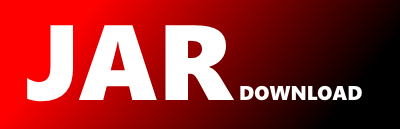
org.hdiv.context.jsf1.support.ExternalContextWrapper Maven / Gradle / Ivy
Show all versions of hdiv-jsf Show documentation
/**
* Copyright 2005-2016 hdiv.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.hdiv.context.jsf1.support;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URL;
import java.security.Principal;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import javax.faces.context.ExternalContext;
public abstract class ExternalContextWrapper extends ExternalContext {
// ----------------------------------------------- Methods from FacesWrapper
/**
* @return the wrapped {@link ExternalContext} instance
* @see javax.faces.FacesWrapper#getWrapped()
*/
public abstract ExternalContext getWrapped();
// -------------------------------------------- Methods from ExternalContext
/**
*
* The default behavior of this method is to call {@link ExternalContext#dispatch(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#dispatch(String)
*/
public void dispatch(String path) throws IOException {
getWrapped().dispatch(path);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#encodeActionURL(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#encodeActionURL(String)
*/
public String encodeActionURL(String url) {
return getWrapped().encodeActionURL(url);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#encodeNamespace(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#encodeNamespace(String)
*/
public String encodeNamespace(String name) {
return getWrapped().encodeNamespace(name);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#encodeResourceURL(String)} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#encodeResourceURL(String)
*/
public String encodeResourceURL(String url) {
return getWrapped().encodeResourceURL(url);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getApplicationMap} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getApplicationMap()
*/
public Map getApplicationMap() {
return getWrapped().getApplicationMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getAuthType} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getAuthType()
*/
public String getAuthType() {
return getWrapped().getAuthType();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getContext} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getContext()
*/
public Object getContext() {
return getWrapped().getContext();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getInitParameter(String)} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getInitParameter(String)
*/
public String getInitParameter(String name) {
return getWrapped().getInitParameter(name);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getInitParameterMap} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getInitParameterMap()
*/
public Map getInitParameterMap() {
return getWrapped().getInitParameterMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRemoteUser} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRemoteUser()
*/
public String getRemoteUser() {
return getWrapped().getRemoteUser();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequest} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequest()
*/
public Object getRequest() {
return getWrapped().getRequest();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestContextPath} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestContextPath()
*/
public String getRequestContextPath() {
return getWrapped().getRequestContextPath();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestCookieMap} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestCookieMap()
*/
public Map getRequestCookieMap() {
return getWrapped().getRequestCookieMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestHeaderMap} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestHeaderMap()
*/
public Map getRequestHeaderMap() {
return getWrapped().getRequestHeaderMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestHeaderValuesMap} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequestHeaderValuesMap()
*/
public Map getRequestHeaderValuesMap() {
return getWrapped().getRequestHeaderValuesMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestLocale} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestLocale()
*/
public Locale getRequestLocale() {
return getWrapped().getRequestLocale();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestLocales} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestLocales()
*/
public Iterator getRequestLocales() {
return getWrapped().getRequestLocales();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestMap} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequestMap()
*/
public Map getRequestMap() {
return getWrapped().getRequestMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestParameterMap} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestParameterMap()
*/
public Map getRequestParameterMap() {
return getWrapped().getRequestParameterMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestParameterNames} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequestParameterNames()
*/
public Iterator getRequestParameterNames() {
return getWrapped().getRequestParameterNames();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestParameterValuesMap} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequestParameterValuesMap()
*/
public Map getRequestParameterValuesMap() {
return getWrapped().getRequestParameterValuesMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestPathInfo} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestPathInfo()
*/
public String getRequestPathInfo() {
return getWrapped().getRequestPathInfo();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestServletPath} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestServletPath()
*/
public String getRequestServletPath() {
return getWrapped().getRequestServletPath();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResource(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getResource(String)
*/
public URL getResource(String path) throws MalformedURLException {
return getWrapped().getResource(path);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResourceAsStream(String)} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getResourceAsStream(String)
*/
public InputStream getResourceAsStream(String path) {
return getWrapped().getResourceAsStream(path);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResourcePaths(String)} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getResourcePaths(String)
*/
public Set getResourcePaths(String path) {
return getWrapped().getResourcePaths(path);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResponse} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getResponse()
*/
public Object getResponse() {
return getWrapped().getResponse();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getSession(boolean)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getSession(boolean)
*/
public Object getSession(boolean create) {
return getWrapped().getSession(create);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getAuthType} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getAuthType()
*/
public Map getSessionMap() {
return getWrapped().getSessionMap();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getUserPrincipal} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getUserPrincipal()
*/
public Principal getUserPrincipal() {
return getWrapped().getUserPrincipal();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#isUserInRole(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#isUserInRole(String)
*/
public boolean isUserInRole(String role) {
return getWrapped().isUserInRole(role);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#log(String)} on the wrapped {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#log(String)
*/
public void log(String message) {
getWrapped().log(message);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#log(String, Throwable)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#log(String, Throwable)
*/
public void log(String message, Throwable exception) {
getWrapped().log(message, exception);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#redirect(String)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#redirect(String)
*/
public void redirect(String url) throws IOException {
getWrapped().redirect(url);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#setRequest(Object)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#setRequest(Object)
*/
public void setRequest(Object request) {
getWrapped().setRequest(request);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#setRequestCharacterEncoding(String)} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#setRequestCharacterEncoding(String)
*/
public void setRequestCharacterEncoding(String encoding) throws UnsupportedEncodingException {
getWrapped().setRequestCharacterEncoding(encoding);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestCharacterEncoding} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getRequestCharacterEncoding()
*/
public String getRequestCharacterEncoding() {
return getWrapped().getRequestCharacterEncoding();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getRequestContentType} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getRequestContentType()
*/
public String getRequestContentType() {
return getWrapped().getRequestContentType();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResponseCharacterEncoding} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getResponseCharacterEncoding()
*/
public String getResponseCharacterEncoding() {
return getWrapped().getResponseCharacterEncoding();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResponseContentType} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#getResponseContentType()
*/
public String getResponseContentType() {
return getWrapped().getResponseContentType();
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#setResponse(Object)} on the wrapped {@link ExternalContext}
* object.
*
*
* @see javax.faces.context.ExternalContext#setResponse(Object)
*/
public void setResponse(Object response) {
getWrapped().setResponse(response);
}
/**
*
* The default behavior of this method is to call {@link ExternalContext#getResponseCharacterEncoding} on the wrapped
* {@link ExternalContext} object.
*
*
* @see javax.faces.context.ExternalContext#getResponseCharacterEncoding()
*/
public void setResponseCharacterEncoding(String encoding) {
getWrapped().setResponseCharacterEncoding(encoding);
}
}