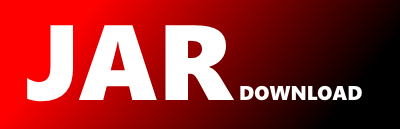
org.hbase.async.generated.ClientPB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of asynchbase Show documentation
Show all versions of asynchbase Show documentation
An alternative HBase client library for applications requiring fully
asynchronous, non-blocking and thread-safe HBase connectivity.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Client.proto
package org.hbase.async.generated;
public final class ClientPB {
private ClientPB() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
/**
* Protobuf enum {@code Consistency}
*
*
**
* Consistency defines the expected consistency level for an operation.
*
*/
public enum Consistency
implements com.google.protobuf.Internal.EnumLite {
/**
* STRONG = 0;
*/
STRONG(0, 0),
/**
* TIMELINE = 1;
*/
TIMELINE(1, 1),
;
/**
* STRONG = 0;
*/
public static final int STRONG_VALUE = 0;
/**
* TIMELINE = 1;
*/
public static final int TIMELINE_VALUE = 1;
public final int getNumber() { return value; }
public static Consistency valueOf(int value) {
switch (value) {
case 0: return STRONG;
case 1: return TIMELINE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Consistency findValueByNumber(int number) {
return Consistency.valueOf(number);
}
};
private final int value;
private Consistency(int index, int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:Consistency)
}
public interface AuthorizationsOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated string label = 1;
/**
* repeated string label = 1;
*/
java.util.List
getLabelList();
/**
* repeated string label = 1;
*/
int getLabelCount();
/**
* repeated string label = 1;
*/
java.lang.String getLabel(int index);
/**
* repeated string label = 1;
*/
com.google.protobuf.ByteString
getLabelBytes(int index);
}
/**
* Protobuf type {@code Authorizations}
*
*
**
* The protocol buffer version of Authorizations.
*
*/
public static final class Authorizations extends
com.google.protobuf.GeneratedMessageLite
implements AuthorizationsOrBuilder {
// Use Authorizations.newBuilder() to construct.
private Authorizations(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Authorizations(boolean noInit) {}
private static final Authorizations defaultInstance;
public static Authorizations getDefaultInstance() {
return defaultInstance;
}
public Authorizations getDefaultInstanceForType() {
return defaultInstance;
}
private Authorizations(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
label_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
label_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
label_ = new com.google.protobuf.UnmodifiableLazyStringList(label_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Authorizations parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Authorizations(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated string label = 1;
public static final int LABEL_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList label_;
/**
* repeated string label = 1;
*/
public java.util.List
getLabelList() {
return label_;
}
/**
* repeated string label = 1;
*/
public int getLabelCount() {
return label_.size();
}
/**
* repeated string label = 1;
*/
public java.lang.String getLabel(int index) {
return label_.get(index);
}
/**
* repeated string label = 1;
*/
public com.google.protobuf.ByteString
getLabelBytes(int index) {
return label_.getByteString(index);
}
private void initFields() {
label_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < label_.size(); i++) {
output.writeBytes(1, label_.getByteString(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < label_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(label_.getByteString(i));
}
size += dataSize;
size += 1 * getLabelList().size();
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Authorizations parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Authorizations prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Authorizations}
*
*
**
* The protocol buffer version of Authorizations.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Authorizations, Builder>
implements org.hbase.async.generated.ClientPB.AuthorizationsOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Authorizations.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
label_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Authorizations getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Authorizations.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Authorizations build() {
org.hbase.async.generated.ClientPB.Authorizations result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Authorizations buildPartial() {
org.hbase.async.generated.ClientPB.Authorizations result = new org.hbase.async.generated.ClientPB.Authorizations(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
label_ = new com.google.protobuf.UnmodifiableLazyStringList(
label_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.label_ = label_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Authorizations other) {
if (other == org.hbase.async.generated.ClientPB.Authorizations.getDefaultInstance()) return this;
if (!other.label_.isEmpty()) {
if (label_.isEmpty()) {
label_ = other.label_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLabelIsMutable();
label_.addAll(other.label_);
}
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Authorizations parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Authorizations) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated string label = 1;
private com.google.protobuf.LazyStringList label_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureLabelIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
label_ = new com.google.protobuf.LazyStringArrayList(label_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string label = 1;
*/
public java.util.List
getLabelList() {
return java.util.Collections.unmodifiableList(label_);
}
/**
* repeated string label = 1;
*/
public int getLabelCount() {
return label_.size();
}
/**
* repeated string label = 1;
*/
public java.lang.String getLabel(int index) {
return label_.get(index);
}
/**
* repeated string label = 1;
*/
public com.google.protobuf.ByteString
getLabelBytes(int index) {
return label_.getByteString(index);
}
/**
* repeated string label = 1;
*/
public Builder setLabel(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLabelIsMutable();
label_.set(index, value);
return this;
}
/**
* repeated string label = 1;
*/
public Builder addLabel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLabelIsMutable();
label_.add(value);
return this;
}
/**
* repeated string label = 1;
*/
public Builder addAllLabel(
java.lang.Iterable values) {
ensureLabelIsMutable();
super.addAll(values, label_);
return this;
}
/**
* repeated string label = 1;
*/
public Builder clearLabel() {
label_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated string label = 1;
*/
public Builder addLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureLabelIsMutable();
label_.add(value);
return this;
}
// @@protoc_insertion_point(builder_scope:Authorizations)
}
static {
defaultInstance = new Authorizations(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Authorizations)
}
public interface CellVisibilityOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required string expression = 1;
/**
* required string expression = 1;
*/
boolean hasExpression();
/**
* required string expression = 1;
*/
java.lang.String getExpression();
/**
* required string expression = 1;
*/
com.google.protobuf.ByteString
getExpressionBytes();
}
/**
* Protobuf type {@code CellVisibility}
*
*
**
* The protocol buffer version of CellVisibility.
*
*/
public static final class CellVisibility extends
com.google.protobuf.GeneratedMessageLite
implements CellVisibilityOrBuilder {
// Use CellVisibility.newBuilder() to construct.
private CellVisibility(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private CellVisibility(boolean noInit) {}
private static final CellVisibility defaultInstance;
public static CellVisibility getDefaultInstance() {
return defaultInstance;
}
public CellVisibility getDefaultInstanceForType() {
return defaultInstance;
}
private CellVisibility(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
expression_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CellVisibility parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CellVisibility(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string expression = 1;
public static final int EXPRESSION_FIELD_NUMBER = 1;
private java.lang.Object expression_;
/**
* required string expression = 1;
*/
public boolean hasExpression() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string expression = 1;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
expression_ = s;
}
return s;
}
}
/**
* required string expression = 1;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
expression_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasExpression()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getExpressionBytes());
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getExpressionBytes());
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CellVisibility parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.CellVisibility prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code CellVisibility}
*
*
**
* The protocol buffer version of CellVisibility.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.CellVisibility, Builder>
implements org.hbase.async.generated.ClientPB.CellVisibilityOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.CellVisibility.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
expression_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.CellVisibility getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.CellVisibility.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.CellVisibility build() {
org.hbase.async.generated.ClientPB.CellVisibility result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.CellVisibility buildPartial() {
org.hbase.async.generated.ClientPB.CellVisibility result = new org.hbase.async.generated.ClientPB.CellVisibility(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.expression_ = expression_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.CellVisibility other) {
if (other == org.hbase.async.generated.ClientPB.CellVisibility.getDefaultInstance()) return this;
if (other.hasExpression()) {
bitField0_ |= 0x00000001;
expression_ = other.expression_;
}
return this;
}
public final boolean isInitialized() {
if (!hasExpression()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.CellVisibility parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.CellVisibility) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string expression = 1;
private java.lang.Object expression_ = "";
/**
* required string expression = 1;
*/
public boolean hasExpression() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string expression = 1;
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
expression_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string expression = 1;
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string expression = 1;
*/
public Builder setExpression(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
expression_ = value;
return this;
}
/**
* required string expression = 1;
*/
public Builder clearExpression() {
bitField0_ = (bitField0_ & ~0x00000001);
expression_ = getDefaultInstance().getExpression();
return this;
}
/**
* required string expression = 1;
*/
public Builder setExpressionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
expression_ = value;
return this;
}
// @@protoc_insertion_point(builder_scope:CellVisibility)
}
static {
defaultInstance = new CellVisibility(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CellVisibility)
}
public interface ColumnOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes family = 1;
/**
* required bytes family = 1;
*/
boolean hasFamily();
/**
* required bytes family = 1;
*/
com.google.protobuf.ByteString getFamily();
// repeated bytes qualifier = 2;
/**
* repeated bytes qualifier = 2;
*/
java.util.List getQualifierList();
/**
* repeated bytes qualifier = 2;
*/
int getQualifierCount();
/**
* repeated bytes qualifier = 2;
*/
com.google.protobuf.ByteString getQualifier(int index);
}
/**
* Protobuf type {@code Column}
*
*
**
* Container for a list of column qualifier names of a family.
*
*/
public static final class Column extends
com.google.protobuf.GeneratedMessageLite
implements ColumnOrBuilder {
// Use Column.newBuilder() to construct.
private Column(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Column(boolean noInit) {}
private static final Column defaultInstance;
public static Column getDefaultInstance() {
return defaultInstance;
}
public Column getDefaultInstanceForType() {
return defaultInstance;
}
private Column(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
family_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
qualifier_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
qualifier_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
qualifier_ = java.util.Collections.unmodifiableList(qualifier_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Column parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Column(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes family = 1;
public static final int FAMILY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString family_;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
// repeated bytes qualifier = 2;
public static final int QUALIFIER_FIELD_NUMBER = 2;
private java.util.List qualifier_;
/**
* repeated bytes qualifier = 2;
*/
public java.util.List
getQualifierList() {
return qualifier_;
}
/**
* repeated bytes qualifier = 2;
*/
public int getQualifierCount() {
return qualifier_.size();
}
/**
* repeated bytes qualifier = 2;
*/
public com.google.protobuf.ByteString getQualifier(int index) {
return qualifier_.get(index);
}
private void initFields() {
family_ = com.google.protobuf.ByteString.EMPTY;
qualifier_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasFamily()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, family_);
}
for (int i = 0; i < qualifier_.size(); i++) {
output.writeBytes(2, qualifier_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, family_);
}
{
int dataSize = 0;
for (int i = 0; i < qualifier_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(qualifier_.get(i));
}
size += dataSize;
size += 1 * getQualifierList().size();
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Column parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Column parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Column parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Column prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Column}
*
*
**
* Container for a list of column qualifier names of a family.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Column, Builder>
implements org.hbase.async.generated.ClientPB.ColumnOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Column.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
family_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
qualifier_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Column getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Column.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Column build() {
org.hbase.async.generated.ClientPB.Column result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Column buildPartial() {
org.hbase.async.generated.ClientPB.Column result = new org.hbase.async.generated.ClientPB.Column(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.family_ = family_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
qualifier_ = java.util.Collections.unmodifiableList(qualifier_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.qualifier_ = qualifier_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Column other) {
if (other == org.hbase.async.generated.ClientPB.Column.getDefaultInstance()) return this;
if (other.hasFamily()) {
setFamily(other.getFamily());
}
if (!other.qualifier_.isEmpty()) {
if (qualifier_.isEmpty()) {
qualifier_ = other.qualifier_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureQualifierIsMutable();
qualifier_.addAll(other.qualifier_);
}
}
return this;
}
public final boolean isInitialized() {
if (!hasFamily()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Column parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Column) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes family = 1;
private com.google.protobuf.ByteString family_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
/**
* required bytes family = 1;
*/
public Builder setFamily(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
family_ = value;
return this;
}
/**
* required bytes family = 1;
*/
public Builder clearFamily() {
bitField0_ = (bitField0_ & ~0x00000001);
family_ = getDefaultInstance().getFamily();
return this;
}
// repeated bytes qualifier = 2;
private java.util.List qualifier_ = java.util.Collections.emptyList();
private void ensureQualifierIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
qualifier_ = new java.util.ArrayList(qualifier_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated bytes qualifier = 2;
*/
public java.util.List
getQualifierList() {
return java.util.Collections.unmodifiableList(qualifier_);
}
/**
* repeated bytes qualifier = 2;
*/
public int getQualifierCount() {
return qualifier_.size();
}
/**
* repeated bytes qualifier = 2;
*/
public com.google.protobuf.ByteString getQualifier(int index) {
return qualifier_.get(index);
}
/**
* repeated bytes qualifier = 2;
*/
public Builder setQualifier(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureQualifierIsMutable();
qualifier_.set(index, value);
return this;
}
/**
* repeated bytes qualifier = 2;
*/
public Builder addQualifier(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureQualifierIsMutable();
qualifier_.add(value);
return this;
}
/**
* repeated bytes qualifier = 2;
*/
public Builder addAllQualifier(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureQualifierIsMutable();
super.addAll(values, qualifier_);
return this;
}
/**
* repeated bytes qualifier = 2;
*/
public Builder clearQualifier() {
qualifier_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// @@protoc_insertion_point(builder_scope:Column)
}
static {
defaultInstance = new Column(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Column)
}
public interface GetOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes row = 1;
/**
* required bytes row = 1;
*/
boolean hasRow();
/**
* required bytes row = 1;
*/
com.google.protobuf.ByteString getRow();
// repeated .Column column = 2;
/**
* repeated .Column column = 2;
*/
java.util.List
getColumnList();
/**
* repeated .Column column = 2;
*/
org.hbase.async.generated.ClientPB.Column getColumn(int index);
/**
* repeated .Column column = 2;
*/
int getColumnCount();
// repeated .NameBytesPair attribute = 3;
/**
* repeated .NameBytesPair attribute = 3;
*/
java.util.List
getAttributeList();
/**
* repeated .NameBytesPair attribute = 3;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index);
/**
* repeated .NameBytesPair attribute = 3;
*/
int getAttributeCount();
// optional .Filter filter = 4;
/**
* optional .Filter filter = 4;
*/
boolean hasFilter();
/**
* optional .Filter filter = 4;
*/
org.hbase.async.generated.FilterPB.Filter getFilter();
// optional .TimeRange time_range = 5;
/**
* optional .TimeRange time_range = 5;
*/
boolean hasTimeRange();
/**
* optional .TimeRange time_range = 5;
*/
org.hbase.async.generated.HBasePB.TimeRange getTimeRange();
// optional uint32 max_versions = 6 [default = 1];
/**
* optional uint32 max_versions = 6 [default = 1];
*/
boolean hasMaxVersions();
/**
* optional uint32 max_versions = 6 [default = 1];
*/
int getMaxVersions();
// optional bool cache_blocks = 7 [default = true];
/**
* optional bool cache_blocks = 7 [default = true];
*/
boolean hasCacheBlocks();
/**
* optional bool cache_blocks = 7 [default = true];
*/
boolean getCacheBlocks();
// optional uint32 store_limit = 8;
/**
* optional uint32 store_limit = 8;
*/
boolean hasStoreLimit();
/**
* optional uint32 store_limit = 8;
*/
int getStoreLimit();
// optional uint32 store_offset = 9;
/**
* optional uint32 store_offset = 9;
*/
boolean hasStoreOffset();
/**
* optional uint32 store_offset = 9;
*/
int getStoreOffset();
// optional bool existence_only = 10 [default = false];
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
boolean hasExistenceOnly();
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
boolean getExistenceOnly();
// optional bool closest_row_before = 11 [default = false];
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
boolean hasClosestRowBefore();
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
boolean getClosestRowBefore();
// optional .Consistency consistency = 12 [default = STRONG];
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
boolean hasConsistency();
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
org.hbase.async.generated.ClientPB.Consistency getConsistency();
// repeated .ColumnFamilyTimeRange cf_time_range = 13;
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
java.util.List
getCfTimeRangeList();
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index);
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
int getCfTimeRangeCount();
}
/**
* Protobuf type {@code Get}
*
*
**
* The protocol buffer version of Get.
* Unless existence_only is specified, return all the requested data
* for the row that matches exactly, or the one that immediately
* precedes it if closest_row_before is specified.
*
*/
public static final class Get extends
com.google.protobuf.GeneratedMessageLite
implements GetOrBuilder {
// Use Get.newBuilder() to construct.
private Get(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Get(boolean noInit) {}
private static final Get defaultInstance;
public static Get getDefaultInstance() {
return defaultInstance;
}
public Get getDefaultInstanceForType() {
return defaultInstance;
}
private Get(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
row_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
column_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
column_.add(input.readMessage(org.hbase.async.generated.ClientPB.Column.PARSER, extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
attribute_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
attribute_.add(input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry));
break;
}
case 34: {
org.hbase.async.generated.FilterPB.Filter.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = filter_.toBuilder();
}
filter_ = input.readMessage(org.hbase.async.generated.FilterPB.Filter.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(filter_);
filter_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 42: {
org.hbase.async.generated.HBasePB.TimeRange.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = timeRange_.toBuilder();
}
timeRange_ = input.readMessage(org.hbase.async.generated.HBasePB.TimeRange.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timeRange_);
timeRange_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 48: {
bitField0_ |= 0x00000008;
maxVersions_ = input.readUInt32();
break;
}
case 56: {
bitField0_ |= 0x00000010;
cacheBlocks_ = input.readBool();
break;
}
case 64: {
bitField0_ |= 0x00000020;
storeLimit_ = input.readUInt32();
break;
}
case 72: {
bitField0_ |= 0x00000040;
storeOffset_ = input.readUInt32();
break;
}
case 80: {
bitField0_ |= 0x00000080;
existenceOnly_ = input.readBool();
break;
}
case 88: {
bitField0_ |= 0x00000100;
closestRowBefore_ = input.readBool();
break;
}
case 96: {
int rawValue = input.readEnum();
org.hbase.async.generated.ClientPB.Consistency value = org.hbase.async.generated.ClientPB.Consistency.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00000200;
consistency_ = value;
}
break;
}
case 106: {
if (!((mutable_bitField0_ & 0x00001000) == 0x00001000)) {
cfTimeRange_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00001000;
}
cfTimeRange_.add(input.readMessage(org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
column_ = java.util.Collections.unmodifiableList(column_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
}
if (((mutable_bitField0_ & 0x00001000) == 0x00001000)) {
cfTimeRange_ = java.util.Collections.unmodifiableList(cfTimeRange_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Get parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Get(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes row = 1;
public static final int ROW_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString row_;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
// repeated .Column column = 2;
public static final int COLUMN_FIELD_NUMBER = 2;
private java.util.List column_;
/**
* repeated .Column column = 2;
*/
public java.util.List getColumnList() {
return column_;
}
/**
* repeated .Column column = 2;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.ColumnOrBuilder>
getColumnOrBuilderList() {
return column_;
}
/**
* repeated .Column column = 2;
*/
public int getColumnCount() {
return column_.size();
}
/**
* repeated .Column column = 2;
*/
public org.hbase.async.generated.ClientPB.Column getColumn(int index) {
return column_.get(index);
}
/**
* repeated .Column column = 2;
*/
public org.hbase.async.generated.ClientPB.ColumnOrBuilder getColumnOrBuilder(
int index) {
return column_.get(index);
}
// repeated .NameBytesPair attribute = 3;
public static final int ATTRIBUTE_FIELD_NUMBER = 3;
private java.util.List attribute_;
/**
* repeated .NameBytesPair attribute = 3;
*/
public java.util.List getAttributeList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder>
getAttributeOrBuilderList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder getAttributeOrBuilder(
int index) {
return attribute_.get(index);
}
// optional .Filter filter = 4;
public static final int FILTER_FIELD_NUMBER = 4;
private org.hbase.async.generated.FilterPB.Filter filter_;
/**
* optional .Filter filter = 4;
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Filter filter = 4;
*/
public org.hbase.async.generated.FilterPB.Filter getFilter() {
return filter_;
}
// optional .TimeRange time_range = 5;
public static final int TIME_RANGE_FIELD_NUMBER = 5;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_;
/**
* optional .TimeRange time_range = 5;
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .TimeRange time_range = 5;
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
// optional uint32 max_versions = 6 [default = 1];
public static final int MAX_VERSIONS_FIELD_NUMBER = 6;
private int maxVersions_;
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public boolean hasMaxVersions() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public int getMaxVersions() {
return maxVersions_;
}
// optional bool cache_blocks = 7 [default = true];
public static final int CACHE_BLOCKS_FIELD_NUMBER = 7;
private boolean cacheBlocks_;
/**
* optional bool cache_blocks = 7 [default = true];
*/
public boolean hasCacheBlocks() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool cache_blocks = 7 [default = true];
*/
public boolean getCacheBlocks() {
return cacheBlocks_;
}
// optional uint32 store_limit = 8;
public static final int STORE_LIMIT_FIELD_NUMBER = 8;
private int storeLimit_;
/**
* optional uint32 store_limit = 8;
*/
public boolean hasStoreLimit() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint32 store_limit = 8;
*/
public int getStoreLimit() {
return storeLimit_;
}
// optional uint32 store_offset = 9;
public static final int STORE_OFFSET_FIELD_NUMBER = 9;
private int storeOffset_;
/**
* optional uint32 store_offset = 9;
*/
public boolean hasStoreOffset() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint32 store_offset = 9;
*/
public int getStoreOffset() {
return storeOffset_;
}
// optional bool existence_only = 10 [default = false];
public static final int EXISTENCE_ONLY_FIELD_NUMBER = 10;
private boolean existenceOnly_;
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public boolean hasExistenceOnly() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public boolean getExistenceOnly() {
return existenceOnly_;
}
// optional bool closest_row_before = 11 [default = false];
public static final int CLOSEST_ROW_BEFORE_FIELD_NUMBER = 11;
private boolean closestRowBefore_;
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public boolean hasClosestRowBefore() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public boolean getClosestRowBefore() {
return closestRowBefore_;
}
// optional .Consistency consistency = 12 [default = STRONG];
public static final int CONSISTENCY_FIELD_NUMBER = 12;
private org.hbase.async.generated.ClientPB.Consistency consistency_;
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public org.hbase.async.generated.ClientPB.Consistency getConsistency() {
return consistency_;
}
// repeated .ColumnFamilyTimeRange cf_time_range = 13;
public static final int CF_TIME_RANGE_FIELD_NUMBER = 13;
private java.util.List cfTimeRange_;
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public java.util.List getCfTimeRangeList() {
return cfTimeRange_;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.ColumnFamilyTimeRangeOrBuilder>
getCfTimeRangeOrBuilderList() {
return cfTimeRange_;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public int getCfTimeRangeCount() {
return cfTimeRange_.size();
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index) {
return cfTimeRange_.get(index);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRangeOrBuilder getCfTimeRangeOrBuilder(
int index) {
return cfTimeRange_.get(index);
}
private void initFields() {
row_ = com.google.protobuf.ByteString.EMPTY;
column_ = java.util.Collections.emptyList();
attribute_ = java.util.Collections.emptyList();
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
maxVersions_ = 1;
cacheBlocks_ = true;
storeLimit_ = 0;
storeOffset_ = 0;
existenceOnly_ = false;
closestRowBefore_ = false;
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
cfTimeRange_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRow()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasFilter()) {
if (!getFilter().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getCfTimeRangeCount(); i++) {
if (!getCfTimeRange(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, row_);
}
for (int i = 0; i < column_.size(); i++) {
output.writeMessage(2, column_.get(i));
}
for (int i = 0; i < attribute_.size(); i++) {
output.writeMessage(3, attribute_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(4, filter_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(5, timeRange_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(6, maxVersions_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(7, cacheBlocks_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeUInt32(8, storeLimit_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeUInt32(9, storeOffset_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBool(10, existenceOnly_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(11, closestRowBefore_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeEnum(12, consistency_.getNumber());
}
for (int i = 0; i < cfTimeRange_.size(); i++) {
output.writeMessage(13, cfTimeRange_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, row_);
}
for (int i = 0; i < column_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, column_.get(i));
}
for (int i = 0; i < attribute_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, attribute_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, filter_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, timeRange_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, maxVersions_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, cacheBlocks_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(8, storeLimit_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(9, storeOffset_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, existenceOnly_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, closestRowBefore_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, consistency_.getNumber());
}
for (int i = 0; i < cfTimeRange_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, cfTimeRange_.get(i));
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Get parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Get parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Get parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Get prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Get}
*
*
**
* The protocol buffer version of Get.
* Unless existence_only is specified, return all the requested data
* for the row that matches exactly, or the one that immediately
* precedes it if closest_row_before is specified.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Get, Builder>
implements org.hbase.async.generated.ClientPB.GetOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Get.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
row_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
maxVersions_ = 1;
bitField0_ = (bitField0_ & ~0x00000020);
cacheBlocks_ = true;
bitField0_ = (bitField0_ & ~0x00000040);
storeLimit_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
storeOffset_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
existenceOnly_ = false;
bitField0_ = (bitField0_ & ~0x00000200);
closestRowBefore_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
bitField0_ = (bitField0_ & ~0x00000800);
cfTimeRange_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Get getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Get build() {
org.hbase.async.generated.ClientPB.Get result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Get buildPartial() {
org.hbase.async.generated.ClientPB.Get result = new org.hbase.async.generated.ClientPB.Get(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.row_ = row_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
column_ = java.util.Collections.unmodifiableList(column_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.column_ = column_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.attribute_ = attribute_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000002;
}
result.filter_ = filter_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000004;
}
result.timeRange_ = timeRange_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.maxVersions_ = maxVersions_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.cacheBlocks_ = cacheBlocks_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000020;
}
result.storeLimit_ = storeLimit_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
result.storeOffset_ = storeOffset_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
result.existenceOnly_ = existenceOnly_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
result.closestRowBefore_ = closestRowBefore_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
result.consistency_ = consistency_;
if (((bitField0_ & 0x00001000) == 0x00001000)) {
cfTimeRange_ = java.util.Collections.unmodifiableList(cfTimeRange_);
bitField0_ = (bitField0_ & ~0x00001000);
}
result.cfTimeRange_ = cfTimeRange_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Get other) {
if (other == org.hbase.async.generated.ClientPB.Get.getDefaultInstance()) return this;
if (other.hasRow()) {
setRow(other.getRow());
}
if (!other.column_.isEmpty()) {
if (column_.isEmpty()) {
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureColumnIsMutable();
column_.addAll(other.column_);
}
}
if (!other.attribute_.isEmpty()) {
if (attribute_.isEmpty()) {
attribute_ = other.attribute_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAttributeIsMutable();
attribute_.addAll(other.attribute_);
}
}
if (other.hasFilter()) {
mergeFilter(other.getFilter());
}
if (other.hasTimeRange()) {
mergeTimeRange(other.getTimeRange());
}
if (other.hasMaxVersions()) {
setMaxVersions(other.getMaxVersions());
}
if (other.hasCacheBlocks()) {
setCacheBlocks(other.getCacheBlocks());
}
if (other.hasStoreLimit()) {
setStoreLimit(other.getStoreLimit());
}
if (other.hasStoreOffset()) {
setStoreOffset(other.getStoreOffset());
}
if (other.hasExistenceOnly()) {
setExistenceOnly(other.getExistenceOnly());
}
if (other.hasClosestRowBefore()) {
setClosestRowBefore(other.getClosestRowBefore());
}
if (other.hasConsistency()) {
setConsistency(other.getConsistency());
}
if (!other.cfTimeRange_.isEmpty()) {
if (cfTimeRange_.isEmpty()) {
cfTimeRange_ = other.cfTimeRange_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureCfTimeRangeIsMutable();
cfTimeRange_.addAll(other.cfTimeRange_);
}
}
return this;
}
public final boolean isInitialized() {
if (!hasRow()) {
return false;
}
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
return false;
}
}
if (hasFilter()) {
if (!getFilter().isInitialized()) {
return false;
}
}
for (int i = 0; i < getCfTimeRangeCount(); i++) {
if (!getCfTimeRange(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Get parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Get) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes row = 1;
private com.google.protobuf.ByteString row_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
/**
* required bytes row = 1;
*/
public Builder setRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
row_ = value;
return this;
}
/**
* required bytes row = 1;
*/
public Builder clearRow() {
bitField0_ = (bitField0_ & ~0x00000001);
row_ = getDefaultInstance().getRow();
return this;
}
// repeated .Column column = 2;
private java.util.List column_ =
java.util.Collections.emptyList();
private void ensureColumnIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
column_ = new java.util.ArrayList(column_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated .Column column = 2;
*/
public java.util.List getColumnList() {
return java.util.Collections.unmodifiableList(column_);
}
/**
* repeated .Column column = 2;
*/
public int getColumnCount() {
return column_.size();
}
/**
* repeated .Column column = 2;
*/
public org.hbase.async.generated.ClientPB.Column getColumn(int index) {
return column_.get(index);
}
/**
* repeated .Column column = 2;
*/
public Builder setColumn(
int index, org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.set(index, value);
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder setColumn(
int index, org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.set(index, builderForValue.build());
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder addColumn(org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(value);
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder addColumn(
int index, org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(index, value);
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder addColumn(
org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.add(builderForValue.build());
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder addColumn(
int index, org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.add(index, builderForValue.build());
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder addAllColumn(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.Column> values) {
ensureColumnIsMutable();
super.addAll(values, column_);
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder clearColumn() {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* repeated .Column column = 2;
*/
public Builder removeColumn(int index) {
ensureColumnIsMutable();
column_.remove(index);
return this;
}
// repeated .NameBytesPair attribute = 3;
private java.util.List attribute_ =
java.util.Collections.emptyList();
private void ensureAttributeIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
attribute_ = new java.util.ArrayList(attribute_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public java.util.List getAttributeList() {
return java.util.Collections.unmodifiableList(attribute_);
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.set(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.set(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder addAttribute(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(value);
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder addAttribute(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder addAllAttribute(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.NameBytesPair> values) {
ensureAttributeIsMutable();
super.addAll(values, attribute_);
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder clearAttribute() {
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* repeated .NameBytesPair attribute = 3;
*/
public Builder removeAttribute(int index) {
ensureAttributeIsMutable();
attribute_.remove(index);
return this;
}
// optional .Filter filter = 4;
private org.hbase.async.generated.FilterPB.Filter filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
/**
* optional .Filter filter = 4;
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .Filter filter = 4;
*/
public org.hbase.async.generated.FilterPB.Filter getFilter() {
return filter_;
}
/**
* optional .Filter filter = 4;
*/
public Builder setFilter(org.hbase.async.generated.FilterPB.Filter value) {
if (value == null) {
throw new NullPointerException();
}
filter_ = value;
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .Filter filter = 4;
*/
public Builder setFilter(
org.hbase.async.generated.FilterPB.Filter.Builder builderForValue) {
filter_ = builderForValue.build();
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .Filter filter = 4;
*/
public Builder mergeFilter(org.hbase.async.generated.FilterPB.Filter value) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
filter_ != org.hbase.async.generated.FilterPB.Filter.getDefaultInstance()) {
filter_ =
org.hbase.async.generated.FilterPB.Filter.newBuilder(filter_).mergeFrom(value).buildPartial();
} else {
filter_ = value;
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .Filter filter = 4;
*/
public Builder clearFilter() {
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
// optional .TimeRange time_range = 5;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
/**
* optional .TimeRange time_range = 5;
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .TimeRange time_range = 5;
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
/**
* optional .TimeRange time_range = 5;
*/
public Builder setTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (value == null) {
throw new NullPointerException();
}
timeRange_ = value;
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .TimeRange time_range = 5;
*/
public Builder setTimeRange(
org.hbase.async.generated.HBasePB.TimeRange.Builder builderForValue) {
timeRange_ = builderForValue.build();
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .TimeRange time_range = 5;
*/
public Builder mergeTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
timeRange_ != org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance()) {
timeRange_ =
org.hbase.async.generated.HBasePB.TimeRange.newBuilder(timeRange_).mergeFrom(value).buildPartial();
} else {
timeRange_ = value;
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .TimeRange time_range = 5;
*/
public Builder clearTimeRange() {
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
// optional uint32 max_versions = 6 [default = 1];
private int maxVersions_ = 1;
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public boolean hasMaxVersions() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public int getMaxVersions() {
return maxVersions_;
}
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public Builder setMaxVersions(int value) {
bitField0_ |= 0x00000020;
maxVersions_ = value;
return this;
}
/**
* optional uint32 max_versions = 6 [default = 1];
*/
public Builder clearMaxVersions() {
bitField0_ = (bitField0_ & ~0x00000020);
maxVersions_ = 1;
return this;
}
// optional bool cache_blocks = 7 [default = true];
private boolean cacheBlocks_ = true;
/**
* optional bool cache_blocks = 7 [default = true];
*/
public boolean hasCacheBlocks() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool cache_blocks = 7 [default = true];
*/
public boolean getCacheBlocks() {
return cacheBlocks_;
}
/**
* optional bool cache_blocks = 7 [default = true];
*/
public Builder setCacheBlocks(boolean value) {
bitField0_ |= 0x00000040;
cacheBlocks_ = value;
return this;
}
/**
* optional bool cache_blocks = 7 [default = true];
*/
public Builder clearCacheBlocks() {
bitField0_ = (bitField0_ & ~0x00000040);
cacheBlocks_ = true;
return this;
}
// optional uint32 store_limit = 8;
private int storeLimit_ ;
/**
* optional uint32 store_limit = 8;
*/
public boolean hasStoreLimit() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 store_limit = 8;
*/
public int getStoreLimit() {
return storeLimit_;
}
/**
* optional uint32 store_limit = 8;
*/
public Builder setStoreLimit(int value) {
bitField0_ |= 0x00000080;
storeLimit_ = value;
return this;
}
/**
* optional uint32 store_limit = 8;
*/
public Builder clearStoreLimit() {
bitField0_ = (bitField0_ & ~0x00000080);
storeLimit_ = 0;
return this;
}
// optional uint32 store_offset = 9;
private int storeOffset_ ;
/**
* optional uint32 store_offset = 9;
*/
public boolean hasStoreOffset() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint32 store_offset = 9;
*/
public int getStoreOffset() {
return storeOffset_;
}
/**
* optional uint32 store_offset = 9;
*/
public Builder setStoreOffset(int value) {
bitField0_ |= 0x00000100;
storeOffset_ = value;
return this;
}
/**
* optional uint32 store_offset = 9;
*/
public Builder clearStoreOffset() {
bitField0_ = (bitField0_ & ~0x00000100);
storeOffset_ = 0;
return this;
}
// optional bool existence_only = 10 [default = false];
private boolean existenceOnly_ ;
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public boolean hasExistenceOnly() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public boolean getExistenceOnly() {
return existenceOnly_;
}
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public Builder setExistenceOnly(boolean value) {
bitField0_ |= 0x00000200;
existenceOnly_ = value;
return this;
}
/**
* optional bool existence_only = 10 [default = false];
*
*
* The result isn't asked for, just check for
* the existence.
*
*/
public Builder clearExistenceOnly() {
bitField0_ = (bitField0_ & ~0x00000200);
existenceOnly_ = false;
return this;
}
// optional bool closest_row_before = 11 [default = false];
private boolean closestRowBefore_ ;
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public boolean hasClosestRowBefore() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public boolean getClosestRowBefore() {
return closestRowBefore_;
}
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public Builder setClosestRowBefore(boolean value) {
bitField0_ |= 0x00000400;
closestRowBefore_ = value;
return this;
}
/**
* optional bool closest_row_before = 11 [default = false];
*
*
* If the row to get doesn't exist, return the
* closest row before.
*
*/
public Builder clearClosestRowBefore() {
bitField0_ = (bitField0_ & ~0x00000400);
closestRowBefore_ = false;
return this;
}
// optional .Consistency consistency = 12 [default = STRONG];
private org.hbase.async.generated.ClientPB.Consistency consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public org.hbase.async.generated.ClientPB.Consistency getConsistency() {
return consistency_;
}
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public Builder setConsistency(org.hbase.async.generated.ClientPB.Consistency value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
consistency_ = value;
return this;
}
/**
* optional .Consistency consistency = 12 [default = STRONG];
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000800);
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
return this;
}
// repeated .ColumnFamilyTimeRange cf_time_range = 13;
private java.util.List cfTimeRange_ =
java.util.Collections.emptyList();
private void ensureCfTimeRangeIsMutable() {
if (!((bitField0_ & 0x00001000) == 0x00001000)) {
cfTimeRange_ = new java.util.ArrayList(cfTimeRange_);
bitField0_ |= 0x00001000;
}
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public java.util.List getCfTimeRangeList() {
return java.util.Collections.unmodifiableList(cfTimeRange_);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public int getCfTimeRangeCount() {
return cfTimeRange_.size();
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index) {
return cfTimeRange_.get(index);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder setCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.set(index, value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder setCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.set(index, builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder addCfTimeRange(org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder addCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(index, value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder addCfTimeRange(
org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder addCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(index, builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder addAllCfTimeRange(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange> values) {
ensureCfTimeRangeIsMutable();
super.addAll(values, cfTimeRange_);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder clearCfTimeRange() {
cfTimeRange_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 13;
*/
public Builder removeCfTimeRange(int index) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.remove(index);
return this;
}
// @@protoc_insertion_point(builder_scope:Get)
}
static {
defaultInstance = new Get(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Get)
}
public interface ResultOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .Cell cell = 1;
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
java.util.List
getCellList();
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
org.hbase.async.generated.CellPB.Cell getCell(int index);
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
int getCellCount();
// optional int32 associated_cell_count = 2;
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
boolean hasAssociatedCellCount();
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
int getAssociatedCellCount();
// optional bool exists = 3;
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
boolean hasExists();
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
boolean getExists();
// optional bool stale = 4 [default = false];
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
boolean hasStale();
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
boolean getStale();
// optional bool partial = 5 [default = false];
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
boolean hasPartial();
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
boolean getPartial();
}
/**
* Protobuf type {@code Result}
*/
public static final class Result extends
com.google.protobuf.GeneratedMessageLite
implements ResultOrBuilder {
// Use Result.newBuilder() to construct.
private Result(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Result(boolean noInit) {}
private static final Result defaultInstance;
public static Result getDefaultInstance() {
return defaultInstance;
}
public Result getDefaultInstanceForType() {
return defaultInstance;
}
private Result(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cell_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
cell_.add(input.readMessage(org.hbase.async.generated.CellPB.Cell.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
associatedCellCount_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000002;
exists_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000004;
stale_ = input.readBool();
break;
}
case 40: {
bitField0_ |= 0x00000008;
partial_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cell_ = java.util.Collections.unmodifiableList(cell_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Result parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Result(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated .Cell cell = 1;
public static final int CELL_FIELD_NUMBER = 1;
private java.util.List cell_;
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public java.util.List getCellList() {
return cell_;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public java.util.List extends org.hbase.async.generated.CellPB.CellOrBuilder>
getCellOrBuilderList() {
return cell_;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public int getCellCount() {
return cell_.size();
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public org.hbase.async.generated.CellPB.Cell getCell(int index) {
return cell_.get(index);
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public org.hbase.async.generated.CellPB.CellOrBuilder getCellOrBuilder(
int index) {
return cell_.get(index);
}
// optional int32 associated_cell_count = 2;
public static final int ASSOCIATED_CELL_COUNT_FIELD_NUMBER = 2;
private int associatedCellCount_;
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public boolean hasAssociatedCellCount() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public int getAssociatedCellCount() {
return associatedCellCount_;
}
// optional bool exists = 3;
public static final int EXISTS_FIELD_NUMBER = 3;
private boolean exists_;
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public boolean hasExists() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public boolean getExists() {
return exists_;
}
// optional bool stale = 4 [default = false];
public static final int STALE_FIELD_NUMBER = 4;
private boolean stale_;
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public boolean hasStale() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public boolean getStale() {
return stale_;
}
// optional bool partial = 5 [default = false];
public static final int PARTIAL_FIELD_NUMBER = 5;
private boolean partial_;
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public boolean hasPartial() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public boolean getPartial() {
return partial_;
}
private void initFields() {
cell_ = java.util.Collections.emptyList();
associatedCellCount_ = 0;
exists_ = false;
stale_ = false;
partial_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < cell_.size(); i++) {
output.writeMessage(1, cell_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(2, associatedCellCount_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(3, exists_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(4, stale_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, partial_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < cell_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, cell_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, associatedCellCount_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, exists_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, stale_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, partial_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Result parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Result parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Result parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Result prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Result}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Result, Builder>
implements org.hbase.async.generated.ClientPB.ResultOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Result.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
cell_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
associatedCellCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
exists_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
stale_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
partial_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Result getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Result build() {
org.hbase.async.generated.ClientPB.Result result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Result buildPartial() {
org.hbase.async.generated.ClientPB.Result result = new org.hbase.async.generated.ClientPB.Result(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
cell_ = java.util.Collections.unmodifiableList(cell_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.cell_ = cell_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.associatedCellCount_ = associatedCellCount_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.exists_ = exists_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.stale_ = stale_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.partial_ = partial_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Result other) {
if (other == org.hbase.async.generated.ClientPB.Result.getDefaultInstance()) return this;
if (!other.cell_.isEmpty()) {
if (cell_.isEmpty()) {
cell_ = other.cell_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCellIsMutable();
cell_.addAll(other.cell_);
}
}
if (other.hasAssociatedCellCount()) {
setAssociatedCellCount(other.getAssociatedCellCount());
}
if (other.hasExists()) {
setExists(other.getExists());
}
if (other.hasStale()) {
setStale(other.getStale());
}
if (other.hasPartial()) {
setPartial(other.getPartial());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Result parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Result) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .Cell cell = 1;
private java.util.List cell_ =
java.util.Collections.emptyList();
private void ensureCellIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
cell_ = new java.util.ArrayList(cell_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public java.util.List getCellList() {
return java.util.Collections.unmodifiableList(cell_);
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public int getCellCount() {
return cell_.size();
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public org.hbase.async.generated.CellPB.Cell getCell(int index) {
return cell_.get(index);
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder setCell(
int index, org.hbase.async.generated.CellPB.Cell value) {
if (value == null) {
throw new NullPointerException();
}
ensureCellIsMutable();
cell_.set(index, value);
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder setCell(
int index, org.hbase.async.generated.CellPB.Cell.Builder builderForValue) {
ensureCellIsMutable();
cell_.set(index, builderForValue.build());
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder addCell(org.hbase.async.generated.CellPB.Cell value) {
if (value == null) {
throw new NullPointerException();
}
ensureCellIsMutable();
cell_.add(value);
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder addCell(
int index, org.hbase.async.generated.CellPB.Cell value) {
if (value == null) {
throw new NullPointerException();
}
ensureCellIsMutable();
cell_.add(index, value);
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder addCell(
org.hbase.async.generated.CellPB.Cell.Builder builderForValue) {
ensureCellIsMutable();
cell_.add(builderForValue.build());
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder addCell(
int index, org.hbase.async.generated.CellPB.Cell.Builder builderForValue) {
ensureCellIsMutable();
cell_.add(index, builderForValue.build());
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder addAllCell(
java.lang.Iterable extends org.hbase.async.generated.CellPB.Cell> values) {
ensureCellIsMutable();
super.addAll(values, cell_);
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder clearCell() {
cell_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated .Cell cell = 1;
*
*
* Result includes the Cells or else it just has a count of Cells
* that are carried otherwise.
*
*/
public Builder removeCell(int index) {
ensureCellIsMutable();
cell_.remove(index);
return this;
}
// optional int32 associated_cell_count = 2;
private int associatedCellCount_ ;
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public boolean hasAssociatedCellCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public int getAssociatedCellCount() {
return associatedCellCount_;
}
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public Builder setAssociatedCellCount(int value) {
bitField0_ |= 0x00000002;
associatedCellCount_ = value;
return this;
}
/**
* optional int32 associated_cell_count = 2;
*
*
* The below count is set when the associated cells are
* not part of this protobuf message; they are passed alongside
* and then this Message is just a placeholder with metadata.
* The count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public Builder clearAssociatedCellCount() {
bitField0_ = (bitField0_ & ~0x00000002);
associatedCellCount_ = 0;
return this;
}
// optional bool exists = 3;
private boolean exists_ ;
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public boolean hasExists() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public boolean getExists() {
return exists_;
}
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public Builder setExists(boolean value) {
bitField0_ |= 0x00000004;
exists_ = value;
return this;
}
/**
* optional bool exists = 3;
*
*
* used for Get to check existence only. Not set if existence_only was not set to true
* in the query.
*
*/
public Builder clearExists() {
bitField0_ = (bitField0_ & ~0x00000004);
exists_ = false;
return this;
}
// optional bool stale = 4 [default = false];
private boolean stale_ ;
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public boolean hasStale() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public boolean getStale() {
return stale_;
}
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public Builder setStale(boolean value) {
bitField0_ |= 0x00000008;
stale_ = value;
return this;
}
/**
* optional bool stale = 4 [default = false];
*
*
* Whether or not the results are coming from possibly stale data
*
*/
public Builder clearStale() {
bitField0_ = (bitField0_ & ~0x00000008);
stale_ = false;
return this;
}
// optional bool partial = 5 [default = false];
private boolean partial_ ;
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public boolean hasPartial() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public boolean getPartial() {
return partial_;
}
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public Builder setPartial(boolean value) {
bitField0_ |= 0x00000010;
partial_ = value;
return this;
}
/**
* optional bool partial = 5 [default = false];
*
*
* Whether or not the entire result could be returned. Results will be split when
* the RPC chunk size limit is reached. Partial results contain only a subset of the
* cells for a row and must be combined with a result containing the remaining cells
* to form a complete result
*
*/
public Builder clearPartial() {
bitField0_ = (bitField0_ & ~0x00000010);
partial_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:Result)
}
static {
defaultInstance = new Result(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Result)
}
public interface GetRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// required .Get get = 2;
/**
* required .Get get = 2;
*/
boolean hasGet();
/**
* required .Get get = 2;
*/
org.hbase.async.generated.ClientPB.Get getGet();
}
/**
* Protobuf type {@code GetRequest}
*
*
**
* The get request. Perform a single Get operation.
*
*/
public static final class GetRequest extends
com.google.protobuf.GeneratedMessageLite
implements GetRequestOrBuilder {
// Use GetRequest.newBuilder() to construct.
private GetRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private GetRequest(boolean noInit) {}
private static final GetRequest defaultInstance;
public static GetRequest getDefaultInstance() {
return defaultInstance;
}
public GetRequest getDefaultInstanceForType() {
return defaultInstance;
}
private GetRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.hbase.async.generated.ClientPB.Get.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = get_.toBuilder();
}
get_ = input.readMessage(org.hbase.async.generated.ClientPB.Get.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(get_);
get_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public GetRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// required .Get get = 2;
public static final int GET_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.Get get_;
/**
* required .Get get = 2;
*/
public boolean hasGet() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .Get get = 2;
*/
public org.hbase.async.generated.ClientPB.Get getGet() {
return get_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasGet()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getGet().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, get_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, get_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.GetRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code GetRequest}
*
*
**
* The get request. Perform a single Get operation.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.GetRequest, Builder>
implements org.hbase.async.generated.ClientPB.GetRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.GetRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.GetRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.GetRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.GetRequest build() {
org.hbase.async.generated.ClientPB.GetRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.GetRequest buildPartial() {
org.hbase.async.generated.ClientPB.GetRequest result = new org.hbase.async.generated.ClientPB.GetRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.get_ = get_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.GetRequest other) {
if (other == org.hbase.async.generated.ClientPB.GetRequest.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasGet()) {
mergeGet(other.getGet());
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!hasGet()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
if (!getGet().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.GetRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.GetRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// required .Get get = 2;
private org.hbase.async.generated.ClientPB.Get get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
/**
* required .Get get = 2;
*/
public boolean hasGet() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .Get get = 2;
*/
public org.hbase.async.generated.ClientPB.Get getGet() {
return get_;
}
/**
* required .Get get = 2;
*/
public Builder setGet(org.hbase.async.generated.ClientPB.Get value) {
if (value == null) {
throw new NullPointerException();
}
get_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* required .Get get = 2;
*/
public Builder setGet(
org.hbase.async.generated.ClientPB.Get.Builder builderForValue) {
get_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* required .Get get = 2;
*/
public Builder mergeGet(org.hbase.async.generated.ClientPB.Get value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
get_ != org.hbase.async.generated.ClientPB.Get.getDefaultInstance()) {
get_ =
org.hbase.async.generated.ClientPB.Get.newBuilder(get_).mergeFrom(value).buildPartial();
} else {
get_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .Get get = 2;
*/
public Builder clearGet() {
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// @@protoc_insertion_point(builder_scope:GetRequest)
}
static {
defaultInstance = new GetRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:GetRequest)
}
public interface GetResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional .Result result = 1;
/**
* optional .Result result = 1;
*/
boolean hasResult();
/**
* optional .Result result = 1;
*/
org.hbase.async.generated.ClientPB.Result getResult();
}
/**
* Protobuf type {@code GetResponse}
*/
public static final class GetResponse extends
com.google.protobuf.GeneratedMessageLite
implements GetResponseOrBuilder {
// Use GetResponse.newBuilder() to construct.
private GetResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private GetResponse(boolean noInit) {}
private static final GetResponse defaultInstance;
public static GetResponse getDefaultInstance() {
return defaultInstance;
}
public GetResponse getDefaultInstanceForType() {
return defaultInstance;
}
private GetResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.ClientPB.Result.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = result_.toBuilder();
}
result_ = input.readMessage(org.hbase.async.generated.ClientPB.Result.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(result_);
result_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public GetResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .Result result = 1;
public static final int RESULT_FIELD_NUMBER = 1;
private org.hbase.async.generated.ClientPB.Result result_;
/**
* optional .Result result = 1;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .Result result = 1;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
private void initFields() {
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, result_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, result_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.GetResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.GetResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code GetResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.GetResponse, Builder>
implements org.hbase.async.generated.ClientPB.GetResponseOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.GetResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.GetResponse getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.GetResponse.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.GetResponse build() {
org.hbase.async.generated.ClientPB.GetResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.GetResponse buildPartial() {
org.hbase.async.generated.ClientPB.GetResponse result = new org.hbase.async.generated.ClientPB.GetResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.result_ = result_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.GetResponse other) {
if (other == org.hbase.async.generated.ClientPB.GetResponse.getDefaultInstance()) return this;
if (other.hasResult()) {
mergeResult(other.getResult());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.GetResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.GetResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .Result result = 1;
private org.hbase.async.generated.ClientPB.Result result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
/**
* optional .Result result = 1;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .Result result = 1;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
/**
* optional .Result result = 1;
*/
public Builder setResult(org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
result_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder setResult(
org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
result_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder mergeResult(org.hbase.async.generated.ClientPB.Result value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
result_ != org.hbase.async.generated.ClientPB.Result.getDefaultInstance()) {
result_ =
org.hbase.async.generated.ClientPB.Result.newBuilder(result_).mergeFrom(value).buildPartial();
} else {
result_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder clearResult() {
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// @@protoc_insertion_point(builder_scope:GetResponse)
}
static {
defaultInstance = new GetResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:GetResponse)
}
public interface ConditionOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes row = 1;
/**
* required bytes row = 1;
*/
boolean hasRow();
/**
* required bytes row = 1;
*/
com.google.protobuf.ByteString getRow();
// required bytes family = 2;
/**
* required bytes family = 2;
*/
boolean hasFamily();
/**
* required bytes family = 2;
*/
com.google.protobuf.ByteString getFamily();
// required bytes qualifier = 3;
/**
* required bytes qualifier = 3;
*/
boolean hasQualifier();
/**
* required bytes qualifier = 3;
*/
com.google.protobuf.ByteString getQualifier();
// required .CompareType compare_type = 4;
/**
* required .CompareType compare_type = 4;
*/
boolean hasCompareType();
/**
* required .CompareType compare_type = 4;
*/
org.hbase.async.generated.HBasePB.CompareType getCompareType();
// required .Comparator comparator = 5;
/**
* required .Comparator comparator = 5;
*/
boolean hasComparator();
/**
* required .Comparator comparator = 5;
*/
org.hbase.async.generated.ComparatorPB.Comparator getComparator();
}
/**
* Protobuf type {@code Condition}
*
*
**
* Condition to check if the value of a given cell (row,
* family, qualifier) matches a value via a given comparator.
*
* Condition is used in check and mutate operations.
*
*/
public static final class Condition extends
com.google.protobuf.GeneratedMessageLite
implements ConditionOrBuilder {
// Use Condition.newBuilder() to construct.
private Condition(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Condition(boolean noInit) {}
private static final Condition defaultInstance;
public static Condition getDefaultInstance() {
return defaultInstance;
}
public Condition getDefaultInstanceForType() {
return defaultInstance;
}
private Condition(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
row_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
family_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
qualifier_ = input.readBytes();
break;
}
case 32: {
int rawValue = input.readEnum();
org.hbase.async.generated.HBasePB.CompareType value = org.hbase.async.generated.HBasePB.CompareType.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00000008;
compareType_ = value;
}
break;
}
case 42: {
org.hbase.async.generated.ComparatorPB.Comparator.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = comparator_.toBuilder();
}
comparator_ = input.readMessage(org.hbase.async.generated.ComparatorPB.Comparator.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(comparator_);
comparator_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Condition parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Condition(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes row = 1;
public static final int ROW_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString row_;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
// required bytes family = 2;
public static final int FAMILY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString family_;
/**
* required bytes family = 2;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes family = 2;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
// required bytes qualifier = 3;
public static final int QUALIFIER_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString qualifier_;
/**
* required bytes qualifier = 3;
*/
public boolean hasQualifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes qualifier = 3;
*/
public com.google.protobuf.ByteString getQualifier() {
return qualifier_;
}
// required .CompareType compare_type = 4;
public static final int COMPARE_TYPE_FIELD_NUMBER = 4;
private org.hbase.async.generated.HBasePB.CompareType compareType_;
/**
* required .CompareType compare_type = 4;
*/
public boolean hasCompareType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .CompareType compare_type = 4;
*/
public org.hbase.async.generated.HBasePB.CompareType getCompareType() {
return compareType_;
}
// required .Comparator comparator = 5;
public static final int COMPARATOR_FIELD_NUMBER = 5;
private org.hbase.async.generated.ComparatorPB.Comparator comparator_;
/**
* required .Comparator comparator = 5;
*/
public boolean hasComparator() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .Comparator comparator = 5;
*/
public org.hbase.async.generated.ComparatorPB.Comparator getComparator() {
return comparator_;
}
private void initFields() {
row_ = com.google.protobuf.ByteString.EMPTY;
family_ = com.google.protobuf.ByteString.EMPTY;
qualifier_ = com.google.protobuf.ByteString.EMPTY;
compareType_ = org.hbase.async.generated.HBasePB.CompareType.LESS;
comparator_ = org.hbase.async.generated.ComparatorPB.Comparator.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRow()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasFamily()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasQualifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCompareType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasComparator()) {
memoizedIsInitialized = 0;
return false;
}
if (!getComparator().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, family_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, qualifier_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, compareType_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, comparator_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, family_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, qualifier_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, compareType_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, comparator_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Condition parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Condition parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Condition parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Condition prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Condition}
*
*
**
* Condition to check if the value of a given cell (row,
* family, qualifier) matches a value via a given comparator.
*
* Condition is used in check and mutate operations.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Condition, Builder>
implements org.hbase.async.generated.ClientPB.ConditionOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Condition.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
row_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
family_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
qualifier_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
compareType_ = org.hbase.async.generated.HBasePB.CompareType.LESS;
bitField0_ = (bitField0_ & ~0x00000008);
comparator_ = org.hbase.async.generated.ComparatorPB.Comparator.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Condition getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Condition build() {
org.hbase.async.generated.ClientPB.Condition result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Condition buildPartial() {
org.hbase.async.generated.ClientPB.Condition result = new org.hbase.async.generated.ClientPB.Condition(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.row_ = row_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.family_ = family_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.qualifier_ = qualifier_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.compareType_ = compareType_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.comparator_ = comparator_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Condition other) {
if (other == org.hbase.async.generated.ClientPB.Condition.getDefaultInstance()) return this;
if (other.hasRow()) {
setRow(other.getRow());
}
if (other.hasFamily()) {
setFamily(other.getFamily());
}
if (other.hasQualifier()) {
setQualifier(other.getQualifier());
}
if (other.hasCompareType()) {
setCompareType(other.getCompareType());
}
if (other.hasComparator()) {
mergeComparator(other.getComparator());
}
return this;
}
public final boolean isInitialized() {
if (!hasRow()) {
return false;
}
if (!hasFamily()) {
return false;
}
if (!hasQualifier()) {
return false;
}
if (!hasCompareType()) {
return false;
}
if (!hasComparator()) {
return false;
}
if (!getComparator().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Condition parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Condition) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes row = 1;
private com.google.protobuf.ByteString row_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
/**
* required bytes row = 1;
*/
public Builder setRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
row_ = value;
return this;
}
/**
* required bytes row = 1;
*/
public Builder clearRow() {
bitField0_ = (bitField0_ & ~0x00000001);
row_ = getDefaultInstance().getRow();
return this;
}
// required bytes family = 2;
private com.google.protobuf.ByteString family_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes family = 2;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes family = 2;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
/**
* required bytes family = 2;
*/
public Builder setFamily(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
family_ = value;
return this;
}
/**
* required bytes family = 2;
*/
public Builder clearFamily() {
bitField0_ = (bitField0_ & ~0x00000002);
family_ = getDefaultInstance().getFamily();
return this;
}
// required bytes qualifier = 3;
private com.google.protobuf.ByteString qualifier_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes qualifier = 3;
*/
public boolean hasQualifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes qualifier = 3;
*/
public com.google.protobuf.ByteString getQualifier() {
return qualifier_;
}
/**
* required bytes qualifier = 3;
*/
public Builder setQualifier(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
qualifier_ = value;
return this;
}
/**
* required bytes qualifier = 3;
*/
public Builder clearQualifier() {
bitField0_ = (bitField0_ & ~0x00000004);
qualifier_ = getDefaultInstance().getQualifier();
return this;
}
// required .CompareType compare_type = 4;
private org.hbase.async.generated.HBasePB.CompareType compareType_ = org.hbase.async.generated.HBasePB.CompareType.LESS;
/**
* required .CompareType compare_type = 4;
*/
public boolean hasCompareType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .CompareType compare_type = 4;
*/
public org.hbase.async.generated.HBasePB.CompareType getCompareType() {
return compareType_;
}
/**
* required .CompareType compare_type = 4;
*/
public Builder setCompareType(org.hbase.async.generated.HBasePB.CompareType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
compareType_ = value;
return this;
}
/**
* required .CompareType compare_type = 4;
*/
public Builder clearCompareType() {
bitField0_ = (bitField0_ & ~0x00000008);
compareType_ = org.hbase.async.generated.HBasePB.CompareType.LESS;
return this;
}
// required .Comparator comparator = 5;
private org.hbase.async.generated.ComparatorPB.Comparator comparator_ = org.hbase.async.generated.ComparatorPB.Comparator.getDefaultInstance();
/**
* required .Comparator comparator = 5;
*/
public boolean hasComparator() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .Comparator comparator = 5;
*/
public org.hbase.async.generated.ComparatorPB.Comparator getComparator() {
return comparator_;
}
/**
* required .Comparator comparator = 5;
*/
public Builder setComparator(org.hbase.async.generated.ComparatorPB.Comparator value) {
if (value == null) {
throw new NullPointerException();
}
comparator_ = value;
bitField0_ |= 0x00000010;
return this;
}
/**
* required .Comparator comparator = 5;
*/
public Builder setComparator(
org.hbase.async.generated.ComparatorPB.Comparator.Builder builderForValue) {
comparator_ = builderForValue.build();
bitField0_ |= 0x00000010;
return this;
}
/**
* required .Comparator comparator = 5;
*/
public Builder mergeComparator(org.hbase.async.generated.ComparatorPB.Comparator value) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
comparator_ != org.hbase.async.generated.ComparatorPB.Comparator.getDefaultInstance()) {
comparator_ =
org.hbase.async.generated.ComparatorPB.Comparator.newBuilder(comparator_).mergeFrom(value).buildPartial();
} else {
comparator_ = value;
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .Comparator comparator = 5;
*/
public Builder clearComparator() {
comparator_ = org.hbase.async.generated.ComparatorPB.Comparator.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
// @@protoc_insertion_point(builder_scope:Condition)
}
static {
defaultInstance = new Condition(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Condition)
}
public interface MutationProtoOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional bytes row = 1;
/**
* optional bytes row = 1;
*/
boolean hasRow();
/**
* optional bytes row = 1;
*/
com.google.protobuf.ByteString getRow();
// optional .MutationProto.MutationType mutate_type = 2;
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
boolean hasMutateType();
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
org.hbase.async.generated.ClientPB.MutationProto.MutationType getMutateType();
// repeated .MutationProto.ColumnValue column_value = 3;
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
java.util.List
getColumnValueList();
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue getColumnValue(int index);
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
int getColumnValueCount();
// optional uint64 timestamp = 4;
/**
* optional uint64 timestamp = 4;
*/
boolean hasTimestamp();
/**
* optional uint64 timestamp = 4;
*/
long getTimestamp();
// repeated .NameBytesPair attribute = 5;
/**
* repeated .NameBytesPair attribute = 5;
*/
java.util.List
getAttributeList();
/**
* repeated .NameBytesPair attribute = 5;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index);
/**
* repeated .NameBytesPair attribute = 5;
*/
int getAttributeCount();
// optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
boolean hasDurability();
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
org.hbase.async.generated.ClientPB.MutationProto.Durability getDurability();
// optional .TimeRange time_range = 7;
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
boolean hasTimeRange();
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
org.hbase.async.generated.HBasePB.TimeRange getTimeRange();
// optional int32 associated_cell_count = 8;
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
boolean hasAssociatedCellCount();
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
int getAssociatedCellCount();
// optional uint64 nonce = 9;
/**
* optional uint64 nonce = 9;
*/
boolean hasNonce();
/**
* optional uint64 nonce = 9;
*/
long getNonce();
}
/**
* Protobuf type {@code MutationProto}
*
*
**
* A specific mutation inside a mutate request.
* It can be an append, increment, put or delete based
* on the mutation type. It can be fully filled in or
* only metadata present because data is being carried
* elsewhere outside of pb.
*
*/
public static final class MutationProto extends
com.google.protobuf.GeneratedMessageLite
implements MutationProtoOrBuilder {
// Use MutationProto.newBuilder() to construct.
private MutationProto(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MutationProto(boolean noInit) {}
private static final MutationProto defaultInstance;
public static MutationProto getDefaultInstance() {
return defaultInstance;
}
public MutationProto getDefaultInstanceForType() {
return defaultInstance;
}
private MutationProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
row_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
org.hbase.async.generated.ClientPB.MutationProto.MutationType value = org.hbase.async.generated.ClientPB.MutationProto.MutationType.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00000002;
mutateType_ = value;
}
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
columnValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
columnValue_.add(input.readMessage(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.PARSER, extensionRegistry));
break;
}
case 32: {
bitField0_ |= 0x00000004;
timestamp_ = input.readUInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
attribute_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
attribute_.add(input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry));
break;
}
case 48: {
int rawValue = input.readEnum();
org.hbase.async.generated.ClientPB.MutationProto.Durability value = org.hbase.async.generated.ClientPB.MutationProto.Durability.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00000008;
durability_ = value;
}
break;
}
case 58: {
org.hbase.async.generated.HBasePB.TimeRange.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = timeRange_.toBuilder();
}
timeRange_ = input.readMessage(org.hbase.async.generated.HBasePB.TimeRange.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timeRange_);
timeRange_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 64: {
bitField0_ |= 0x00000020;
associatedCellCount_ = input.readInt32();
break;
}
case 72: {
bitField0_ |= 0x00000040;
nonce_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
columnValue_ = java.util.Collections.unmodifiableList(columnValue_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MutationProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MutationProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code MutationProto.Durability}
*/
public enum Durability
implements com.google.protobuf.Internal.EnumLite {
/**
* USE_DEFAULT = 0;
*/
USE_DEFAULT(0, 0),
/**
* SKIP_WAL = 1;
*/
SKIP_WAL(1, 1),
/**
* ASYNC_WAL = 2;
*/
ASYNC_WAL(2, 2),
/**
* SYNC_WAL = 3;
*/
SYNC_WAL(3, 3),
/**
* FSYNC_WAL = 4;
*/
FSYNC_WAL(4, 4),
;
/**
* USE_DEFAULT = 0;
*/
public static final int USE_DEFAULT_VALUE = 0;
/**
* SKIP_WAL = 1;
*/
public static final int SKIP_WAL_VALUE = 1;
/**
* ASYNC_WAL = 2;
*/
public static final int ASYNC_WAL_VALUE = 2;
/**
* SYNC_WAL = 3;
*/
public static final int SYNC_WAL_VALUE = 3;
/**
* FSYNC_WAL = 4;
*/
public static final int FSYNC_WAL_VALUE = 4;
public final int getNumber() { return value; }
public static Durability valueOf(int value) {
switch (value) {
case 0: return USE_DEFAULT;
case 1: return SKIP_WAL;
case 2: return ASYNC_WAL;
case 3: return SYNC_WAL;
case 4: return FSYNC_WAL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Durability findValueByNumber(int number) {
return Durability.valueOf(number);
}
};
private final int value;
private Durability(int index, int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:MutationProto.Durability)
}
/**
* Protobuf enum {@code MutationProto.MutationType}
*/
public enum MutationType
implements com.google.protobuf.Internal.EnumLite {
/**
* APPEND = 0;
*/
APPEND(0, 0),
/**
* INCREMENT = 1;
*/
INCREMENT(1, 1),
/**
* PUT = 2;
*/
PUT(2, 2),
/**
* DELETE = 3;
*/
DELETE(3, 3),
;
/**
* APPEND = 0;
*/
public static final int APPEND_VALUE = 0;
/**
* INCREMENT = 1;
*/
public static final int INCREMENT_VALUE = 1;
/**
* PUT = 2;
*/
public static final int PUT_VALUE = 2;
/**
* DELETE = 3;
*/
public static final int DELETE_VALUE = 3;
public final int getNumber() { return value; }
public static MutationType valueOf(int value) {
switch (value) {
case 0: return APPEND;
case 1: return INCREMENT;
case 2: return PUT;
case 3: return DELETE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MutationType findValueByNumber(int number) {
return MutationType.valueOf(number);
}
};
private final int value;
private MutationType(int index, int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:MutationProto.MutationType)
}
/**
* Protobuf enum {@code MutationProto.DeleteType}
*/
public enum DeleteType
implements com.google.protobuf.Internal.EnumLite {
/**
* DELETE_ONE_VERSION = 0;
*/
DELETE_ONE_VERSION(0, 0),
/**
* DELETE_MULTIPLE_VERSIONS = 1;
*/
DELETE_MULTIPLE_VERSIONS(1, 1),
/**
* DELETE_FAMILY = 2;
*/
DELETE_FAMILY(2, 2),
/**
* DELETE_FAMILY_VERSION = 3;
*/
DELETE_FAMILY_VERSION(3, 3),
;
/**
* DELETE_ONE_VERSION = 0;
*/
public static final int DELETE_ONE_VERSION_VALUE = 0;
/**
* DELETE_MULTIPLE_VERSIONS = 1;
*/
public static final int DELETE_MULTIPLE_VERSIONS_VALUE = 1;
/**
* DELETE_FAMILY = 2;
*/
public static final int DELETE_FAMILY_VALUE = 2;
/**
* DELETE_FAMILY_VERSION = 3;
*/
public static final int DELETE_FAMILY_VERSION_VALUE = 3;
public final int getNumber() { return value; }
public static DeleteType valueOf(int value) {
switch (value) {
case 0: return DELETE_ONE_VERSION;
case 1: return DELETE_MULTIPLE_VERSIONS;
case 2: return DELETE_FAMILY;
case 3: return DELETE_FAMILY_VERSION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DeleteType findValueByNumber(int number) {
return DeleteType.valueOf(number);
}
};
private final int value;
private DeleteType(int index, int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:MutationProto.DeleteType)
}
public interface ColumnValueOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes family = 1;
/**
* required bytes family = 1;
*/
boolean hasFamily();
/**
* required bytes family = 1;
*/
com.google.protobuf.ByteString getFamily();
// repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
java.util.List
getQualifierValueList();
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue getQualifierValue(int index);
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
int getQualifierValueCount();
}
/**
* Protobuf type {@code MutationProto.ColumnValue}
*/
public static final class ColumnValue extends
com.google.protobuf.GeneratedMessageLite
implements ColumnValueOrBuilder {
// Use ColumnValue.newBuilder() to construct.
private ColumnValue(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private ColumnValue(boolean noInit) {}
private static final ColumnValue defaultInstance;
public static ColumnValue getDefaultInstance() {
return defaultInstance;
}
public ColumnValue getDefaultInstanceForType() {
return defaultInstance;
}
private ColumnValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
family_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
qualifierValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
qualifierValue_.add(input.readMessage(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
qualifierValue_ = java.util.Collections.unmodifiableList(qualifierValue_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ColumnValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnValue(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface QualifierValueOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional bytes qualifier = 1;
/**
* optional bytes qualifier = 1;
*/
boolean hasQualifier();
/**
* optional bytes qualifier = 1;
*/
com.google.protobuf.ByteString getQualifier();
// optional bytes value = 2;
/**
* optional bytes value = 2;
*/
boolean hasValue();
/**
* optional bytes value = 2;
*/
com.google.protobuf.ByteString getValue();
// optional uint64 timestamp = 3;
/**
* optional uint64 timestamp = 3;
*/
boolean hasTimestamp();
/**
* optional uint64 timestamp = 3;
*/
long getTimestamp();
// optional .MutationProto.DeleteType delete_type = 4;
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
boolean hasDeleteType();
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
org.hbase.async.generated.ClientPB.MutationProto.DeleteType getDeleteType();
// optional bytes tags = 5;
/**
* optional bytes tags = 5;
*/
boolean hasTags();
/**
* optional bytes tags = 5;
*/
com.google.protobuf.ByteString getTags();
}
/**
* Protobuf type {@code MutationProto.ColumnValue.QualifierValue}
*/
public static final class QualifierValue extends
com.google.protobuf.GeneratedMessageLite
implements QualifierValueOrBuilder {
// Use QualifierValue.newBuilder() to construct.
private QualifierValue(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private QualifierValue(boolean noInit) {}
private static final QualifierValue defaultInstance;
public static QualifierValue getDefaultInstance() {
return defaultInstance;
}
public QualifierValue getDefaultInstanceForType() {
return defaultInstance;
}
private QualifierValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
qualifier_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
timestamp_ = input.readUInt64();
break;
}
case 32: {
int rawValue = input.readEnum();
org.hbase.async.generated.ClientPB.MutationProto.DeleteType value = org.hbase.async.generated.ClientPB.MutationProto.DeleteType.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00000008;
deleteType_ = value;
}
break;
}
case 42: {
bitField0_ |= 0x00000010;
tags_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public QualifierValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QualifierValue(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional bytes qualifier = 1;
public static final int QUALIFIER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString qualifier_;
/**
* optional bytes qualifier = 1;
*/
public boolean hasQualifier() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes qualifier = 1;
*/
public com.google.protobuf.ByteString getQualifier() {
return qualifier_;
}
// optional bytes value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString value_;
/**
* optional bytes value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes value = 2;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
// optional uint64 timestamp = 3;
public static final int TIMESTAMP_FIELD_NUMBER = 3;
private long timestamp_;
/**
* optional uint64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
// optional .MutationProto.DeleteType delete_type = 4;
public static final int DELETE_TYPE_FIELD_NUMBER = 4;
private org.hbase.async.generated.ClientPB.MutationProto.DeleteType deleteType_;
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public boolean hasDeleteType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public org.hbase.async.generated.ClientPB.MutationProto.DeleteType getDeleteType() {
return deleteType_;
}
// optional bytes tags = 5;
public static final int TAGS_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString tags_;
/**
* optional bytes tags = 5;
*/
public boolean hasTags() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bytes tags = 5;
*/
public com.google.protobuf.ByteString getTags() {
return tags_;
}
private void initFields() {
qualifier_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
timestamp_ = 0L;
deleteType_ = org.hbase.async.generated.ClientPB.MutationProto.DeleteType.DELETE_ONE_VERSION;
tags_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, qualifier_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, value_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, deleteType_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, tags_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, qualifier_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, value_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, deleteType_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, tags_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MutationProto.ColumnValue.QualifierValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue, Builder>
implements org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValueOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
qualifier_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
value_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
deleteType_ = org.hbase.async.generated.ClientPB.MutationProto.DeleteType.DELETE_ONE_VERSION;
bitField0_ = (bitField0_ & ~0x00000008);
tags_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue build() {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue buildPartial() {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue result = new org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.qualifier_ = qualifier_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.deleteType_ = deleteType_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.tags_ = tags_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue other) {
if (other == org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.getDefaultInstance()) return this;
if (other.hasQualifier()) {
setQualifier(other.getQualifier());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasDeleteType()) {
setDeleteType(other.getDeleteType());
}
if (other.hasTags()) {
setTags(other.getTags());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional bytes qualifier = 1;
private com.google.protobuf.ByteString qualifier_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes qualifier = 1;
*/
public boolean hasQualifier() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes qualifier = 1;
*/
public com.google.protobuf.ByteString getQualifier() {
return qualifier_;
}
/**
* optional bytes qualifier = 1;
*/
public Builder setQualifier(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
qualifier_ = value;
return this;
}
/**
* optional bytes qualifier = 1;
*/
public Builder clearQualifier() {
bitField0_ = (bitField0_ & ~0x00000001);
qualifier_ = getDefaultInstance().getQualifier();
return this;
}
// optional bytes value = 2;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes value = 2;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* optional bytes value = 2;
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
return this;
}
/**
* optional bytes value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
return this;
}
// optional uint64 timestamp = 3;
private long timestamp_ ;
/**
* optional uint64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional uint64 timestamp = 3;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000004;
timestamp_ = value;
return this;
}
/**
* optional uint64 timestamp = 3;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
return this;
}
// optional .MutationProto.DeleteType delete_type = 4;
private org.hbase.async.generated.ClientPB.MutationProto.DeleteType deleteType_ = org.hbase.async.generated.ClientPB.MutationProto.DeleteType.DELETE_ONE_VERSION;
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public boolean hasDeleteType() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public org.hbase.async.generated.ClientPB.MutationProto.DeleteType getDeleteType() {
return deleteType_;
}
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public Builder setDeleteType(org.hbase.async.generated.ClientPB.MutationProto.DeleteType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
deleteType_ = value;
return this;
}
/**
* optional .MutationProto.DeleteType delete_type = 4;
*/
public Builder clearDeleteType() {
bitField0_ = (bitField0_ & ~0x00000008);
deleteType_ = org.hbase.async.generated.ClientPB.MutationProto.DeleteType.DELETE_ONE_VERSION;
return this;
}
// optional bytes tags = 5;
private com.google.protobuf.ByteString tags_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes tags = 5;
*/
public boolean hasTags() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bytes tags = 5;
*/
public com.google.protobuf.ByteString getTags() {
return tags_;
}
/**
* optional bytes tags = 5;
*/
public Builder setTags(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
tags_ = value;
return this;
}
/**
* optional bytes tags = 5;
*/
public Builder clearTags() {
bitField0_ = (bitField0_ & ~0x00000010);
tags_ = getDefaultInstance().getTags();
return this;
}
// @@protoc_insertion_point(builder_scope:MutationProto.ColumnValue.QualifierValue)
}
static {
defaultInstance = new QualifierValue(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MutationProto.ColumnValue.QualifierValue)
}
private int bitField0_;
// required bytes family = 1;
public static final int FAMILY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString family_;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
// repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
public static final int QUALIFIER_VALUE_FIELD_NUMBER = 2;
private java.util.List qualifierValue_;
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public java.util.List getQualifierValueList() {
return qualifierValue_;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValueOrBuilder>
getQualifierValueOrBuilderList() {
return qualifierValue_;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public int getQualifierValueCount() {
return qualifierValue_.size();
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue getQualifierValue(int index) {
return qualifierValue_.get(index);
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValueOrBuilder getQualifierValueOrBuilder(
int index) {
return qualifierValue_.get(index);
}
private void initFields() {
family_ = com.google.protobuf.ByteString.EMPTY;
qualifierValue_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasFamily()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, family_);
}
for (int i = 0; i < qualifierValue_.size(); i++) {
output.writeMessage(2, qualifierValue_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, family_);
}
for (int i = 0; i < qualifierValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, qualifierValue_.get(i));
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MutationProto.ColumnValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue, Builder>
implements org.hbase.async.generated.ClientPB.MutationProto.ColumnValueOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
family_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
qualifierValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue build() {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue buildPartial() {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue result = new org.hbase.async.generated.ClientPB.MutationProto.ColumnValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.family_ = family_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
qualifierValue_ = java.util.Collections.unmodifiableList(qualifierValue_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.qualifierValue_ = qualifierValue_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue other) {
if (other == org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.getDefaultInstance()) return this;
if (other.hasFamily()) {
setFamily(other.getFamily());
}
if (!other.qualifierValue_.isEmpty()) {
if (qualifierValue_.isEmpty()) {
qualifierValue_ = other.qualifierValue_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureQualifierValueIsMutable();
qualifierValue_.addAll(other.qualifierValue_);
}
}
return this;
}
public final boolean isInitialized() {
if (!hasFamily()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MutationProto.ColumnValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes family = 1;
private com.google.protobuf.ByteString family_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
/**
* required bytes family = 1;
*/
public Builder setFamily(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
family_ = value;
return this;
}
/**
* required bytes family = 1;
*/
public Builder clearFamily() {
bitField0_ = (bitField0_ & ~0x00000001);
family_ = getDefaultInstance().getFamily();
return this;
}
// repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
private java.util.List qualifierValue_ =
java.util.Collections.emptyList();
private void ensureQualifierValueIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
qualifierValue_ = new java.util.ArrayList(qualifierValue_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public java.util.List getQualifierValueList() {
return java.util.Collections.unmodifiableList(qualifierValue_);
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public int getQualifierValueCount() {
return qualifierValue_.size();
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue getQualifierValue(int index) {
return qualifierValue_.get(index);
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder setQualifierValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureQualifierValueIsMutable();
qualifierValue_.set(index, value);
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder setQualifierValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.Builder builderForValue) {
ensureQualifierValueIsMutable();
qualifierValue_.set(index, builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder addQualifierValue(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureQualifierValueIsMutable();
qualifierValue_.add(value);
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder addQualifierValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureQualifierValueIsMutable();
qualifierValue_.add(index, value);
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder addQualifierValue(
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.Builder builderForValue) {
ensureQualifierValueIsMutable();
qualifierValue_.add(builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder addQualifierValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue.Builder builderForValue) {
ensureQualifierValueIsMutable();
qualifierValue_.add(index, builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder addAllQualifierValue(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.QualifierValue> values) {
ensureQualifierValueIsMutable();
super.addAll(values, qualifierValue_);
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder clearQualifierValue() {
qualifierValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* repeated .MutationProto.ColumnValue.QualifierValue qualifier_value = 2;
*/
public Builder removeQualifierValue(int index) {
ensureQualifierValueIsMutable();
qualifierValue_.remove(index);
return this;
}
// @@protoc_insertion_point(builder_scope:MutationProto.ColumnValue)
}
static {
defaultInstance = new ColumnValue(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MutationProto.ColumnValue)
}
private int bitField0_;
// optional bytes row = 1;
public static final int ROW_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString row_;
/**
* optional bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
// optional .MutationProto.MutationType mutate_type = 2;
public static final int MUTATE_TYPE_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.MutationProto.MutationType mutateType_;
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public boolean hasMutateType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto.MutationType getMutateType() {
return mutateType_;
}
// repeated .MutationProto.ColumnValue column_value = 3;
public static final int COLUMN_VALUE_FIELD_NUMBER = 3;
private java.util.List columnValue_;
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public java.util.List getColumnValueList() {
return columnValue_;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.MutationProto.ColumnValueOrBuilder>
getColumnValueOrBuilderList() {
return columnValue_;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public int getColumnValueCount() {
return columnValue_.size();
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue getColumnValue(int index) {
return columnValue_.get(index);
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValueOrBuilder getColumnValueOrBuilder(
int index) {
return columnValue_.get(index);
}
// optional uint64 timestamp = 4;
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_;
/**
* optional uint64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
// repeated .NameBytesPair attribute = 5;
public static final int ATTRIBUTE_FIELD_NUMBER = 5;
private java.util.List attribute_;
/**
* repeated .NameBytesPair attribute = 5;
*/
public java.util.List getAttributeList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder>
getAttributeOrBuilderList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder getAttributeOrBuilder(
int index) {
return attribute_.get(index);
}
// optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
public static final int DURABILITY_FIELD_NUMBER = 6;
private org.hbase.async.generated.ClientPB.MutationProto.Durability durability_;
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public boolean hasDurability() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public org.hbase.async.generated.ClientPB.MutationProto.Durability getDurability() {
return durability_;
}
// optional .TimeRange time_range = 7;
public static final int TIME_RANGE_FIELD_NUMBER = 7;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_;
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
// optional int32 associated_cell_count = 8;
public static final int ASSOCIATED_CELL_COUNT_FIELD_NUMBER = 8;
private int associatedCellCount_;
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public boolean hasAssociatedCellCount() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public int getAssociatedCellCount() {
return associatedCellCount_;
}
// optional uint64 nonce = 9;
public static final int NONCE_FIELD_NUMBER = 9;
private long nonce_;
/**
* optional uint64 nonce = 9;
*/
public boolean hasNonce() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint64 nonce = 9;
*/
public long getNonce() {
return nonce_;
}
private void initFields() {
row_ = com.google.protobuf.ByteString.EMPTY;
mutateType_ = org.hbase.async.generated.ClientPB.MutationProto.MutationType.APPEND;
columnValue_ = java.util.Collections.emptyList();
timestamp_ = 0L;
attribute_ = java.util.Collections.emptyList();
durability_ = org.hbase.async.generated.ClientPB.MutationProto.Durability.USE_DEFAULT;
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
associatedCellCount_ = 0;
nonce_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getColumnValueCount(); i++) {
if (!getColumnValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, mutateType_.getNumber());
}
for (int i = 0; i < columnValue_.size(); i++) {
output.writeMessage(3, columnValue_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(4, timestamp_);
}
for (int i = 0; i < attribute_.size(); i++) {
output.writeMessage(5, attribute_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(6, durability_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(7, timeRange_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt32(8, associatedCellCount_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeUInt64(9, nonce_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, mutateType_.getNumber());
}
for (int i = 0; i < columnValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, columnValue_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, timestamp_);
}
for (int i = 0; i < attribute_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, attribute_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, durability_.getNumber());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, timeRange_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, associatedCellCount_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(9, nonce_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutationProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MutationProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MutationProto}
*
*
**
* A specific mutation inside a mutate request.
* It can be an append, increment, put or delete based
* on the mutation type. It can be fully filled in or
* only metadata present because data is being carried
* elsewhere outside of pb.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MutationProto, Builder>
implements org.hbase.async.generated.ClientPB.MutationProtoOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MutationProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
row_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
mutateType_ = org.hbase.async.generated.ClientPB.MutationProto.MutationType.APPEND;
bitField0_ = (bitField0_ & ~0x00000002);
columnValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
durability_ = org.hbase.async.generated.ClientPB.MutationProto.Durability.USE_DEFAULT;
bitField0_ = (bitField0_ & ~0x00000020);
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000040);
associatedCellCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
nonce_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MutationProto getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MutationProto build() {
org.hbase.async.generated.ClientPB.MutationProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MutationProto buildPartial() {
org.hbase.async.generated.ClientPB.MutationProto result = new org.hbase.async.generated.ClientPB.MutationProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.row_ = row_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.mutateType_ = mutateType_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
columnValue_ = java.util.Collections.unmodifiableList(columnValue_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.columnValue_ = columnValue_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.timestamp_ = timestamp_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.attribute_ = attribute_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.durability_ = durability_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.timeRange_ = timeRange_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000020;
}
result.associatedCellCount_ = associatedCellCount_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
result.nonce_ = nonce_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MutationProto other) {
if (other == org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance()) return this;
if (other.hasRow()) {
setRow(other.getRow());
}
if (other.hasMutateType()) {
setMutateType(other.getMutateType());
}
if (!other.columnValue_.isEmpty()) {
if (columnValue_.isEmpty()) {
columnValue_ = other.columnValue_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureColumnValueIsMutable();
columnValue_.addAll(other.columnValue_);
}
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (!other.attribute_.isEmpty()) {
if (attribute_.isEmpty()) {
attribute_ = other.attribute_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureAttributeIsMutable();
attribute_.addAll(other.attribute_);
}
}
if (other.hasDurability()) {
setDurability(other.getDurability());
}
if (other.hasTimeRange()) {
mergeTimeRange(other.getTimeRange());
}
if (other.hasAssociatedCellCount()) {
setAssociatedCellCount(other.getAssociatedCellCount());
}
if (other.hasNonce()) {
setNonce(other.getNonce());
}
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getColumnValueCount(); i++) {
if (!getColumnValue(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MutationProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MutationProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional bytes row = 1;
private com.google.protobuf.ByteString row_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
/**
* optional bytes row = 1;
*/
public Builder setRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
row_ = value;
return this;
}
/**
* optional bytes row = 1;
*/
public Builder clearRow() {
bitField0_ = (bitField0_ & ~0x00000001);
row_ = getDefaultInstance().getRow();
return this;
}
// optional .MutationProto.MutationType mutate_type = 2;
private org.hbase.async.generated.ClientPB.MutationProto.MutationType mutateType_ = org.hbase.async.generated.ClientPB.MutationProto.MutationType.APPEND;
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public boolean hasMutateType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto.MutationType getMutateType() {
return mutateType_;
}
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public Builder setMutateType(org.hbase.async.generated.ClientPB.MutationProto.MutationType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
mutateType_ = value;
return this;
}
/**
* optional .MutationProto.MutationType mutate_type = 2;
*/
public Builder clearMutateType() {
bitField0_ = (bitField0_ & ~0x00000002);
mutateType_ = org.hbase.async.generated.ClientPB.MutationProto.MutationType.APPEND;
return this;
}
// repeated .MutationProto.ColumnValue column_value = 3;
private java.util.List columnValue_ =
java.util.Collections.emptyList();
private void ensureColumnValueIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
columnValue_ = new java.util.ArrayList(columnValue_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public java.util.List getColumnValueList() {
return java.util.Collections.unmodifiableList(columnValue_);
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public int getColumnValueCount() {
return columnValue_.size();
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public org.hbase.async.generated.ClientPB.MutationProto.ColumnValue getColumnValue(int index) {
return columnValue_.get(index);
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder setColumnValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnValueIsMutable();
columnValue_.set(index, value);
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder setColumnValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.Builder builderForValue) {
ensureColumnValueIsMutable();
columnValue_.set(index, builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder addColumnValue(org.hbase.async.generated.ClientPB.MutationProto.ColumnValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnValueIsMutable();
columnValue_.add(value);
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder addColumnValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnValueIsMutable();
columnValue_.add(index, value);
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder addColumnValue(
org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.Builder builderForValue) {
ensureColumnValueIsMutable();
columnValue_.add(builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder addColumnValue(
int index, org.hbase.async.generated.ClientPB.MutationProto.ColumnValue.Builder builderForValue) {
ensureColumnValueIsMutable();
columnValue_.add(index, builderForValue.build());
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder addAllColumnValue(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.MutationProto.ColumnValue> values) {
ensureColumnValueIsMutable();
super.addAll(values, columnValue_);
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder clearColumnValue() {
columnValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* repeated .MutationProto.ColumnValue column_value = 3;
*/
public Builder removeColumnValue(int index) {
ensureColumnValueIsMutable();
columnValue_.remove(index);
return this;
}
// optional uint64 timestamp = 4;
private long timestamp_ ;
/**
* optional uint64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional uint64 timestamp = 4;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000008;
timestamp_ = value;
return this;
}
/**
* optional uint64 timestamp = 4;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
timestamp_ = 0L;
return this;
}
// repeated .NameBytesPair attribute = 5;
private java.util.List attribute_ =
java.util.Collections.emptyList();
private void ensureAttributeIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
attribute_ = new java.util.ArrayList(attribute_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public java.util.List getAttributeList() {
return java.util.Collections.unmodifiableList(attribute_);
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.set(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.set(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder addAttribute(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(value);
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder addAttribute(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder addAllAttribute(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.NameBytesPair> values) {
ensureAttributeIsMutable();
super.addAll(values, attribute_);
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder clearAttribute() {
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* repeated .NameBytesPair attribute = 5;
*/
public Builder removeAttribute(int index) {
ensureAttributeIsMutable();
attribute_.remove(index);
return this;
}
// optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
private org.hbase.async.generated.ClientPB.MutationProto.Durability durability_ = org.hbase.async.generated.ClientPB.MutationProto.Durability.USE_DEFAULT;
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public boolean hasDurability() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public org.hbase.async.generated.ClientPB.MutationProto.Durability getDurability() {
return durability_;
}
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public Builder setDurability(org.hbase.async.generated.ClientPB.MutationProto.Durability value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
durability_ = value;
return this;
}
/**
* optional .MutationProto.Durability durability = 6 [default = USE_DEFAULT];
*/
public Builder clearDurability() {
bitField0_ = (bitField0_ & ~0x00000020);
durability_ = org.hbase.async.generated.ClientPB.MutationProto.Durability.USE_DEFAULT;
return this;
}
// optional .TimeRange time_range = 7;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public Builder setTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (value == null) {
throw new NullPointerException();
}
timeRange_ = value;
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public Builder setTimeRange(
org.hbase.async.generated.HBasePB.TimeRange.Builder builderForValue) {
timeRange_ = builderForValue.build();
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public Builder mergeTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
timeRange_ != org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance()) {
timeRange_ =
org.hbase.async.generated.HBasePB.TimeRange.newBuilder(timeRange_).mergeFrom(value).buildPartial();
} else {
timeRange_ = value;
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .TimeRange time_range = 7;
*
*
* For some mutations, a result may be returned, in which case,
* time range can be specified for potential performance gain
*
*/
public Builder clearTimeRange() {
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
// optional int32 associated_cell_count = 8;
private int associatedCellCount_ ;
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public boolean hasAssociatedCellCount() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public int getAssociatedCellCount() {
return associatedCellCount_;
}
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public Builder setAssociatedCellCount(int value) {
bitField0_ |= 0x00000080;
associatedCellCount_ = value;
return this;
}
/**
* optional int32 associated_cell_count = 8;
*
*
* The below count is set when the associated cells are NOT
* part of this protobuf message; they are passed alongside
* and then this Message is a placeholder with metadata. The
* count is needed to know how many to peel off the block of Cells as
* ours. NOTE: This is different from the pb managed cell_count of the
* 'cell' field above which is non-null when the cells are pb'd.
*
*/
public Builder clearAssociatedCellCount() {
bitField0_ = (bitField0_ & ~0x00000080);
associatedCellCount_ = 0;
return this;
}
// optional uint64 nonce = 9;
private long nonce_ ;
/**
* optional uint64 nonce = 9;
*/
public boolean hasNonce() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint64 nonce = 9;
*/
public long getNonce() {
return nonce_;
}
/**
* optional uint64 nonce = 9;
*/
public Builder setNonce(long value) {
bitField0_ |= 0x00000100;
nonce_ = value;
return this;
}
/**
* optional uint64 nonce = 9;
*/
public Builder clearNonce() {
bitField0_ = (bitField0_ & ~0x00000100);
nonce_ = 0L;
return this;
}
// @@protoc_insertion_point(builder_scope:MutationProto)
}
static {
defaultInstance = new MutationProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MutationProto)
}
public interface MutateRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// required .MutationProto mutation = 2;
/**
* required .MutationProto mutation = 2;
*/
boolean hasMutation();
/**
* required .MutationProto mutation = 2;
*/
org.hbase.async.generated.ClientPB.MutationProto getMutation();
// optional .Condition condition = 3;
/**
* optional .Condition condition = 3;
*/
boolean hasCondition();
/**
* optional .Condition condition = 3;
*/
org.hbase.async.generated.ClientPB.Condition getCondition();
// optional uint64 nonce_group = 4;
/**
* optional uint64 nonce_group = 4;
*/
boolean hasNonceGroup();
/**
* optional uint64 nonce_group = 4;
*/
long getNonceGroup();
}
/**
* Protobuf type {@code MutateRequest}
*
*
**
* The mutate request. Perform a single Mutate operation.
*
* Optionally, you can specify a condition. The mutate
* will take place only if the condition is met. Otherwise,
* the mutate will be ignored. In the response result,
* parameter processed is used to indicate if the mutate
* actually happened.
*
*/
public static final class MutateRequest extends
com.google.protobuf.GeneratedMessageLite
implements MutateRequestOrBuilder {
// Use MutateRequest.newBuilder() to construct.
private MutateRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MutateRequest(boolean noInit) {}
private static final MutateRequest defaultInstance;
public static MutateRequest getDefaultInstance() {
return defaultInstance;
}
public MutateRequest getDefaultInstanceForType() {
return defaultInstance;
}
private MutateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.hbase.async.generated.ClientPB.MutationProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = mutation_.toBuilder();
}
mutation_ = input.readMessage(org.hbase.async.generated.ClientPB.MutationProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mutation_);
mutation_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.hbase.async.generated.ClientPB.Condition.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = condition_.toBuilder();
}
condition_ = input.readMessage(org.hbase.async.generated.ClientPB.Condition.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(condition_);
condition_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 32: {
bitField0_ |= 0x00000008;
nonceGroup_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MutateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MutateRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// required .MutationProto mutation = 2;
public static final int MUTATION_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.MutationProto mutation_;
/**
* required .MutationProto mutation = 2;
*/
public boolean hasMutation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .MutationProto mutation = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto getMutation() {
return mutation_;
}
// optional .Condition condition = 3;
public static final int CONDITION_FIELD_NUMBER = 3;
private org.hbase.async.generated.ClientPB.Condition condition_;
/**
* optional .Condition condition = 3;
*/
public boolean hasCondition() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Condition condition = 3;
*/
public org.hbase.async.generated.ClientPB.Condition getCondition() {
return condition_;
}
// optional uint64 nonce_group = 4;
public static final int NONCE_GROUP_FIELD_NUMBER = 4;
private long nonceGroup_;
/**
* optional uint64 nonce_group = 4;
*/
public boolean hasNonceGroup() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 nonce_group = 4;
*/
public long getNonceGroup() {
return nonceGroup_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
nonceGroup_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMutation()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getMutation().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasCondition()) {
if (!getCondition().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, mutation_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, condition_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt64(4, nonceGroup_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, mutation_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, condition_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, nonceGroup_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MutateRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MutateRequest}
*
*
**
* The mutate request. Perform a single Mutate operation.
*
* Optionally, you can specify a condition. The mutate
* will take place only if the condition is met. Otherwise,
* the mutate will be ignored. In the response result,
* parameter processed is used to indicate if the mutate
* actually happened.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MutateRequest, Builder>
implements org.hbase.async.generated.ClientPB.MutateRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MutateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
nonceGroup_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MutateRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MutateRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MutateRequest build() {
org.hbase.async.generated.ClientPB.MutateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MutateRequest buildPartial() {
org.hbase.async.generated.ClientPB.MutateRequest result = new org.hbase.async.generated.ClientPB.MutateRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.mutation_ = mutation_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.condition_ = condition_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.nonceGroup_ = nonceGroup_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MutateRequest other) {
if (other == org.hbase.async.generated.ClientPB.MutateRequest.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasMutation()) {
mergeMutation(other.getMutation());
}
if (other.hasCondition()) {
mergeCondition(other.getCondition());
}
if (other.hasNonceGroup()) {
setNonceGroup(other.getNonceGroup());
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!hasMutation()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
if (!getMutation().isInitialized()) {
return false;
}
if (hasCondition()) {
if (!getCondition().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MutateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MutateRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// required .MutationProto mutation = 2;
private org.hbase.async.generated.ClientPB.MutationProto mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
/**
* required .MutationProto mutation = 2;
*/
public boolean hasMutation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .MutationProto mutation = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto getMutation() {
return mutation_;
}
/**
* required .MutationProto mutation = 2;
*/
public Builder setMutation(org.hbase.async.generated.ClientPB.MutationProto value) {
if (value == null) {
throw new NullPointerException();
}
mutation_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* required .MutationProto mutation = 2;
*/
public Builder setMutation(
org.hbase.async.generated.ClientPB.MutationProto.Builder builderForValue) {
mutation_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* required .MutationProto mutation = 2;
*/
public Builder mergeMutation(org.hbase.async.generated.ClientPB.MutationProto value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
mutation_ != org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance()) {
mutation_ =
org.hbase.async.generated.ClientPB.MutationProto.newBuilder(mutation_).mergeFrom(value).buildPartial();
} else {
mutation_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .MutationProto mutation = 2;
*/
public Builder clearMutation() {
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// optional .Condition condition = 3;
private org.hbase.async.generated.ClientPB.Condition condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
/**
* optional .Condition condition = 3;
*/
public boolean hasCondition() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Condition condition = 3;
*/
public org.hbase.async.generated.ClientPB.Condition getCondition() {
return condition_;
}
/**
* optional .Condition condition = 3;
*/
public Builder setCondition(org.hbase.async.generated.ClientPB.Condition value) {
if (value == null) {
throw new NullPointerException();
}
condition_ = value;
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder setCondition(
org.hbase.async.generated.ClientPB.Condition.Builder builderForValue) {
condition_ = builderForValue.build();
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder mergeCondition(org.hbase.async.generated.ClientPB.Condition value) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
condition_ != org.hbase.async.generated.ClientPB.Condition.getDefaultInstance()) {
condition_ =
org.hbase.async.generated.ClientPB.Condition.newBuilder(condition_).mergeFrom(value).buildPartial();
} else {
condition_ = value;
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder clearCondition() {
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
// optional uint64 nonce_group = 4;
private long nonceGroup_ ;
/**
* optional uint64 nonce_group = 4;
*/
public boolean hasNonceGroup() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 nonce_group = 4;
*/
public long getNonceGroup() {
return nonceGroup_;
}
/**
* optional uint64 nonce_group = 4;
*/
public Builder setNonceGroup(long value) {
bitField0_ |= 0x00000008;
nonceGroup_ = value;
return this;
}
/**
* optional uint64 nonce_group = 4;
*/
public Builder clearNonceGroup() {
bitField0_ = (bitField0_ & ~0x00000008);
nonceGroup_ = 0L;
return this;
}
// @@protoc_insertion_point(builder_scope:MutateRequest)
}
static {
defaultInstance = new MutateRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MutateRequest)
}
public interface MutateResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional .Result result = 1;
/**
* optional .Result result = 1;
*/
boolean hasResult();
/**
* optional .Result result = 1;
*/
org.hbase.async.generated.ClientPB.Result getResult();
// optional bool processed = 2;
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
boolean hasProcessed();
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
boolean getProcessed();
}
/**
* Protobuf type {@code MutateResponse}
*/
public static final class MutateResponse extends
com.google.protobuf.GeneratedMessageLite
implements MutateResponseOrBuilder {
// Use MutateResponse.newBuilder() to construct.
private MutateResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MutateResponse(boolean noInit) {}
private static final MutateResponse defaultInstance;
public static MutateResponse getDefaultInstance() {
return defaultInstance;
}
public MutateResponse getDefaultInstanceForType() {
return defaultInstance;
}
private MutateResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.ClientPB.Result.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = result_.toBuilder();
}
result_ = input.readMessage(org.hbase.async.generated.ClientPB.Result.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(result_);
result_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
processed_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MutateResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MutateResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .Result result = 1;
public static final int RESULT_FIELD_NUMBER = 1;
private org.hbase.async.generated.ClientPB.Result result_;
/**
* optional .Result result = 1;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .Result result = 1;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
// optional bool processed = 2;
public static final int PROCESSED_FIELD_NUMBER = 2;
private boolean processed_;
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public boolean hasProcessed() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public boolean getProcessed() {
return processed_;
}
private void initFields() {
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
processed_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, result_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, processed_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, result_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, processed_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MutateResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MutateResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MutateResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MutateResponse, Builder>
implements org.hbase.async.generated.ClientPB.MutateResponseOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MutateResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
processed_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MutateResponse getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MutateResponse.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MutateResponse build() {
org.hbase.async.generated.ClientPB.MutateResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MutateResponse buildPartial() {
org.hbase.async.generated.ClientPB.MutateResponse result = new org.hbase.async.generated.ClientPB.MutateResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.result_ = result_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.processed_ = processed_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MutateResponse other) {
if (other == org.hbase.async.generated.ClientPB.MutateResponse.getDefaultInstance()) return this;
if (other.hasResult()) {
mergeResult(other.getResult());
}
if (other.hasProcessed()) {
setProcessed(other.getProcessed());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MutateResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MutateResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .Result result = 1;
private org.hbase.async.generated.ClientPB.Result result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
/**
* optional .Result result = 1;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .Result result = 1;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
/**
* optional .Result result = 1;
*/
public Builder setResult(org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
result_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder setResult(
org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
result_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder mergeResult(org.hbase.async.generated.ClientPB.Result value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
result_ != org.hbase.async.generated.ClientPB.Result.getDefaultInstance()) {
result_ =
org.hbase.async.generated.ClientPB.Result.newBuilder(result_).mergeFrom(value).buildPartial();
} else {
result_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .Result result = 1;
*/
public Builder clearResult() {
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// optional bool processed = 2;
private boolean processed_ ;
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public boolean hasProcessed() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public boolean getProcessed() {
return processed_;
}
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public Builder setProcessed(boolean value) {
bitField0_ |= 0x00000002;
processed_ = value;
return this;
}
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
public Builder clearProcessed() {
bitField0_ = (bitField0_ & ~0x00000002);
processed_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:MutateResponse)
}
static {
defaultInstance = new MutateResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MutateResponse)
}
public interface ScanOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .Column column = 1;
/**
* repeated .Column column = 1;
*/
java.util.List
getColumnList();
/**
* repeated .Column column = 1;
*/
org.hbase.async.generated.ClientPB.Column getColumn(int index);
/**
* repeated .Column column = 1;
*/
int getColumnCount();
// repeated .NameBytesPair attribute = 2;
/**
* repeated .NameBytesPair attribute = 2;
*/
java.util.List
getAttributeList();
/**
* repeated .NameBytesPair attribute = 2;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index);
/**
* repeated .NameBytesPair attribute = 2;
*/
int getAttributeCount();
// optional bytes start_row = 3;
/**
* optional bytes start_row = 3;
*/
boolean hasStartRow();
/**
* optional bytes start_row = 3;
*/
com.google.protobuf.ByteString getStartRow();
// optional bytes stop_row = 4;
/**
* optional bytes stop_row = 4;
*/
boolean hasStopRow();
/**
* optional bytes stop_row = 4;
*/
com.google.protobuf.ByteString getStopRow();
// optional .Filter filter = 5;
/**
* optional .Filter filter = 5;
*/
boolean hasFilter();
/**
* optional .Filter filter = 5;
*/
org.hbase.async.generated.FilterPB.Filter getFilter();
// optional .TimeRange time_range = 6;
/**
* optional .TimeRange time_range = 6;
*/
boolean hasTimeRange();
/**
* optional .TimeRange time_range = 6;
*/
org.hbase.async.generated.HBasePB.TimeRange getTimeRange();
// optional uint32 max_versions = 7 [default = 1];
/**
* optional uint32 max_versions = 7 [default = 1];
*/
boolean hasMaxVersions();
/**
* optional uint32 max_versions = 7 [default = 1];
*/
int getMaxVersions();
// optional bool cache_blocks = 8 [default = true];
/**
* optional bool cache_blocks = 8 [default = true];
*/
boolean hasCacheBlocks();
/**
* optional bool cache_blocks = 8 [default = true];
*/
boolean getCacheBlocks();
// optional uint32 batch_size = 9;
/**
* optional uint32 batch_size = 9;
*/
boolean hasBatchSize();
/**
* optional uint32 batch_size = 9;
*/
int getBatchSize();
// optional uint64 max_result_size = 10;
/**
* optional uint64 max_result_size = 10;
*/
boolean hasMaxResultSize();
/**
* optional uint64 max_result_size = 10;
*/
long getMaxResultSize();
// optional uint32 store_limit = 11;
/**
* optional uint32 store_limit = 11;
*/
boolean hasStoreLimit();
/**
* optional uint32 store_limit = 11;
*/
int getStoreLimit();
// optional uint32 store_offset = 12;
/**
* optional uint32 store_offset = 12;
*/
boolean hasStoreOffset();
/**
* optional uint32 store_offset = 12;
*/
int getStoreOffset();
// optional bool load_column_families_on_demand = 13;
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
boolean hasLoadColumnFamiliesOnDemand();
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
boolean getLoadColumnFamiliesOnDemand();
// optional bool small = 14;
/**
* optional bool small = 14;
*/
boolean hasSmall();
/**
* optional bool small = 14;
*/
boolean getSmall();
// optional bool reversed = 15 [default = false];
/**
* optional bool reversed = 15 [default = false];
*/
boolean hasReversed();
/**
* optional bool reversed = 15 [default = false];
*/
boolean getReversed();
// optional .Consistency consistency = 16 [default = STRONG];
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
boolean hasConsistency();
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
org.hbase.async.generated.ClientPB.Consistency getConsistency();
// optional uint32 caching = 17;
/**
* optional uint32 caching = 17;
*/
boolean hasCaching();
/**
* optional uint32 caching = 17;
*/
int getCaching();
// optional bool allow_partial_results = 18;
/**
* optional bool allow_partial_results = 18;
*/
boolean hasAllowPartialResults();
/**
* optional bool allow_partial_results = 18;
*/
boolean getAllowPartialResults();
// repeated .ColumnFamilyTimeRange cf_time_range = 19;
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
java.util.List
getCfTimeRangeList();
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index);
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
int getCfTimeRangeCount();
}
/**
* Protobuf type {@code Scan}
*
*
**
* Instead of get from a table, you can scan it with optional filters.
* You can specify the row key range, time range, the columns/families
* to scan and so on.
*
* This scan is used the first time in a scan request. The response of
* the initial scan will return a scanner id, which should be used to
* fetch result batches later on before it is closed.
*
*/
public static final class Scan extends
com.google.protobuf.GeneratedMessageLite
implements ScanOrBuilder {
// Use Scan.newBuilder() to construct.
private Scan(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Scan(boolean noInit) {}
private static final Scan defaultInstance;
public static Scan getDefaultInstance() {
return defaultInstance;
}
public Scan getDefaultInstanceForType() {
return defaultInstance;
}
private Scan(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
column_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
column_.add(input.readMessage(org.hbase.async.generated.ClientPB.Column.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
attribute_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
attribute_.add(input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry));
break;
}
case 26: {
bitField0_ |= 0x00000001;
startRow_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000002;
stopRow_ = input.readBytes();
break;
}
case 42: {
org.hbase.async.generated.FilterPB.Filter.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = filter_.toBuilder();
}
filter_ = input.readMessage(org.hbase.async.generated.FilterPB.Filter.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(filter_);
filter_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 50: {
org.hbase.async.generated.HBasePB.TimeRange.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = timeRange_.toBuilder();
}
timeRange_ = input.readMessage(org.hbase.async.generated.HBasePB.TimeRange.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timeRange_);
timeRange_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 56: {
bitField0_ |= 0x00000010;
maxVersions_ = input.readUInt32();
break;
}
case 64: {
bitField0_ |= 0x00000020;
cacheBlocks_ = input.readBool();
break;
}
case 72: {
bitField0_ |= 0x00000040;
batchSize_ = input.readUInt32();
break;
}
case 80: {
bitField0_ |= 0x00000080;
maxResultSize_ = input.readUInt64();
break;
}
case 88: {
bitField0_ |= 0x00000100;
storeLimit_ = input.readUInt32();
break;
}
case 96: {
bitField0_ |= 0x00000200;
storeOffset_ = input.readUInt32();
break;
}
case 104: {
bitField0_ |= 0x00000400;
loadColumnFamiliesOnDemand_ = input.readBool();
break;
}
case 112: {
bitField0_ |= 0x00000800;
small_ = input.readBool();
break;
}
case 120: {
bitField0_ |= 0x00001000;
reversed_ = input.readBool();
break;
}
case 128: {
int rawValue = input.readEnum();
org.hbase.async.generated.ClientPB.Consistency value = org.hbase.async.generated.ClientPB.Consistency.valueOf(rawValue);
if (value != null) {
bitField0_ |= 0x00002000;
consistency_ = value;
}
break;
}
case 136: {
bitField0_ |= 0x00004000;
caching_ = input.readUInt32();
break;
}
case 144: {
bitField0_ |= 0x00008000;
allowPartialResults_ = input.readBool();
break;
}
case 154: {
if (!((mutable_bitField0_ & 0x00040000) == 0x00040000)) {
cfTimeRange_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00040000;
}
cfTimeRange_.add(input.readMessage(org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
column_ = java.util.Collections.unmodifiableList(column_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
}
if (((mutable_bitField0_ & 0x00040000) == 0x00040000)) {
cfTimeRange_ = java.util.Collections.unmodifiableList(cfTimeRange_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Scan parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Scan(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated .Column column = 1;
public static final int COLUMN_FIELD_NUMBER = 1;
private java.util.List column_;
/**
* repeated .Column column = 1;
*/
public java.util.List getColumnList() {
return column_;
}
/**
* repeated .Column column = 1;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.ColumnOrBuilder>
getColumnOrBuilderList() {
return column_;
}
/**
* repeated .Column column = 1;
*/
public int getColumnCount() {
return column_.size();
}
/**
* repeated .Column column = 1;
*/
public org.hbase.async.generated.ClientPB.Column getColumn(int index) {
return column_.get(index);
}
/**
* repeated .Column column = 1;
*/
public org.hbase.async.generated.ClientPB.ColumnOrBuilder getColumnOrBuilder(
int index) {
return column_.get(index);
}
// repeated .NameBytesPair attribute = 2;
public static final int ATTRIBUTE_FIELD_NUMBER = 2;
private java.util.List attribute_;
/**
* repeated .NameBytesPair attribute = 2;
*/
public java.util.List getAttributeList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder>
getAttributeOrBuilderList() {
return attribute_;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public org.hbase.async.generated.HBasePB.NameBytesPairOrBuilder getAttributeOrBuilder(
int index) {
return attribute_.get(index);
}
// optional bytes start_row = 3;
public static final int START_ROW_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString startRow_;
/**
* optional bytes start_row = 3;
*/
public boolean hasStartRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes start_row = 3;
*/
public com.google.protobuf.ByteString getStartRow() {
return startRow_;
}
// optional bytes stop_row = 4;
public static final int STOP_ROW_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString stopRow_;
/**
* optional bytes stop_row = 4;
*/
public boolean hasStopRow() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes stop_row = 4;
*/
public com.google.protobuf.ByteString getStopRow() {
return stopRow_;
}
// optional .Filter filter = 5;
public static final int FILTER_FIELD_NUMBER = 5;
private org.hbase.async.generated.FilterPB.Filter filter_;
/**
* optional .Filter filter = 5;
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Filter filter = 5;
*/
public org.hbase.async.generated.FilterPB.Filter getFilter() {
return filter_;
}
// optional .TimeRange time_range = 6;
public static final int TIME_RANGE_FIELD_NUMBER = 6;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_;
/**
* optional .TimeRange time_range = 6;
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TimeRange time_range = 6;
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
// optional uint32 max_versions = 7 [default = 1];
public static final int MAX_VERSIONS_FIELD_NUMBER = 7;
private int maxVersions_;
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public boolean hasMaxVersions() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public int getMaxVersions() {
return maxVersions_;
}
// optional bool cache_blocks = 8 [default = true];
public static final int CACHE_BLOCKS_FIELD_NUMBER = 8;
private boolean cacheBlocks_;
/**
* optional bool cache_blocks = 8 [default = true];
*/
public boolean hasCacheBlocks() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool cache_blocks = 8 [default = true];
*/
public boolean getCacheBlocks() {
return cacheBlocks_;
}
// optional uint32 batch_size = 9;
public static final int BATCH_SIZE_FIELD_NUMBER = 9;
private int batchSize_;
/**
* optional uint32 batch_size = 9;
*/
public boolean hasBatchSize() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint32 batch_size = 9;
*/
public int getBatchSize() {
return batchSize_;
}
// optional uint64 max_result_size = 10;
public static final int MAX_RESULT_SIZE_FIELD_NUMBER = 10;
private long maxResultSize_;
/**
* optional uint64 max_result_size = 10;
*/
public boolean hasMaxResultSize() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint64 max_result_size = 10;
*/
public long getMaxResultSize() {
return maxResultSize_;
}
// optional uint32 store_limit = 11;
public static final int STORE_LIMIT_FIELD_NUMBER = 11;
private int storeLimit_;
/**
* optional uint32 store_limit = 11;
*/
public boolean hasStoreLimit() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint32 store_limit = 11;
*/
public int getStoreLimit() {
return storeLimit_;
}
// optional uint32 store_offset = 12;
public static final int STORE_OFFSET_FIELD_NUMBER = 12;
private int storeOffset_;
/**
* optional uint32 store_offset = 12;
*/
public boolean hasStoreOffset() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 store_offset = 12;
*/
public int getStoreOffset() {
return storeOffset_;
}
// optional bool load_column_families_on_demand = 13;
public static final int LOAD_COLUMN_FAMILIES_ON_DEMAND_FIELD_NUMBER = 13;
private boolean loadColumnFamiliesOnDemand_;
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public boolean hasLoadColumnFamiliesOnDemand() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public boolean getLoadColumnFamiliesOnDemand() {
return loadColumnFamiliesOnDemand_;
}
// optional bool small = 14;
public static final int SMALL_FIELD_NUMBER = 14;
private boolean small_;
/**
* optional bool small = 14;
*/
public boolean hasSmall() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional bool small = 14;
*/
public boolean getSmall() {
return small_;
}
// optional bool reversed = 15 [default = false];
public static final int REVERSED_FIELD_NUMBER = 15;
private boolean reversed_;
/**
* optional bool reversed = 15 [default = false];
*/
public boolean hasReversed() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool reversed = 15 [default = false];
*/
public boolean getReversed() {
return reversed_;
}
// optional .Consistency consistency = 16 [default = STRONG];
public static final int CONSISTENCY_FIELD_NUMBER = 16;
private org.hbase.async.generated.ClientPB.Consistency consistency_;
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public org.hbase.async.generated.ClientPB.Consistency getConsistency() {
return consistency_;
}
// optional uint32 caching = 17;
public static final int CACHING_FIELD_NUMBER = 17;
private int caching_;
/**
* optional uint32 caching = 17;
*/
public boolean hasCaching() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional uint32 caching = 17;
*/
public int getCaching() {
return caching_;
}
// optional bool allow_partial_results = 18;
public static final int ALLOW_PARTIAL_RESULTS_FIELD_NUMBER = 18;
private boolean allowPartialResults_;
/**
* optional bool allow_partial_results = 18;
*/
public boolean hasAllowPartialResults() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bool allow_partial_results = 18;
*/
public boolean getAllowPartialResults() {
return allowPartialResults_;
}
// repeated .ColumnFamilyTimeRange cf_time_range = 19;
public static final int CF_TIME_RANGE_FIELD_NUMBER = 19;
private java.util.List cfTimeRange_;
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public java.util.List getCfTimeRangeList() {
return cfTimeRange_;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.ColumnFamilyTimeRangeOrBuilder>
getCfTimeRangeOrBuilderList() {
return cfTimeRange_;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public int getCfTimeRangeCount() {
return cfTimeRange_.size();
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index) {
return cfTimeRange_.get(index);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRangeOrBuilder getCfTimeRangeOrBuilder(
int index) {
return cfTimeRange_.get(index);
}
private void initFields() {
column_ = java.util.Collections.emptyList();
attribute_ = java.util.Collections.emptyList();
startRow_ = com.google.protobuf.ByteString.EMPTY;
stopRow_ = com.google.protobuf.ByteString.EMPTY;
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
maxVersions_ = 1;
cacheBlocks_ = true;
batchSize_ = 0;
maxResultSize_ = 0L;
storeLimit_ = 0;
storeOffset_ = 0;
loadColumnFamiliesOnDemand_ = false;
small_ = false;
reversed_ = false;
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
caching_ = 0;
allowPartialResults_ = false;
cfTimeRange_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasFilter()) {
if (!getFilter().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getCfTimeRangeCount(); i++) {
if (!getCfTimeRange(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < column_.size(); i++) {
output.writeMessage(1, column_.get(i));
}
for (int i = 0; i < attribute_.size(); i++) {
output.writeMessage(2, attribute_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(3, startRow_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(4, stopRow_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(5, filter_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(6, timeRange_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(7, maxVersions_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(8, cacheBlocks_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeUInt32(9, batchSize_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt64(10, maxResultSize_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeUInt32(11, storeLimit_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeUInt32(12, storeOffset_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBool(13, loadColumnFamiliesOnDemand_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBool(14, small_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(15, reversed_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeEnum(16, consistency_.getNumber());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeUInt32(17, caching_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBool(18, allowPartialResults_);
}
for (int i = 0; i < cfTimeRange_.size(); i++) {
output.writeMessage(19, cfTimeRange_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < column_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, column_.get(i));
}
for (int i = 0; i < attribute_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, attribute_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, startRow_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, stopRow_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, filter_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, timeRange_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(7, maxVersions_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, cacheBlocks_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(9, batchSize_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(10, maxResultSize_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(11, storeLimit_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(12, storeOffset_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, loadColumnFamiliesOnDemand_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, small_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, reversed_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(16, consistency_.getNumber());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(17, caching_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(18, allowPartialResults_);
}
for (int i = 0; i < cfTimeRange_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, cfTimeRange_.get(i));
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Scan parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Scan parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Scan parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Scan prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Scan}
*
*
**
* Instead of get from a table, you can scan it with optional filters.
* You can specify the row key range, time range, the columns/families
* to scan and so on.
*
* This scan is used the first time in a scan request. The response of
* the initial scan will return a scanner id, which should be used to
* fetch result batches later on before it is closed.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Scan, Builder>
implements org.hbase.async.generated.ClientPB.ScanOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Scan.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
startRow_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
stopRow_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000020);
maxVersions_ = 1;
bitField0_ = (bitField0_ & ~0x00000040);
cacheBlocks_ = true;
bitField0_ = (bitField0_ & ~0x00000080);
batchSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
maxResultSize_ = 0L;
bitField0_ = (bitField0_ & ~0x00000200);
storeLimit_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
storeOffset_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
loadColumnFamiliesOnDemand_ = false;
bitField0_ = (bitField0_ & ~0x00001000);
small_ = false;
bitField0_ = (bitField0_ & ~0x00002000);
reversed_ = false;
bitField0_ = (bitField0_ & ~0x00004000);
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
bitField0_ = (bitField0_ & ~0x00008000);
caching_ = 0;
bitField0_ = (bitField0_ & ~0x00010000);
allowPartialResults_ = false;
bitField0_ = (bitField0_ & ~0x00020000);
cfTimeRange_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00040000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Scan getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Scan.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Scan build() {
org.hbase.async.generated.ClientPB.Scan result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Scan buildPartial() {
org.hbase.async.generated.ClientPB.Scan result = new org.hbase.async.generated.ClientPB.Scan(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
column_ = java.util.Collections.unmodifiableList(column_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.column_ = column_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
attribute_ = java.util.Collections.unmodifiableList(attribute_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.attribute_ = attribute_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000001;
}
result.startRow_ = startRow_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000002;
}
result.stopRow_ = stopRow_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000004;
}
result.filter_ = filter_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.timeRange_ = timeRange_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.maxVersions_ = maxVersions_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000020;
}
result.cacheBlocks_ = cacheBlocks_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
result.batchSize_ = batchSize_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
result.maxResultSize_ = maxResultSize_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
result.storeLimit_ = storeLimit_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
result.storeOffset_ = storeOffset_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000400;
}
result.loadColumnFamiliesOnDemand_ = loadColumnFamiliesOnDemand_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00000800;
}
result.small_ = small_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00001000;
}
result.reversed_ = reversed_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00002000;
}
result.consistency_ = consistency_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00004000;
}
result.caching_ = caching_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00008000;
}
result.allowPartialResults_ = allowPartialResults_;
if (((bitField0_ & 0x00040000) == 0x00040000)) {
cfTimeRange_ = java.util.Collections.unmodifiableList(cfTimeRange_);
bitField0_ = (bitField0_ & ~0x00040000);
}
result.cfTimeRange_ = cfTimeRange_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Scan other) {
if (other == org.hbase.async.generated.ClientPB.Scan.getDefaultInstance()) return this;
if (!other.column_.isEmpty()) {
if (column_.isEmpty()) {
column_ = other.column_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnIsMutable();
column_.addAll(other.column_);
}
}
if (!other.attribute_.isEmpty()) {
if (attribute_.isEmpty()) {
attribute_ = other.attribute_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAttributeIsMutable();
attribute_.addAll(other.attribute_);
}
}
if (other.hasStartRow()) {
setStartRow(other.getStartRow());
}
if (other.hasStopRow()) {
setStopRow(other.getStopRow());
}
if (other.hasFilter()) {
mergeFilter(other.getFilter());
}
if (other.hasTimeRange()) {
mergeTimeRange(other.getTimeRange());
}
if (other.hasMaxVersions()) {
setMaxVersions(other.getMaxVersions());
}
if (other.hasCacheBlocks()) {
setCacheBlocks(other.getCacheBlocks());
}
if (other.hasBatchSize()) {
setBatchSize(other.getBatchSize());
}
if (other.hasMaxResultSize()) {
setMaxResultSize(other.getMaxResultSize());
}
if (other.hasStoreLimit()) {
setStoreLimit(other.getStoreLimit());
}
if (other.hasStoreOffset()) {
setStoreOffset(other.getStoreOffset());
}
if (other.hasLoadColumnFamiliesOnDemand()) {
setLoadColumnFamiliesOnDemand(other.getLoadColumnFamiliesOnDemand());
}
if (other.hasSmall()) {
setSmall(other.getSmall());
}
if (other.hasReversed()) {
setReversed(other.getReversed());
}
if (other.hasConsistency()) {
setConsistency(other.getConsistency());
}
if (other.hasCaching()) {
setCaching(other.getCaching());
}
if (other.hasAllowPartialResults()) {
setAllowPartialResults(other.getAllowPartialResults());
}
if (!other.cfTimeRange_.isEmpty()) {
if (cfTimeRange_.isEmpty()) {
cfTimeRange_ = other.cfTimeRange_;
bitField0_ = (bitField0_ & ~0x00040000);
} else {
ensureCfTimeRangeIsMutable();
cfTimeRange_.addAll(other.cfTimeRange_);
}
}
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getColumnCount(); i++) {
if (!getColumn(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getAttributeCount(); i++) {
if (!getAttribute(i).isInitialized()) {
return false;
}
}
if (hasFilter()) {
if (!getFilter().isInitialized()) {
return false;
}
}
for (int i = 0; i < getCfTimeRangeCount(); i++) {
if (!getCfTimeRange(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Scan parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Scan) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .Column column = 1;
private java.util.List column_ =
java.util.Collections.emptyList();
private void ensureColumnIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
column_ = new java.util.ArrayList(column_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .Column column = 1;
*/
public java.util.List getColumnList() {
return java.util.Collections.unmodifiableList(column_);
}
/**
* repeated .Column column = 1;
*/
public int getColumnCount() {
return column_.size();
}
/**
* repeated .Column column = 1;
*/
public org.hbase.async.generated.ClientPB.Column getColumn(int index) {
return column_.get(index);
}
/**
* repeated .Column column = 1;
*/
public Builder setColumn(
int index, org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.set(index, value);
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder setColumn(
int index, org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.set(index, builderForValue.build());
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder addColumn(org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(value);
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder addColumn(
int index, org.hbase.async.generated.ClientPB.Column value) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnIsMutable();
column_.add(index, value);
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder addColumn(
org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.add(builderForValue.build());
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder addColumn(
int index, org.hbase.async.generated.ClientPB.Column.Builder builderForValue) {
ensureColumnIsMutable();
column_.add(index, builderForValue.build());
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder addAllColumn(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.Column> values) {
ensureColumnIsMutable();
super.addAll(values, column_);
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder clearColumn() {
column_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated .Column column = 1;
*/
public Builder removeColumn(int index) {
ensureColumnIsMutable();
column_.remove(index);
return this;
}
// repeated .NameBytesPair attribute = 2;
private java.util.List attribute_ =
java.util.Collections.emptyList();
private void ensureAttributeIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
attribute_ = new java.util.ArrayList(attribute_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public java.util.List getAttributeList() {
return java.util.Collections.unmodifiableList(attribute_);
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public int getAttributeCount() {
return attribute_.size();
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getAttribute(int index) {
return attribute_.get(index);
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.set(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder setAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.set(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder addAttribute(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(value);
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeIsMutable();
attribute_.add(index, value);
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder addAttribute(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder addAttribute(
int index, org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
ensureAttributeIsMutable();
attribute_.add(index, builderForValue.build());
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder addAllAttribute(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.NameBytesPair> values) {
ensureAttributeIsMutable();
super.addAll(values, attribute_);
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder clearAttribute() {
attribute_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* repeated .NameBytesPair attribute = 2;
*/
public Builder removeAttribute(int index) {
ensureAttributeIsMutable();
attribute_.remove(index);
return this;
}
// optional bytes start_row = 3;
private com.google.protobuf.ByteString startRow_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes start_row = 3;
*/
public boolean hasStartRow() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes start_row = 3;
*/
public com.google.protobuf.ByteString getStartRow() {
return startRow_;
}
/**
* optional bytes start_row = 3;
*/
public Builder setStartRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
startRow_ = value;
return this;
}
/**
* optional bytes start_row = 3;
*/
public Builder clearStartRow() {
bitField0_ = (bitField0_ & ~0x00000004);
startRow_ = getDefaultInstance().getStartRow();
return this;
}
// optional bytes stop_row = 4;
private com.google.protobuf.ByteString stopRow_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes stop_row = 4;
*/
public boolean hasStopRow() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes stop_row = 4;
*/
public com.google.protobuf.ByteString getStopRow() {
return stopRow_;
}
/**
* optional bytes stop_row = 4;
*/
public Builder setStopRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
stopRow_ = value;
return this;
}
/**
* optional bytes stop_row = 4;
*/
public Builder clearStopRow() {
bitField0_ = (bitField0_ & ~0x00000008);
stopRow_ = getDefaultInstance().getStopRow();
return this;
}
// optional .Filter filter = 5;
private org.hbase.async.generated.FilterPB.Filter filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
/**
* optional .Filter filter = 5;
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .Filter filter = 5;
*/
public org.hbase.async.generated.FilterPB.Filter getFilter() {
return filter_;
}
/**
* optional .Filter filter = 5;
*/
public Builder setFilter(org.hbase.async.generated.FilterPB.Filter value) {
if (value == null) {
throw new NullPointerException();
}
filter_ = value;
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .Filter filter = 5;
*/
public Builder setFilter(
org.hbase.async.generated.FilterPB.Filter.Builder builderForValue) {
filter_ = builderForValue.build();
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .Filter filter = 5;
*/
public Builder mergeFilter(org.hbase.async.generated.FilterPB.Filter value) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
filter_ != org.hbase.async.generated.FilterPB.Filter.getDefaultInstance()) {
filter_ =
org.hbase.async.generated.FilterPB.Filter.newBuilder(filter_).mergeFrom(value).buildPartial();
} else {
filter_ = value;
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .Filter filter = 5;
*/
public Builder clearFilter() {
filter_ = org.hbase.async.generated.FilterPB.Filter.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
// optional .TimeRange time_range = 6;
private org.hbase.async.generated.HBasePB.TimeRange timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
/**
* optional .TimeRange time_range = 6;
*/
public boolean hasTimeRange() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .TimeRange time_range = 6;
*/
public org.hbase.async.generated.HBasePB.TimeRange getTimeRange() {
return timeRange_;
}
/**
* optional .TimeRange time_range = 6;
*/
public Builder setTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (value == null) {
throw new NullPointerException();
}
timeRange_ = value;
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TimeRange time_range = 6;
*/
public Builder setTimeRange(
org.hbase.async.generated.HBasePB.TimeRange.Builder builderForValue) {
timeRange_ = builderForValue.build();
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TimeRange time_range = 6;
*/
public Builder mergeTimeRange(org.hbase.async.generated.HBasePB.TimeRange value) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
timeRange_ != org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance()) {
timeRange_ =
org.hbase.async.generated.HBasePB.TimeRange.newBuilder(timeRange_).mergeFrom(value).buildPartial();
} else {
timeRange_ = value;
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .TimeRange time_range = 6;
*/
public Builder clearTimeRange() {
timeRange_ = org.hbase.async.generated.HBasePB.TimeRange.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
// optional uint32 max_versions = 7 [default = 1];
private int maxVersions_ = 1;
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public boolean hasMaxVersions() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public int getMaxVersions() {
return maxVersions_;
}
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public Builder setMaxVersions(int value) {
bitField0_ |= 0x00000040;
maxVersions_ = value;
return this;
}
/**
* optional uint32 max_versions = 7 [default = 1];
*/
public Builder clearMaxVersions() {
bitField0_ = (bitField0_ & ~0x00000040);
maxVersions_ = 1;
return this;
}
// optional bool cache_blocks = 8 [default = true];
private boolean cacheBlocks_ = true;
/**
* optional bool cache_blocks = 8 [default = true];
*/
public boolean hasCacheBlocks() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool cache_blocks = 8 [default = true];
*/
public boolean getCacheBlocks() {
return cacheBlocks_;
}
/**
* optional bool cache_blocks = 8 [default = true];
*/
public Builder setCacheBlocks(boolean value) {
bitField0_ |= 0x00000080;
cacheBlocks_ = value;
return this;
}
/**
* optional bool cache_blocks = 8 [default = true];
*/
public Builder clearCacheBlocks() {
bitField0_ = (bitField0_ & ~0x00000080);
cacheBlocks_ = true;
return this;
}
// optional uint32 batch_size = 9;
private int batchSize_ ;
/**
* optional uint32 batch_size = 9;
*/
public boolean hasBatchSize() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint32 batch_size = 9;
*/
public int getBatchSize() {
return batchSize_;
}
/**
* optional uint32 batch_size = 9;
*/
public Builder setBatchSize(int value) {
bitField0_ |= 0x00000100;
batchSize_ = value;
return this;
}
/**
* optional uint32 batch_size = 9;
*/
public Builder clearBatchSize() {
bitField0_ = (bitField0_ & ~0x00000100);
batchSize_ = 0;
return this;
}
// optional uint64 max_result_size = 10;
private long maxResultSize_ ;
/**
* optional uint64 max_result_size = 10;
*/
public boolean hasMaxResultSize() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint64 max_result_size = 10;
*/
public long getMaxResultSize() {
return maxResultSize_;
}
/**
* optional uint64 max_result_size = 10;
*/
public Builder setMaxResultSize(long value) {
bitField0_ |= 0x00000200;
maxResultSize_ = value;
return this;
}
/**
* optional uint64 max_result_size = 10;
*/
public Builder clearMaxResultSize() {
bitField0_ = (bitField0_ & ~0x00000200);
maxResultSize_ = 0L;
return this;
}
// optional uint32 store_limit = 11;
private int storeLimit_ ;
/**
* optional uint32 store_limit = 11;
*/
public boolean hasStoreLimit() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional uint32 store_limit = 11;
*/
public int getStoreLimit() {
return storeLimit_;
}
/**
* optional uint32 store_limit = 11;
*/
public Builder setStoreLimit(int value) {
bitField0_ |= 0x00000400;
storeLimit_ = value;
return this;
}
/**
* optional uint32 store_limit = 11;
*/
public Builder clearStoreLimit() {
bitField0_ = (bitField0_ & ~0x00000400);
storeLimit_ = 0;
return this;
}
// optional uint32 store_offset = 12;
private int storeOffset_ ;
/**
* optional uint32 store_offset = 12;
*/
public boolean hasStoreOffset() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional uint32 store_offset = 12;
*/
public int getStoreOffset() {
return storeOffset_;
}
/**
* optional uint32 store_offset = 12;
*/
public Builder setStoreOffset(int value) {
bitField0_ |= 0x00000800;
storeOffset_ = value;
return this;
}
/**
* optional uint32 store_offset = 12;
*/
public Builder clearStoreOffset() {
bitField0_ = (bitField0_ & ~0x00000800);
storeOffset_ = 0;
return this;
}
// optional bool load_column_families_on_demand = 13;
private boolean loadColumnFamiliesOnDemand_ ;
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public boolean hasLoadColumnFamiliesOnDemand() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public boolean getLoadColumnFamiliesOnDemand() {
return loadColumnFamiliesOnDemand_;
}
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public Builder setLoadColumnFamiliesOnDemand(boolean value) {
bitField0_ |= 0x00001000;
loadColumnFamiliesOnDemand_ = value;
return this;
}
/**
* optional bool load_column_families_on_demand = 13;
*
*
* DO NOT add defaults to load_column_families_on_demand.
*
*/
public Builder clearLoadColumnFamiliesOnDemand() {
bitField0_ = (bitField0_ & ~0x00001000);
loadColumnFamiliesOnDemand_ = false;
return this;
}
// optional bool small = 14;
private boolean small_ ;
/**
* optional bool small = 14;
*/
public boolean hasSmall() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bool small = 14;
*/
public boolean getSmall() {
return small_;
}
/**
* optional bool small = 14;
*/
public Builder setSmall(boolean value) {
bitField0_ |= 0x00002000;
small_ = value;
return this;
}
/**
* optional bool small = 14;
*/
public Builder clearSmall() {
bitField0_ = (bitField0_ & ~0x00002000);
small_ = false;
return this;
}
// optional bool reversed = 15 [default = false];
private boolean reversed_ ;
/**
* optional bool reversed = 15 [default = false];
*/
public boolean hasReversed() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool reversed = 15 [default = false];
*/
public boolean getReversed() {
return reversed_;
}
/**
* optional bool reversed = 15 [default = false];
*/
public Builder setReversed(boolean value) {
bitField0_ |= 0x00004000;
reversed_ = value;
return this;
}
/**
* optional bool reversed = 15 [default = false];
*/
public Builder clearReversed() {
bitField0_ = (bitField0_ & ~0x00004000);
reversed_ = false;
return this;
}
// optional .Consistency consistency = 16 [default = STRONG];
private org.hbase.async.generated.ClientPB.Consistency consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public org.hbase.async.generated.ClientPB.Consistency getConsistency() {
return consistency_;
}
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public Builder setConsistency(org.hbase.async.generated.ClientPB.Consistency value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
consistency_ = value;
return this;
}
/**
* optional .Consistency consistency = 16 [default = STRONG];
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00008000);
consistency_ = org.hbase.async.generated.ClientPB.Consistency.STRONG;
return this;
}
// optional uint32 caching = 17;
private int caching_ ;
/**
* optional uint32 caching = 17;
*/
public boolean hasCaching() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional uint32 caching = 17;
*/
public int getCaching() {
return caching_;
}
/**
* optional uint32 caching = 17;
*/
public Builder setCaching(int value) {
bitField0_ |= 0x00010000;
caching_ = value;
return this;
}
/**
* optional uint32 caching = 17;
*/
public Builder clearCaching() {
bitField0_ = (bitField0_ & ~0x00010000);
caching_ = 0;
return this;
}
// optional bool allow_partial_results = 18;
private boolean allowPartialResults_ ;
/**
* optional bool allow_partial_results = 18;
*/
public boolean hasAllowPartialResults() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional bool allow_partial_results = 18;
*/
public boolean getAllowPartialResults() {
return allowPartialResults_;
}
/**
* optional bool allow_partial_results = 18;
*/
public Builder setAllowPartialResults(boolean value) {
bitField0_ |= 0x00020000;
allowPartialResults_ = value;
return this;
}
/**
* optional bool allow_partial_results = 18;
*/
public Builder clearAllowPartialResults() {
bitField0_ = (bitField0_ & ~0x00020000);
allowPartialResults_ = false;
return this;
}
// repeated .ColumnFamilyTimeRange cf_time_range = 19;
private java.util.List cfTimeRange_ =
java.util.Collections.emptyList();
private void ensureCfTimeRangeIsMutable() {
if (!((bitField0_ & 0x00040000) == 0x00040000)) {
cfTimeRange_ = new java.util.ArrayList(cfTimeRange_);
bitField0_ |= 0x00040000;
}
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public java.util.List getCfTimeRangeList() {
return java.util.Collections.unmodifiableList(cfTimeRange_);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public int getCfTimeRangeCount() {
return cfTimeRange_.size();
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange getCfTimeRange(int index) {
return cfTimeRange_.get(index);
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder setCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.set(index, value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder setCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.set(index, builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder addCfTimeRange(org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder addCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange value) {
if (value == null) {
throw new NullPointerException();
}
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(index, value);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder addCfTimeRange(
org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder addCfTimeRange(
int index, org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange.Builder builderForValue) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.add(index, builderForValue.build());
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder addAllCfTimeRange(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.ColumnFamilyTimeRange> values) {
ensureCfTimeRangeIsMutable();
super.addAll(values, cfTimeRange_);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder clearCfTimeRange() {
cfTimeRange_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00040000);
return this;
}
/**
* repeated .ColumnFamilyTimeRange cf_time_range = 19;
*/
public Builder removeCfTimeRange(int index) {
ensureCfTimeRangeIsMutable();
cfTimeRange_.remove(index);
return this;
}
// @@protoc_insertion_point(builder_scope:Scan)
}
static {
defaultInstance = new Scan(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Scan)
}
public interface ScanRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional .RegionSpecifier region = 1;
/**
* optional .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* optional .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// optional .Scan scan = 2;
/**
* optional .Scan scan = 2;
*/
boolean hasScan();
/**
* optional .Scan scan = 2;
*/
org.hbase.async.generated.ClientPB.Scan getScan();
// optional uint64 scanner_id = 3;
/**
* optional uint64 scanner_id = 3;
*/
boolean hasScannerId();
/**
* optional uint64 scanner_id = 3;
*/
long getScannerId();
// optional uint32 number_of_rows = 4;
/**
* optional uint32 number_of_rows = 4;
*/
boolean hasNumberOfRows();
/**
* optional uint32 number_of_rows = 4;
*/
int getNumberOfRows();
// optional bool close_scanner = 5;
/**
* optional bool close_scanner = 5;
*/
boolean hasCloseScanner();
/**
* optional bool close_scanner = 5;
*/
boolean getCloseScanner();
// optional uint64 next_call_seq = 6;
/**
* optional uint64 next_call_seq = 6;
*/
boolean hasNextCallSeq();
/**
* optional uint64 next_call_seq = 6;
*/
long getNextCallSeq();
// optional bool client_handles_partials = 7;
/**
* optional bool client_handles_partials = 7;
*/
boolean hasClientHandlesPartials();
/**
* optional bool client_handles_partials = 7;
*/
boolean getClientHandlesPartials();
// optional bool client_handles_heartbeats = 8;
/**
* optional bool client_handles_heartbeats = 8;
*/
boolean hasClientHandlesHeartbeats();
/**
* optional bool client_handles_heartbeats = 8;
*/
boolean getClientHandlesHeartbeats();
// optional bool track_scan_metrics = 9;
/**
* optional bool track_scan_metrics = 9;
*/
boolean hasTrackScanMetrics();
/**
* optional bool track_scan_metrics = 9;
*/
boolean getTrackScanMetrics();
// optional bool renew = 10 [default = false];
/**
* optional bool renew = 10 [default = false];
*/
boolean hasRenew();
/**
* optional bool renew = 10 [default = false];
*/
boolean getRenew();
}
/**
* Protobuf type {@code ScanRequest}
*
*
**
* A scan request. Initially, it should specify a scan. Later on, you
* can use the scanner id returned to fetch result batches with a different
* scan request.
*
* The scanner will remain open if there are more results, and it's not
* asked to be closed explicitly.
*
* You can fetch the results and ask the scanner to be closed to save
* a trip if you are not interested in remaining results.
*
*/
public static final class ScanRequest extends
com.google.protobuf.GeneratedMessageLite
implements ScanRequestOrBuilder {
// Use ScanRequest.newBuilder() to construct.
private ScanRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private ScanRequest(boolean noInit) {}
private static final ScanRequest defaultInstance;
public static ScanRequest getDefaultInstance() {
return defaultInstance;
}
public ScanRequest getDefaultInstanceForType() {
return defaultInstance;
}
private ScanRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.hbase.async.generated.ClientPB.Scan.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = scan_.toBuilder();
}
scan_ = input.readMessage(org.hbase.async.generated.ClientPB.Scan.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(scan_);
scan_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 24: {
bitField0_ |= 0x00000004;
scannerId_ = input.readUInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
numberOfRows_ = input.readUInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
closeScanner_ = input.readBool();
break;
}
case 48: {
bitField0_ |= 0x00000020;
nextCallSeq_ = input.readUInt64();
break;
}
case 56: {
bitField0_ |= 0x00000040;
clientHandlesPartials_ = input.readBool();
break;
}
case 64: {
bitField0_ |= 0x00000080;
clientHandlesHeartbeats_ = input.readBool();
break;
}
case 72: {
bitField0_ |= 0x00000100;
trackScanMetrics_ = input.readBool();
break;
}
case 80: {
bitField0_ |= 0x00000200;
renew_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ScanRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScanRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* optional .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// optional .Scan scan = 2;
public static final int SCAN_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.Scan scan_;
/**
* optional .Scan scan = 2;
*/
public boolean hasScan() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Scan scan = 2;
*/
public org.hbase.async.generated.ClientPB.Scan getScan() {
return scan_;
}
// optional uint64 scanner_id = 3;
public static final int SCANNER_ID_FIELD_NUMBER = 3;
private long scannerId_;
/**
* optional uint64 scanner_id = 3;
*/
public boolean hasScannerId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 scanner_id = 3;
*/
public long getScannerId() {
return scannerId_;
}
// optional uint32 number_of_rows = 4;
public static final int NUMBER_OF_ROWS_FIELD_NUMBER = 4;
private int numberOfRows_;
/**
* optional uint32 number_of_rows = 4;
*/
public boolean hasNumberOfRows() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 number_of_rows = 4;
*/
public int getNumberOfRows() {
return numberOfRows_;
}
// optional bool close_scanner = 5;
public static final int CLOSE_SCANNER_FIELD_NUMBER = 5;
private boolean closeScanner_;
/**
* optional bool close_scanner = 5;
*/
public boolean hasCloseScanner() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool close_scanner = 5;
*/
public boolean getCloseScanner() {
return closeScanner_;
}
// optional uint64 next_call_seq = 6;
public static final int NEXT_CALL_SEQ_FIELD_NUMBER = 6;
private long nextCallSeq_;
/**
* optional uint64 next_call_seq = 6;
*/
public boolean hasNextCallSeq() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint64 next_call_seq = 6;
*/
public long getNextCallSeq() {
return nextCallSeq_;
}
// optional bool client_handles_partials = 7;
public static final int CLIENT_HANDLES_PARTIALS_FIELD_NUMBER = 7;
private boolean clientHandlesPartials_;
/**
* optional bool client_handles_partials = 7;
*/
public boolean hasClientHandlesPartials() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool client_handles_partials = 7;
*/
public boolean getClientHandlesPartials() {
return clientHandlesPartials_;
}
// optional bool client_handles_heartbeats = 8;
public static final int CLIENT_HANDLES_HEARTBEATS_FIELD_NUMBER = 8;
private boolean clientHandlesHeartbeats_;
/**
* optional bool client_handles_heartbeats = 8;
*/
public boolean hasClientHandlesHeartbeats() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool client_handles_heartbeats = 8;
*/
public boolean getClientHandlesHeartbeats() {
return clientHandlesHeartbeats_;
}
// optional bool track_scan_metrics = 9;
public static final int TRACK_SCAN_METRICS_FIELD_NUMBER = 9;
private boolean trackScanMetrics_;
/**
* optional bool track_scan_metrics = 9;
*/
public boolean hasTrackScanMetrics() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool track_scan_metrics = 9;
*/
public boolean getTrackScanMetrics() {
return trackScanMetrics_;
}
// optional bool renew = 10 [default = false];
public static final int RENEW_FIELD_NUMBER = 10;
private boolean renew_;
/**
* optional bool renew = 10 [default = false];
*/
public boolean hasRenew() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool renew = 10 [default = false];
*/
public boolean getRenew() {
return renew_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
scan_ = org.hbase.async.generated.ClientPB.Scan.getDefaultInstance();
scannerId_ = 0L;
numberOfRows_ = 0;
closeScanner_ = false;
nextCallSeq_ = 0L;
clientHandlesPartials_ = false;
clientHandlesHeartbeats_ = false;
trackScanMetrics_ = false;
renew_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasRegion()) {
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasScan()) {
if (!getScan().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, scan_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, scannerId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(4, numberOfRows_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, closeScanner_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeUInt64(6, nextCallSeq_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(7, clientHandlesPartials_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBool(8, clientHandlesHeartbeats_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, trackScanMetrics_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBool(10, renew_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, scan_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, scannerId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, numberOfRows_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, closeScanner_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, nextCallSeq_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, clientHandlesPartials_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, clientHandlesHeartbeats_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, trackScanMetrics_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, renew_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.ScanRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code ScanRequest}
*
*
**
* A scan request. Initially, it should specify a scan. Later on, you
* can use the scanner id returned to fetch result batches with a different
* scan request.
*
* The scanner will remain open if there are more results, and it's not
* asked to be closed explicitly.
*
* You can fetch the results and ask the scanner to be closed to save
* a trip if you are not interested in remaining results.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.ScanRequest, Builder>
implements org.hbase.async.generated.ClientPB.ScanRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.ScanRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
scan_ = org.hbase.async.generated.ClientPB.Scan.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
scannerId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
numberOfRows_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
closeScanner_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
nextCallSeq_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
clientHandlesPartials_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
clientHandlesHeartbeats_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
trackScanMetrics_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
renew_ = false;
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.ScanRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.ScanRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.ScanRequest build() {
org.hbase.async.generated.ClientPB.ScanRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.ScanRequest buildPartial() {
org.hbase.async.generated.ClientPB.ScanRequest result = new org.hbase.async.generated.ClientPB.ScanRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.scan_ = scan_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.scannerId_ = scannerId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.numberOfRows_ = numberOfRows_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.closeScanner_ = closeScanner_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.nextCallSeq_ = nextCallSeq_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.clientHandlesPartials_ = clientHandlesPartials_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.clientHandlesHeartbeats_ = clientHandlesHeartbeats_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.trackScanMetrics_ = trackScanMetrics_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.renew_ = renew_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.ScanRequest other) {
if (other == org.hbase.async.generated.ClientPB.ScanRequest.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasScan()) {
mergeScan(other.getScan());
}
if (other.hasScannerId()) {
setScannerId(other.getScannerId());
}
if (other.hasNumberOfRows()) {
setNumberOfRows(other.getNumberOfRows());
}
if (other.hasCloseScanner()) {
setCloseScanner(other.getCloseScanner());
}
if (other.hasNextCallSeq()) {
setNextCallSeq(other.getNextCallSeq());
}
if (other.hasClientHandlesPartials()) {
setClientHandlesPartials(other.getClientHandlesPartials());
}
if (other.hasClientHandlesHeartbeats()) {
setClientHandlesHeartbeats(other.getClientHandlesHeartbeats());
}
if (other.hasTrackScanMetrics()) {
setTrackScanMetrics(other.getTrackScanMetrics());
}
if (other.hasRenew()) {
setRenew(other.getRenew());
}
return this;
}
public final boolean isInitialized() {
if (hasRegion()) {
if (!getRegion().isInitialized()) {
return false;
}
}
if (hasScan()) {
if (!getScan().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.ScanRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.ScanRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* optional .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* optional .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// optional .Scan scan = 2;
private org.hbase.async.generated.ClientPB.Scan scan_ = org.hbase.async.generated.ClientPB.Scan.getDefaultInstance();
/**
* optional .Scan scan = 2;
*/
public boolean hasScan() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Scan scan = 2;
*/
public org.hbase.async.generated.ClientPB.Scan getScan() {
return scan_;
}
/**
* optional .Scan scan = 2;
*/
public Builder setScan(org.hbase.async.generated.ClientPB.Scan value) {
if (value == null) {
throw new NullPointerException();
}
scan_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Scan scan = 2;
*/
public Builder setScan(
org.hbase.async.generated.ClientPB.Scan.Builder builderForValue) {
scan_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Scan scan = 2;
*/
public Builder mergeScan(org.hbase.async.generated.ClientPB.Scan value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
scan_ != org.hbase.async.generated.ClientPB.Scan.getDefaultInstance()) {
scan_ =
org.hbase.async.generated.ClientPB.Scan.newBuilder(scan_).mergeFrom(value).buildPartial();
} else {
scan_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Scan scan = 2;
*/
public Builder clearScan() {
scan_ = org.hbase.async.generated.ClientPB.Scan.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// optional uint64 scanner_id = 3;
private long scannerId_ ;
/**
* optional uint64 scanner_id = 3;
*/
public boolean hasScannerId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 scanner_id = 3;
*/
public long getScannerId() {
return scannerId_;
}
/**
* optional uint64 scanner_id = 3;
*/
public Builder setScannerId(long value) {
bitField0_ |= 0x00000004;
scannerId_ = value;
return this;
}
/**
* optional uint64 scanner_id = 3;
*/
public Builder clearScannerId() {
bitField0_ = (bitField0_ & ~0x00000004);
scannerId_ = 0L;
return this;
}
// optional uint32 number_of_rows = 4;
private int numberOfRows_ ;
/**
* optional uint32 number_of_rows = 4;
*/
public boolean hasNumberOfRows() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 number_of_rows = 4;
*/
public int getNumberOfRows() {
return numberOfRows_;
}
/**
* optional uint32 number_of_rows = 4;
*/
public Builder setNumberOfRows(int value) {
bitField0_ |= 0x00000008;
numberOfRows_ = value;
return this;
}
/**
* optional uint32 number_of_rows = 4;
*/
public Builder clearNumberOfRows() {
bitField0_ = (bitField0_ & ~0x00000008);
numberOfRows_ = 0;
return this;
}
// optional bool close_scanner = 5;
private boolean closeScanner_ ;
/**
* optional bool close_scanner = 5;
*/
public boolean hasCloseScanner() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool close_scanner = 5;
*/
public boolean getCloseScanner() {
return closeScanner_;
}
/**
* optional bool close_scanner = 5;
*/
public Builder setCloseScanner(boolean value) {
bitField0_ |= 0x00000010;
closeScanner_ = value;
return this;
}
/**
* optional bool close_scanner = 5;
*/
public Builder clearCloseScanner() {
bitField0_ = (bitField0_ & ~0x00000010);
closeScanner_ = false;
return this;
}
// optional uint64 next_call_seq = 6;
private long nextCallSeq_ ;
/**
* optional uint64 next_call_seq = 6;
*/
public boolean hasNextCallSeq() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint64 next_call_seq = 6;
*/
public long getNextCallSeq() {
return nextCallSeq_;
}
/**
* optional uint64 next_call_seq = 6;
*/
public Builder setNextCallSeq(long value) {
bitField0_ |= 0x00000020;
nextCallSeq_ = value;
return this;
}
/**
* optional uint64 next_call_seq = 6;
*/
public Builder clearNextCallSeq() {
bitField0_ = (bitField0_ & ~0x00000020);
nextCallSeq_ = 0L;
return this;
}
// optional bool client_handles_partials = 7;
private boolean clientHandlesPartials_ ;
/**
* optional bool client_handles_partials = 7;
*/
public boolean hasClientHandlesPartials() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool client_handles_partials = 7;
*/
public boolean getClientHandlesPartials() {
return clientHandlesPartials_;
}
/**
* optional bool client_handles_partials = 7;
*/
public Builder setClientHandlesPartials(boolean value) {
bitField0_ |= 0x00000040;
clientHandlesPartials_ = value;
return this;
}
/**
* optional bool client_handles_partials = 7;
*/
public Builder clearClientHandlesPartials() {
bitField0_ = (bitField0_ & ~0x00000040);
clientHandlesPartials_ = false;
return this;
}
// optional bool client_handles_heartbeats = 8;
private boolean clientHandlesHeartbeats_ ;
/**
* optional bool client_handles_heartbeats = 8;
*/
public boolean hasClientHandlesHeartbeats() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool client_handles_heartbeats = 8;
*/
public boolean getClientHandlesHeartbeats() {
return clientHandlesHeartbeats_;
}
/**
* optional bool client_handles_heartbeats = 8;
*/
public Builder setClientHandlesHeartbeats(boolean value) {
bitField0_ |= 0x00000080;
clientHandlesHeartbeats_ = value;
return this;
}
/**
* optional bool client_handles_heartbeats = 8;
*/
public Builder clearClientHandlesHeartbeats() {
bitField0_ = (bitField0_ & ~0x00000080);
clientHandlesHeartbeats_ = false;
return this;
}
// optional bool track_scan_metrics = 9;
private boolean trackScanMetrics_ ;
/**
* optional bool track_scan_metrics = 9;
*/
public boolean hasTrackScanMetrics() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool track_scan_metrics = 9;
*/
public boolean getTrackScanMetrics() {
return trackScanMetrics_;
}
/**
* optional bool track_scan_metrics = 9;
*/
public Builder setTrackScanMetrics(boolean value) {
bitField0_ |= 0x00000100;
trackScanMetrics_ = value;
return this;
}
/**
* optional bool track_scan_metrics = 9;
*/
public Builder clearTrackScanMetrics() {
bitField0_ = (bitField0_ & ~0x00000100);
trackScanMetrics_ = false;
return this;
}
// optional bool renew = 10 [default = false];
private boolean renew_ ;
/**
* optional bool renew = 10 [default = false];
*/
public boolean hasRenew() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool renew = 10 [default = false];
*/
public boolean getRenew() {
return renew_;
}
/**
* optional bool renew = 10 [default = false];
*/
public Builder setRenew(boolean value) {
bitField0_ |= 0x00000200;
renew_ = value;
return this;
}
/**
* optional bool renew = 10 [default = false];
*/
public Builder clearRenew() {
bitField0_ = (bitField0_ & ~0x00000200);
renew_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:ScanRequest)
}
static {
defaultInstance = new ScanRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ScanRequest)
}
public interface ScanResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated uint32 cells_per_result = 1;
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
java.util.List getCellsPerResultList();
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
int getCellsPerResultCount();
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
int getCellsPerResult(int index);
// optional uint64 scanner_id = 2;
/**
* optional uint64 scanner_id = 2;
*/
boolean hasScannerId();
/**
* optional uint64 scanner_id = 2;
*/
long getScannerId();
// optional bool more_results = 3;
/**
* optional bool more_results = 3;
*/
boolean hasMoreResults();
/**
* optional bool more_results = 3;
*/
boolean getMoreResults();
// optional uint32 ttl = 4;
/**
* optional uint32 ttl = 4;
*/
boolean hasTtl();
/**
* optional uint32 ttl = 4;
*/
int getTtl();
// repeated .Result results = 5;
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
java.util.List
getResultsList();
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
org.hbase.async.generated.ClientPB.Result getResults(int index);
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
int getResultsCount();
// optional bool stale = 6;
/**
* optional bool stale = 6;
*/
boolean hasStale();
/**
* optional bool stale = 6;
*/
boolean getStale();
// repeated bool partial_flag_per_result = 7;
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
java.util.List getPartialFlagPerResultList();
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
int getPartialFlagPerResultCount();
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
boolean getPartialFlagPerResult(int index);
// optional bool more_results_in_region = 8;
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
boolean hasMoreResultsInRegion();
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
boolean getMoreResultsInRegion();
// optional bool heartbeat_message = 9;
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
boolean hasHeartbeatMessage();
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
boolean getHeartbeatMessage();
// optional .ScanMetrics scan_metrics = 10;
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
boolean hasScanMetrics();
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
org.hbase.async.generated.MapReducePB.ScanMetrics getScanMetrics();
}
/**
* Protobuf type {@code ScanResponse}
*
*
**
* The scan response. If there are no more results, more_results will
* be false. If it is not specified, it means there are more.
*
*/
public static final class ScanResponse extends
com.google.protobuf.GeneratedMessageLite
implements ScanResponseOrBuilder {
// Use ScanResponse.newBuilder() to construct.
private ScanResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private ScanResponse(boolean noInit) {}
private static final ScanResponse defaultInstance;
public static ScanResponse getDefaultInstance() {
return defaultInstance;
}
public ScanResponse getDefaultInstanceForType() {
return defaultInstance;
}
private ScanResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cellsPerResult_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
cellsPerResult_.add(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
cellsPerResult_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
cellsPerResult_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 16: {
bitField0_ |= 0x00000001;
scannerId_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000002;
moreResults_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000004;
ttl_ = input.readUInt32();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
results_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
results_.add(input.readMessage(org.hbase.async.generated.ClientPB.Result.PARSER, extensionRegistry));
break;
}
case 48: {
bitField0_ |= 0x00000008;
stale_ = input.readBool();
break;
}
case 56: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
partialFlagPerResult_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
partialFlagPerResult_.add(input.readBool());
break;
}
case 58: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040) && input.getBytesUntilLimit() > 0) {
partialFlagPerResult_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
while (input.getBytesUntilLimit() > 0) {
partialFlagPerResult_.add(input.readBool());
}
input.popLimit(limit);
break;
}
case 64: {
bitField0_ |= 0x00000010;
moreResultsInRegion_ = input.readBool();
break;
}
case 72: {
bitField0_ |= 0x00000020;
heartbeatMessage_ = input.readBool();
break;
}
case 82: {
org.hbase.async.generated.MapReducePB.ScanMetrics.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = scanMetrics_.toBuilder();
}
scanMetrics_ = input.readMessage(org.hbase.async.generated.MapReducePB.ScanMetrics.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(scanMetrics_);
scanMetrics_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cellsPerResult_ = java.util.Collections.unmodifiableList(cellsPerResult_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
results_ = java.util.Collections.unmodifiableList(results_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
partialFlagPerResult_ = java.util.Collections.unmodifiableList(partialFlagPerResult_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ScanResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScanResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated uint32 cells_per_result = 1;
public static final int CELLS_PER_RESULT_FIELD_NUMBER = 1;
private java.util.List cellsPerResult_;
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public java.util.List
getCellsPerResultList() {
return cellsPerResult_;
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public int getCellsPerResultCount() {
return cellsPerResult_.size();
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public int getCellsPerResult(int index) {
return cellsPerResult_.get(index);
}
// optional uint64 scanner_id = 2;
public static final int SCANNER_ID_FIELD_NUMBER = 2;
private long scannerId_;
/**
* optional uint64 scanner_id = 2;
*/
public boolean hasScannerId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint64 scanner_id = 2;
*/
public long getScannerId() {
return scannerId_;
}
// optional bool more_results = 3;
public static final int MORE_RESULTS_FIELD_NUMBER = 3;
private boolean moreResults_;
/**
* optional bool more_results = 3;
*/
public boolean hasMoreResults() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool more_results = 3;
*/
public boolean getMoreResults() {
return moreResults_;
}
// optional uint32 ttl = 4;
public static final int TTL_FIELD_NUMBER = 4;
private int ttl_;
/**
* optional uint32 ttl = 4;
*/
public boolean hasTtl() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 ttl = 4;
*/
public int getTtl() {
return ttl_;
}
// repeated .Result results = 5;
public static final int RESULTS_FIELD_NUMBER = 5;
private java.util.List results_;
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public java.util.List getResultsList() {
return results_;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public java.util.List extends org.hbase.async.generated.ClientPB.ResultOrBuilder>
getResultsOrBuilderList() {
return results_;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public int getResultsCount() {
return results_.size();
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public org.hbase.async.generated.ClientPB.Result getResults(int index) {
return results_.get(index);
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public org.hbase.async.generated.ClientPB.ResultOrBuilder getResultsOrBuilder(
int index) {
return results_.get(index);
}
// optional bool stale = 6;
public static final int STALE_FIELD_NUMBER = 6;
private boolean stale_;
/**
* optional bool stale = 6;
*/
public boolean hasStale() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool stale = 6;
*/
public boolean getStale() {
return stale_;
}
// repeated bool partial_flag_per_result = 7;
public static final int PARTIAL_FLAG_PER_RESULT_FIELD_NUMBER = 7;
private java.util.List partialFlagPerResult_;
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public java.util.List
getPartialFlagPerResultList() {
return partialFlagPerResult_;
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public int getPartialFlagPerResultCount() {
return partialFlagPerResult_.size();
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public boolean getPartialFlagPerResult(int index) {
return partialFlagPerResult_.get(index);
}
// optional bool more_results_in_region = 8;
public static final int MORE_RESULTS_IN_REGION_FIELD_NUMBER = 8;
private boolean moreResultsInRegion_;
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public boolean hasMoreResultsInRegion() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public boolean getMoreResultsInRegion() {
return moreResultsInRegion_;
}
// optional bool heartbeat_message = 9;
public static final int HEARTBEAT_MESSAGE_FIELD_NUMBER = 9;
private boolean heartbeatMessage_;
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public boolean hasHeartbeatMessage() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public boolean getHeartbeatMessage() {
return heartbeatMessage_;
}
// optional .ScanMetrics scan_metrics = 10;
public static final int SCAN_METRICS_FIELD_NUMBER = 10;
private org.hbase.async.generated.MapReducePB.ScanMetrics scanMetrics_;
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public boolean hasScanMetrics() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public org.hbase.async.generated.MapReducePB.ScanMetrics getScanMetrics() {
return scanMetrics_;
}
private void initFields() {
cellsPerResult_ = java.util.Collections.emptyList();
scannerId_ = 0L;
moreResults_ = false;
ttl_ = 0;
results_ = java.util.Collections.emptyList();
stale_ = false;
partialFlagPerResult_ = java.util.Collections.emptyList();
moreResultsInRegion_ = false;
heartbeatMessage_ = false;
scanMetrics_ = org.hbase.async.generated.MapReducePB.ScanMetrics.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < cellsPerResult_.size(); i++) {
output.writeUInt32(1, cellsPerResult_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(2, scannerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(3, moreResults_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(4, ttl_);
}
for (int i = 0; i < results_.size(); i++) {
output.writeMessage(5, results_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(6, stale_);
}
for (int i = 0; i < partialFlagPerResult_.size(); i++) {
output.writeBool(7, partialFlagPerResult_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(8, moreResultsInRegion_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(9, heartbeatMessage_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(10, scanMetrics_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < cellsPerResult_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(cellsPerResult_.get(i));
}
size += dataSize;
size += 1 * getCellsPerResultList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, scannerId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, moreResults_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, ttl_);
}
for (int i = 0; i < results_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, results_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, stale_);
}
{
int dataSize = 0;
dataSize = 1 * getPartialFlagPerResultList().size();
size += dataSize;
size += 1 * getPartialFlagPerResultList().size();
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, moreResultsInRegion_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, heartbeatMessage_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, scanMetrics_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ScanResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.ScanResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code ScanResponse}
*
*
**
* The scan response. If there are no more results, more_results will
* be false. If it is not specified, it means there are more.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.ScanResponse, Builder>
implements org.hbase.async.generated.ClientPB.ScanResponseOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.ScanResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
cellsPerResult_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
scannerId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
moreResults_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
ttl_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
results_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
stale_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
partialFlagPerResult_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
moreResultsInRegion_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
heartbeatMessage_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
scanMetrics_ = org.hbase.async.generated.MapReducePB.ScanMetrics.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.ScanResponse getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.ScanResponse.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.ScanResponse build() {
org.hbase.async.generated.ClientPB.ScanResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.ScanResponse buildPartial() {
org.hbase.async.generated.ClientPB.ScanResponse result = new org.hbase.async.generated.ClientPB.ScanResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
cellsPerResult_ = java.util.Collections.unmodifiableList(cellsPerResult_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.cellsPerResult_ = cellsPerResult_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.scannerId_ = scannerId_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.moreResults_ = moreResults_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.ttl_ = ttl_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
results_ = java.util.Collections.unmodifiableList(results_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.results_ = results_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.stale_ = stale_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
partialFlagPerResult_ = java.util.Collections.unmodifiableList(partialFlagPerResult_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.partialFlagPerResult_ = partialFlagPerResult_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000010;
}
result.moreResultsInRegion_ = moreResultsInRegion_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000020;
}
result.heartbeatMessage_ = heartbeatMessage_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000040;
}
result.scanMetrics_ = scanMetrics_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.ScanResponse other) {
if (other == org.hbase.async.generated.ClientPB.ScanResponse.getDefaultInstance()) return this;
if (!other.cellsPerResult_.isEmpty()) {
if (cellsPerResult_.isEmpty()) {
cellsPerResult_ = other.cellsPerResult_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCellsPerResultIsMutable();
cellsPerResult_.addAll(other.cellsPerResult_);
}
}
if (other.hasScannerId()) {
setScannerId(other.getScannerId());
}
if (other.hasMoreResults()) {
setMoreResults(other.getMoreResults());
}
if (other.hasTtl()) {
setTtl(other.getTtl());
}
if (!other.results_.isEmpty()) {
if (results_.isEmpty()) {
results_ = other.results_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureResultsIsMutable();
results_.addAll(other.results_);
}
}
if (other.hasStale()) {
setStale(other.getStale());
}
if (!other.partialFlagPerResult_.isEmpty()) {
if (partialFlagPerResult_.isEmpty()) {
partialFlagPerResult_ = other.partialFlagPerResult_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensurePartialFlagPerResultIsMutable();
partialFlagPerResult_.addAll(other.partialFlagPerResult_);
}
}
if (other.hasMoreResultsInRegion()) {
setMoreResultsInRegion(other.getMoreResultsInRegion());
}
if (other.hasHeartbeatMessage()) {
setHeartbeatMessage(other.getHeartbeatMessage());
}
if (other.hasScanMetrics()) {
mergeScanMetrics(other.getScanMetrics());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.ScanResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.ScanResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated uint32 cells_per_result = 1;
private java.util.List cellsPerResult_ = java.util.Collections.emptyList();
private void ensureCellsPerResultIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
cellsPerResult_ = new java.util.ArrayList(cellsPerResult_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public java.util.List
getCellsPerResultList() {
return java.util.Collections.unmodifiableList(cellsPerResult_);
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public int getCellsPerResultCount() {
return cellsPerResult_.size();
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public int getCellsPerResult(int index) {
return cellsPerResult_.get(index);
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public Builder setCellsPerResult(
int index, int value) {
ensureCellsPerResultIsMutable();
cellsPerResult_.set(index, value);
return this;
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public Builder addCellsPerResult(int value) {
ensureCellsPerResultIsMutable();
cellsPerResult_.add(value);
return this;
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public Builder addAllCellsPerResult(
java.lang.Iterable extends java.lang.Integer> values) {
ensureCellsPerResultIsMutable();
super.addAll(values, cellsPerResult_);
return this;
}
/**
* repeated uint32 cells_per_result = 1;
*
*
* This field is filled in if we are doing cellblocks. A cellblock is made up
* of all Cells serialized out as one cellblock BUT responses from a server
* have their Cells grouped by Result. So we can reconstitute the
* Results on the client-side, this field is a list of counts of Cells
* in each Result that makes up the response. For example, if this field
* has 3, 3, 3 in it, then we know that on the client, we are to make
* three Results each of three Cells each.
*
*/
public Builder clearCellsPerResult() {
cellsPerResult_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// optional uint64 scanner_id = 2;
private long scannerId_ ;
/**
* optional uint64 scanner_id = 2;
*/
public boolean hasScannerId() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 scanner_id = 2;
*/
public long getScannerId() {
return scannerId_;
}
/**
* optional uint64 scanner_id = 2;
*/
public Builder setScannerId(long value) {
bitField0_ |= 0x00000002;
scannerId_ = value;
return this;
}
/**
* optional uint64 scanner_id = 2;
*/
public Builder clearScannerId() {
bitField0_ = (bitField0_ & ~0x00000002);
scannerId_ = 0L;
return this;
}
// optional bool more_results = 3;
private boolean moreResults_ ;
/**
* optional bool more_results = 3;
*/
public boolean hasMoreResults() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool more_results = 3;
*/
public boolean getMoreResults() {
return moreResults_;
}
/**
* optional bool more_results = 3;
*/
public Builder setMoreResults(boolean value) {
bitField0_ |= 0x00000004;
moreResults_ = value;
return this;
}
/**
* optional bool more_results = 3;
*/
public Builder clearMoreResults() {
bitField0_ = (bitField0_ & ~0x00000004);
moreResults_ = false;
return this;
}
// optional uint32 ttl = 4;
private int ttl_ ;
/**
* optional uint32 ttl = 4;
*/
public boolean hasTtl() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 ttl = 4;
*/
public int getTtl() {
return ttl_;
}
/**
* optional uint32 ttl = 4;
*/
public Builder setTtl(int value) {
bitField0_ |= 0x00000008;
ttl_ = value;
return this;
}
/**
* optional uint32 ttl = 4;
*/
public Builder clearTtl() {
bitField0_ = (bitField0_ & ~0x00000008);
ttl_ = 0;
return this;
}
// repeated .Result results = 5;
private java.util.List results_ =
java.util.Collections.emptyList();
private void ensureResultsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
results_ = new java.util.ArrayList(results_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public java.util.List getResultsList() {
return java.util.Collections.unmodifiableList(results_);
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public int getResultsCount() {
return results_.size();
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public org.hbase.async.generated.ClientPB.Result getResults(int index) {
return results_.get(index);
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder setResults(
int index, org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultsIsMutable();
results_.set(index, value);
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder setResults(
int index, org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
ensureResultsIsMutable();
results_.set(index, builderForValue.build());
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder addResults(org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultsIsMutable();
results_.add(value);
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder addResults(
int index, org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultsIsMutable();
results_.add(index, value);
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder addResults(
org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
ensureResultsIsMutable();
results_.add(builderForValue.build());
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder addResults(
int index, org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
ensureResultsIsMutable();
results_.add(index, builderForValue.build());
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder addAllResults(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.Result> values) {
ensureResultsIsMutable();
super.addAll(values, results_);
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder clearResults() {
results_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* repeated .Result results = 5;
*
*
* If cells are not carried in an accompanying cellblock, then they are pb'd here.
* This field is mutually exclusive with cells_per_result (since the Cells will
* be inside the pb'd Result)
*
*/
public Builder removeResults(int index) {
ensureResultsIsMutable();
results_.remove(index);
return this;
}
// optional bool stale = 6;
private boolean stale_ ;
/**
* optional bool stale = 6;
*/
public boolean hasStale() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool stale = 6;
*/
public boolean getStale() {
return stale_;
}
/**
* optional bool stale = 6;
*/
public Builder setStale(boolean value) {
bitField0_ |= 0x00000020;
stale_ = value;
return this;
}
/**
* optional bool stale = 6;
*/
public Builder clearStale() {
bitField0_ = (bitField0_ & ~0x00000020);
stale_ = false;
return this;
}
// repeated bool partial_flag_per_result = 7;
private java.util.List partialFlagPerResult_ = java.util.Collections.emptyList();
private void ensurePartialFlagPerResultIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
partialFlagPerResult_ = new java.util.ArrayList(partialFlagPerResult_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public java.util.List
getPartialFlagPerResultList() {
return java.util.Collections.unmodifiableList(partialFlagPerResult_);
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public int getPartialFlagPerResultCount() {
return partialFlagPerResult_.size();
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public boolean getPartialFlagPerResult(int index) {
return partialFlagPerResult_.get(index);
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public Builder setPartialFlagPerResult(
int index, boolean value) {
ensurePartialFlagPerResultIsMutable();
partialFlagPerResult_.set(index, value);
return this;
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public Builder addPartialFlagPerResult(boolean value) {
ensurePartialFlagPerResultIsMutable();
partialFlagPerResult_.add(value);
return this;
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public Builder addAllPartialFlagPerResult(
java.lang.Iterable extends java.lang.Boolean> values) {
ensurePartialFlagPerResultIsMutable();
super.addAll(values, partialFlagPerResult_);
return this;
}
/**
* repeated bool partial_flag_per_result = 7;
*
*
* This field is filled in if we are doing cellblocks. In the event that a row
* could not fit all of its cells into a single RPC chunk, the results will be
* returned as partials, and reconstructed into a complete result on the client
* side. This field is a list of flags indicating whether or not the result
* that the cells belong to is a partial result. For example, if this field
* has false, false, true in it, then we know that on the client side, we need to
* make another RPC request since the last result was only a partial.
*
*/
public Builder clearPartialFlagPerResult() {
partialFlagPerResult_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
// optional bool more_results_in_region = 8;
private boolean moreResultsInRegion_ ;
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public boolean hasMoreResultsInRegion() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public boolean getMoreResultsInRegion() {
return moreResultsInRegion_;
}
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public Builder setMoreResultsInRegion(boolean value) {
bitField0_ |= 0x00000080;
moreResultsInRegion_ = value;
return this;
}
/**
* optional bool more_results_in_region = 8;
*
*
* A server may choose to limit the number of results returned to the client for
* reasons such as the size in bytes or quantity of results accumulated. This field
* will true when more results exist in the current region.
*
*/
public Builder clearMoreResultsInRegion() {
bitField0_ = (bitField0_ & ~0x00000080);
moreResultsInRegion_ = false;
return this;
}
// optional bool heartbeat_message = 9;
private boolean heartbeatMessage_ ;
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public boolean hasHeartbeatMessage() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public boolean getHeartbeatMessage() {
return heartbeatMessage_;
}
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public Builder setHeartbeatMessage(boolean value) {
bitField0_ |= 0x00000100;
heartbeatMessage_ = value;
return this;
}
/**
* optional bool heartbeat_message = 9;
*
*
* This field is filled in if the server is sending back a heartbeat message.
* Heartbeat messages are sent back to the client to prevent the scanner from
* timing out. Seeing a heartbeat message communicates to the Client that the
* server would have continued to scan had the time limit not been reached.
*
*/
public Builder clearHeartbeatMessage() {
bitField0_ = (bitField0_ & ~0x00000100);
heartbeatMessage_ = false;
return this;
}
// optional .ScanMetrics scan_metrics = 10;
private org.hbase.async.generated.MapReducePB.ScanMetrics scanMetrics_ = org.hbase.async.generated.MapReducePB.ScanMetrics.getDefaultInstance();
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public boolean hasScanMetrics() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public org.hbase.async.generated.MapReducePB.ScanMetrics getScanMetrics() {
return scanMetrics_;
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public Builder setScanMetrics(org.hbase.async.generated.MapReducePB.ScanMetrics value) {
if (value == null) {
throw new NullPointerException();
}
scanMetrics_ = value;
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public Builder setScanMetrics(
org.hbase.async.generated.MapReducePB.ScanMetrics.Builder builderForValue) {
scanMetrics_ = builderForValue.build();
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public Builder mergeScanMetrics(org.hbase.async.generated.MapReducePB.ScanMetrics value) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
scanMetrics_ != org.hbase.async.generated.MapReducePB.ScanMetrics.getDefaultInstance()) {
scanMetrics_ =
org.hbase.async.generated.MapReducePB.ScanMetrics.newBuilder(scanMetrics_).mergeFrom(value).buildPartial();
} else {
scanMetrics_ = value;
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .ScanMetrics scan_metrics = 10;
*
*
* This field is filled in if the client has requested that scan metrics be tracked.
* The metrics tracked here are sent back to the client to be tracked together with
* the existing client side metrics.
*
*/
public Builder clearScanMetrics() {
scanMetrics_ = org.hbase.async.generated.MapReducePB.ScanMetrics.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
// @@protoc_insertion_point(builder_scope:ScanResponse)
}
static {
defaultInstance = new ScanResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ScanResponse)
}
public interface BulkLoadHFileRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
java.util.List
getFamilyPathList();
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath getFamilyPath(int index);
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
int getFamilyPathCount();
// optional bool assign_seq_num = 3;
/**
* optional bool assign_seq_num = 3;
*/
boolean hasAssignSeqNum();
/**
* optional bool assign_seq_num = 3;
*/
boolean getAssignSeqNum();
}
/**
* Protobuf type {@code BulkLoadHFileRequest}
*
*
**
* Atomically bulk load multiple HFiles (say from different column families)
* into an open region.
*
*/
public static final class BulkLoadHFileRequest extends
com.google.protobuf.GeneratedMessageLite
implements BulkLoadHFileRequestOrBuilder {
// Use BulkLoadHFileRequest.newBuilder() to construct.
private BulkLoadHFileRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private BulkLoadHFileRequest(boolean noInit) {}
private static final BulkLoadHFileRequest defaultInstance;
public static BulkLoadHFileRequest getDefaultInstance() {
return defaultInstance;
}
public BulkLoadHFileRequest getDefaultInstanceForType() {
return defaultInstance;
}
private BulkLoadHFileRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
familyPath_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
familyPath_.add(input.readMessage(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.PARSER, extensionRegistry));
break;
}
case 24: {
bitField0_ |= 0x00000002;
assignSeqNum_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
familyPath_ = java.util.Collections.unmodifiableList(familyPath_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public BulkLoadHFileRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BulkLoadHFileRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface FamilyPathOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes family = 1;
/**
* required bytes family = 1;
*/
boolean hasFamily();
/**
* required bytes family = 1;
*/
com.google.protobuf.ByteString getFamily();
// required string path = 2;
/**
* required string path = 2;
*/
boolean hasPath();
/**
* required string path = 2;
*/
java.lang.String getPath();
/**
* required string path = 2;
*/
com.google.protobuf.ByteString
getPathBytes();
}
/**
* Protobuf type {@code BulkLoadHFileRequest.FamilyPath}
*/
public static final class FamilyPath extends
com.google.protobuf.GeneratedMessageLite
implements FamilyPathOrBuilder {
// Use FamilyPath.newBuilder() to construct.
private FamilyPath(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private FamilyPath(boolean noInit) {}
private static final FamilyPath defaultInstance;
public static FamilyPath getDefaultInstance() {
return defaultInstance;
}
public FamilyPath getDefaultInstanceForType() {
return defaultInstance;
}
private FamilyPath(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
family_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
path_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public FamilyPath parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FamilyPath(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes family = 1;
public static final int FAMILY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString family_;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
// required string path = 2;
public static final int PATH_FIELD_NUMBER = 2;
private java.lang.Object path_;
/**
* required string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
* required string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
family_ = com.google.protobuf.ByteString.EMPTY;
path_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasFamily()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPath()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, family_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getPathBytes());
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, family_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getPathBytes());
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code BulkLoadHFileRequest.FamilyPath}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath, Builder>
implements org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPathOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
family_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath build() {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath buildPartial() {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath result = new org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.family_ = family_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.path_ = path_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath other) {
if (other == org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.getDefaultInstance()) return this;
if (other.hasFamily()) {
setFamily(other.getFamily());
}
if (other.hasPath()) {
bitField0_ |= 0x00000002;
path_ = other.path_;
}
return this;
}
public final boolean isInitialized() {
if (!hasFamily()) {
return false;
}
if (!hasPath()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes family = 1;
private com.google.protobuf.ByteString family_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes family = 1;
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes family = 1;
*/
public com.google.protobuf.ByteString getFamily() {
return family_;
}
/**
* required bytes family = 1;
*/
public Builder setFamily(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
family_ = value;
return this;
}
/**
* required bytes family = 1;
*/
public Builder clearFamily() {
bitField0_ = (bitField0_ & ~0x00000001);
family_ = getDefaultInstance().getFamily();
return this;
}
// required string path = 2;
private java.lang.Object path_ = "";
/**
* required string path = 2;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string path = 2;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string path = 2;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string path = 2;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
return this;
}
/**
* required string path = 2;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000002);
path_ = getDefaultInstance().getPath();
return this;
}
/**
* required string path = 2;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
path_ = value;
return this;
}
// @@protoc_insertion_point(builder_scope:BulkLoadHFileRequest.FamilyPath)
}
static {
defaultInstance = new FamilyPath(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:BulkLoadHFileRequest.FamilyPath)
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
public static final int FAMILY_PATH_FIELD_NUMBER = 2;
private java.util.List familyPath_;
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public java.util.List getFamilyPathList() {
return familyPath_;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPathOrBuilder>
getFamilyPathOrBuilderList() {
return familyPath_;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public int getFamilyPathCount() {
return familyPath_.size();
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath getFamilyPath(int index) {
return familyPath_.get(index);
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPathOrBuilder getFamilyPathOrBuilder(
int index) {
return familyPath_.get(index);
}
// optional bool assign_seq_num = 3;
public static final int ASSIGN_SEQ_NUM_FIELD_NUMBER = 3;
private boolean assignSeqNum_;
/**
* optional bool assign_seq_num = 3;
*/
public boolean hasAssignSeqNum() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool assign_seq_num = 3;
*/
public boolean getAssignSeqNum() {
return assignSeqNum_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
familyPath_ = java.util.Collections.emptyList();
assignSeqNum_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getFamilyPathCount(); i++) {
if (!getFamilyPath(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
for (int i = 0; i < familyPath_.size(); i++) {
output.writeMessage(2, familyPath_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(3, assignSeqNum_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
for (int i = 0; i < familyPath_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, familyPath_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, assignSeqNum_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code BulkLoadHFileRequest}
*
*
**
* Atomically bulk load multiple HFiles (say from different column families)
* into an open region.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest, Builder>
implements org.hbase.async.generated.ClientPB.BulkLoadHFileRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
familyPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
assignSeqNum_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest build() {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest buildPartial() {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest result = new org.hbase.async.generated.ClientPB.BulkLoadHFileRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
familyPath_ = java.util.Collections.unmodifiableList(familyPath_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.familyPath_ = familyPath_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.assignSeqNum_ = assignSeqNum_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest other) {
if (other == org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (!other.familyPath_.isEmpty()) {
if (familyPath_.isEmpty()) {
familyPath_ = other.familyPath_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureFamilyPathIsMutable();
familyPath_.addAll(other.familyPath_);
}
}
if (other.hasAssignSeqNum()) {
setAssignSeqNum(other.getAssignSeqNum());
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
for (int i = 0; i < getFamilyPathCount(); i++) {
if (!getFamilyPath(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.BulkLoadHFileRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
private java.util.List familyPath_ =
java.util.Collections.emptyList();
private void ensureFamilyPathIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
familyPath_ = new java.util.ArrayList(familyPath_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public java.util.List getFamilyPathList() {
return java.util.Collections.unmodifiableList(familyPath_);
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public int getFamilyPathCount() {
return familyPath_.size();
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath getFamilyPath(int index) {
return familyPath_.get(index);
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder setFamilyPath(
int index, org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath value) {
if (value == null) {
throw new NullPointerException();
}
ensureFamilyPathIsMutable();
familyPath_.set(index, value);
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder setFamilyPath(
int index, org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.Builder builderForValue) {
ensureFamilyPathIsMutable();
familyPath_.set(index, builderForValue.build());
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder addFamilyPath(org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath value) {
if (value == null) {
throw new NullPointerException();
}
ensureFamilyPathIsMutable();
familyPath_.add(value);
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder addFamilyPath(
int index, org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath value) {
if (value == null) {
throw new NullPointerException();
}
ensureFamilyPathIsMutable();
familyPath_.add(index, value);
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder addFamilyPath(
org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.Builder builderForValue) {
ensureFamilyPathIsMutable();
familyPath_.add(builderForValue.build());
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder addFamilyPath(
int index, org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath.Builder builderForValue) {
ensureFamilyPathIsMutable();
familyPath_.add(index, builderForValue.build());
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder addAllFamilyPath(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.BulkLoadHFileRequest.FamilyPath> values) {
ensureFamilyPathIsMutable();
super.addAll(values, familyPath_);
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder clearFamilyPath() {
familyPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* repeated .BulkLoadHFileRequest.FamilyPath family_path = 2;
*/
public Builder removeFamilyPath(int index) {
ensureFamilyPathIsMutable();
familyPath_.remove(index);
return this;
}
// optional bool assign_seq_num = 3;
private boolean assignSeqNum_ ;
/**
* optional bool assign_seq_num = 3;
*/
public boolean hasAssignSeqNum() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool assign_seq_num = 3;
*/
public boolean getAssignSeqNum() {
return assignSeqNum_;
}
/**
* optional bool assign_seq_num = 3;
*/
public Builder setAssignSeqNum(boolean value) {
bitField0_ |= 0x00000004;
assignSeqNum_ = value;
return this;
}
/**
* optional bool assign_seq_num = 3;
*/
public Builder clearAssignSeqNum() {
bitField0_ = (bitField0_ & ~0x00000004);
assignSeqNum_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:BulkLoadHFileRequest)
}
static {
defaultInstance = new BulkLoadHFileRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:BulkLoadHFileRequest)
}
public interface BulkLoadHFileResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bool loaded = 1;
/**
* required bool loaded = 1;
*/
boolean hasLoaded();
/**
* required bool loaded = 1;
*/
boolean getLoaded();
}
/**
* Protobuf type {@code BulkLoadHFileResponse}
*/
public static final class BulkLoadHFileResponse extends
com.google.protobuf.GeneratedMessageLite
implements BulkLoadHFileResponseOrBuilder {
// Use BulkLoadHFileResponse.newBuilder() to construct.
private BulkLoadHFileResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private BulkLoadHFileResponse(boolean noInit) {}
private static final BulkLoadHFileResponse defaultInstance;
public static BulkLoadHFileResponse getDefaultInstance() {
return defaultInstance;
}
public BulkLoadHFileResponse getDefaultInstanceForType() {
return defaultInstance;
}
private BulkLoadHFileResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
loaded_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public BulkLoadHFileResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BulkLoadHFileResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bool loaded = 1;
public static final int LOADED_FIELD_NUMBER = 1;
private boolean loaded_;
/**
* required bool loaded = 1;
*/
public boolean hasLoaded() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bool loaded = 1;
*/
public boolean getLoaded() {
return loaded_;
}
private void initFields() {
loaded_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasLoaded()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(1, loaded_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, loaded_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.BulkLoadHFileResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code BulkLoadHFileResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.BulkLoadHFileResponse, Builder>
implements org.hbase.async.generated.ClientPB.BulkLoadHFileResponseOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.BulkLoadHFileResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
loaded_ = false;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileResponse getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.BulkLoadHFileResponse.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileResponse build() {
org.hbase.async.generated.ClientPB.BulkLoadHFileResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.BulkLoadHFileResponse buildPartial() {
org.hbase.async.generated.ClientPB.BulkLoadHFileResponse result = new org.hbase.async.generated.ClientPB.BulkLoadHFileResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.loaded_ = loaded_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.BulkLoadHFileResponse other) {
if (other == org.hbase.async.generated.ClientPB.BulkLoadHFileResponse.getDefaultInstance()) return this;
if (other.hasLoaded()) {
setLoaded(other.getLoaded());
}
return this;
}
public final boolean isInitialized() {
if (!hasLoaded()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.BulkLoadHFileResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.BulkLoadHFileResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bool loaded = 1;
private boolean loaded_ ;
/**
* required bool loaded = 1;
*/
public boolean hasLoaded() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bool loaded = 1;
*/
public boolean getLoaded() {
return loaded_;
}
/**
* required bool loaded = 1;
*/
public Builder setLoaded(boolean value) {
bitField0_ |= 0x00000001;
loaded_ = value;
return this;
}
/**
* required bool loaded = 1;
*/
public Builder clearLoaded() {
bitField0_ = (bitField0_ & ~0x00000001);
loaded_ = false;
return this;
}
// @@protoc_insertion_point(builder_scope:BulkLoadHFileResponse)
}
static {
defaultInstance = new BulkLoadHFileResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:BulkLoadHFileResponse)
}
public interface CoprocessorServiceCallOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required bytes row = 1;
/**
* required bytes row = 1;
*/
boolean hasRow();
/**
* required bytes row = 1;
*/
com.google.protobuf.ByteString getRow();
// required string service_name = 2;
/**
* required string service_name = 2;
*/
boolean hasServiceName();
/**
* required string service_name = 2;
*/
java.lang.String getServiceName();
/**
* required string service_name = 2;
*/
com.google.protobuf.ByteString
getServiceNameBytes();
// required string method_name = 3;
/**
* required string method_name = 3;
*/
boolean hasMethodName();
/**
* required string method_name = 3;
*/
java.lang.String getMethodName();
/**
* required string method_name = 3;
*/
com.google.protobuf.ByteString
getMethodNameBytes();
// required bytes request = 4;
/**
* required bytes request = 4;
*/
boolean hasRequest();
/**
* required bytes request = 4;
*/
com.google.protobuf.ByteString getRequest();
}
/**
* Protobuf type {@code CoprocessorServiceCall}
*/
public static final class CoprocessorServiceCall extends
com.google.protobuf.GeneratedMessageLite
implements CoprocessorServiceCallOrBuilder {
// Use CoprocessorServiceCall.newBuilder() to construct.
private CoprocessorServiceCall(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private CoprocessorServiceCall(boolean noInit) {}
private static final CoprocessorServiceCall defaultInstance;
public static CoprocessorServiceCall getDefaultInstance() {
return defaultInstance;
}
public CoprocessorServiceCall getDefaultInstanceForType() {
return defaultInstance;
}
private CoprocessorServiceCall(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
row_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serviceName_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
methodName_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
request_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CoprocessorServiceCall parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CoprocessorServiceCall(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes row = 1;
public static final int ROW_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString row_;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
// required string service_name = 2;
public static final int SERVICE_NAME_FIELD_NUMBER = 2;
private java.lang.Object serviceName_;
/**
* required string service_name = 2;
*/
public boolean hasServiceName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string service_name = 2;
*/
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serviceName_ = s;
}
return s;
}
}
/**
* required string service_name = 2;
*/
public com.google.protobuf.ByteString
getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string method_name = 3;
public static final int METHOD_NAME_FIELD_NUMBER = 3;
private java.lang.Object methodName_;
/**
* required string method_name = 3;
*/
public boolean hasMethodName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string method_name = 3;
*/
public java.lang.String getMethodName() {
java.lang.Object ref = methodName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
methodName_ = s;
}
return s;
}
}
/**
* required string method_name = 3;
*/
public com.google.protobuf.ByteString
getMethodNameBytes() {
java.lang.Object ref = methodName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
methodName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required bytes request = 4;
public static final int REQUEST_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString request_;
/**
* required bytes request = 4;
*/
public boolean hasRequest() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes request = 4;
*/
public com.google.protobuf.ByteString getRequest() {
return request_;
}
private void initFields() {
row_ = com.google.protobuf.ByteString.EMPTY;
serviceName_ = "";
methodName_ = "";
request_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRow()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServiceName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMethodName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequest()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServiceNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMethodNameBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, request_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, row_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServiceNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMethodNameBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, request_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceCall parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.CoprocessorServiceCall prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code CoprocessorServiceCall}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.CoprocessorServiceCall, Builder>
implements org.hbase.async.generated.ClientPB.CoprocessorServiceCallOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.CoprocessorServiceCall.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
row_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
serviceName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
methodName_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
request_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall build() {
org.hbase.async.generated.ClientPB.CoprocessorServiceCall result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall buildPartial() {
org.hbase.async.generated.ClientPB.CoprocessorServiceCall result = new org.hbase.async.generated.ClientPB.CoprocessorServiceCall(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.row_ = row_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serviceName_ = serviceName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.methodName_ = methodName_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.request_ = request_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.CoprocessorServiceCall other) {
if (other == org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance()) return this;
if (other.hasRow()) {
setRow(other.getRow());
}
if (other.hasServiceName()) {
bitField0_ |= 0x00000002;
serviceName_ = other.serviceName_;
}
if (other.hasMethodName()) {
bitField0_ |= 0x00000004;
methodName_ = other.methodName_;
}
if (other.hasRequest()) {
setRequest(other.getRequest());
}
return this;
}
public final boolean isInitialized() {
if (!hasRow()) {
return false;
}
if (!hasServiceName()) {
return false;
}
if (!hasMethodName()) {
return false;
}
if (!hasRequest()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.CoprocessorServiceCall parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.CoprocessorServiceCall) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes row = 1;
private com.google.protobuf.ByteString row_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes row = 1;
*/
public boolean hasRow() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes row = 1;
*/
public com.google.protobuf.ByteString getRow() {
return row_;
}
/**
* required bytes row = 1;
*/
public Builder setRow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
row_ = value;
return this;
}
/**
* required bytes row = 1;
*/
public Builder clearRow() {
bitField0_ = (bitField0_ & ~0x00000001);
row_ = getDefaultInstance().getRow();
return this;
}
// required string service_name = 2;
private java.lang.Object serviceName_ = "";
/**
* required string service_name = 2;
*/
public boolean hasServiceName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string service_name = 2;
*/
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
serviceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string service_name = 2;
*/
public com.google.protobuf.ByteString
getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string service_name = 2;
*/
public Builder setServiceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serviceName_ = value;
return this;
}
/**
* required string service_name = 2;
*/
public Builder clearServiceName() {
bitField0_ = (bitField0_ & ~0x00000002);
serviceName_ = getDefaultInstance().getServiceName();
return this;
}
/**
* required string service_name = 2;
*/
public Builder setServiceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serviceName_ = value;
return this;
}
// required string method_name = 3;
private java.lang.Object methodName_ = "";
/**
* required string method_name = 3;
*/
public boolean hasMethodName() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string method_name = 3;
*/
public java.lang.String getMethodName() {
java.lang.Object ref = methodName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
methodName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string method_name = 3;
*/
public com.google.protobuf.ByteString
getMethodNameBytes() {
java.lang.Object ref = methodName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
methodName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string method_name = 3;
*/
public Builder setMethodName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
methodName_ = value;
return this;
}
/**
* required string method_name = 3;
*/
public Builder clearMethodName() {
bitField0_ = (bitField0_ & ~0x00000004);
methodName_ = getDefaultInstance().getMethodName();
return this;
}
/**
* required string method_name = 3;
*/
public Builder setMethodNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
methodName_ = value;
return this;
}
// required bytes request = 4;
private com.google.protobuf.ByteString request_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes request = 4;
*/
public boolean hasRequest() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes request = 4;
*/
public com.google.protobuf.ByteString getRequest() {
return request_;
}
/**
* required bytes request = 4;
*/
public Builder setRequest(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
request_ = value;
return this;
}
/**
* required bytes request = 4;
*/
public Builder clearRequest() {
bitField0_ = (bitField0_ & ~0x00000008);
request_ = getDefaultInstance().getRequest();
return this;
}
// @@protoc_insertion_point(builder_scope:CoprocessorServiceCall)
}
static {
defaultInstance = new CoprocessorServiceCall(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CoprocessorServiceCall)
}
public interface CoprocessorServiceResultOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional .NameBytesPair value = 1;
/**
* optional .NameBytesPair value = 1;
*/
boolean hasValue();
/**
* optional .NameBytesPair value = 1;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getValue();
}
/**
* Protobuf type {@code CoprocessorServiceResult}
*/
public static final class CoprocessorServiceResult extends
com.google.protobuf.GeneratedMessageLite
implements CoprocessorServiceResultOrBuilder {
// Use CoprocessorServiceResult.newBuilder() to construct.
private CoprocessorServiceResult(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private CoprocessorServiceResult(boolean noInit) {}
private static final CoprocessorServiceResult defaultInstance;
public static CoprocessorServiceResult getDefaultInstance() {
return defaultInstance;
}
public CoprocessorServiceResult getDefaultInstanceForType() {
return defaultInstance;
}
private CoprocessorServiceResult(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.NameBytesPair.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CoprocessorServiceResult parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CoprocessorServiceResult(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .NameBytesPair value = 1;
public static final int VALUE_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.NameBytesPair value_;
/**
* optional .NameBytesPair value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .NameBytesPair value = 1;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getValue() {
return value_;
}
private void initFields() {
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasValue()) {
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, value_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, value_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.CoprocessorServiceResult prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code CoprocessorServiceResult}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.CoprocessorServiceResult, Builder>
implements org.hbase.async.generated.ClientPB.CoprocessorServiceResultOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.CoprocessorServiceResult.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResult getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResult build() {
org.hbase.async.generated.ClientPB.CoprocessorServiceResult result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResult buildPartial() {
org.hbase.async.generated.ClientPB.CoprocessorServiceResult result = new org.hbase.async.generated.ClientPB.CoprocessorServiceResult(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.CoprocessorServiceResult other) {
if (other == org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance()) return this;
if (other.hasValue()) {
mergeValue(other.getValue());
}
return this;
}
public final boolean isInitialized() {
if (hasValue()) {
if (!getValue().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.CoprocessorServiceResult parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.CoprocessorServiceResult) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .NameBytesPair value = 1;
private org.hbase.async.generated.HBasePB.NameBytesPair value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
/**
* optional .NameBytesPair value = 1;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .NameBytesPair value = 1;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getValue() {
return value_;
}
/**
* optional .NameBytesPair value = 1;
*/
public Builder setValue(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .NameBytesPair value = 1;
*/
public Builder setValue(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
value_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .NameBytesPair value = 1;
*/
public Builder mergeValue(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
value_ != org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance()) {
value_ =
org.hbase.async.generated.HBasePB.NameBytesPair.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .NameBytesPair value = 1;
*/
public Builder clearValue() {
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// @@protoc_insertion_point(builder_scope:CoprocessorServiceResult)
}
static {
defaultInstance = new CoprocessorServiceResult(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CoprocessorServiceResult)
}
public interface CoprocessorServiceRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// required .CoprocessorServiceCall call = 2;
/**
* required .CoprocessorServiceCall call = 2;
*/
boolean hasCall();
/**
* required .CoprocessorServiceCall call = 2;
*/
org.hbase.async.generated.ClientPB.CoprocessorServiceCall getCall();
}
/**
* Protobuf type {@code CoprocessorServiceRequest}
*/
public static final class CoprocessorServiceRequest extends
com.google.protobuf.GeneratedMessageLite
implements CoprocessorServiceRequestOrBuilder {
// Use CoprocessorServiceRequest.newBuilder() to construct.
private CoprocessorServiceRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private CoprocessorServiceRequest(boolean noInit) {}
private static final CoprocessorServiceRequest defaultInstance;
public static CoprocessorServiceRequest getDefaultInstance() {
return defaultInstance;
}
public CoprocessorServiceRequest getDefaultInstanceForType() {
return defaultInstance;
}
private CoprocessorServiceRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = call_.toBuilder();
}
call_ = input.readMessage(org.hbase.async.generated.ClientPB.CoprocessorServiceCall.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(call_);
call_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CoprocessorServiceRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CoprocessorServiceRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// required .CoprocessorServiceCall call = 2;
public static final int CALL_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.CoprocessorServiceCall call_;
/**
* required .CoprocessorServiceCall call = 2;
*/
public boolean hasCall() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall getCall() {
return call_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
call_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCall()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getCall().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, call_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, call_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.CoprocessorServiceRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code CoprocessorServiceRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.CoprocessorServiceRequest, Builder>
implements org.hbase.async.generated.ClientPB.CoprocessorServiceRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.CoprocessorServiceRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
call_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.CoprocessorServiceRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceRequest build() {
org.hbase.async.generated.ClientPB.CoprocessorServiceRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceRequest buildPartial() {
org.hbase.async.generated.ClientPB.CoprocessorServiceRequest result = new org.hbase.async.generated.ClientPB.CoprocessorServiceRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.call_ = call_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.CoprocessorServiceRequest other) {
if (other == org.hbase.async.generated.ClientPB.CoprocessorServiceRequest.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasCall()) {
mergeCall(other.getCall());
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!hasCall()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
if (!getCall().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.CoprocessorServiceRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.CoprocessorServiceRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// required .CoprocessorServiceCall call = 2;
private org.hbase.async.generated.ClientPB.CoprocessorServiceCall call_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
/**
* required .CoprocessorServiceCall call = 2;
*/
public boolean hasCall() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall getCall() {
return call_;
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public Builder setCall(org.hbase.async.generated.ClientPB.CoprocessorServiceCall value) {
if (value == null) {
throw new NullPointerException();
}
call_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public Builder setCall(
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.Builder builderForValue) {
call_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public Builder mergeCall(org.hbase.async.generated.ClientPB.CoprocessorServiceCall value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
call_ != org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance()) {
call_ =
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.newBuilder(call_).mergeFrom(value).buildPartial();
} else {
call_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .CoprocessorServiceCall call = 2;
*/
public Builder clearCall() {
call_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// @@protoc_insertion_point(builder_scope:CoprocessorServiceRequest)
}
static {
defaultInstance = new CoprocessorServiceRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CoprocessorServiceRequest)
}
public interface CoprocessorServiceResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// required .NameBytesPair value = 2;
/**
* required .NameBytesPair value = 2;
*/
boolean hasValue();
/**
* required .NameBytesPair value = 2;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getValue();
}
/**
* Protobuf type {@code CoprocessorServiceResponse}
*/
public static final class CoprocessorServiceResponse extends
com.google.protobuf.GeneratedMessageLite
implements CoprocessorServiceResponseOrBuilder {
// Use CoprocessorServiceResponse.newBuilder() to construct.
private CoprocessorServiceResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private CoprocessorServiceResponse(boolean noInit) {}
private static final CoprocessorServiceResponse defaultInstance;
public static CoprocessorServiceResponse getDefaultInstance() {
return defaultInstance;
}
public CoprocessorServiceResponse getDefaultInstanceForType() {
return defaultInstance;
}
private CoprocessorServiceResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
org.hbase.async.generated.HBasePB.NameBytesPair.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CoprocessorServiceResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CoprocessorServiceResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// required .NameBytesPair value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private org.hbase.async.generated.HBasePB.NameBytesPair value_;
/**
* required .NameBytesPair value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NameBytesPair value = 2;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getValue() {
return value_;
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, value_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, value_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.CoprocessorServiceResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code CoprocessorServiceResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.CoprocessorServiceResponse, Builder>
implements org.hbase.async.generated.ClientPB.CoprocessorServiceResponseOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.CoprocessorServiceResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResponse getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.CoprocessorServiceResponse.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResponse build() {
org.hbase.async.generated.ClientPB.CoprocessorServiceResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.CoprocessorServiceResponse buildPartial() {
org.hbase.async.generated.ClientPB.CoprocessorServiceResponse result = new org.hbase.async.generated.ClientPB.CoprocessorServiceResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.CoprocessorServiceResponse other) {
if (other == org.hbase.async.generated.ClientPB.CoprocessorServiceResponse.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!hasValue()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
if (!getValue().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.CoprocessorServiceResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.CoprocessorServiceResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// required .NameBytesPair value = 2;
private org.hbase.async.generated.HBasePB.NameBytesPair value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
/**
* required .NameBytesPair value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .NameBytesPair value = 2;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getValue() {
return value_;
}
/**
* required .NameBytesPair value = 2;
*/
public Builder setValue(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NameBytesPair value = 2;
*/
public Builder setValue(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
value_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NameBytesPair value = 2;
*/
public Builder mergeValue(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
value_ != org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance()) {
value_ =
org.hbase.async.generated.HBasePB.NameBytesPair.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .NameBytesPair value = 2;
*/
public Builder clearValue() {
value_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// @@protoc_insertion_point(builder_scope:CoprocessorServiceResponse)
}
static {
defaultInstance = new CoprocessorServiceResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CoprocessorServiceResponse)
}
public interface ActionOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional uint32 index = 1;
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
boolean hasIndex();
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
int getIndex();
// optional .MutationProto mutation = 2;
/**
* optional .MutationProto mutation = 2;
*/
boolean hasMutation();
/**
* optional .MutationProto mutation = 2;
*/
org.hbase.async.generated.ClientPB.MutationProto getMutation();
// optional .Get get = 3;
/**
* optional .Get get = 3;
*/
boolean hasGet();
/**
* optional .Get get = 3;
*/
org.hbase.async.generated.ClientPB.Get getGet();
// optional .CoprocessorServiceCall service_call = 4;
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
boolean hasServiceCall();
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
org.hbase.async.generated.ClientPB.CoprocessorServiceCall getServiceCall();
}
/**
* Protobuf type {@code Action}
*
*
* Either a Get or a Mutation
*
*/
public static final class Action extends
com.google.protobuf.GeneratedMessageLite
implements ActionOrBuilder {
// Use Action.newBuilder() to construct.
private Action(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private Action(boolean noInit) {}
private static final Action defaultInstance;
public static Action getDefaultInstance() {
return defaultInstance;
}
public Action getDefaultInstanceForType() {
return defaultInstance;
}
private Action(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
index_ = input.readUInt32();
break;
}
case 18: {
org.hbase.async.generated.ClientPB.MutationProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = mutation_.toBuilder();
}
mutation_ = input.readMessage(org.hbase.async.generated.ClientPB.MutationProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mutation_);
mutation_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.hbase.async.generated.ClientPB.Get.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = get_.toBuilder();
}
get_ = input.readMessage(org.hbase.async.generated.ClientPB.Get.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(get_);
get_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = serviceCall_.toBuilder();
}
serviceCall_ = input.readMessage(org.hbase.async.generated.ClientPB.CoprocessorServiceCall.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(serviceCall_);
serviceCall_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Action parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Action(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 index = 1;
public static final int INDEX_FIELD_NUMBER = 1;
private int index_;
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public int getIndex() {
return index_;
}
// optional .MutationProto mutation = 2;
public static final int MUTATION_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.MutationProto mutation_;
/**
* optional .MutationProto mutation = 2;
*/
public boolean hasMutation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .MutationProto mutation = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto getMutation() {
return mutation_;
}
// optional .Get get = 3;
public static final int GET_FIELD_NUMBER = 3;
private org.hbase.async.generated.ClientPB.Get get_;
/**
* optional .Get get = 3;
*/
public boolean hasGet() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Get get = 3;
*/
public org.hbase.async.generated.ClientPB.Get getGet() {
return get_;
}
// optional .CoprocessorServiceCall service_call = 4;
public static final int SERVICE_CALL_FIELD_NUMBER = 4;
private org.hbase.async.generated.ClientPB.CoprocessorServiceCall serviceCall_;
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public boolean hasServiceCall() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall getServiceCall() {
return serviceCall_;
}
private void initFields() {
index_ = 0;
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
serviceCall_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasMutation()) {
if (!getMutation().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasGet()) {
if (!getGet().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasServiceCall()) {
if (!getServiceCall().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, index_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, mutation_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, get_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, serviceCall_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, index_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, mutation_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, get_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, serviceCall_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Action parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.Action parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.Action parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.Action prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code Action}
*
*
* Either a Get or a Mutation
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.Action, Builder>
implements org.hbase.async.generated.ClientPB.ActionOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.Action.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
index_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
serviceCall_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.Action getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.Action.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.Action build() {
org.hbase.async.generated.ClientPB.Action result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.Action buildPartial() {
org.hbase.async.generated.ClientPB.Action result = new org.hbase.async.generated.ClientPB.Action(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.index_ = index_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.mutation_ = mutation_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.get_ = get_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.serviceCall_ = serviceCall_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.Action other) {
if (other == org.hbase.async.generated.ClientPB.Action.getDefaultInstance()) return this;
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasMutation()) {
mergeMutation(other.getMutation());
}
if (other.hasGet()) {
mergeGet(other.getGet());
}
if (other.hasServiceCall()) {
mergeServiceCall(other.getServiceCall());
}
return this;
}
public final boolean isInitialized() {
if (hasMutation()) {
if (!getMutation().isInitialized()) {
return false;
}
}
if (hasGet()) {
if (!getGet().isInitialized()) {
return false;
}
}
if (hasServiceCall()) {
if (!getServiceCall().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.Action parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.Action) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 index = 1;
private int index_ ;
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public int getIndex() {
return index_;
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public Builder setIndex(int value) {
bitField0_ |= 0x00000001;
index_ = value;
return this;
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi action, useful aligning
* result with what was originally submitted.
*
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
index_ = 0;
return this;
}
// optional .MutationProto mutation = 2;
private org.hbase.async.generated.ClientPB.MutationProto mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
/**
* optional .MutationProto mutation = 2;
*/
public boolean hasMutation() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .MutationProto mutation = 2;
*/
public org.hbase.async.generated.ClientPB.MutationProto getMutation() {
return mutation_;
}
/**
* optional .MutationProto mutation = 2;
*/
public Builder setMutation(org.hbase.async.generated.ClientPB.MutationProto value) {
if (value == null) {
throw new NullPointerException();
}
mutation_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .MutationProto mutation = 2;
*/
public Builder setMutation(
org.hbase.async.generated.ClientPB.MutationProto.Builder builderForValue) {
mutation_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .MutationProto mutation = 2;
*/
public Builder mergeMutation(org.hbase.async.generated.ClientPB.MutationProto value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
mutation_ != org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance()) {
mutation_ =
org.hbase.async.generated.ClientPB.MutationProto.newBuilder(mutation_).mergeFrom(value).buildPartial();
} else {
mutation_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .MutationProto mutation = 2;
*/
public Builder clearMutation() {
mutation_ = org.hbase.async.generated.ClientPB.MutationProto.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// optional .Get get = 3;
private org.hbase.async.generated.ClientPB.Get get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
/**
* optional .Get get = 3;
*/
public boolean hasGet() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Get get = 3;
*/
public org.hbase.async.generated.ClientPB.Get getGet() {
return get_;
}
/**
* optional .Get get = 3;
*/
public Builder setGet(org.hbase.async.generated.ClientPB.Get value) {
if (value == null) {
throw new NullPointerException();
}
get_ = value;
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Get get = 3;
*/
public Builder setGet(
org.hbase.async.generated.ClientPB.Get.Builder builderForValue) {
get_ = builderForValue.build();
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Get get = 3;
*/
public Builder mergeGet(org.hbase.async.generated.ClientPB.Get value) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
get_ != org.hbase.async.generated.ClientPB.Get.getDefaultInstance()) {
get_ =
org.hbase.async.generated.ClientPB.Get.newBuilder(get_).mergeFrom(value).buildPartial();
} else {
get_ = value;
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Get get = 3;
*/
public Builder clearGet() {
get_ = org.hbase.async.generated.ClientPB.Get.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
// optional .CoprocessorServiceCall service_call = 4;
private org.hbase.async.generated.ClientPB.CoprocessorServiceCall serviceCall_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public boolean hasServiceCall() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceCall getServiceCall() {
return serviceCall_;
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public Builder setServiceCall(org.hbase.async.generated.ClientPB.CoprocessorServiceCall value) {
if (value == null) {
throw new NullPointerException();
}
serviceCall_ = value;
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public Builder setServiceCall(
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.Builder builderForValue) {
serviceCall_ = builderForValue.build();
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public Builder mergeServiceCall(org.hbase.async.generated.ClientPB.CoprocessorServiceCall value) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
serviceCall_ != org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance()) {
serviceCall_ =
org.hbase.async.generated.ClientPB.CoprocessorServiceCall.newBuilder(serviceCall_).mergeFrom(value).buildPartial();
} else {
serviceCall_ = value;
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceCall service_call = 4;
*/
public Builder clearServiceCall() {
serviceCall_ = org.hbase.async.generated.ClientPB.CoprocessorServiceCall.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
// @@protoc_insertion_point(builder_scope:Action)
}
static {
defaultInstance = new Action(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Action)
}
public interface RegionActionOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// required .RegionSpecifier region = 1;
/**
* required .RegionSpecifier region = 1;
*/
boolean hasRegion();
/**
* required .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion();
// optional bool atomic = 2;
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
boolean hasAtomic();
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
boolean getAtomic();
// repeated .Action action = 3;
/**
* repeated .Action action = 3;
*/
java.util.List
getActionList();
/**
* repeated .Action action = 3;
*/
org.hbase.async.generated.ClientPB.Action getAction(int index);
/**
* repeated .Action action = 3;
*/
int getActionCount();
}
/**
* Protobuf type {@code RegionAction}
*
*
**
* Actions to run against a Region.
*
*/
public static final class RegionAction extends
com.google.protobuf.GeneratedMessageLite
implements RegionActionOrBuilder {
// Use RegionAction.newBuilder() to construct.
private RegionAction(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private RegionAction(boolean noInit) {}
private static final RegionAction defaultInstance;
public static RegionAction getDefaultInstance() {
return defaultInstance;
}
public RegionAction getDefaultInstanceForType() {
return defaultInstance;
}
private RegionAction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = region_.toBuilder();
}
region_ = input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(region_);
region_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
atomic_ = input.readBool();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
action_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
action_.add(input.readMessage(org.hbase.async.generated.ClientPB.Action.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
action_ = java.util.Collections.unmodifiableList(action_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RegionAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionAction(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_;
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
// optional bool atomic = 2;
public static final int ATOMIC_FIELD_NUMBER = 2;
private boolean atomic_;
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public boolean hasAtomic() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public boolean getAtomic() {
return atomic_;
}
// repeated .Action action = 3;
public static final int ACTION_FIELD_NUMBER = 3;
private java.util.List action_;
/**
* repeated .Action action = 3;
*/
public java.util.List getActionList() {
return action_;
}
/**
* repeated .Action action = 3;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.ActionOrBuilder>
getActionOrBuilderList() {
return action_;
}
/**
* repeated .Action action = 3;
*/
public int getActionCount() {
return action_.size();
}
/**
* repeated .Action action = 3;
*/
public org.hbase.async.generated.ClientPB.Action getAction(int index) {
return action_.get(index);
}
/**
* repeated .Action action = 3;
*/
public org.hbase.async.generated.ClientPB.ActionOrBuilder getActionOrBuilder(
int index) {
return action_.get(index);
}
private void initFields() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
atomic_ = false;
action_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRegion()) {
memoizedIsInitialized = 0;
return false;
}
if (!getRegion().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getActionCount(); i++) {
if (!getAction(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, atomic_);
}
for (int i = 0; i < action_.size(); i++) {
output.writeMessage(3, action_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, atomic_);
}
for (int i = 0; i < action_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, action_.get(i));
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.RegionAction prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code RegionAction}
*
*
**
* Actions to run against a Region.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.RegionAction, Builder>
implements org.hbase.async.generated.ClientPB.RegionActionOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.RegionAction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
atomic_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.RegionAction getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.RegionAction.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.RegionAction build() {
org.hbase.async.generated.ClientPB.RegionAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.RegionAction buildPartial() {
org.hbase.async.generated.ClientPB.RegionAction result = new org.hbase.async.generated.ClientPB.RegionAction(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.region_ = region_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.atomic_ = atomic_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
action_ = java.util.Collections.unmodifiableList(action_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.action_ = action_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.RegionAction other) {
if (other == org.hbase.async.generated.ClientPB.RegionAction.getDefaultInstance()) return this;
if (other.hasRegion()) {
mergeRegion(other.getRegion());
}
if (other.hasAtomic()) {
setAtomic(other.getAtomic());
}
if (!other.action_.isEmpty()) {
if (action_.isEmpty()) {
action_ = other.action_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureActionIsMutable();
action_.addAll(other.action_);
}
}
return this;
}
public final boolean isInitialized() {
if (!hasRegion()) {
return false;
}
if (!getRegion().isInitialized()) {
return false;
}
for (int i = 0; i < getActionCount(); i++) {
if (!getAction(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.RegionAction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.RegionAction) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .RegionSpecifier region = 1;
private org.hbase.async.generated.HBasePB.RegionSpecifier region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
/**
* required .RegionSpecifier region = 1;
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion() {
return region_;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder setRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
region_ = builderForValue.build();
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder mergeRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
region_ != org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance()) {
region_ =
org.hbase.async.generated.HBasePB.RegionSpecifier.newBuilder(region_).mergeFrom(value).buildPartial();
} else {
region_ = value;
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = org.hbase.async.generated.HBasePB.RegionSpecifier.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
// optional bool atomic = 2;
private boolean atomic_ ;
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public boolean hasAtomic() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public boolean getAtomic() {
return atomic_;
}
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public Builder setAtomic(boolean value) {
bitField0_ |= 0x00000002;
atomic_ = value;
return this;
}
/**
* optional bool atomic = 2;
*
*
* When set, run mutations as atomic unit.
*
*/
public Builder clearAtomic() {
bitField0_ = (bitField0_ & ~0x00000002);
atomic_ = false;
return this;
}
// repeated .Action action = 3;
private java.util.List action_ =
java.util.Collections.emptyList();
private void ensureActionIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
action_ = new java.util.ArrayList(action_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated .Action action = 3;
*/
public java.util.List getActionList() {
return java.util.Collections.unmodifiableList(action_);
}
/**
* repeated .Action action = 3;
*/
public int getActionCount() {
return action_.size();
}
/**
* repeated .Action action = 3;
*/
public org.hbase.async.generated.ClientPB.Action getAction(int index) {
return action_.get(index);
}
/**
* repeated .Action action = 3;
*/
public Builder setAction(
int index, org.hbase.async.generated.ClientPB.Action value) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.set(index, value);
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder setAction(
int index, org.hbase.async.generated.ClientPB.Action.Builder builderForValue) {
ensureActionIsMutable();
action_.set(index, builderForValue.build());
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder addAction(org.hbase.async.generated.ClientPB.Action value) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.add(value);
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder addAction(
int index, org.hbase.async.generated.ClientPB.Action value) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.add(index, value);
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder addAction(
org.hbase.async.generated.ClientPB.Action.Builder builderForValue) {
ensureActionIsMutable();
action_.add(builderForValue.build());
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder addAction(
int index, org.hbase.async.generated.ClientPB.Action.Builder builderForValue) {
ensureActionIsMutable();
action_.add(index, builderForValue.build());
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder addAllAction(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.Action> values) {
ensureActionIsMutable();
super.addAll(values, action_);
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder clearAction() {
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* repeated .Action action = 3;
*/
public Builder removeAction(int index) {
ensureActionIsMutable();
action_.remove(index);
return this;
}
// @@protoc_insertion_point(builder_scope:RegionAction)
}
static {
defaultInstance = new RegionAction(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RegionAction)
}
public interface RegionLoadStatsOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional int32 memstoreLoad = 1 [default = 0];
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
boolean hasMemstoreLoad();
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
int getMemstoreLoad();
// optional int32 heapOccupancy = 2 [default = 0];
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
boolean hasHeapOccupancy();
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
int getHeapOccupancy();
// optional int32 compactionPressure = 3 [default = 0];
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
boolean hasCompactionPressure();
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
int getCompactionPressure();
}
/**
* Protobuf type {@code RegionLoadStats}
*
*
*
* Statistics about the current load on the region
*
*/
public static final class RegionLoadStats extends
com.google.protobuf.GeneratedMessageLite
implements RegionLoadStatsOrBuilder {
// Use RegionLoadStats.newBuilder() to construct.
private RegionLoadStats(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private RegionLoadStats(boolean noInit) {}
private static final RegionLoadStats defaultInstance;
public static RegionLoadStats getDefaultInstance() {
return defaultInstance;
}
public RegionLoadStats getDefaultInstanceForType() {
return defaultInstance;
}
private RegionLoadStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
memstoreLoad_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
heapOccupancy_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
compactionPressure_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RegionLoadStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionLoadStats(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional int32 memstoreLoad = 1 [default = 0];
public static final int MEMSTORELOAD_FIELD_NUMBER = 1;
private int memstoreLoad_;
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public boolean hasMemstoreLoad() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public int getMemstoreLoad() {
return memstoreLoad_;
}
// optional int32 heapOccupancy = 2 [default = 0];
public static final int HEAPOCCUPANCY_FIELD_NUMBER = 2;
private int heapOccupancy_;
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public boolean hasHeapOccupancy() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public int getHeapOccupancy() {
return heapOccupancy_;
}
// optional int32 compactionPressure = 3 [default = 0];
public static final int COMPACTIONPRESSURE_FIELD_NUMBER = 3;
private int compactionPressure_;
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public boolean hasCompactionPressure() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public int getCompactionPressure() {
return compactionPressure_;
}
private void initFields() {
memstoreLoad_ = 0;
heapOccupancy_ = 0;
compactionPressure_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, memstoreLoad_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, heapOccupancy_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, compactionPressure_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, memstoreLoad_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, heapOccupancy_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, compactionPressure_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionLoadStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.RegionLoadStats prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code RegionLoadStats}
*
*
*
* Statistics about the current load on the region
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.RegionLoadStats, Builder>
implements org.hbase.async.generated.ClientPB.RegionLoadStatsOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.RegionLoadStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
memstoreLoad_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
heapOccupancy_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
compactionPressure_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.RegionLoadStats getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.RegionLoadStats build() {
org.hbase.async.generated.ClientPB.RegionLoadStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.RegionLoadStats buildPartial() {
org.hbase.async.generated.ClientPB.RegionLoadStats result = new org.hbase.async.generated.ClientPB.RegionLoadStats(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.memstoreLoad_ = memstoreLoad_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.heapOccupancy_ = heapOccupancy_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.compactionPressure_ = compactionPressure_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.RegionLoadStats other) {
if (other == org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance()) return this;
if (other.hasMemstoreLoad()) {
setMemstoreLoad(other.getMemstoreLoad());
}
if (other.hasHeapOccupancy()) {
setHeapOccupancy(other.getHeapOccupancy());
}
if (other.hasCompactionPressure()) {
setCompactionPressure(other.getCompactionPressure());
}
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.RegionLoadStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.RegionLoadStats) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional int32 memstoreLoad = 1 [default = 0];
private int memstoreLoad_ ;
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public boolean hasMemstoreLoad() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public int getMemstoreLoad() {
return memstoreLoad_;
}
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public Builder setMemstoreLoad(int value) {
bitField0_ |= 0x00000001;
memstoreLoad_ = value;
return this;
}
/**
* optional int32 memstoreLoad = 1 [default = 0];
*
*
* Percent load on the memstore. Guaranteed to be positive, between 0 and 100.
*
*/
public Builder clearMemstoreLoad() {
bitField0_ = (bitField0_ & ~0x00000001);
memstoreLoad_ = 0;
return this;
}
// optional int32 heapOccupancy = 2 [default = 0];
private int heapOccupancy_ ;
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public boolean hasHeapOccupancy() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public int getHeapOccupancy() {
return heapOccupancy_;
}
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public Builder setHeapOccupancy(int value) {
bitField0_ |= 0x00000002;
heapOccupancy_ = value;
return this;
}
/**
* optional int32 heapOccupancy = 2 [default = 0];
*
*
* Percent JVM heap occupancy. Guaranteed to be positive, between 0 and 100.
* We can move this to "ServerLoadStats" should we develop them.
*
*/
public Builder clearHeapOccupancy() {
bitField0_ = (bitField0_ & ~0x00000002);
heapOccupancy_ = 0;
return this;
}
// optional int32 compactionPressure = 3 [default = 0];
private int compactionPressure_ ;
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public boolean hasCompactionPressure() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public int getCompactionPressure() {
return compactionPressure_;
}
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public Builder setCompactionPressure(int value) {
bitField0_ |= 0x00000004;
compactionPressure_ = value;
return this;
}
/**
* optional int32 compactionPressure = 3 [default = 0];
*
*
* Compaction pressure. Guaranteed to be positive, between 0 and 100.
*
*/
public Builder clearCompactionPressure() {
bitField0_ = (bitField0_ & ~0x00000004);
compactionPressure_ = 0;
return this;
}
// @@protoc_insertion_point(builder_scope:RegionLoadStats)
}
static {
defaultInstance = new RegionLoadStats(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RegionLoadStats)
}
public interface MultiRegionLoadStatsOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .RegionSpecifier region = 1;
/**
* repeated .RegionSpecifier region = 1;
*/
java.util.List
getRegionList();
/**
* repeated .RegionSpecifier region = 1;
*/
org.hbase.async.generated.HBasePB.RegionSpecifier getRegion(int index);
/**
* repeated .RegionSpecifier region = 1;
*/
int getRegionCount();
// repeated .RegionLoadStats stat = 2;
/**
* repeated .RegionLoadStats stat = 2;
*/
java.util.List
getStatList();
/**
* repeated .RegionLoadStats stat = 2;
*/
org.hbase.async.generated.ClientPB.RegionLoadStats getStat(int index);
/**
* repeated .RegionLoadStats stat = 2;
*/
int getStatCount();
}
/**
* Protobuf type {@code MultiRegionLoadStats}
*/
public static final class MultiRegionLoadStats extends
com.google.protobuf.GeneratedMessageLite
implements MultiRegionLoadStatsOrBuilder {
// Use MultiRegionLoadStats.newBuilder() to construct.
private MultiRegionLoadStats(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MultiRegionLoadStats(boolean noInit) {}
private static final MultiRegionLoadStats defaultInstance;
public static MultiRegionLoadStats getDefaultInstance() {
return defaultInstance;
}
public MultiRegionLoadStats getDefaultInstanceForType() {
return defaultInstance;
}
private MultiRegionLoadStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
region_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
region_.add(input.readMessage(org.hbase.async.generated.HBasePB.RegionSpecifier.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
stat_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
stat_.add(input.readMessage(org.hbase.async.generated.ClientPB.RegionLoadStats.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
region_ = java.util.Collections.unmodifiableList(region_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
stat_ = java.util.Collections.unmodifiableList(stat_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MultiRegionLoadStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MultiRegionLoadStats(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .RegionSpecifier region = 1;
public static final int REGION_FIELD_NUMBER = 1;
private java.util.List region_;
/**
* repeated .RegionSpecifier region = 1;
*/
public java.util.List getRegionList() {
return region_;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public java.util.List extends org.hbase.async.generated.HBasePB.RegionSpecifierOrBuilder>
getRegionOrBuilderList() {
return region_;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public int getRegionCount() {
return region_.size();
}
/**
* repeated .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion(int index) {
return region_.get(index);
}
/**
* repeated .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifierOrBuilder getRegionOrBuilder(
int index) {
return region_.get(index);
}
// repeated .RegionLoadStats stat = 2;
public static final int STAT_FIELD_NUMBER = 2;
private java.util.List stat_;
/**
* repeated .RegionLoadStats stat = 2;
*/
public java.util.List getStatList() {
return stat_;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.RegionLoadStatsOrBuilder>
getStatOrBuilderList() {
return stat_;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public int getStatCount() {
return stat_.size();
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public org.hbase.async.generated.ClientPB.RegionLoadStats getStat(int index) {
return stat_.get(index);
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public org.hbase.async.generated.ClientPB.RegionLoadStatsOrBuilder getStatOrBuilder(
int index) {
return stat_.get(index);
}
private void initFields() {
region_ = java.util.Collections.emptyList();
stat_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getRegionCount(); i++) {
if (!getRegion(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < region_.size(); i++) {
output.writeMessage(1, region_.get(i));
}
for (int i = 0; i < stat_.size(); i++) {
output.writeMessage(2, stat_.get(i));
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < region_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, region_.get(i));
}
for (int i = 0; i < stat_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, stat_.get(i));
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRegionLoadStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MultiRegionLoadStats prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MultiRegionLoadStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MultiRegionLoadStats, Builder>
implements org.hbase.async.generated.ClientPB.MultiRegionLoadStatsOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MultiRegionLoadStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
region_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
stat_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MultiRegionLoadStats getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MultiRegionLoadStats.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MultiRegionLoadStats build() {
org.hbase.async.generated.ClientPB.MultiRegionLoadStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MultiRegionLoadStats buildPartial() {
org.hbase.async.generated.ClientPB.MultiRegionLoadStats result = new org.hbase.async.generated.ClientPB.MultiRegionLoadStats(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
region_ = java.util.Collections.unmodifiableList(region_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.region_ = region_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
stat_ = java.util.Collections.unmodifiableList(stat_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.stat_ = stat_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MultiRegionLoadStats other) {
if (other == org.hbase.async.generated.ClientPB.MultiRegionLoadStats.getDefaultInstance()) return this;
if (!other.region_.isEmpty()) {
if (region_.isEmpty()) {
region_ = other.region_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRegionIsMutable();
region_.addAll(other.region_);
}
}
if (!other.stat_.isEmpty()) {
if (stat_.isEmpty()) {
stat_ = other.stat_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureStatIsMutable();
stat_.addAll(other.stat_);
}
}
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getRegionCount(); i++) {
if (!getRegion(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MultiRegionLoadStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MultiRegionLoadStats) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .RegionSpecifier region = 1;
private java.util.List region_ =
java.util.Collections.emptyList();
private void ensureRegionIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
region_ = new java.util.ArrayList(region_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .RegionSpecifier region = 1;
*/
public java.util.List getRegionList() {
return java.util.Collections.unmodifiableList(region_);
}
/**
* repeated .RegionSpecifier region = 1;
*/
public int getRegionCount() {
return region_.size();
}
/**
* repeated .RegionSpecifier region = 1;
*/
public org.hbase.async.generated.HBasePB.RegionSpecifier getRegion(int index) {
return region_.get(index);
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder setRegion(
int index, org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionIsMutable();
region_.set(index, value);
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder setRegion(
int index, org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
ensureRegionIsMutable();
region_.set(index, builderForValue.build());
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder addRegion(org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionIsMutable();
region_.add(value);
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder addRegion(
int index, org.hbase.async.generated.HBasePB.RegionSpecifier value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionIsMutable();
region_.add(index, value);
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder addRegion(
org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
ensureRegionIsMutable();
region_.add(builderForValue.build());
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder addRegion(
int index, org.hbase.async.generated.HBasePB.RegionSpecifier.Builder builderForValue) {
ensureRegionIsMutable();
region_.add(index, builderForValue.build());
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder addAllRegion(
java.lang.Iterable extends org.hbase.async.generated.HBasePB.RegionSpecifier> values) {
ensureRegionIsMutable();
super.addAll(values, region_);
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder clearRegion() {
region_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated .RegionSpecifier region = 1;
*/
public Builder removeRegion(int index) {
ensureRegionIsMutable();
region_.remove(index);
return this;
}
// repeated .RegionLoadStats stat = 2;
private java.util.List stat_ =
java.util.Collections.emptyList();
private void ensureStatIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
stat_ = new java.util.ArrayList(stat_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public java.util.List getStatList() {
return java.util.Collections.unmodifiableList(stat_);
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public int getStatCount() {
return stat_.size();
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public org.hbase.async.generated.ClientPB.RegionLoadStats getStat(int index) {
return stat_.get(index);
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder setStat(
int index, org.hbase.async.generated.ClientPB.RegionLoadStats value) {
if (value == null) {
throw new NullPointerException();
}
ensureStatIsMutable();
stat_.set(index, value);
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder setStat(
int index, org.hbase.async.generated.ClientPB.RegionLoadStats.Builder builderForValue) {
ensureStatIsMutable();
stat_.set(index, builderForValue.build());
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder addStat(org.hbase.async.generated.ClientPB.RegionLoadStats value) {
if (value == null) {
throw new NullPointerException();
}
ensureStatIsMutable();
stat_.add(value);
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder addStat(
int index, org.hbase.async.generated.ClientPB.RegionLoadStats value) {
if (value == null) {
throw new NullPointerException();
}
ensureStatIsMutable();
stat_.add(index, value);
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder addStat(
org.hbase.async.generated.ClientPB.RegionLoadStats.Builder builderForValue) {
ensureStatIsMutable();
stat_.add(builderForValue.build());
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder addStat(
int index, org.hbase.async.generated.ClientPB.RegionLoadStats.Builder builderForValue) {
ensureStatIsMutable();
stat_.add(index, builderForValue.build());
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder addAllStat(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.RegionLoadStats> values) {
ensureStatIsMutable();
super.addAll(values, stat_);
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder clearStat() {
stat_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* repeated .RegionLoadStats stat = 2;
*/
public Builder removeStat(int index) {
ensureStatIsMutable();
stat_.remove(index);
return this;
}
// @@protoc_insertion_point(builder_scope:MultiRegionLoadStats)
}
static {
defaultInstance = new MultiRegionLoadStats(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MultiRegionLoadStats)
}
public interface ResultOrExceptionOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// optional uint32 index = 1;
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
boolean hasIndex();
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
int getIndex();
// optional .Result result = 2;
/**
* optional .Result result = 2;
*/
boolean hasResult();
/**
* optional .Result result = 2;
*/
org.hbase.async.generated.ClientPB.Result getResult();
// optional .NameBytesPair exception = 3;
/**
* optional .NameBytesPair exception = 3;
*/
boolean hasException();
/**
* optional .NameBytesPair exception = 3;
*/
org.hbase.async.generated.HBasePB.NameBytesPair getException();
// optional .CoprocessorServiceResult service_result = 4;
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
boolean hasServiceResult();
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
org.hbase.async.generated.ClientPB.CoprocessorServiceResult getServiceResult();
// optional .RegionLoadStats loadStats = 5 [deprecated = true];
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated boolean hasLoadStats();
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated org.hbase.async.generated.ClientPB.RegionLoadStats getLoadStats();
}
/**
* Protobuf type {@code ResultOrException}
*
*
**
* Either a Result or an Exception NameBytesPair (keyed by
* exception name whose value is the exception stringified)
* or maybe empty if no result and no exception.
*
*/
public static final class ResultOrException extends
com.google.protobuf.GeneratedMessageLite
implements ResultOrExceptionOrBuilder {
// Use ResultOrException.newBuilder() to construct.
private ResultOrException(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private ResultOrException(boolean noInit) {}
private static final ResultOrException defaultInstance;
public static ResultOrException getDefaultInstance() {
return defaultInstance;
}
public ResultOrException getDefaultInstanceForType() {
return defaultInstance;
}
private ResultOrException(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
index_ = input.readUInt32();
break;
}
case 18: {
org.hbase.async.generated.ClientPB.Result.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = result_.toBuilder();
}
result_ = input.readMessage(org.hbase.async.generated.ClientPB.Result.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(result_);
result_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
org.hbase.async.generated.HBasePB.NameBytesPair.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = exception_.toBuilder();
}
exception_ = input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(exception_);
exception_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
org.hbase.async.generated.ClientPB.CoprocessorServiceResult.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = serviceResult_.toBuilder();
}
serviceResult_ = input.readMessage(org.hbase.async.generated.ClientPB.CoprocessorServiceResult.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(serviceResult_);
serviceResult_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
org.hbase.async.generated.ClientPB.RegionLoadStats.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = loadStats_.toBuilder();
}
loadStats_ = input.readMessage(org.hbase.async.generated.ClientPB.RegionLoadStats.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(loadStats_);
loadStats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ResultOrException parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResultOrException(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 index = 1;
public static final int INDEX_FIELD_NUMBER = 1;
private int index_;
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public int getIndex() {
return index_;
}
// optional .Result result = 2;
public static final int RESULT_FIELD_NUMBER = 2;
private org.hbase.async.generated.ClientPB.Result result_;
/**
* optional .Result result = 2;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Result result = 2;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
// optional .NameBytesPair exception = 3;
public static final int EXCEPTION_FIELD_NUMBER = 3;
private org.hbase.async.generated.HBasePB.NameBytesPair exception_;
/**
* optional .NameBytesPair exception = 3;
*/
public boolean hasException() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .NameBytesPair exception = 3;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getException() {
return exception_;
}
// optional .CoprocessorServiceResult service_result = 4;
public static final int SERVICE_RESULT_FIELD_NUMBER = 4;
private org.hbase.async.generated.ClientPB.CoprocessorServiceResult serviceResult_;
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public boolean hasServiceResult() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceResult getServiceResult() {
return serviceResult_;
}
// optional .RegionLoadStats loadStats = 5 [deprecated = true];
public static final int LOADSTATS_FIELD_NUMBER = 5;
private org.hbase.async.generated.ClientPB.RegionLoadStats loadStats_;
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public boolean hasLoadStats() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public org.hbase.async.generated.ClientPB.RegionLoadStats getLoadStats() {
return loadStats_;
}
private void initFields() {
index_ = 0;
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
serviceResult_ = org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance();
loadStats_ = org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasException()) {
if (!getException().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasServiceResult()) {
if (!getServiceResult().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, index_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, result_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, exception_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, serviceResult_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, loadStats_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, index_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, result_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, exception_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, serviceResult_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, loadStats_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.ResultOrException parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.ResultOrException prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code ResultOrException}
*
*
**
* Either a Result or an Exception NameBytesPair (keyed by
* exception name whose value is the exception stringified)
* or maybe empty if no result and no exception.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.ResultOrException, Builder>
implements org.hbase.async.generated.ClientPB.ResultOrExceptionOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.ResultOrException.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
index_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
serviceResult_ = org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
loadStats_ = org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.ResultOrException getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.ResultOrException.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.ResultOrException build() {
org.hbase.async.generated.ClientPB.ResultOrException result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.ResultOrException buildPartial() {
org.hbase.async.generated.ClientPB.ResultOrException result = new org.hbase.async.generated.ClientPB.ResultOrException(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.index_ = index_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.result_ = result_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.exception_ = exception_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.serviceResult_ = serviceResult_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.loadStats_ = loadStats_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.ResultOrException other) {
if (other == org.hbase.async.generated.ClientPB.ResultOrException.getDefaultInstance()) return this;
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasResult()) {
mergeResult(other.getResult());
}
if (other.hasException()) {
mergeException(other.getException());
}
if (other.hasServiceResult()) {
mergeServiceResult(other.getServiceResult());
}
if (other.hasLoadStats()) {
mergeLoadStats(other.getLoadStats());
}
return this;
}
public final boolean isInitialized() {
if (hasException()) {
if (!getException().isInitialized()) {
return false;
}
}
if (hasServiceResult()) {
if (!getServiceResult().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.ResultOrException parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.ResultOrException) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 index = 1;
private int index_ ;
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public int getIndex() {
return index_;
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public Builder setIndex(int value) {
bitField0_ |= 0x00000001;
index_ = value;
return this;
}
/**
* optional uint32 index = 1;
*
*
* If part of a multi call, save original index of the list of all
* passed so can align this response w/ original request.
*
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000001);
index_ = 0;
return this;
}
// optional .Result result = 2;
private org.hbase.async.generated.ClientPB.Result result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
/**
* optional .Result result = 2;
*/
public boolean hasResult() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Result result = 2;
*/
public org.hbase.async.generated.ClientPB.Result getResult() {
return result_;
}
/**
* optional .Result result = 2;
*/
public Builder setResult(org.hbase.async.generated.ClientPB.Result value) {
if (value == null) {
throw new NullPointerException();
}
result_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Result result = 2;
*/
public Builder setResult(
org.hbase.async.generated.ClientPB.Result.Builder builderForValue) {
result_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Result result = 2;
*/
public Builder mergeResult(org.hbase.async.generated.ClientPB.Result value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
result_ != org.hbase.async.generated.ClientPB.Result.getDefaultInstance()) {
result_ =
org.hbase.async.generated.ClientPB.Result.newBuilder(result_).mergeFrom(value).buildPartial();
} else {
result_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .Result result = 2;
*/
public Builder clearResult() {
result_ = org.hbase.async.generated.ClientPB.Result.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// optional .NameBytesPair exception = 3;
private org.hbase.async.generated.HBasePB.NameBytesPair exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
/**
* optional .NameBytesPair exception = 3;
*/
public boolean hasException() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .NameBytesPair exception = 3;
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getException() {
return exception_;
}
/**
* optional .NameBytesPair exception = 3;
*/
public Builder setException(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
exception_ = value;
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NameBytesPair exception = 3;
*/
public Builder setException(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
exception_ = builderForValue.build();
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NameBytesPair exception = 3;
*/
public Builder mergeException(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
exception_ != org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance()) {
exception_ =
org.hbase.async.generated.HBasePB.NameBytesPair.newBuilder(exception_).mergeFrom(value).buildPartial();
} else {
exception_ = value;
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .NameBytesPair exception = 3;
*/
public Builder clearException() {
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
// optional .CoprocessorServiceResult service_result = 4;
private org.hbase.async.generated.ClientPB.CoprocessorServiceResult serviceResult_ = org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance();
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public boolean hasServiceResult() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public org.hbase.async.generated.ClientPB.CoprocessorServiceResult getServiceResult() {
return serviceResult_;
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public Builder setServiceResult(org.hbase.async.generated.ClientPB.CoprocessorServiceResult value) {
if (value == null) {
throw new NullPointerException();
}
serviceResult_ = value;
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public Builder setServiceResult(
org.hbase.async.generated.ClientPB.CoprocessorServiceResult.Builder builderForValue) {
serviceResult_ = builderForValue.build();
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public Builder mergeServiceResult(org.hbase.async.generated.ClientPB.CoprocessorServiceResult value) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
serviceResult_ != org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance()) {
serviceResult_ =
org.hbase.async.generated.ClientPB.CoprocessorServiceResult.newBuilder(serviceResult_).mergeFrom(value).buildPartial();
} else {
serviceResult_ = value;
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .CoprocessorServiceResult service_result = 4;
*
*
* result if this was a coprocessor service call
*
*/
public Builder clearServiceResult() {
serviceResult_ = org.hbase.async.generated.ClientPB.CoprocessorServiceResult.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
// optional .RegionLoadStats loadStats = 5 [deprecated = true];
private org.hbase.async.generated.ClientPB.RegionLoadStats loadStats_ = org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance();
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public boolean hasLoadStats() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public org.hbase.async.generated.ClientPB.RegionLoadStats getLoadStats() {
return loadStats_;
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public Builder setLoadStats(org.hbase.async.generated.ClientPB.RegionLoadStats value) {
if (value == null) {
throw new NullPointerException();
}
loadStats_ = value;
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public Builder setLoadStats(
org.hbase.async.generated.ClientPB.RegionLoadStats.Builder builderForValue) {
loadStats_ = builderForValue.build();
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public Builder mergeLoadStats(org.hbase.async.generated.ClientPB.RegionLoadStats value) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
loadStats_ != org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance()) {
loadStats_ =
org.hbase.async.generated.ClientPB.RegionLoadStats.newBuilder(loadStats_).mergeFrom(value).buildPartial();
} else {
loadStats_ = value;
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RegionLoadStats loadStats = 5 [deprecated = true];
*
*
* current load on the region
*
*/
@java.lang.Deprecated public Builder clearLoadStats() {
loadStats_ = org.hbase.async.generated.ClientPB.RegionLoadStats.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
// @@protoc_insertion_point(builder_scope:ResultOrException)
}
static {
defaultInstance = new ResultOrException(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ResultOrException)
}
public interface RegionActionResultOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .ResultOrException resultOrException = 1;
/**
* repeated .ResultOrException resultOrException = 1;
*/
java.util.List
getResultOrExceptionList();
/**
* repeated .ResultOrException resultOrException = 1;
*/
org.hbase.async.generated.ClientPB.ResultOrException getResultOrException(int index);
/**
* repeated .ResultOrException resultOrException = 1;
*/
int getResultOrExceptionCount();
// optional .NameBytesPair exception = 2;
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
boolean hasException();
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
org.hbase.async.generated.HBasePB.NameBytesPair getException();
}
/**
* Protobuf type {@code RegionActionResult}
*
*
**
* The result of a RegionAction.
*
*/
public static final class RegionActionResult extends
com.google.protobuf.GeneratedMessageLite
implements RegionActionResultOrBuilder {
// Use RegionActionResult.newBuilder() to construct.
private RegionActionResult(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private RegionActionResult(boolean noInit) {}
private static final RegionActionResult defaultInstance;
public static RegionActionResult getDefaultInstance() {
return defaultInstance;
}
public RegionActionResult getDefaultInstanceForType() {
return defaultInstance;
}
private RegionActionResult(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
resultOrException_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
resultOrException_.add(input.readMessage(org.hbase.async.generated.ClientPB.ResultOrException.PARSER, extensionRegistry));
break;
}
case 18: {
org.hbase.async.generated.HBasePB.NameBytesPair.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = exception_.toBuilder();
}
exception_ = input.readMessage(org.hbase.async.generated.HBasePB.NameBytesPair.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(exception_);
exception_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
resultOrException_ = java.util.Collections.unmodifiableList(resultOrException_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RegionActionResult parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RegionActionResult(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated .ResultOrException resultOrException = 1;
public static final int RESULTOREXCEPTION_FIELD_NUMBER = 1;
private java.util.List resultOrException_;
/**
* repeated .ResultOrException resultOrException = 1;
*/
public java.util.List getResultOrExceptionList() {
return resultOrException_;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.ResultOrExceptionOrBuilder>
getResultOrExceptionOrBuilderList() {
return resultOrException_;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public int getResultOrExceptionCount() {
return resultOrException_.size();
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public org.hbase.async.generated.ClientPB.ResultOrException getResultOrException(int index) {
return resultOrException_.get(index);
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public org.hbase.async.generated.ClientPB.ResultOrExceptionOrBuilder getResultOrExceptionOrBuilder(
int index) {
return resultOrException_.get(index);
}
// optional .NameBytesPair exception = 2;
public static final int EXCEPTION_FIELD_NUMBER = 2;
private org.hbase.async.generated.HBasePB.NameBytesPair exception_;
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public boolean hasException() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getException() {
return exception_;
}
private void initFields() {
resultOrException_ = java.util.Collections.emptyList();
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getResultOrExceptionCount(); i++) {
if (!getResultOrException(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasException()) {
if (!getException().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < resultOrException_.size(); i++) {
output.writeMessage(1, resultOrException_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(2, exception_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < resultOrException_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, resultOrException_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, exception_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.RegionActionResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.RegionActionResult prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code RegionActionResult}
*
*
**
* The result of a RegionAction.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.RegionActionResult, Builder>
implements org.hbase.async.generated.ClientPB.RegionActionResultOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.RegionActionResult.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
resultOrException_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.RegionActionResult getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.RegionActionResult.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.RegionActionResult build() {
org.hbase.async.generated.ClientPB.RegionActionResult result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.RegionActionResult buildPartial() {
org.hbase.async.generated.ClientPB.RegionActionResult result = new org.hbase.async.generated.ClientPB.RegionActionResult(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
resultOrException_ = java.util.Collections.unmodifiableList(resultOrException_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.resultOrException_ = resultOrException_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.exception_ = exception_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.RegionActionResult other) {
if (other == org.hbase.async.generated.ClientPB.RegionActionResult.getDefaultInstance()) return this;
if (!other.resultOrException_.isEmpty()) {
if (resultOrException_.isEmpty()) {
resultOrException_ = other.resultOrException_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureResultOrExceptionIsMutable();
resultOrException_.addAll(other.resultOrException_);
}
}
if (other.hasException()) {
mergeException(other.getException());
}
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getResultOrExceptionCount(); i++) {
if (!getResultOrException(i).isInitialized()) {
return false;
}
}
if (hasException()) {
if (!getException().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.RegionActionResult parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.RegionActionResult) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .ResultOrException resultOrException = 1;
private java.util.List resultOrException_ =
java.util.Collections.emptyList();
private void ensureResultOrExceptionIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
resultOrException_ = new java.util.ArrayList(resultOrException_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public java.util.List getResultOrExceptionList() {
return java.util.Collections.unmodifiableList(resultOrException_);
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public int getResultOrExceptionCount() {
return resultOrException_.size();
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public org.hbase.async.generated.ClientPB.ResultOrException getResultOrException(int index) {
return resultOrException_.get(index);
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder setResultOrException(
int index, org.hbase.async.generated.ClientPB.ResultOrException value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultOrExceptionIsMutable();
resultOrException_.set(index, value);
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder setResultOrException(
int index, org.hbase.async.generated.ClientPB.ResultOrException.Builder builderForValue) {
ensureResultOrExceptionIsMutable();
resultOrException_.set(index, builderForValue.build());
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder addResultOrException(org.hbase.async.generated.ClientPB.ResultOrException value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultOrExceptionIsMutable();
resultOrException_.add(value);
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder addResultOrException(
int index, org.hbase.async.generated.ClientPB.ResultOrException value) {
if (value == null) {
throw new NullPointerException();
}
ensureResultOrExceptionIsMutable();
resultOrException_.add(index, value);
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder addResultOrException(
org.hbase.async.generated.ClientPB.ResultOrException.Builder builderForValue) {
ensureResultOrExceptionIsMutable();
resultOrException_.add(builderForValue.build());
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder addResultOrException(
int index, org.hbase.async.generated.ClientPB.ResultOrException.Builder builderForValue) {
ensureResultOrExceptionIsMutable();
resultOrException_.add(index, builderForValue.build());
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder addAllResultOrException(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.ResultOrException> values) {
ensureResultOrExceptionIsMutable();
super.addAll(values, resultOrException_);
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder clearResultOrException() {
resultOrException_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated .ResultOrException resultOrException = 1;
*/
public Builder removeResultOrException(int index) {
ensureResultOrExceptionIsMutable();
resultOrException_.remove(index);
return this;
}
// optional .NameBytesPair exception = 2;
private org.hbase.async.generated.HBasePB.NameBytesPair exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public boolean hasException() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public org.hbase.async.generated.HBasePB.NameBytesPair getException() {
return exception_;
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public Builder setException(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (value == null) {
throw new NullPointerException();
}
exception_ = value;
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public Builder setException(
org.hbase.async.generated.HBasePB.NameBytesPair.Builder builderForValue) {
exception_ = builderForValue.build();
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public Builder mergeException(org.hbase.async.generated.HBasePB.NameBytesPair value) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
exception_ != org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance()) {
exception_ =
org.hbase.async.generated.HBasePB.NameBytesPair.newBuilder(exception_).mergeFrom(value).buildPartial();
} else {
exception_ = value;
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .NameBytesPair exception = 2;
*
*
* If the operation failed globally for this region, this exception is set
*
*/
public Builder clearException() {
exception_ = org.hbase.async.generated.HBasePB.NameBytesPair.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
// @@protoc_insertion_point(builder_scope:RegionActionResult)
}
static {
defaultInstance = new RegionActionResult(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RegionActionResult)
}
public interface MultiRequestOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .RegionAction regionAction = 1;
/**
* repeated .RegionAction regionAction = 1;
*/
java.util.List
getRegionActionList();
/**
* repeated .RegionAction regionAction = 1;
*/
org.hbase.async.generated.ClientPB.RegionAction getRegionAction(int index);
/**
* repeated .RegionAction regionAction = 1;
*/
int getRegionActionCount();
// optional uint64 nonceGroup = 2;
/**
* optional uint64 nonceGroup = 2;
*/
boolean hasNonceGroup();
/**
* optional uint64 nonceGroup = 2;
*/
long getNonceGroup();
// optional .Condition condition = 3;
/**
* optional .Condition condition = 3;
*/
boolean hasCondition();
/**
* optional .Condition condition = 3;
*/
org.hbase.async.generated.ClientPB.Condition getCondition();
}
/**
* Protobuf type {@code MultiRequest}
*
*
**
* Execute a list of actions on a given region in order.
* Nothing prevents a request to contains a set of RegionAction on the same region.
* For this reason, the matching between the MultiRequest and the MultiResponse is not
* done by the region specifier but by keeping the order of the RegionActionResult vs.
* the order of the RegionAction.
*
*/
public static final class MultiRequest extends
com.google.protobuf.GeneratedMessageLite
implements MultiRequestOrBuilder {
// Use MultiRequest.newBuilder() to construct.
private MultiRequest(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MultiRequest(boolean noInit) {}
private static final MultiRequest defaultInstance;
public static MultiRequest getDefaultInstance() {
return defaultInstance;
}
public MultiRequest getDefaultInstanceForType() {
return defaultInstance;
}
private MultiRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
regionAction_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
regionAction_.add(input.readMessage(org.hbase.async.generated.ClientPB.RegionAction.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
nonceGroup_ = input.readUInt64();
break;
}
case 26: {
org.hbase.async.generated.ClientPB.Condition.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = condition_.toBuilder();
}
condition_ = input.readMessage(org.hbase.async.generated.ClientPB.Condition.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(condition_);
condition_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
regionAction_ = java.util.Collections.unmodifiableList(regionAction_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MultiRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MultiRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// repeated .RegionAction regionAction = 1;
public static final int REGIONACTION_FIELD_NUMBER = 1;
private java.util.List regionAction_;
/**
* repeated .RegionAction regionAction = 1;
*/
public java.util.List getRegionActionList() {
return regionAction_;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public java.util.List extends org.hbase.async.generated.ClientPB.RegionActionOrBuilder>
getRegionActionOrBuilderList() {
return regionAction_;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public int getRegionActionCount() {
return regionAction_.size();
}
/**
* repeated .RegionAction regionAction = 1;
*/
public org.hbase.async.generated.ClientPB.RegionAction getRegionAction(int index) {
return regionAction_.get(index);
}
/**
* repeated .RegionAction regionAction = 1;
*/
public org.hbase.async.generated.ClientPB.RegionActionOrBuilder getRegionActionOrBuilder(
int index) {
return regionAction_.get(index);
}
// optional uint64 nonceGroup = 2;
public static final int NONCEGROUP_FIELD_NUMBER = 2;
private long nonceGroup_;
/**
* optional uint64 nonceGroup = 2;
*/
public boolean hasNonceGroup() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint64 nonceGroup = 2;
*/
public long getNonceGroup() {
return nonceGroup_;
}
// optional .Condition condition = 3;
public static final int CONDITION_FIELD_NUMBER = 3;
private org.hbase.async.generated.ClientPB.Condition condition_;
/**
* optional .Condition condition = 3;
*/
public boolean hasCondition() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .Condition condition = 3;
*/
public org.hbase.async.generated.ClientPB.Condition getCondition() {
return condition_;
}
private void initFields() {
regionAction_ = java.util.Collections.emptyList();
nonceGroup_ = 0L;
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getRegionActionCount(); i++) {
if (!getRegionAction(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasCondition()) {
if (!getCondition().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < regionAction_.size(); i++) {
output.writeMessage(1, regionAction_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(2, nonceGroup_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(3, condition_);
}
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < regionAction_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, regionAction_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, nonceGroup_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, condition_);
}
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.hbase.async.generated.ClientPB.MultiRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.hbase.async.generated.ClientPB.MultiRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
/**
* Protobuf type {@code MultiRequest}
*
*
**
* Execute a list of actions on a given region in order.
* Nothing prevents a request to contains a set of RegionAction on the same region.
* For this reason, the matching between the MultiRequest and the MultiResponse is not
* done by the region specifier but by keeping the order of the RegionActionResult vs.
* the order of the RegionAction.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
org.hbase.async.generated.ClientPB.MultiRequest, Builder>
implements org.hbase.async.generated.ClientPB.MultiRequestOrBuilder {
// Construct using org.hbase.async.generated.ClientPB.MultiRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
regionAction_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
nonceGroup_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public org.hbase.async.generated.ClientPB.MultiRequest getDefaultInstanceForType() {
return org.hbase.async.generated.ClientPB.MultiRequest.getDefaultInstance();
}
public org.hbase.async.generated.ClientPB.MultiRequest build() {
org.hbase.async.generated.ClientPB.MultiRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.hbase.async.generated.ClientPB.MultiRequest buildPartial() {
org.hbase.async.generated.ClientPB.MultiRequest result = new org.hbase.async.generated.ClientPB.MultiRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
regionAction_ = java.util.Collections.unmodifiableList(regionAction_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.regionAction_ = regionAction_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.nonceGroup_ = nonceGroup_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.condition_ = condition_;
result.bitField0_ = to_bitField0_;
return result;
}
public Builder mergeFrom(org.hbase.async.generated.ClientPB.MultiRequest other) {
if (other == org.hbase.async.generated.ClientPB.MultiRequest.getDefaultInstance()) return this;
if (!other.regionAction_.isEmpty()) {
if (regionAction_.isEmpty()) {
regionAction_ = other.regionAction_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRegionActionIsMutable();
regionAction_.addAll(other.regionAction_);
}
}
if (other.hasNonceGroup()) {
setNonceGroup(other.getNonceGroup());
}
if (other.hasCondition()) {
mergeCondition(other.getCondition());
}
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getRegionActionCount(); i++) {
if (!getRegionAction(i).isInitialized()) {
return false;
}
}
if (hasCondition()) {
if (!getCondition().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.hbase.async.generated.ClientPB.MultiRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.hbase.async.generated.ClientPB.MultiRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .RegionAction regionAction = 1;
private java.util.List regionAction_ =
java.util.Collections.emptyList();
private void ensureRegionActionIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
regionAction_ = new java.util.ArrayList(regionAction_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .RegionAction regionAction = 1;
*/
public java.util.List getRegionActionList() {
return java.util.Collections.unmodifiableList(regionAction_);
}
/**
* repeated .RegionAction regionAction = 1;
*/
public int getRegionActionCount() {
return regionAction_.size();
}
/**
* repeated .RegionAction regionAction = 1;
*/
public org.hbase.async.generated.ClientPB.RegionAction getRegionAction(int index) {
return regionAction_.get(index);
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder setRegionAction(
int index, org.hbase.async.generated.ClientPB.RegionAction value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionActionIsMutable();
regionAction_.set(index, value);
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder setRegionAction(
int index, org.hbase.async.generated.ClientPB.RegionAction.Builder builderForValue) {
ensureRegionActionIsMutable();
regionAction_.set(index, builderForValue.build());
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder addRegionAction(org.hbase.async.generated.ClientPB.RegionAction value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionActionIsMutable();
regionAction_.add(value);
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder addRegionAction(
int index, org.hbase.async.generated.ClientPB.RegionAction value) {
if (value == null) {
throw new NullPointerException();
}
ensureRegionActionIsMutable();
regionAction_.add(index, value);
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder addRegionAction(
org.hbase.async.generated.ClientPB.RegionAction.Builder builderForValue) {
ensureRegionActionIsMutable();
regionAction_.add(builderForValue.build());
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder addRegionAction(
int index, org.hbase.async.generated.ClientPB.RegionAction.Builder builderForValue) {
ensureRegionActionIsMutable();
regionAction_.add(index, builderForValue.build());
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder addAllRegionAction(
java.lang.Iterable extends org.hbase.async.generated.ClientPB.RegionAction> values) {
ensureRegionActionIsMutable();
super.addAll(values, regionAction_);
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder clearRegionAction() {
regionAction_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* repeated .RegionAction regionAction = 1;
*/
public Builder removeRegionAction(int index) {
ensureRegionActionIsMutable();
regionAction_.remove(index);
return this;
}
// optional uint64 nonceGroup = 2;
private long nonceGroup_ ;
/**
* optional uint64 nonceGroup = 2;
*/
public boolean hasNonceGroup() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 nonceGroup = 2;
*/
public long getNonceGroup() {
return nonceGroup_;
}
/**
* optional uint64 nonceGroup = 2;
*/
public Builder setNonceGroup(long value) {
bitField0_ |= 0x00000002;
nonceGroup_ = value;
return this;
}
/**
* optional uint64 nonceGroup = 2;
*/
public Builder clearNonceGroup() {
bitField0_ = (bitField0_ & ~0x00000002);
nonceGroup_ = 0L;
return this;
}
// optional .Condition condition = 3;
private org.hbase.async.generated.ClientPB.Condition condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
/**
* optional .Condition condition = 3;
*/
public boolean hasCondition() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .Condition condition = 3;
*/
public org.hbase.async.generated.ClientPB.Condition getCondition() {
return condition_;
}
/**
* optional .Condition condition = 3;
*/
public Builder setCondition(org.hbase.async.generated.ClientPB.Condition value) {
if (value == null) {
throw new NullPointerException();
}
condition_ = value;
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder setCondition(
org.hbase.async.generated.ClientPB.Condition.Builder builderForValue) {
condition_ = builderForValue.build();
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder mergeCondition(org.hbase.async.generated.ClientPB.Condition value) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
condition_ != org.hbase.async.generated.ClientPB.Condition.getDefaultInstance()) {
condition_ =
org.hbase.async.generated.ClientPB.Condition.newBuilder(condition_).mergeFrom(value).buildPartial();
} else {
condition_ = value;
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .Condition condition = 3;
*/
public Builder clearCondition() {
condition_ = org.hbase.async.generated.ClientPB.Condition.getDefaultInstance();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
// @@protoc_insertion_point(builder_scope:MultiRequest)
}
static {
defaultInstance = new MultiRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MultiRequest)
}
public interface MultiResponseOrBuilder
extends com.google.protobuf.MessageLiteOrBuilder {
// repeated .RegionActionResult regionActionResult = 1;
/**
* repeated .RegionActionResult regionActionResult = 1;
*/
java.util.List
getRegionActionResultList();
/**
* repeated .RegionActionResult regionActionResult = 1;
*/
org.hbase.async.generated.ClientPB.RegionActionResult getRegionActionResult(int index);
/**
* repeated .RegionActionResult regionActionResult = 1;
*/
int getRegionActionResultCount();
// optional bool processed = 2;
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
boolean hasProcessed();
/**
* optional bool processed = 2;
*
*
* used for mutate to indicate processed only
*
*/
boolean getProcessed();
// optional .MultiRegionLoadStats regionStatistics = 3;
/**
* optional .MultiRegionLoadStats regionStatistics = 3;
*/
boolean hasRegionStatistics();
/**
* optional .MultiRegionLoadStats regionStatistics = 3;
*/
org.hbase.async.generated.ClientPB.MultiRegionLoadStats getRegionStatistics();
}
/**
* Protobuf type {@code MultiResponse}
*/
public static final class MultiResponse extends
com.google.protobuf.GeneratedMessageLite
implements MultiResponseOrBuilder {
// Use MultiResponse.newBuilder() to construct.
private MultiResponse(com.google.protobuf.GeneratedMessageLite.Builder builder) {
super(builder);
}
private MultiResponse(boolean noInit) {}
private static final MultiResponse defaultInstance;
public static MultiResponse getDefaultInstance() {
return defaultInstance;
}
public MultiResponse getDefaultInstanceForType() {
return defaultInstance;
}
private MultiResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
regionActionResult_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
regionActionResult_.add(input.readMessage(org.hbase.async.generated.ClientPB.RegionActionResult.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
processed_ = input.readBool();
break;
}
case 26: {
org.hbase.async.generated.ClientPB.MultiRegionLoadStats.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = regionStatistics_.toBuilder();
}
regionStatistics_ = input.readMessage(org.hbase.async.generated.ClientPB.MultiRegionLoadStats.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(regionStatistics_);
regionStatistics_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
regionActionResult_ = java.util.Collections.unmodifiableList(regionActionResult_);
}
makeExtensionsImmutable();
}
}
public static com.google.protobuf.Parser