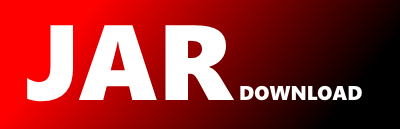
org.heigit.ohsome.oshdb.api.generic.OSHDBCombinedIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oshdb-api Show documentation
Show all versions of oshdb-api Show documentation
API to query the OpenStreetMap History Database. Includes MapReduce functionality to filter, analyze and aggregate data.
The newest version!
package org.heigit.ohsome.oshdb.api.generic;
import java.io.Serializable;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.jetbrains.annotations.NotNull;
/**
* A data type for an index ("map key") consisting of two independent variables.
*
* @param the type of the fist part of the index
* @param the type of the second part of the index
*/
public class OSHDBCombinedIndex<
U extends Comparable & Serializable,
V extends Comparable & Serializable>
implements Comparable>, Serializable {
private final U index1;
private final V index2;
public OSHDBCombinedIndex(U index1, V index2) {
this.index1 = index1;
this.index2 = index2;
}
public U getFirstIndex() {
return this.index1;
}
public V getSecondIndex() {
return this.index2;
}
@Override
public int compareTo(@NotNull OSHDBCombinedIndex other) {
int c = this.index1.compareTo(other.index1);
if (c == 0) {
c = this.index2.compareTo(other.index2);
}
return c;
}
@Override
public boolean equals(Object obj) {
return this == obj || obj instanceof OSHDBCombinedIndex
&& java.util.Objects.equals(this.index1, ((OSHDBCombinedIndex) obj).index1)
&& java.util.Objects.equals(this.index2, ((OSHDBCombinedIndex) obj).index2);
}
@Override
public int hashCode() {
return java.util.Objects.hash(index1, index2);
}
@Override
public String toString() {
return this.getFirstIndex().toString() + "&" + this.getSecondIndex().toString();
}
/**
* Helper function that converts the dual-index data structure returned by aggregation operations
* on this object to a nested Map structure, which can be easier to process further on.
*
* This version creates a map for each <U> index value, containing maps containing
* results by timestamps.
*
* @param result the "flat" result data structure that should be converted to a nested structure
* @param an arbitrary data type, used for the data value items
* @param an arbitrary data type, used for the index'es key items
* @param an arbitrary data type, used for the index'es key items
* @return a nested data structure: for each index part there is a separate level of nested maps
*/
public static & Serializable, V extends Comparable & Serializable>
SortedMap> nest(
Map, A> result
) {
TreeMap> ret = new TreeMap<>();
result.forEach((index, data) -> {
if (!ret.containsKey(index.getFirstIndex())) {
ret.put(index.getFirstIndex(), new TreeMap<>());
}
ret.get(index.getFirstIndex()).put(index.getSecondIndex(), data);
});
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy