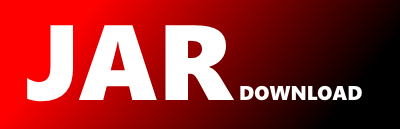
ncsa.hdf.view.DefaultMetaDataView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hdf-java Show documentation
Show all versions of hdf-java Show documentation
APIs to access native hdf4 and hdf5 libraries.
The newest version!
/*****************************************************************************
* Copyright by The HDF Group. *
* Copyright by the Board of Trustees of the University of Illinois. *
* All rights reserved. *
* *
* This file is part of the HDF Java Products distribution. *
* The full copyright notice, including terms governing use, modification, *
* and redistribution, is contained in the files COPYING and Copyright.html. *
* COPYING can be found at the root of the source code distribution tree. *
* Or, see http://hdfgroup.org/products/hdf-java/doc/Copyright.html. *
* If you do not have access to either file, you may request a copy from *
* [email protected]. *
****************************************************************************/
package ncsa.hdf.view;
import ncsa.hdf.object.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.border.*;
import javax.swing.event.*;
import javax.swing.table.*;
import javax.swing.tree.*;
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.Point;
import java.awt.Insets;
import java.awt.Dimension;
import java.util.*;
import java.io.File;
import java.lang.reflect.Array;
/**
* DefaultMetadataView is an dialog window used to show data properties.
* Data properties include attributes and general information such as
* object type, data type and data space.
*
* @author Peter X. Cao
* @version 2.4 9/6/2007
*/
public class DefaultMetaDataView extends JDialog
implements ActionListener, MetaDataView
{
public static final long serialVersionUID = HObject.serialVersionUID;
/**
* The main HDFView.
*/
private ViewManager viewer;
/** The HDF data object */
private HObject hObject;
private JTabbedPane tabbedPane = null;
private JTextArea attrContentArea;
private JTable attrTable; // table to hold a list of attributes
private DefaultTableModel attrTableModel;
private JLabel attrNumberLabel;
private int numAttributes;
private boolean isH5, isH4;
private byte[] userBlock;
private JTextArea userBlockArea;
private JButton jamButton;
/**
* Constructs a DefaultMetadataView with the given HDFView.
*/
public DefaultMetaDataView(ViewManager theView) {
super((JFrame)theView, false);
setDefaultCloseOperation(JInternalFrame.DISPOSE_ON_CLOSE);
viewer = theView;
hObject = viewer.getTreeView().getCurrentObject();
numAttributes = 0;
userBlock = null;
userBlockArea = null;
if (hObject == null) {
dispose();
} else if ( hObject.getPath()== null) {
setTitle("Properties - "+hObject.getName());
} else {
setTitle("Properties - "+hObject.getPath()+hObject.getName());
}
isH5 = hObject.getFileFormat().isThisType(FileFormat.getFileFormat(FileFormat.FILE_TYPE_HDF5));
isH4 = hObject.getFileFormat().isThisType(FileFormat.getFileFormat(FileFormat.FILE_TYPE_HDF4));
tabbedPane = new JTabbedPane();
// get the metadata information before add GUI components */
try { hObject.getMetadata(); } catch (Exception ex) {}
tabbedPane.addTab("General", createGeneralPropertyPanel());
tabbedPane.addTab("Attributes", createAttributePanel());
boolean isRoot = ((hObject instanceof Group) && ((Group)hObject).isRoot());
if (isH5 && isRoot)
{
// add panel to display user block
tabbedPane.addTab("User Block", createUserBlockPanel());
}
tabbedPane.setSelectedIndex(0);
JPanel bPanel = new JPanel();
JButton b = new JButton(" Close ");
b.setMnemonic(KeyEvent.VK_C);
b.setActionCommand("Close");
b.addActionListener(this);
bPanel.add(b);
//Add the tabbed pane to this panel.
JPanel contentPane = (JPanel)getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.setBorder(BorderFactory.createEmptyBorder(5,5,5,5));
contentPane.setPreferredSize(new Dimension(620, 400));
contentPane.add("Center", tabbedPane);
contentPane.add("South", bPanel);
// locate the H5Property dialog
Point l = getParent().getLocation();
l.x += 250;
l.y += 80;
setLocation(l);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
Object source = e.getSource();
String cmd = e.getActionCommand();
if (cmd.equals("Close")) {
dispose();
}
else if (cmd.equals("Add attribute")) {
addAttribute(hObject);
}
else if (cmd.equals("Delete attribute")) {
deleteAttribute(hObject);
}
else if (cmd.equals("Jam user block")) {
writeUserBlock();
}
else if (cmd.equals("Display user block as")) {
int type = 0;
String typeName = (String)((JComboBox)source).getSelectedItem();
jamButton.setEnabled(false);
userBlockArea.setEditable(false);
if (typeName.equalsIgnoreCase("Text"))
{
type = 0;
jamButton.setEnabled(true);
userBlockArea.setEditable(true);
}
else if (typeName.equalsIgnoreCase("Binary"))
{
type = 2;
}
else if (typeName.equalsIgnoreCase("Octal"))
{
type = 8;
}
else if (typeName.equalsIgnoreCase("Hexadecimal"))
{
type = 16;
}
else if (typeName.equalsIgnoreCase("Decimal"))
{
type = 10;
}
showUserBlockAs(type);
}
}
/** returns the data object displayed in this data viewer */
public HObject getDataObject() {
return hObject;
}
/** Disposes of this dataobserver. */
public void dispose() {
super.dispose();
}
/** add an attribute to a data object.*/
public Attribute addAttribute(HObject obj) {
if (obj == null) {
return null;
}
DefaultMutableTreeNode node = (DefaultMutableTreeNode)obj.getFileFormat().getRootNode();
NewAttributeDialog dialog = new NewAttributeDialog(this, obj, node.breadthFirstEnumeration());
dialog.setVisible(true);
Attribute attr = dialog.getAttribute();
if (attr == null) {
return null;
}
String rowData[] = new String[4]; // name, value, type, size
boolean isUnsigned = false;
rowData[0] = attr.getName();
rowData[2] = attr.getType().getDatatypeDescription();
isUnsigned = attr.getType().isUnsigned();
rowData[1] = attr.toString(", ");
long dims[] = attr.getDataDims();
rowData[3] = String.valueOf(dims[0]);
for (int j=1; j 0)
{
String rowData[][] = new String[n][3];
String names[] = compound.getMemberNames();
Datatype types[] = compound.getMemberTypes();
int orders[] = compound.getMemberOrders();
System.out.println("################# "+names);System.out.flush();
System.out.println("################# "+types);System.out.flush();
System.out.println("################# "+orders);System.out.flush();
for (int i=0; i 0)
} // if (d instanceof Compound)
// add compression and data lauoyt information
//try { d.getMetadata(); } catch (Exception ex) {}
String chunkInfo = "";
long[] chunks = d.getChunkSize();
if (chunks == null) {
chunkInfo = "NONE";
} else
{
int n = chunks.length;
chunkInfo = String.valueOf(chunks[0]);
for (int i=1; i= 0 ) {
NT = cName.charAt(cIndex+1);
}
boolean isUnsigned = attr.isUnsigned();
double d = 0;
String theToken = null;
long max=0, min=0;
for (int i=0; i max) || (d < min)) {
JOptionPane.showMessageDialog(
getOwner(),
"Data is out of range["+min+", "+max+"]: "+newValue,
getTitle(),
JOptionPane.ERROR_MESSAGE);
} else {
Array.setByte (data, i, (byte)d);
}
break;
}
case 'S':
{
if (isUnsigned)
{
min = 0;
max = 65535;
}
else
{
min = Short.MIN_VALUE;
max = Short.MAX_VALUE;
}
if ((d > max) || (d < min)) {
JOptionPane.showMessageDialog(
getOwner(),
"Data is out of range["+min+", "+max+"]: "+newValue,
getTitle(),
JOptionPane.ERROR_MESSAGE);
} else {
Array.setShort (data, i, (short)d);
}
break;
}
case 'I':
{
if (isUnsigned)
{
min = 0;
max = 4294967295L;
}
else
{
min = Integer.MIN_VALUE;
max = Integer.MAX_VALUE;
}
if ((d > max) || (d < min)) {
JOptionPane.showMessageDialog(
getOwner(),
"Data is out of range["+min+", "+max+"]: "+newValue,
getTitle(),
JOptionPane.ERROR_MESSAGE);
} else {
Array.setInt (data, i, (int)d);
}
break;
}
case 'J':
Array.setLong (data, i, (long)d); break;
case 'F':
Array.setFloat (data, i, (float)d); break;
case 'D':
default: Array.set (data, i, newValue); break;
}
}
try {
hObject.getFileFormat().writeAttribute(hObject, attr, true);
} catch (Exception ex)
{
JOptionPane.showMessageDialog(
getOwner(),
ex.getMessage(),
getTitle(),
JOptionPane.ERROR_MESSAGE);
return;
}
// update the attribute table
attrTable.setValueAt(attr.toString(", "), row, 1);
}
private void writeUserBlock()
{
if (!isH5) {
return;
}
int blkSize0 = 0;
if (userBlock != null)
{
blkSize0 = userBlock.length;
// The super block space is allocated by offset 0, 512, 1024, 2048, etc
if (blkSize0>0)
{
int offset = 512;
while (offset < blkSize0) {
offset *= 2;
}
blkSize0 = offset;
}
}
int blkSize1 = 0;
String userBlockStr = userBlockArea.getText();
if (userBlockStr == null )
{
if (blkSize0<=0) {
return; // nothing to write
} else {
userBlockStr = " "; // want to wipe out old userblock content
}
}
byte buf[] = null;
buf = userBlockStr.getBytes();
blkSize1 = buf.length;
if (blkSize1 <= blkSize0)
{
java.io.RandomAccessFile raf = null;
try { raf = new java.io.RandomAccessFile(hObject.getFile(), "rw"); }
catch (Exception ex) {
JOptionPane.showMessageDialog(
this,
"Can't open output file: "+hObject.getFile(),
getTitle(),
JOptionPane.ERROR_MESSAGE);
return;
}
try {
raf.seek(0);
raf.write(buf, 0, buf.length);
raf.seek(buf.length);
if (blkSize0 > buf.length)
{
byte[] padBuf = new byte[blkSize0-buf.length];
raf.write(padBuf, 0, padBuf.length);
}
} catch (Exception ex) {}
try { raf.close();} catch (Exception ex) {}
JOptionPane.showMessageDialog(
this,
"Saving user block is successful.",
getTitle(),
JOptionPane.INFORMATION_MESSAGE);
}
else
{
// must rewrite the whole file
// must rewrite the whole file
int op = JOptionPane.showConfirmDialog(this,
"The user block to write is "+blkSize1+" (bytes),\n"+
"which is larger than the user block space in file "+blkSize0+" (bytes).\n"+
"To expand the user block, the file must be rewriten.\n\n"+
"Do you want to replace the current file? Click "+
"\n\"Yes\" to replace the current file,"+
"\n\"No\" to save to a different file, "+
"\n\"Cancel\" to quit without saving the change.\n\n ",
getTitle(),
JOptionPane.YES_NO_CANCEL_OPTION,
JOptionPane.WARNING_MESSAGE);
if (op == JOptionPane.CANCEL_OPTION) {
return;
}
String fin = hObject.getFile();
String fout = fin+"~copy.h5";
if (fin.endsWith(".h5")) {
fout = fin.substring(0, fin.length()-3)+"~copy.h5";
} else if (fin.endsWith(".hdf5")) {
fout = fin.substring(0, fin.length()-5)+"~copy.h5";
}
File outFile = null;
if (op == JOptionPane.NO_OPTION)
{
JFileChooser fchooser = new JFileChooser();
fchooser.setFileFilter(DefaultFileFilter.getFileFilterHDF5());
fchooser.setSelectedFile(new File(fout));
int returnVal = fchooser.showSaveDialog(this);
if(returnVal != JFileChooser.APPROVE_OPTION) {
return;
}
File choosedFile = fchooser.getSelectedFile();
if (choosedFile == null) {
return ;
}
outFile = choosedFile;
fout = outFile.getAbsolutePath();
}
else
{
outFile = new File(fout);
}
if (!outFile.exists())
{
try { outFile.createNewFile(); }
catch (Exception ex)
{
JOptionPane.showMessageDialog(
this,
"Fail to write user block into file. ",
getTitle(),
JOptionPane.ERROR_MESSAGE);
return;
}
}
// close the file
ActionEvent e = new ActionEvent(this, ActionEvent.ACTION_PERFORMED, "Close file");
((HDFView)viewer).actionPerformed(e);
if (DefaultFileFilter.setHDF5UserBlock(fin, fout, buf))
{
if (op == JOptionPane.NO_OPTION) {
fin = fout; // open the new file
} else
{
File oldFile = new File(fin);
boolean status = oldFile.delete();
if (status) {
outFile.renameTo(oldFile);
} else
{
JOptionPane.showMessageDialog(
this,
"Cannot replace the current file.\nPlease save to a different file.",
getTitle(),
JOptionPane.ERROR_MESSAGE);
outFile.delete();
}
}
}
else
{
JOptionPane.showMessageDialog(
this,
"Fail to write user block into file. ",
getTitle(),
JOptionPane.ERROR_MESSAGE);
outFile.delete();
}
// reopen the file
dispose();
e = new ActionEvent(this, ActionEvent.ACTION_PERFORMED, "Open file://"+fin);
((HDFView)viewer).actionPerformed(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy