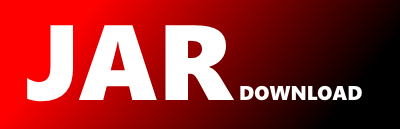
ncsa.hdf.view.HDFView Maven / Gradle / Ivy
Show all versions of hdf-java Show documentation
/*****************************************************************************
* Copyright by The HDF Group. *
* Copyright by the Board of Trustees of the University of Illinois. *
* All rights reserved. *
* *
* This file is part of the HDF Java Products distribution. *
* The full copyright notice, including terms governing use, modification, *
* and redistribution, is contained in the files COPYING and Copyright.html. *
* COPYING can be found at the root of the source code distribution tree. *
* Or, see http://hdfgroup.org/products/hdf-java/doc/Copyright.html. *
* If you do not have access to either file, you may request a copy from *
* [email protected]. *
****************************************************************************/
package ncsa.hdf.view;
import ncsa.hdf.object.*;
import javax.swing.*;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.dnd.DnDConstants;
import java.awt.dnd.DropTarget;
import java.awt.dnd.DropTargetDragEvent;
import java.awt.dnd.DropTargetDropEvent;
import java.awt.dnd.DropTargetEvent;
import java.awt.dnd.DropTargetListener;
import java.awt.event.*;
import java.io.*;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.util.*;
import javax.swing.event.*;
import java.net.URL;
import java.lang.reflect.*;
import java.awt.Event;
import java.awt.Insets;
import java.awt.Dimension;
import java.awt.Component;
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.Toolkit;
import java.awt.Image;
import java.awt.Font;
/**
* HDFView is the main class of this HDF visual tool.
* It is used to layout the graphical components of the hdfview. The major GUI
* components of the HDFView include Menubar, Toolbar, TreeView, ContentView,
* and MessageArea.
*
* The HDFView is designed in such a way that it does not have direct access to
* the HDF library. All the HDF library access is done through HDF objects.
* Therefore, the HDFView package depends on the object package but not the
* library package. The source code of the view package (ncsa.hdf.view) should
* be complied with the library package (ncsa.hdf.hdflib and ncsa.hdf.hdf5lib).
*
* @author Peter X. Cao
* @version 2.4 9/6/2007
*/
public class HDFView extends JFrame implements ViewManager, ActionListener,
ChangeListener, DropTargetListener
{
public static final long serialVersionUID = HObject.serialVersionUID;
/** a list of tree view implementation. */
private static List treeViews;
/** a list of image view implementation. */
private static List imageViews;
/** a list of tree table implementation. */
private static List tableViews;
/** a list of Text view implementation. */
private static List textViews;
/** a list of metadata view implementation. */
private static List metaDataViews;
/** a list of palette view implementation. */
private static List paletteViews;
/** a list of help view implementation. */
private static List helpViews;
private static final String aboutHDFView =
"HDF Viewer, "+ "Version "+ViewProperties.VERSION+"\n"+
"For "+System.getProperty("os.name")+"\n\n"+
"Copyright "+'\u00a9'+" 2006-2010 The HDF Group.\n"+
"All rights reserved.";
private static final String JAVA_COMPILER = "jdk 1.6.0";
/** the directory where the HDFView is installed */
private String rootDir;
/** the current working directory */
private String currentDir;
/** the current working file */
private String currentFile;
/** the view properties */
private ViewProperties props;
/** the list of most recent files */
//private Vector recentFiles;
/** GUI component: the TreeView */
private TreeView treeView;
/** The offset when a new dataview is added into the main window. */
private int frameOffset;
/** GUI component: the panel which is used to display the data content */
private final JDesktopPane contentPane;
/** GUI component: the text area for showing status message */
private final JTextArea statusArea;
/** GUI component: the text area for quick attribute view */
private final JTextArea attributeArea;
/* create tab pane to display attributes and status information */
private final JTabbedPane infoTabbedPane;
/** the main menu bar */
private JMenuBar menuBar;
/** GUI component: a list of current data windwos */
private final JMenu windowMenu;
/** GUI component: file menu on the menubar */
private final JMenu fileMenu;
/** the string buffer holding the status message */
private final StringBuffer message;
/** the string buffer holding the meadata information */
private final StringBuffer metadata;
private final Toolkit toolkit;
/** The list of GUI components related to editing */
private final List editGUIs;
/** The list of GUI components related to HDF5 */
private final List h5GUIs;
/** The list of GUI components related to HDF4 */
private final List h4GUIs;
/** to add and display url */
private JComboBox urlBar;
private UserOptionsDialog userOptionDialog;
private Constructor ctrSrbFileDialog = null;
private JDialog srbFileDialog = null;
/**
* Constructs the HDFView with a given root directory, where the
* HDFView is installed, and opens the given file in the viewer.
*
* @param root the directory where the HDFView is installed.
* @param flist a list of files to open.
*/
public HDFView(String root, List flist, int width, int height, int x, int y)
{
super("HDFView");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
// set the module class jar files to the class path
rootDir = root;
currentFile = null;
frameOffset = 0;
userOptionDialog = null;
ctrSrbFileDialog = null;
toolkit = Toolkit.getDefaultToolkit();
ViewProperties.loadIcons(rootDir);
ViewProperties.loadExtClass();
editGUIs = new Vector();
h4GUIs = new Vector();
h5GUIs = new Vector();
// load the view properties
props = new ViewProperties(rootDir);
try { props.load();} catch (Exception ex){;}
//recentFiles = ViewProperties.getMRF();
currentDir = ViewProperties.getWorkDir();
if (currentDir == null) {
currentDir = System.getProperty("user.dir");
}
treeViews = ViewProperties.getTreeViewList();
metaDataViews = ViewProperties.getMetaDataViewList();
textViews = ViewProperties.getTextViewList();
tableViews = ViewProperties.getTableViewList();
imageViews = ViewProperties.getImageViewList();
paletteViews = ViewProperties.getPaletteViewList();
helpViews = ViewProperties.getHelpViewList();
// initialize GUI components
statusArea = new JTextArea();
statusArea.setEditable(false);
statusArea.setBackground(new java.awt.Color(240, 240, 240));
statusArea.setLineWrap(true);
message = new StringBuffer();
metadata = new StringBuffer();
showStatus("HDFView root - "+rootDir);
showStatus("User property file - "+ViewProperties.getPropertyFile());
attributeArea = new JTextArea();
attributeArea.setEditable(false);
attributeArea.setBackground(new java.awt.Color(240, 240, 240));
attributeArea.setLineWrap(true);
// create tab pane to display attributes and status information
infoTabbedPane = new JTabbedPane(JTabbedPane.BOTTOM);
infoTabbedPane.addChangeListener(this);
contentPane = new JDesktopPane();
windowMenu = new JMenu( "Window" );
fileMenu = new JMenu( "File" );
int n = treeViews.size();
Class theClass = null;
for (int i=0; i
* ||=========||=============================||
* || || ||
* || || ||
* || TreeView|| ContentPane ||
* || || ||
* ||=========||=============================||
* || Message Area ||
* ||========================================||
*
*/
private void createMainWindow(int width, int height, int x, int y)
{
// create splitpane to separate treeview and the contentpane
JScrollPane treeScroller = new JScrollPane((Component)treeView);
JScrollPane contentScroller = new JScrollPane(contentPane);
JSplitPane topSplitPane = new JSplitPane(
JSplitPane.HORIZONTAL_SPLIT,
treeScroller,
contentScroller);
topSplitPane.setDividerLocation(200);
infoTabbedPane.addTab("Log Info", new JScrollPane(statusArea));
infoTabbedPane.addTab("Metadata ", new JScrollPane(attributeArea));
infoTabbedPane.setSelectedIndex(1);
// create splitpane to separate message area and treeview-contentpane
topSplitPane.setBorder(null); // refer to Java bug #4131528
JSplitPane splitPane = new JSplitPane(
JSplitPane.VERTICAL_SPLIT,
topSplitPane,
infoTabbedPane);
// set the window size
//float inset = 0.17f; // for UG only.
float inset = 0.04f;
Dimension d = toolkit.getScreenSize();
if (height > 300) {
d.height = height;
} else {
d.height = (int)((1-2*inset)*d.height);
}
if (width > 300) {
d.width = width;
} else {
d.width = (int)(0.9*(double)d.height);
}
// TEST
if (treeView.getClass().getName().startsWith("ext.erdc")) {
topSplitPane.setDividerLocation(500);
d.width = (int)(0.9*toolkit.getScreenSize().width);
d.height = (int)(d.width*0.618);
}
splitPane.setDividerLocation(d.height-180);
this.setLocation(x, y);
try {
this.setIconImage(((ImageIcon)ViewProperties.getHdfIcon()).getImage());
} catch (Exception ex ) {}
this.setJMenuBar(menuBar = createMenuBar());
JToolBar toolBar = createToolBar();
/** create URL address bar */
urlBar = new JComboBox(ViewProperties.getMRF());
urlBar.setMaximumRowCount(ViewProperties.MAX_RECENT_FILES);
urlBar.setEditable(true);
urlBar.addActionListener(this);
urlBar.setActionCommand("Open file: from file bar");
urlBar.setSelectedIndex(-1);
JPanel urlPane = new JPanel();
urlPane.setLayout(new BorderLayout());
JButton b = new JButton("File/URL");
urlPane.add(b, BorderLayout.WEST);
b.addActionListener(this);
b.setActionCommand("Popup URL list");
urlPane.add(urlBar, BorderLayout.CENTER);
JPanel toolPane = new JPanel();
toolPane.setLayout(new GridLayout(2,1,0,0));
toolPane.add(toolBar);
toolPane.add(urlPane);
JPanel mainPane = (JPanel)getContentPane();
mainPane.setLayout(new BorderLayout());
mainPane.add(toolPane, BorderLayout.NORTH);
mainPane.add(splitPane, BorderLayout.CENTER);
mainPane.setPreferredSize(d);
}
private JMenuBar createMenuBar()
{
JMenuBar mbar = new JMenuBar();
JMenu menu = null;
JMenuItem item;
// add file menu
fileMenu.setMnemonic('f');
mbar.add(fileMenu);
item = new JMenuItem( "Open");
item.setMnemonic(KeyEvent.VK_O);
item.addActionListener(this);
item.setActionCommand("Open file");
item.setAccelerator( KeyStroke.getKeyStroke(KeyEvent.VK_O, Event.CTRL_MASK, true));
fileMenu.add(item);
item = new JMenuItem( "Open Read-Only");
item.setMnemonic(KeyEvent.VK_R);
item.addActionListener(this);
item.setActionCommand("Open file read-only");
if (!ViewProperties.isReadOnly())
fileMenu.add(item);
// boolean isSrbSupported = true;
// try {
// Class.forName("ncsa.hdf.srb.H5SRB");
// Class.forName("ncsa.hdf.srb.SRBFileDialog");
// } catch (Throwable ex) {isSrbSupported = false;}
//
// if (isSrbSupported) {
// item = new JMenuItem( "Open from iRODS");
// item.setMnemonic(KeyEvent.VK_S);
// item.addActionListener(this);
// item.setActionCommand("Open from irods");
// fileMenu.add(item);
// }
fileMenu.addSeparator();
JMenu newFileMenu = new JMenu("New");
item = new JMenuItem( "HDF4");
item.setActionCommand("New HDF4 file");
item.setMnemonic(KeyEvent.VK_4);
item.addActionListener(this);
h4GUIs.add(item);
newFileMenu.add(item);
item = new JMenuItem( "HDF5");
item.setActionCommand("New HDF5 file");
item.setMnemonic(KeyEvent.VK_5);
item.addActionListener(this);
h5GUIs.add(item);
newFileMenu.add(item);
fileMenu.add(newFileMenu);
fileMenu.addSeparator();
item = new JMenuItem( "Close");
item.setMnemonic(KeyEvent.VK_C);
item.addActionListener(this);
item.setActionCommand("Close file");
fileMenu.add(item);
item = new JMenuItem( "Close All");
item.setMnemonic(KeyEvent.VK_A);
item.addActionListener(this);
item.setActionCommand("Close all file");
fileMenu.add(item);
fileMenu.addSeparator();
item = new JMenuItem( "Save");
item.setMnemonic(KeyEvent.VK_S);
item.addActionListener(this);
item.setActionCommand("Save current file");
fileMenu.add(item);
item = new JMenuItem( "Save As");
item.setMnemonic(KeyEvent.VK_A);
item.addActionListener(this);
item.setActionCommand("Save current file as");
fileMenu.add(item);
fileMenu.addSeparator();
item = new JMenuItem( "Exit");
item.setMnemonic(KeyEvent.VK_X);
item.addActionListener(this);
item.setActionCommand("Exit");
item.setAccelerator(
KeyStroke.getKeyStroke(KeyEvent.VK_Q, Event.CTRL_MASK, true));
fileMenu.add(item);
fileMenu.addSeparator();
// add window menu
windowMenu.setMnemonic('w');
mbar.add(windowMenu);
item = new JMenuItem( "Cascade");
item.setMnemonic(KeyEvent.VK_C);
item.setActionCommand("Cascade all windows");
item.addActionListener(this);
windowMenu.add(item);
item = new JMenuItem( "Tile");
item.setMnemonic(KeyEvent.VK_T);
item.setActionCommand("Tile all windows");
item.addActionListener(this);
windowMenu.add(item);
windowMenu.addSeparator();
item = new JMenuItem( "Close Window");
item.setMnemonic(KeyEvent.VK_W);
item.setActionCommand("Close a window");
item.addActionListener(this);
windowMenu.add(item);
item = new JMenuItem( "Close All");
item.setMnemonic(KeyEvent.VK_A);
item.setActionCommand("Close all windows");
item.addActionListener(this);
windowMenu.add(item);
windowMenu.addSeparator();
// add tool menu
menu = new JMenu( "Tools" );
menu.setMnemonic('T');
mbar.add(menu);
JMenu imageSubmenu = new JMenu("Convert Image To");
item = new JMenuItem( "HDF4");
item.setActionCommand("Convert image file: Image to HDF4");
item.addActionListener(this);
h4GUIs.add(item);
imageSubmenu.add(item);
item = new JMenuItem( "HDF5");
item.setActionCommand("Convert image file: Image to HDF5");
item.addActionListener(this);
h5GUIs.add(item);
imageSubmenu.add(item);
menu.add(imageSubmenu);
menu.addSeparator();
item = new JMenuItem( "User Options");
item.setMnemonic(KeyEvent.VK_O);
item.setActionCommand("User options");
item.addActionListener(this);
menu.add(item);
menu.addSeparator();
item = new JMenuItem( "Register File Format");
item.setMnemonic(KeyEvent.VK_R);
item.setActionCommand("Register file format");
item.addActionListener(this);
menu.add(item);
item = new JMenuItem( "Unregister File Format");
item.setMnemonic(KeyEvent.VK_U);
item.setActionCommand("Unregister file format");
item.addActionListener(this);
menu.add(item);
// add help menu
menu = new JMenu("Help");
menu.setMnemonic('H');
mbar.add(menu);
item = new JMenuItem( "User's Guide");
item.setMnemonic(KeyEvent.VK_U);
item.setActionCommand("Users guide");
item.addActionListener(this);
menu.add(item);
menu.addSeparator();
if ((helpViews != null) && (helpViews.size() > 0))
{
int n = helpViews.size();
for (int i=0; i