corresponding item in an examined array
*/
public static Matcher arrayContaining(List> itemMatchers) {
return new IsArrayContainingInOrder(itemMatchers);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy