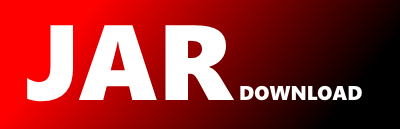
org.heigit.bigspatialdata.oshdb.api.db.OSHDBIgnite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oshdb-api Show documentation
Show all versions of oshdb-api Show documentation
API to query the OpenStreetMap History Database. Includes MapReduce functionality to filter, analyze and aggregate data.
package org.heigit.bigspatialdata.oshdb.api.db;
import com.google.common.base.Joiner;
import java.io.File;
import java.util.Collection;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.apache.ignite.Ignite;
import org.apache.ignite.Ignition;
import org.apache.ignite.lang.IgniteRunnable;
import org.heigit.bigspatialdata.oshdb.api.mapreducer.MapReducer;
import org.heigit.bigspatialdata.oshdb.api.mapreducer.backend.MapReducerIgniteAffinityCall;
import org.heigit.bigspatialdata.oshdb.api.mapreducer.backend.MapReducerIgniteLocalPeek;
import org.heigit.bigspatialdata.oshdb.api.mapreducer.backend.MapReducerIgniteScanQuery;
import org.heigit.bigspatialdata.oshdb.api.object.OSHDBMapReducible;
import org.heigit.bigspatialdata.oshdb.osm.OSMType;
import org.heigit.bigspatialdata.oshdb.util.TableNames;
import org.heigit.bigspatialdata.oshdb.util.exceptions.OSHDBTableNotFoundException;
/**
* OSHDB database backend connector to a Ignite system.
*/
public class OSHDBIgnite extends OSHDBDatabase implements AutoCloseable {
public enum ComputeMode {
LocalPeek,
ScanQuery,
AffinityCall
}
private final transient Ignite ignite;
private ComputeMode computeMode = ComputeMode.LocalPeek;
private IgniteRunnable onCloseCallback = null;
public OSHDBIgnite() {
this(new File("ignite-config.xml"));
}
public OSHDBIgnite(Ignite ignite) {
this.ignite = ignite;
}
public OSHDBIgnite(String igniteConfigFilePath) {
this(new File(igniteConfigFilePath));
}
/**
* Opens a connection to oshdb data stored on an Ignite cluster.
*
* @param igniteConfig ignite configuration file
*/
public OSHDBIgnite(File igniteConfig) {
Ignition.setClientMode(true);
this.ignite = Ignition.start(igniteConfig.toString());
}
@Override
public OSHDBIgnite prefix(String prefix) {
return (OSHDBIgnite) super.prefix(prefix);
}
@Override
public MapReducer createMapReducer(Class forClass) {
MapReducer mapReducer;
Collection allCaches = this.getIgnite().cacheNames();
Collection expectedCaches = Stream.of(OSMType.values())
.map(TableNames::forOSMType).filter(Optional::isPresent).map(Optional::get)
.map(t -> t.toString(this.prefix()))
.collect(Collectors.toList());
if (!allCaches.containsAll(expectedCaches)) {
throw new OSHDBTableNotFoundException(Joiner.on(", ").join(expectedCaches));
}
switch (this.computeMode()) {
case LocalPeek:
mapReducer = new MapReducerIgniteLocalPeek(this, forClass);
break;
case ScanQuery:
mapReducer = new MapReducerIgniteScanQuery(this, forClass);
break;
case AffinityCall:
mapReducer = new MapReducerIgniteAffinityCall(this, forClass);
break;
default:
throw new UnsupportedOperationException("Backend not implemented for this compute mode.");
}
return mapReducer;
}
@Override
public String metadata(String property) {
// todo: implement this
return null;
}
public Ignite getIgnite() {
return this.ignite;
}
public void close() {
this.ignite.close();
}
/**
* Sets the compute mode.
*
* @param computeMode the compute mode to be used in calculations on this oshdb backend
* @return this backend
*/
public OSHDBIgnite computeMode(ComputeMode computeMode) {
this.computeMode = computeMode;
return this;
}
/**
* Gets the set compute mode.
*
* @return the currently set compute mode
*/
public ComputeMode computeMode() {
return this.computeMode;
}
/**
* Sets a callback to be executed on all ignite workers after the query has been finished.
*
* This can be used to close connections to (temporary) databases that were used to store or
* retrieve intermediate data.
*
* @param action the callback to execute after a query is done
* @return the current oshdb database object
*/
public OSHDBIgnite onClose(IgniteRunnable action) {
this.onCloseCallback = action;
return this;
}
/**
* Gets the onClose callback.
*
* @return the currently set onClose callback
*/
public Optional onClose() {
if (this.onCloseCallback == null) {
return Optional.empty();
} else {
return Optional.of(this.onCloseCallback);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy